In C++, you can define a constant using the `const` keyword or the `constexpr` keyword for compile-time constants, as shown in the following example:
const int MAX_VALUE = 100; // using const
constexpr int MIN_VALUE = 0; // using constexpr
Understanding Constants in C++
What is a Constant?
A constant is a value that cannot be altered by the program during its execution. In C++, constants play a pivotal role in making the code more readable and maintainable. Unlike variables, constants are meant to remain unchanged after a value has been assigned, effectively preventing accidental modifications that could lead to errors.
C++ Constant Definition
In C++, a constant can be defined using the `const` keyword, `#define` preprocessor directive, or `constexpr`. The use of constants enhances code clarity, allowing developers to convey intent more explicitly. Constants can significantly reduce risks related to "magic numbers," which are unexplained numerical literals scattered throughout the code. By replacing these magic numbers with named constants, you enhance readability and facilitate future modifications.
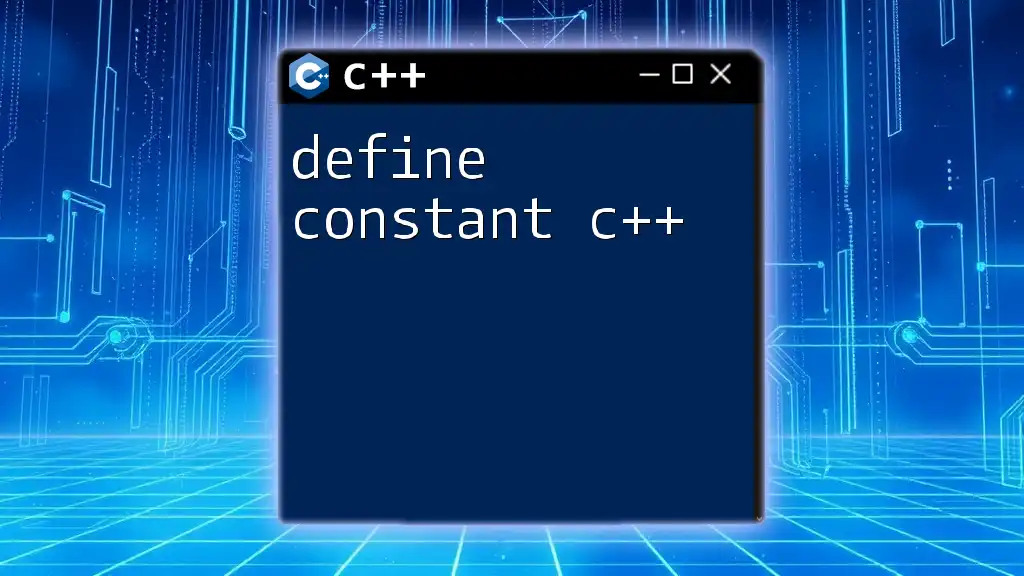
Declaring and Defining Constants in C++
Declaring a Constant in C++
In C++, you declare a constant using the `const` keyword, followed by the data type and the name of the constant. This syntax enforces an immutable state for the variable.
For example:
const int DAYS_IN_WEEK = 7;
In this snippet, `DAYS_IN_WEEK` is a constant integer that holds the value 7. Attempts to modify it later in the code will result in a compilation error, reinforcing its immutability.
Define Constants in C++ Using `#define`
Another way to create constants is by using the `#define` preprocessor directive. Unlike `const`, which links constants to a specific data type, `#define` creates a preprocessor macro that replaces occurrences of the defined name with the specified value before compilation.
Example:
#define PI 3.14159
This line ensures that every occurrence of `PI` in the code will become `3.14159`, which can be useful for mathematical applications. However, unlike `const`, `#define` lacks type safety, which may lead to unexpected behaviors in some circumstances.
Using `constexpr` for Compile-time Constants
The `constexpr` specifier allows for defining constants that the compiler can evaluate at compile time. This feature not only provides better performance but also enforces immutability and type safety.
For instance:
constexpr int MAX_USERS = 100;
By declaring `MAX_USERS` as `constexpr`, we inform the compiler that this value can be evaluated during compilation, making it possible to use this constant in scenarios that require compile-time expressions.
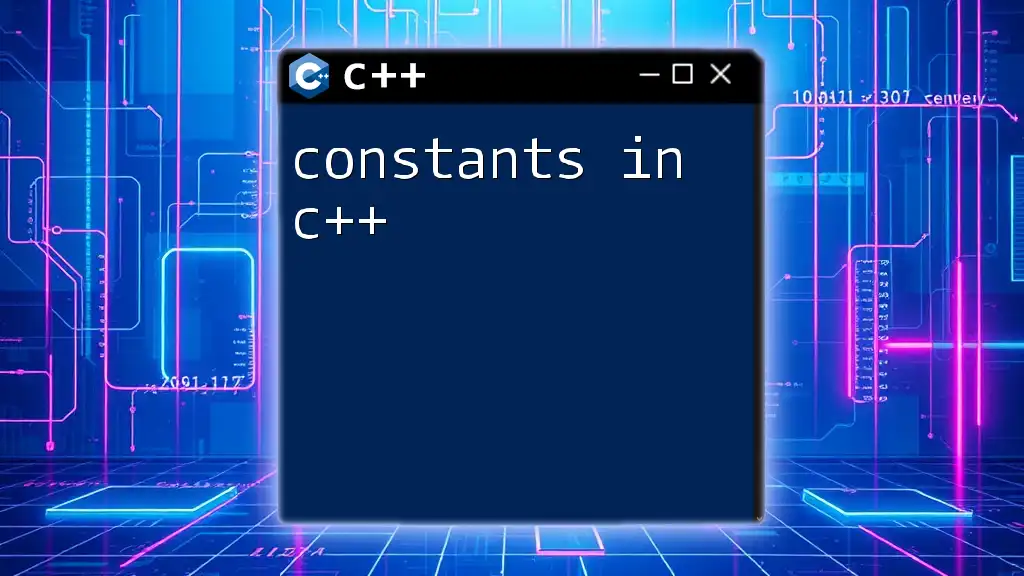
Best Practices for Using Constants in C++
Naming Conventions
Naming your constants clearly and descriptively is crucial for code legibility. A common practice is to use uppercase letters with underscores separating words, which distinguishes constants from regular variables.
Example:
const int MAX_SPEED_LIMIT = 120;
This convention helps in easily identifying constants while reading through the code.
When to Use Constants
Constants should be utilized instead of variables in instances where a value is known beforehand and will not be modified. This practice helps eliminate magic numbers, enhancing the clarity of your logic.
For example, a formula for calculating the area of a circle could be written as follows:
const double PI = 3.14159;
double area = PI * (radius * radius);
Using `PI` as a constant clarifies the intent of the code and reduces the risk of arbitrary numerical changes.
Organizing Constants in Code
To maintain cleaner and more organized code, it's advisable to group constants in dedicated header files. This approach eases the process of updating and maintaining your constants without affecting other parts of your code.
Example of a constants header file `constants.h`:
// constants.h
const double GRAVITY = 9.81;
const double LIGHT_SPEED = 299792458;
By including the `constants.h` file in other source files, you can maintain a centralized repository for all your constant definitions.
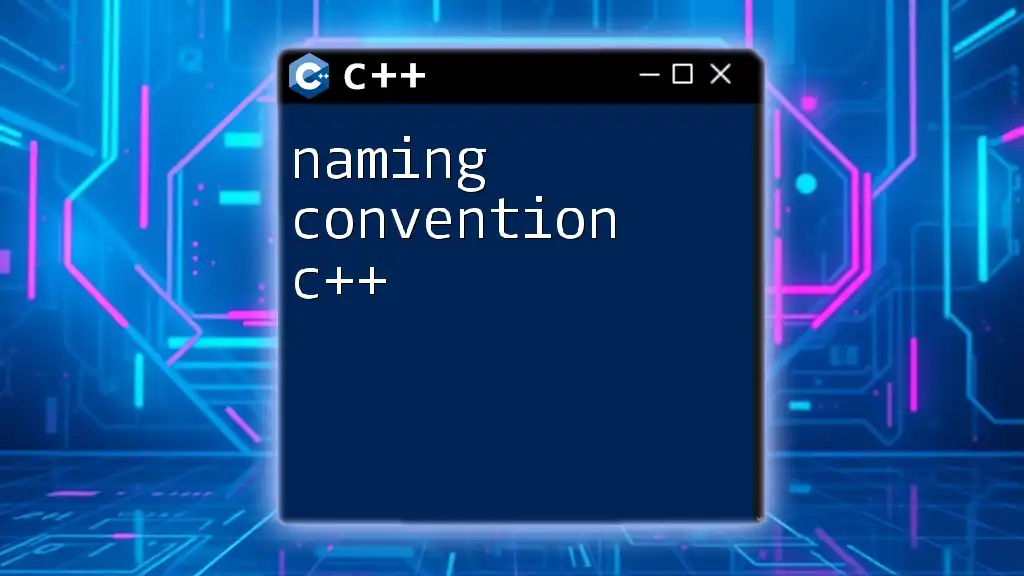
Accessing Constants in C++
Accessing Constants in Functions
Constants can be easily accessed within functions, promoting reuse across different parts of your code. For instance, you might want to calculate the circumference of circles using a defined `PI` constant.
Example:
void calculateCircumference(double radius) {
double circumference = 2 * PI * radius;
}
By using the pre-defined constant `PI`, the function’s user does not need to understand its value, making it more straightforward.
Constants in Class Definitions
You can also define constants within a class using static members. This way, constants can be associated with a class while preventing instance-specific states.
Example:
class Circle {
public:
static const double PI;
Circle(double r) : radius(r) {}
private:
double radius;
};
const double Circle::PI = 3.14159;
The `Circle` class uses the `PI` constant as a shared member across all instances, ensuring consistency while promoting encapsulation.
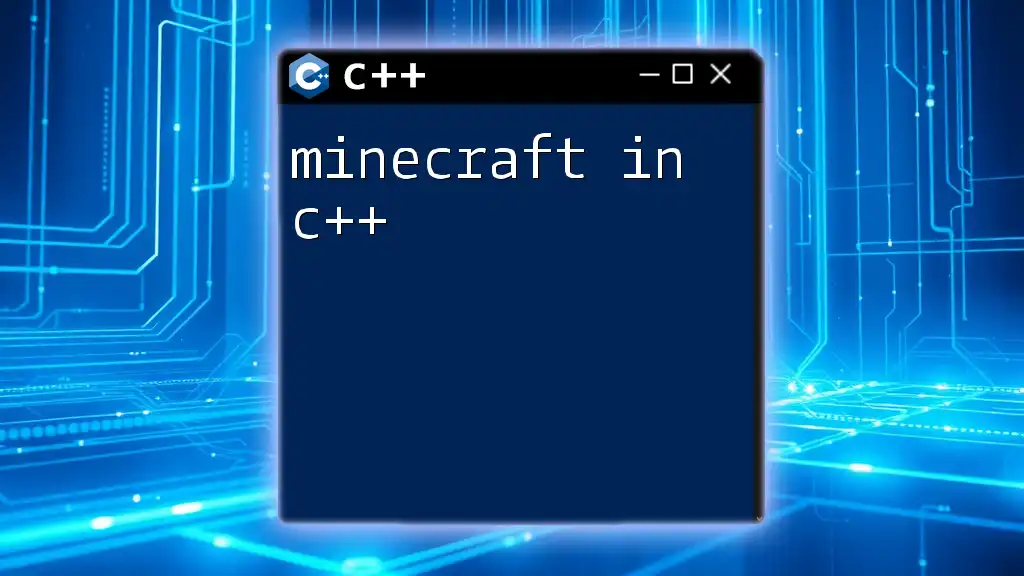
Common Pitfalls to Avoid
Misnaming Constants
Make sure to follow naming conventions consistently to avoid confusion. A poorly named constant may mislead or confuse other developers (or even your future self) about its purpose, which can hinder code maintenance and understanding.
Forgetting Immutability
Forgetting that constants cannot be altered after their initial assignment can lead to compilation errors. Always remember that attempting to change a constant's value will result in errors, reinforcing the necessity of using `const`.
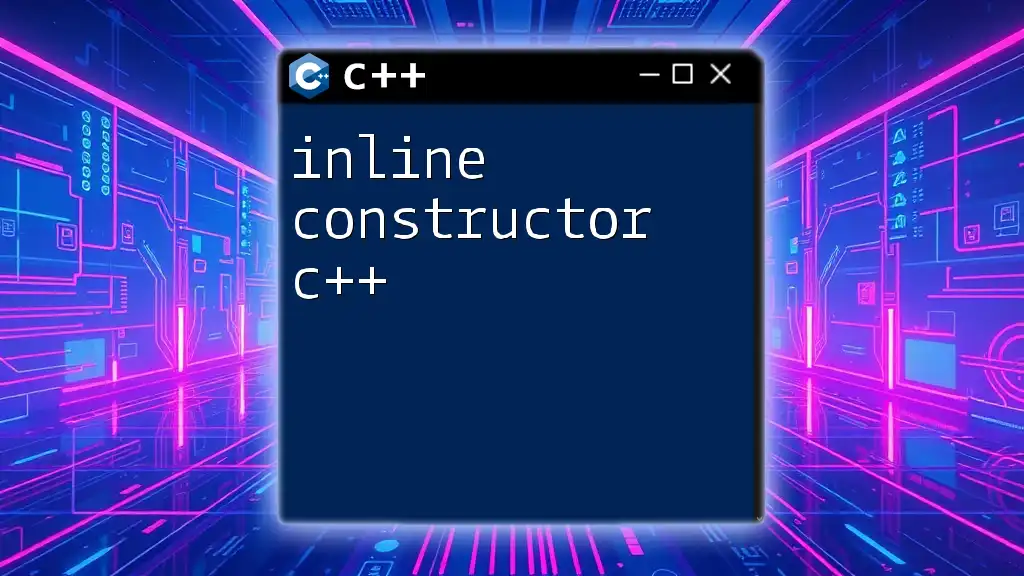
Conclusion
In summary, understanding how to define constants in C++ is essential for developing clean, readable, and maintainable code. By using various methods like `const`, `#define`, and `constexpr`, you can effectively manage values that should remain unchanged throughout your code's lifecycle. Embracing consistent naming conventions and organizing constants can lead to better code practices, while awareness of common pitfalls ensures your coding journey is smooth and efficient.
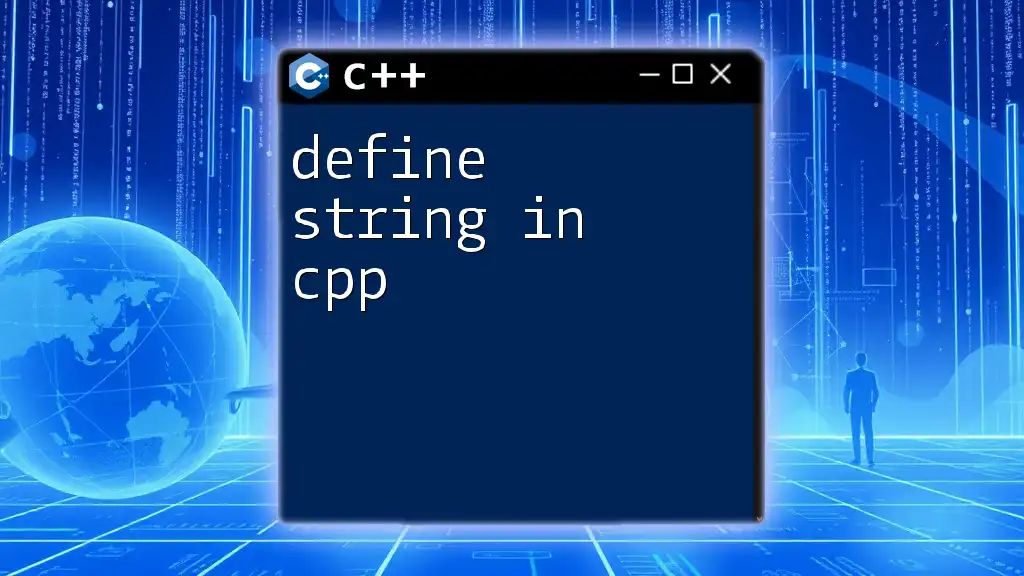
Additional Resources
To further your knowledge on C++, consider exploring various books and online courses focusing on advanced topics. Always make sure to consult reference documentation for practical insights and additional examples related to constants and other programming constructs.