The `std::find` function in C++ is used to search for a specific element in a range of elements, returning an iterator to the first occurrence or to the end of the range if the element is not found.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
What is std::find?
`std::find` is a powerful function provided by the C++ Standard Library that allows developers to search through a range of elements to locate a specific value. Part of the Standard Template Library (STL), this function simplifies the process of searching by abstracting away the underlying mechanisms and providing a clean, straightforward interface.
Understanding the utility of `std::find` is crucial for effective C++ programming. It not only saves time but also enhances code readability, making it easier to maintain and debug.

Syntax of std::find
The general syntax for `std::find` is as follows:
template<class ForwardIt, class T>
ForwardIt std::find(ForwardIt first, ForwardIt last, const T& value);
This syntax defines a template function that works with iterators.

Parameters Explained
- ForwardIt first: This parameter refers to the beginning of the range in which you want to search. It can be any forward iterator.
- ForwardIt last: This indicates the end of the range (exclusive). The search will not include this position.
- const T& value: This is the value you are searching for within the specified range.
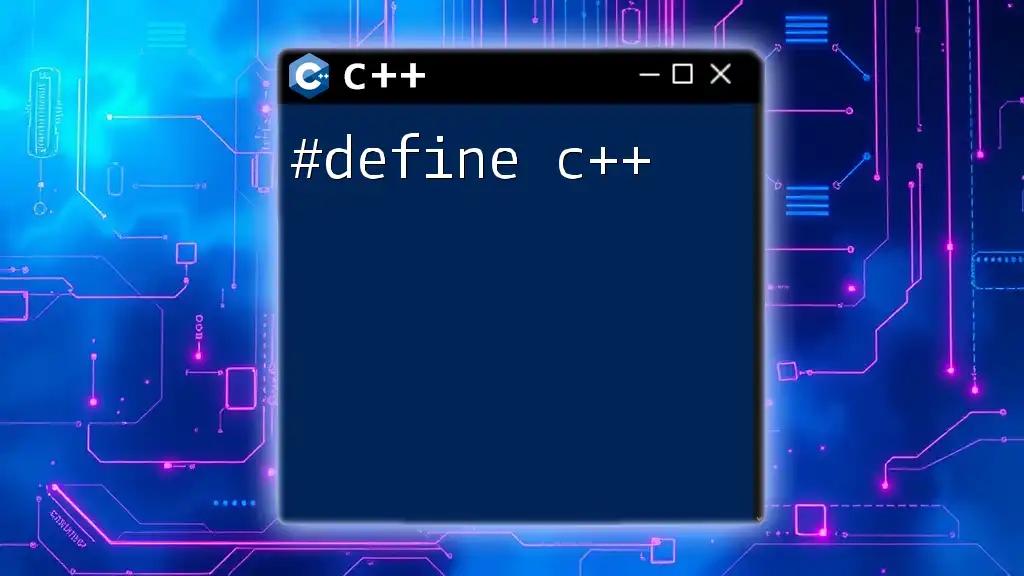
Return Value
The function returns an iterator pointing to the first occurrence of the specified value. If the value is not found, it returns the `last` iterator, indicating that the entire range has been searched without success. Understanding how to effectively utilize this returned iterator is essential for further operations in your code.

Practical Usage of std::find
Basic Example of std::find
Let’s start with a straightforward example demonstrating how `std::find` works with a vector of integers.
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find(numbers.begin(), numbers.end(), 3);
if (it != numbers.end()) {
std::cout << "Found: " << *it << std::endl;
} else {
std::cout << "Not Found" << std::endl;
}
}
In this snippet, we're searching for the number `3` in a vector of integers. If found, a message is printed displaying the found number; otherwise, we inform the user that the number is not present.
More Complex Usage with Custom Types
Searching in a Vector of Objects
Sometimes, you may need to search for a more complex type, such as a user-defined class. Below is an example where we define a simple class and utilize `std::find` to locate an object in a vector.
#include <algorithm>
#include <iostream>
#include <vector>
class Person {
public:
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
bool operator==(const Person& p1, const Person& p2) {
return p1.name == p2.name && p1.age == p2.age;
}
int main() {
std::vector<Person> people = { Person("Alice", 30), Person("Bob", 25) };
Person search_for("Bob", 25);
auto it = std::find(people.begin(), people.end(), search_for);
if (it != people.end()) {
std::cout << "Found: " << it->name << std::endl;
} else {
std::cout << "Not Found" << std::endl;
}
}
In this example, we define a `Person` class and create a vector of `Person` objects. To enable `std::find` to compare `Person` instances, we override the `operator==`, allowing us to check for equality based on the `name` and `age` attributes.

Performance Considerations
Time Complexity of std::find
It is important to recognize that `std::find` operates with linear time complexity, O(n). This means that in the worst-case scenario, it checks each element in the provided range until it finds the target value or reaches the end. Therefore, while `std::find` is very convenient, in cases of large datasets or when performance is critical, it may be more efficient to explore other searching options.
Comparing std::find with Other Search Methods
When optimal performance is a concern, one might consider alternative searching methods, such as binary search. However, binary search requires a sorted range, while `std::find` can operate on any range. Thus, while `std::find` may not be the fastest option, it is often the most versatile, especially for unordered collections.
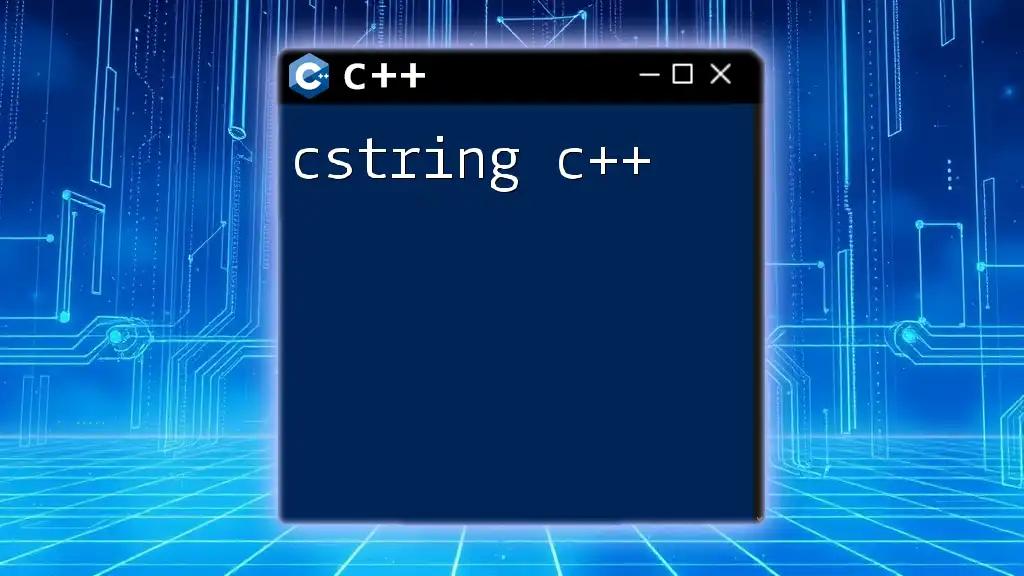
Common Pitfalls and How to Avoid Them
Attempting to Use std::find with Non-Forward Iterators
When using `std::find`, it is crucial that the iterators passed as parameters are forward iterators. If you mistakenly attempt to use reverse iterators or other iterator types that do not fulfill this requirement, you might encounter compilation errors or undefined behavior. Always ensure your iterators are compatible with the function's expectations.
Using std::find with Non-Equality Comparable Types
Another common issue arises when the type being searched does not support equality comparison. If your class does not define `operator==`, the function will fail to find the value, possibly returning an erroneous result. To avoid this pitfall, always ensure that the type being searched has an appropriate equality operator defined.

Conclusion
In summary, `std::find` is an essential tool in the C++ programmer's toolkit, offering simplicity and effectiveness in searching through collections of data. Its straightforward interface allows for quick implementations, while its flexibility ensures it can be utilized across various data types.
Embracing the `std::find` function can enhance your coding efficiency, allowing you to focus on solving more complex problems rather than re-implementing search algorithms. By practicing its usage in different contexts, you will gain a deeper understanding of C++ and the STL.
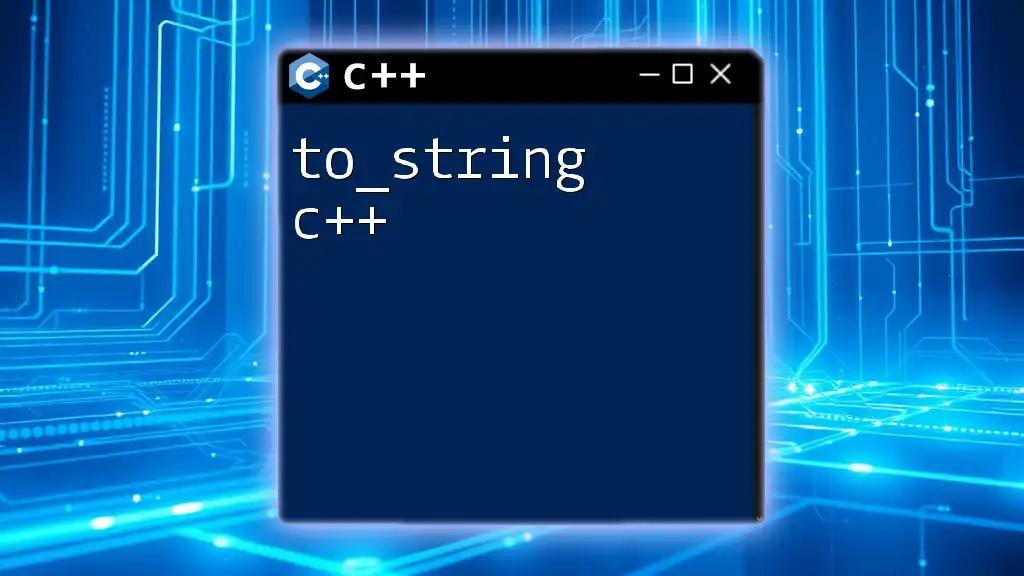
References and Additional Resources
For further exploration of `std::find` and other STL components, you might consider the following resources:
- The official C++ documentation website for STL: [cppreference.com](https://en.cppreference.com/w/cpp/algorithm/find)
- Recommended books such as "The C++ Programming Language" by Bjarne Stroustrup or "Effective C++" by Scott Meyers.
With this knowledge in hand, you are now well-equipped to incorporate `std::find` into your C++ projects effectively!