`const_cast` in C++ is used to add or remove the `const` qualifier from a variable, allowing you to modify a variable that was originally declared as `const`.
#include <iostream>
int main() {
const int x = 10;
int* y = const_cast<int*>(&x); // Remove const-ness
*y = 20; // Modifying const value (undefined behavior)
std::cout << "Modified value: " << *y << std::endl;
return 0;
}
Understanding const_cast
What is const_cast?
`const_cast` is a powerful casting operator introduced in C++ to change the constness of a variable. It allows you to remove the const modifier on a variable, enabling you to convert a pointer or reference of a const type to a non-const type. This operation is particularly useful in situations where you have a constant variable and you need to pass it to a function that requires a non-const pointer or reference.
Purpose and usage of const_cast: The primary purpose of `const_cast` is to enable modifications to constant objects or to enable non-const pointers to point to const data. However, it is essential to use this feature judiciously, as it can lead to undefined behavior if the original object was indeed intended to be constant.
Key differences from other casting operators: Unlike static_cast and dynamic_cast, `const_cast` specifically focuses on modifying the constness of variables. It is crucial not to confuse it with the typical type conversions managed by other casting operators.
Why Use const_cast?
There are several scenarios where using `const_cast` is advantageous. Here are a few notable use cases:
- Legacy Code Integration: When dealing with older libraries or legacy code that requires a non-const pointer.
- Callbacks and Interfaces: When interfacing with APIs that do not maintain const correctness and necessitate mutable pointers.
- Specific Design Patterns: In design patterns that may require mutable access to certain parameters while ensuring the function signature remains as non-const.
Understanding the importance of const correctness in C++ is vital. It prevents manipulation of data that should not change, thereby promoting safer and more maintainable code. Utilizing `const_cast` improperly can undermine this const correctness.
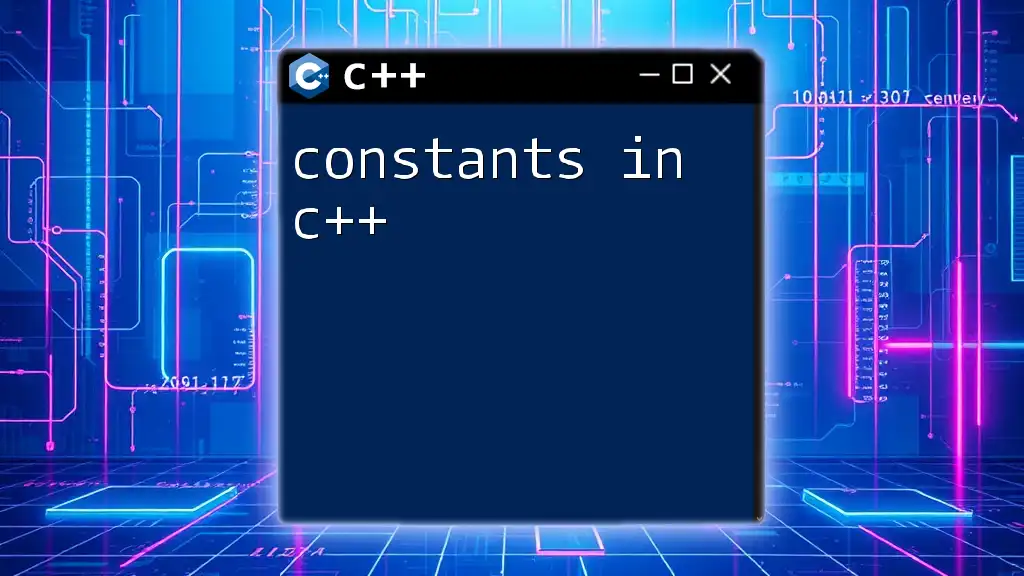
The Mechanics of const_cast
Basic Syntax of const_cast c++
The syntax for `const_cast` is straightforward, allowing you to express your intent clearly. The basic syntax looks like this:
const_cast<new_type>(expression)
Here's a simple code snippet demonstrating how to use `const_cast`:
const int x = 10;
int* y = const_cast<int*>(&x);
In this case, `const_cast` is used to cast away the `const` from `x`. This creates a pointer `y` that points to `x`, which may inadvertently lead to modifying the original constant value if further operations are performed.
Converting Between const and non-const
Const to Non-const Conversion
When you perform `const_cast` to convert a `const` type to a non-const type, you must be cautious. Here's how this conversion operates:
void modifyValue(const int* ptr) {
int* nonConstPtr = const_cast<int*>(ptr);
*nonConstPtr = 20; // Unsafe if original data was actually const
}
In this example, `modifyValue` takes a `const int*` pointer as an argument, then converts it to a non-const pointer. If `ptr` points to a truly constant value, this operation leads to undefined behavior.
Non-const to Const Conversion
`const_cast` can also be useful for converting non-const pointers or references to const versions. Here’s an example:
int z = 15;
const int* constPtr = const_cast<const int*>(&z);
This converts a pointer to a non-const integer `z` into a const pointer. This is generally safer; however, modifying `z` through the original pointer remains valid and should be approached with caution.
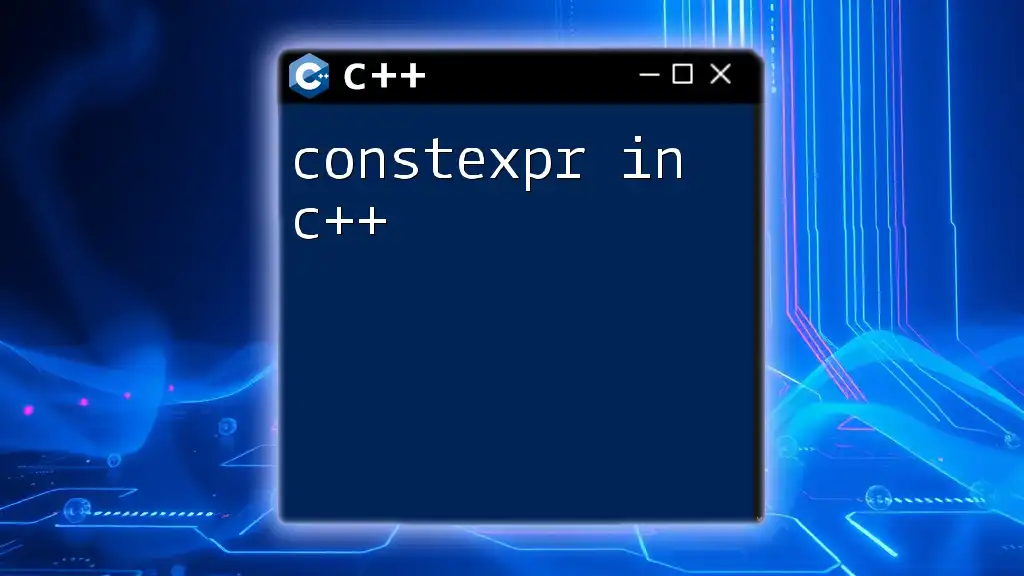
Practical Examples of Using const_cast
Example 1: Modifying a Const Object in a Function
One common scenario where `const_cast` comes into play is when you want to modify a constant object within a function. The following example illustrates this:
void updateValue(const int* p) {
int* modifiablePtr = const_cast<int*>(p);
*modifiablePtr = 99; // Danger!
}
In this code, the const pointer `p` is converted, and the value it points to is modified. This could lead to catastrophic errors if `p` was originally declared as a constant.
Example 2: Interfacing with Legacy Code
`const_cast` proves to be invaluable when you need to interface with older codebases that may not uphold modern const-correct practices. Consider this example:
void legacyFunction(int* p);
void wrapperFunction(const int* p) {
legacyFunction(const_cast<int*>(p));
}
Here, `wrapperFunction` takes a pointer to a constant integer and converts it to a non-const version using `const_cast` to send it to `legacyFunction`. This pattern demonstrates how `const_cast` can bridge the gap between new code and older implementations, though careful attention is necessary to prevent unintended side effects.
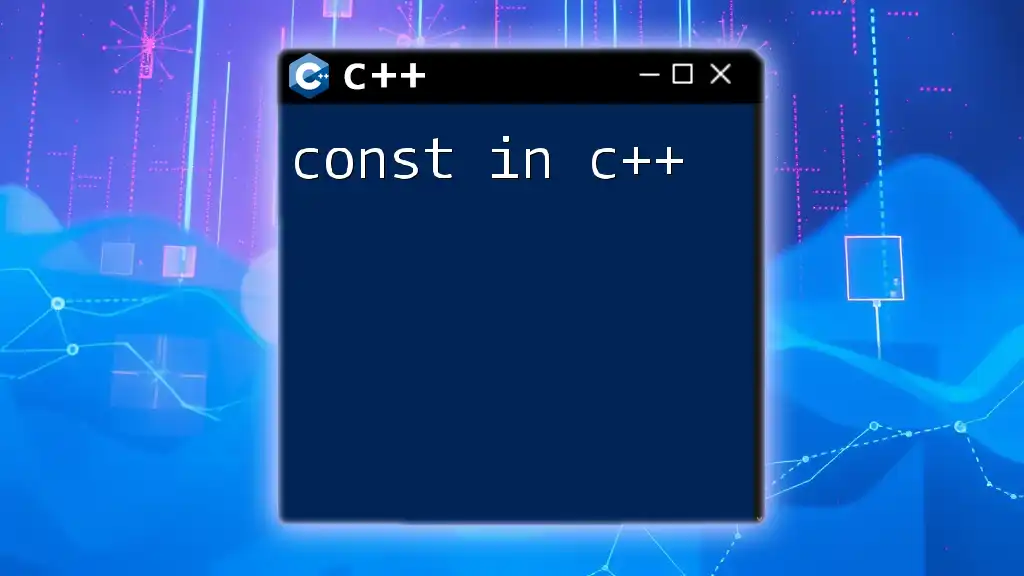
Best Practices and Cautions
When to Avoid const_cast
Despite its utility, there are several circumstances where you should avoid using `const_cast`:
- Unintended Side Effects: If you convert a const object, it may lead to unpredictable behavior if the original data was supposed to remain unaffected.
- Code Maintainability: Overusing `const_cast` can make code harder to read and maintain. It's always better to design functions that respect constness from the beginning.
Consider employing alternatives, such as passing parameters by const reference or directly handling mutable data, instead of relying on `const_cast`.
Conclusion
In summary, `const_cast in C++` is a specialized tool that allows programmers to modify the constness of variables in specific scenarios. While it can provide flexibility, it must be used carefully to avoid violations of const correctness and subsequent undefined behavior.
Understanding the power and cautions associated with `const_cast` enables developers to write safer and more efficient code. Always strive to maintain const correctness, resorting to `const_cast` only when absolutely necessary. By doing this, you'll ensure your applications are robust, maintainable, and reliable.
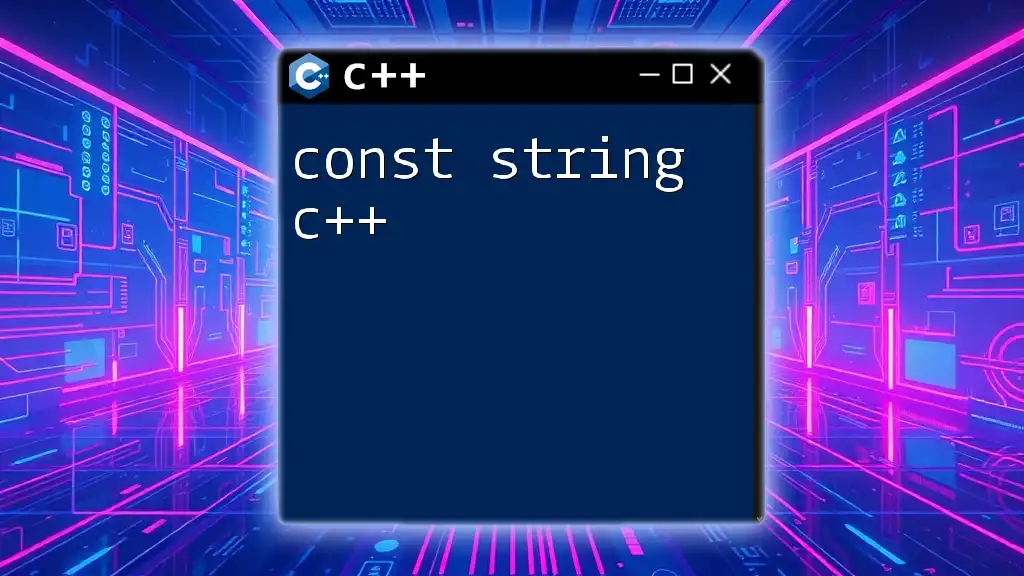
Additional Resources
For further reading on casting and const correctness in C++, consider exploring reputable resources like textbooks, online courses, or community-driven forums. These materials can enhance your understanding and proficiency in C++.