In C++, you can define a constant using the `const` keyword, which allows you to create a variable whose value cannot be changed after it is initialized.
const int MAX_USERS = 100;
What is a Constant in C++?
A constant in C++ is a type of variable whose value remains unchanged throughout the program. Using constants allows programmers to make their code clearer and reduces the risk of errors caused by unintentionally altering values that should remain fixed. Constants can represent values such as mathematical constants, fixed settings, or unchanging parameters that improve code readability and maintainability.
Types of Constants
C++ supports several types of constants, each serving a different purpose:
-
Numeric Constants: These include both integer and floating-point constants. For example, the numbers `42` and `3.14` are numeric constants.
-
Character Constants: These are single characters enclosed in single quotes, like `'A'` or `'#'`.
-
String Constants: These are sequences of characters enclosed in double quotes. For instance, `"Hello, World!"` is a string constant.
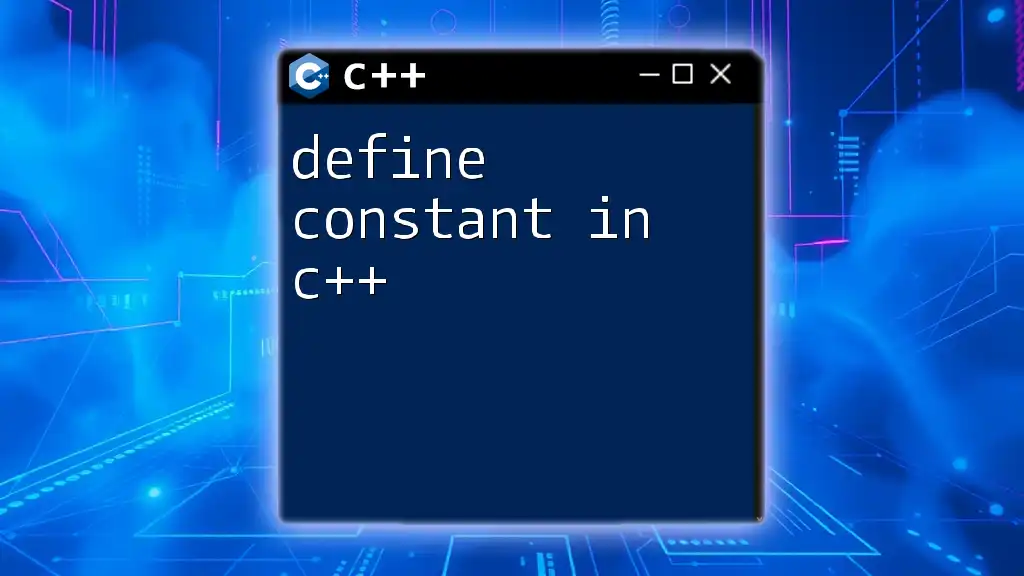
How to Define Constants in C++
Defining constants in C++ is straightforward and often accomplished using the `const` keyword. This keyword indicates that a variable’s value will not change after it has been initialized.
Define `const` in C++
The syntax for defining a constant with `const` is simple. When you declare a variable using `const`, you're essentially saying, “This variable's value should remain constant throughout the program.”
Here’s a basic example of using `const`:
const int MAX_USERS = 100; // Defining a constant integer
In this example, `MAX_USERS` is a constant that holds the value `100`. Attempting to modify `MAX_USERS` later in the code will result in a compilation error, ensuring that its value remains unchanged.
Benefits of Using `const`
Using `const` in your code comes with several advantages:
-
Clarity: It makes your intentions clear to anyone reading the code, indicating that a value is intended to be constant.
-
Error Prevention: Since constants cannot be altered after definition, this reduces the chance of bugs caused by unintentional changes.
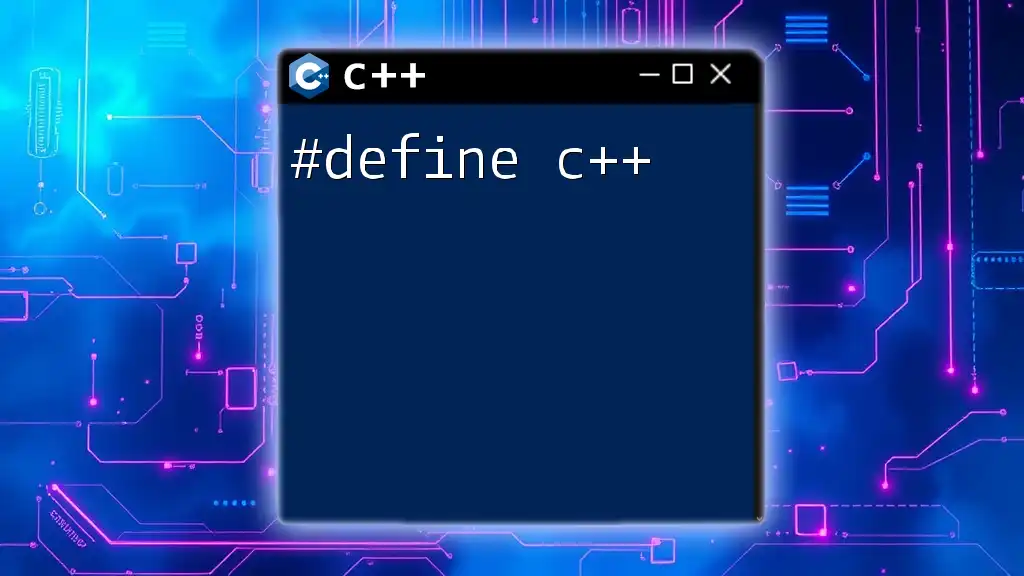
C++ Constant Definition with `#define`
In addition to the `const` keyword, C++ also allows the use of the preprocessor directive `#define` for defining constants. However, it's essential to note that `#define` does not abide by type safety as `const` does.
Using `#define` for Constants
The syntax for defining a constant with `#define` is quite different from using `const`. Here’s how you would use `#define`:
#define PI 3.14 // Defining a constant using #define
In this example, `PI` is defined as a constant with the value `3.14`. When the preprocessor runs, it replaces all instances of `PI` in the code with `3.14`.
Comparison of `#define` vs `const`
While both `#define` and `const` can define constants, they have notable differences:
-
Type Safety: `const` allows for type checking, while `#define` does not. This means that misusing a `#define` constant can lead to runtime errors that are harder to debug.
-
Scope: `const` respects variable scopes, and constants defined with `const` will only exist within the specified scope. In contrast, `#define` constants are globally accessible throughout the entire source file.
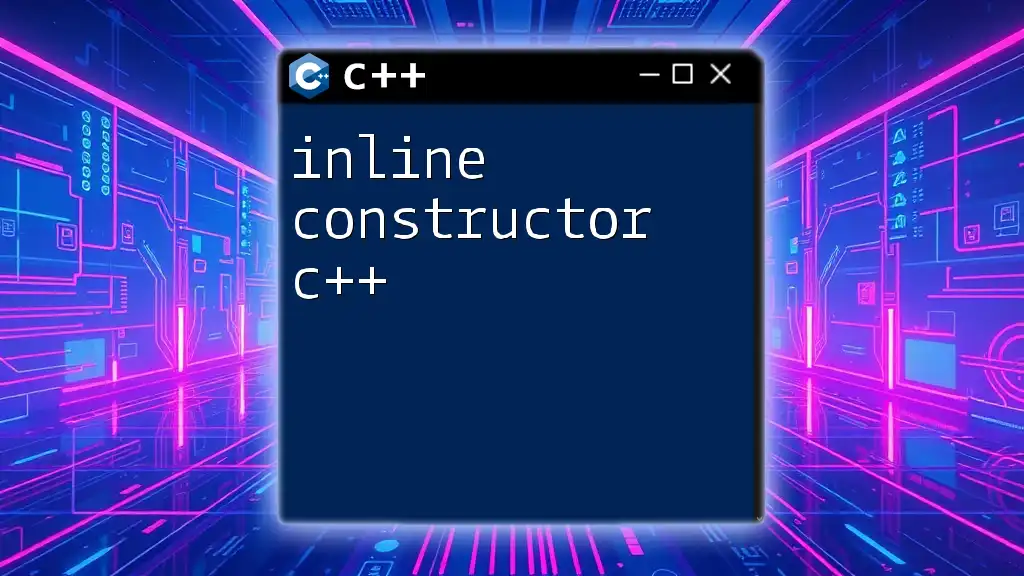
Defining Constants: Best Practices
To maximize the benefits of using constants in your code, consider the following best practices:
-
Use Uppercase Letters: It's a common convention to name constants using all capital letters (e.g., `MAX_LENGTH`). This approach enhances readability and distinguishes constants from regular variables.
-
Scoping: Be mindful of where you define constants. Using `const` within local functions limits their visibility, helping avoid potential conflicts.
Namespace for Constants
Organizing constants within a namespace can improve code organization:
namespace Math {
const double PI = 3.14159;
}
In this example, the constant `PI` is declared within the `Math` namespace, making it easier to manage and reference without name collisions.
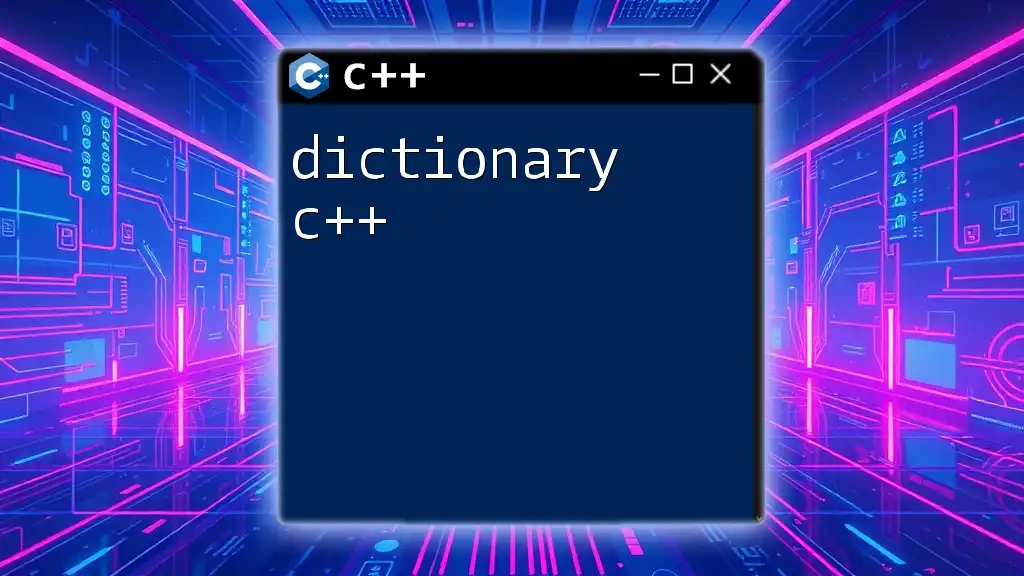
When to Use `const`, `constexpr`, and `#define`
Understanding when to use each option for defining constants is crucial for effective coding in C++:
-
Use `const`: When you want type safety and visibility scoped to blocks or classes.
-
Use `constexpr`: For values that need to be evaluated at compile time. It also ensures type safety.
Understanding `constexpr`
The `constexpr` keyword allows you to define constant expressions that the compiler can evaluate at compile time. This is particularly useful for productivity and performance:
constexpr int MAX_USERS = 200; // Must be known at compile time
In this case, `MAX_USERS` is defined as a `constexpr`, meaning the value `200` will be determined at compile time.
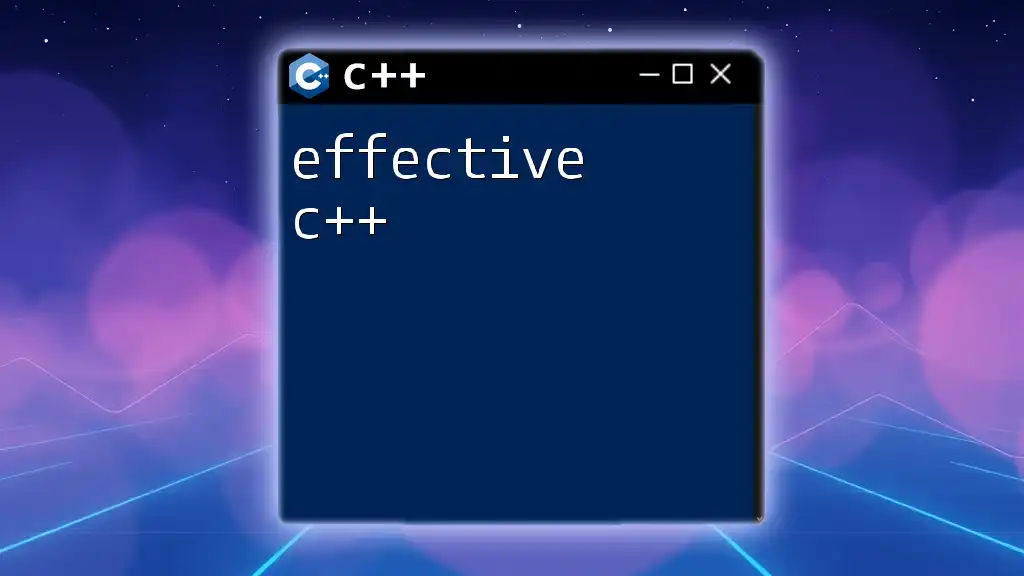
Common Mistakes with Constants in C++
Understanding common pitfalls can help you use constants more effectively in C++. Here are some frequent mistakes developers might encounter:
Re-defining Constants
One of the most common errors is attempting to redefine a constant. For example, if you try to reassign a value to `MAX_USERS`, it will result in a compilation error.
Misusing `#define`
Another issue arises from the global scope of `#define`. Since `#define` is not type-safe, it can lead to unexpected behavior and debugging headaches if used improperly in large codebases.
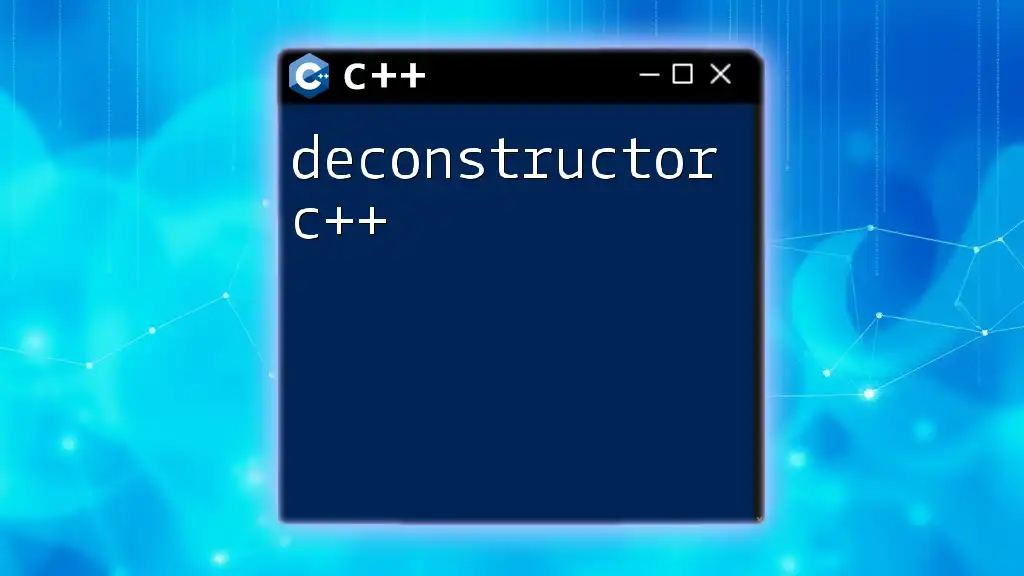
Conclusion
In conclusion, defining constants in C++ is a fundamental aspect of writing clean, maintainable, and bug-free code. By utilizing `const`, `#define`, and `constexpr`, developers can ensure their variables behave as intended, providing clarity and reducing the likelihood of errors. The proper use of constants not only aids in code organization but also enhances the overall quality of programming in C++.
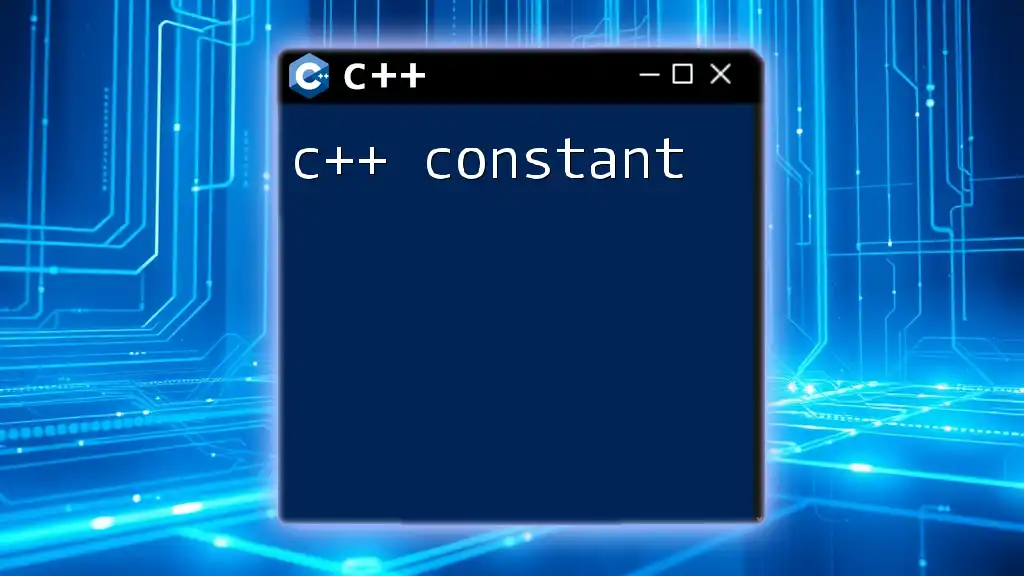
Additional Resources
For those further interested in the topic of defining constants in C++ and best practices, additional reading materials and tutorials can provide deeper insights and advanced techniques.