The `.find` function in C++ is used to locate the position of a specified substring within a string, returning the starting index of the first occurrence or `std::string::npos` if not found.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::size_t found = str.find("world");
if (found != std::string::npos) {
std::cout << "'world' found at: " << found << std::endl;
} else {
std::cout << "'world' not found" << std::endl;
}
return 0;
}
Understanding the .find Function in C++
What is .find in C++?
The `.find` function in C++ is a powerful string and container manipulation tool that allows developers to locate substrings or elements within a larger data structure. It plays a critical role in data searching and is widely used in various applications, from simple string comparisons to complex data processing tasks. Understanding how to utilize `.find` effectively can greatly enhance your programming capabilities.
Syntax of the .find Function
The general syntax for the `.find` function varies slightly depending on whether you are using it with strings or containers like vectors. However, they share some common parameters:
size_t find(const string& str, size_t position = 0) const;
size_t find(char ch, size_t position = 0) const;
In this syntax:
- `str`: The substring you want to search for.
- `ch`: The character you want to search for.
- `position`: The starting point for the search (default is 0).

Using .find with Strings
Finding Substrings in C++
The most common usage of `.find` is to locate substrings within a string. When you call `.find`, it will return the position of the first occurrence of the substring. If the substring is not found, the function will return `std::string::npos`.
Code Snippet: Basic Example
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
size_t found = str.find("World");
if (found != std::string::npos)
std::cout << "Found 'World' at: " << found << '\n';
return 0;
}
Explanation: In this example, the program searches for the substring "World" in the string "Hello, World!". When found, it returns the index of the starting position (7). If the substring were absent, it would print nothing. The importance of checking against `std::string::npos` cannot be overstated, as this ensures that your program reacts appropriately to the search results.
Searching for Characters
Finding a Single Character
The `.find` function can also locate a single character within a string. It operates similarly to finding substrings, returning the character's position if found.
Code Snippet: Character Search
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char c = 'o';
size_t pos = str.find(c);
if (pos != std::string::npos)
std::cout << "Found 'o' at position: " << pos << '\n';
return 0;
}
In this code, we search for the character 'o'. The position returned will indicate where the first 'o' appears in the string.
Finding the Last Occurrence
If you need to locate the last occurrence of a character or substring, you can utilize the `.rfind()` method, which functions similarly to `.find()` but searches from the end of the string.
Code Snippet: Last Occurrence
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
size_t last_found = str.rfind("o");
if (last_found != std::string::npos)
std::cout << "Last occurrence of 'o' is at position: " << last_found << '\n';
return 0;
}
This code demonstrates how to find the last occurrence of the character 'o'. In this case, it will return 8, which is the position of the 'o' in "World".

Using .find with Containers
Finding Elements in Vectors
In addition to strings, `.find` can be applied when searching for elements within containers, such as vectors. This requires using iterators from the `<algorithm>` library in C++.
Code Snippet: Using find with Vectors
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Found 3 in vector at position: " << std::distance(vec.begin(), it) << '\n';
}
return 0;
}
In this code, we create a vector of integers and search for the number 3. The iterator returned by `std::find` allows us to verify if the number exists and calculate its position by using `std::distance`.

Error Handling with .find
Understanding Return Values
Understanding the return values of `.find` is vital for effective error handling. The function returns `std::string::npos` when it does not find the target substring or character. This return value should always be checked to determine if the search was successful. Utilize conditional statements to manage situations where the sought-after element is not present in the original string or container.
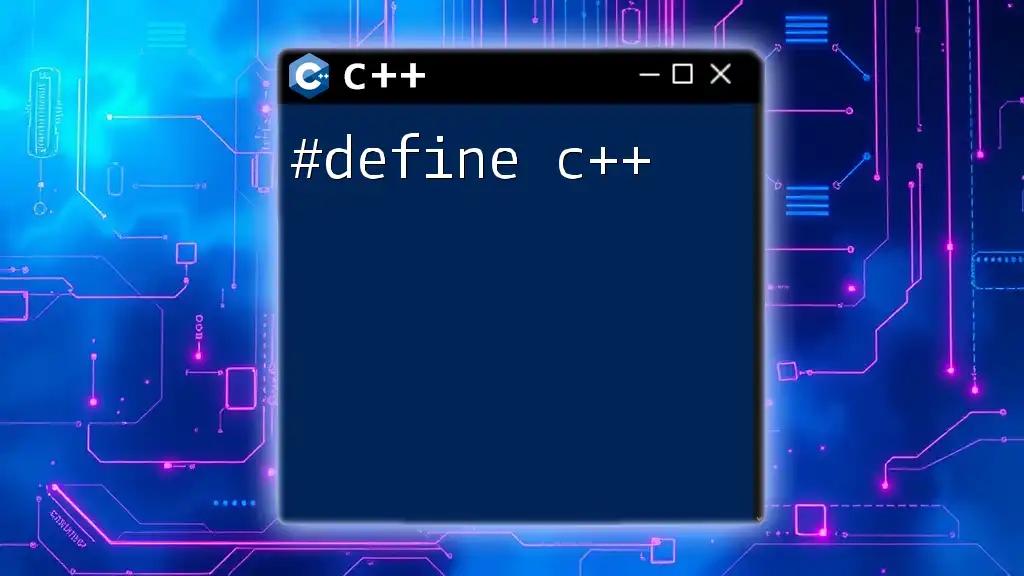
Performance Considerations
Time Complexity of .find
The time complexity of the `.find` function varies depending on the data structure used. For strings, the complexity is O(n), where n is the length of the string, as it may require scanning each character in the worst case. In contrast, using `.find` with sorted containers (like sets or maps) can significantly reduce the worst-case time complexity by leveraging efficient searching algorithms.
Optimizing your approach to data searching can lead to significant performance improvements, particularly in larger datasets. Consider utilizing alternative data types or algorithms if your application requires heavy string manipulation.
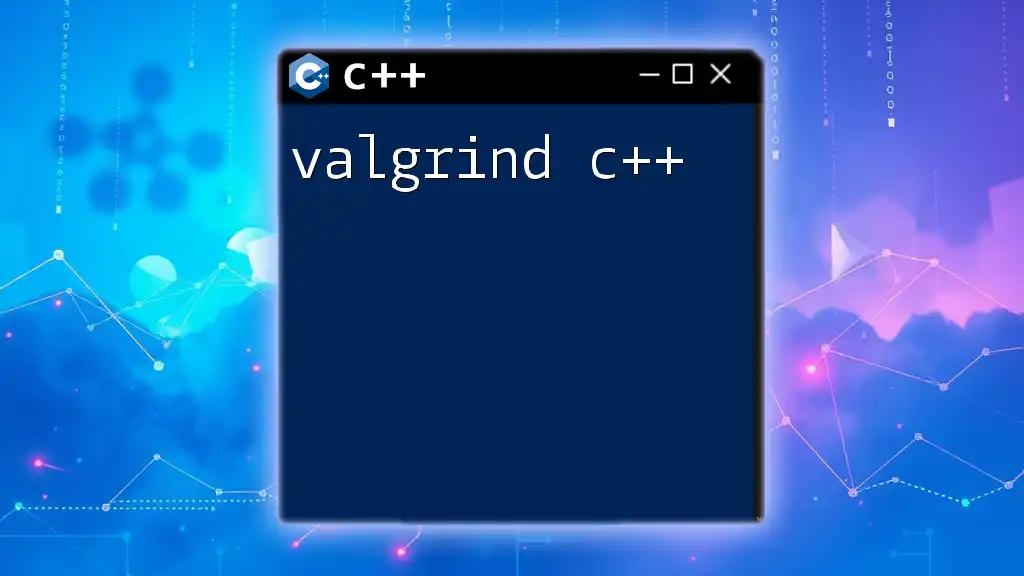
Conclusion
The `.find` function in C++ is an essential tool for developers looking to enhance their string and container manipulation skills. By mastering its syntax, understanding its use cases, and knowing how to handle errors and optimize performance, you can become more efficient in your coding practices. Whether you are searching for a substring or an element within a container, the `.find` method is versatile and integral to efficient C++ programming.
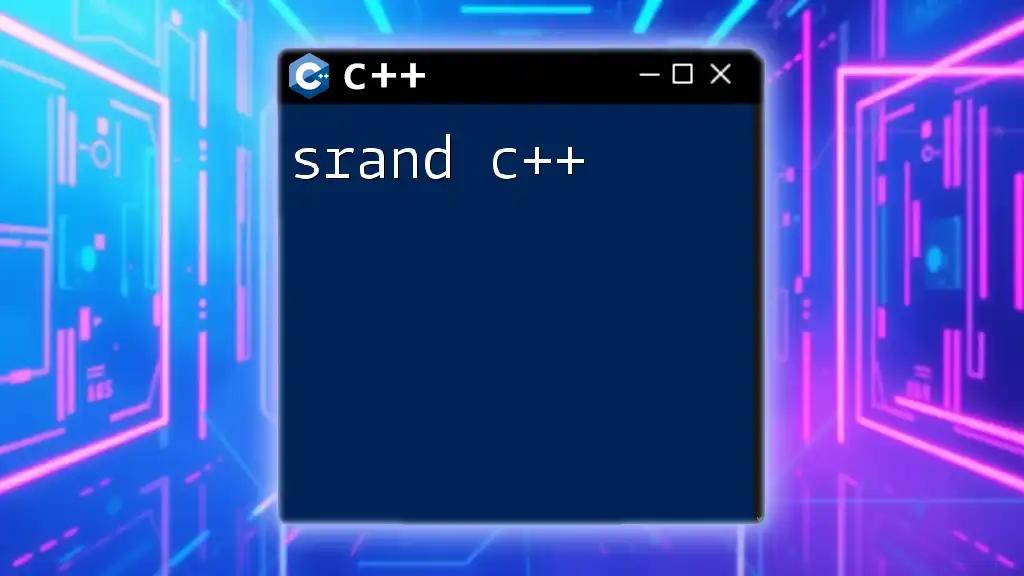
Additional Resources
To continue your learning journey about C++ string and container manipulation, explore additional resources such as official C++ documentation and online tutorials. These materials can provide deeper insights and further examples to solidify your understanding.

FAQs
Feeling stuck? Here are some common questions developers have about using `.find` in C++:
- What if I need to search for multiple characters? Consider using loops or regular expressions for more advanced searching.
- Can I use `.find` with custom data types? You can, but you'll need to ensure that the data type supports comparison operators.
With this comprehensive guide, you are now equipped to effectively utilize the `.find` function in your C++ programming endeavors. Practice various scenarios to deepen your understanding and confidence in using this crucial function.