In C++, a vector is a dynamic array provided by the Standard Template Library (STL) that can resize itself automatically when elements are added or removed.
Here’s an example of how to define a vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector; // Define a vector of integers
return 0;
}
What is a Vector in C++?
A vector is a dynamic array provided by the Standard Template Library (STL) in C++. It allows you to store a collection of items that can change in size during the program's execution. This feature makes vectors particularly useful in scenarios where the amount of data is not known at compile time.
Key characteristics of vectors include:
- Dynamic Resizing: Unlike traditional arrays, vectors can grow or shrink in size as you add or remove elements.
- Element Access via Indices: Vectors provide fast access to their elements using indices, similar to arrays.
- Contiguous Storage: The elements in a vector are stored in contiguous memory, which means there is little overhead involved for element access.

How to Define a Vector in C++
To begin using vectors in C++, it is crucial to include the appropriate header file:
#include <vector>
Basic Syntax for Defining a Vector
The basic format to define a vector is:
std::vector<data_type> vector_name;
For example, to define a vector of integers, you would write:
std::vector<int> numbers;
Defining a Vector with Initial Values
You can also initialize a vector with preset values. This is done by using curly braces `{}`. For instance:
std::vector<int> numbers = {1, 2, 3, 4, 5};
Defining a Vector with a Specified Size
Sometimes you need to define a vector with a known size. This allows you to specify the number of elements, which will be initialized to a default value (0 for numeric types):
std::vector<int> numbers(5); // Creates a vector of size 5, initialized to 0
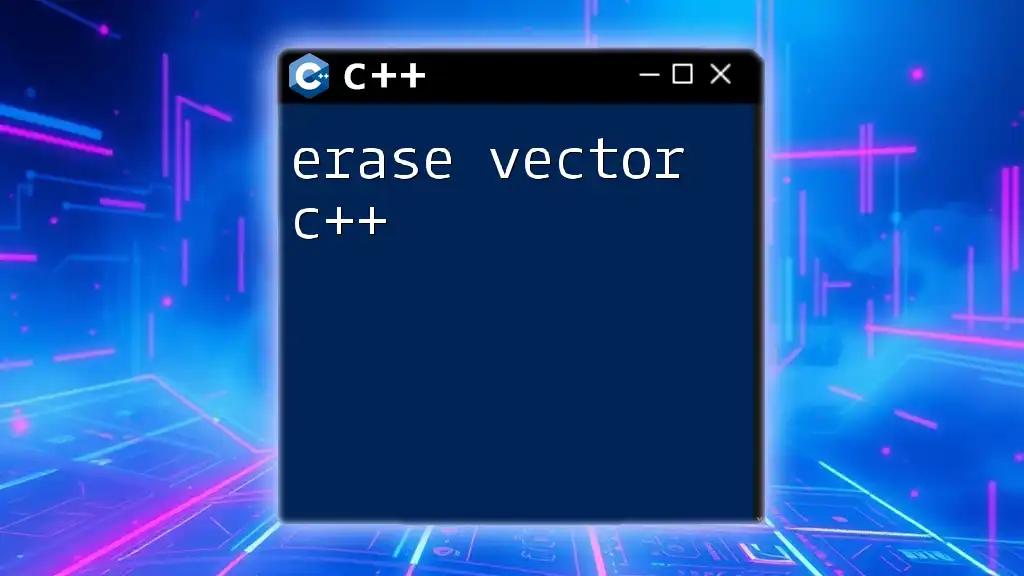
Working with Vectors
Accessing Elements in a Vector
You can access elements in a vector using the `[]` operator or the `at()` method. The primary difference is that `at()` provides bounds checking, making it safer:
std::cout << numbers[0]; // Outputs the first element
std::cout << numbers.at(0); // Outputs the first element safely
Modifying Elements in a Vector
Setting values in a vector can be accomplished similarly to how you would with an array:
numbers[0] = 10; // Sets the first element to 10
Adding Elements to a Vector
To add elements dynamically to the end of a vector, you can use the `push_back()` method. This method increases the size of the vector by one:
numbers.push_back(6); // Adds 6 to the end of the vector
Removing Elements from a Vector
To remove the last element from a vector, you can utilize the `pop_back()` method, which effectively reduces the size of the vector by one:
numbers.pop_back(); // Removes the last element
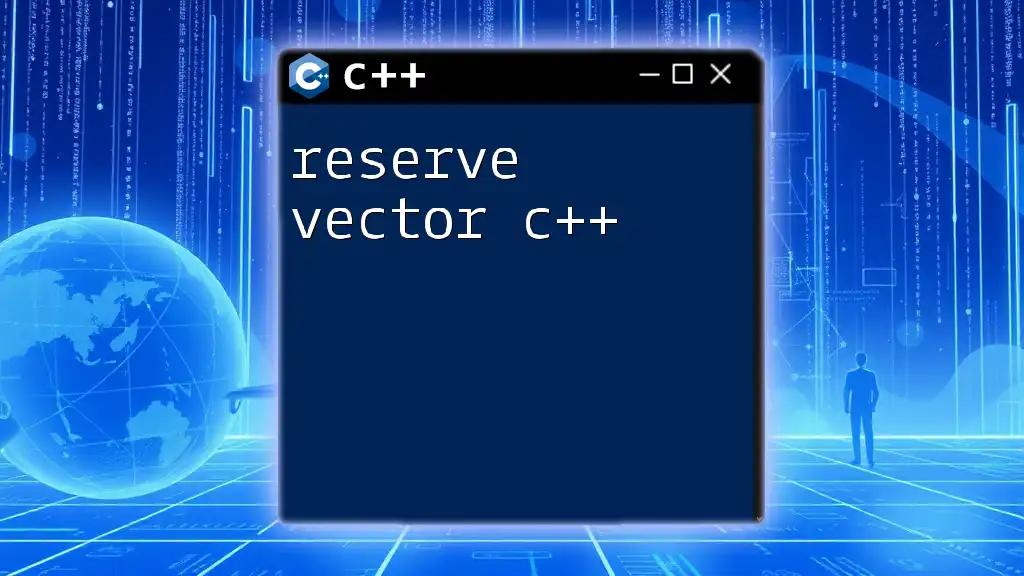
Practical Examples of Vectors
Example 1: Storing User Input
Vectors are ideal for storing user-generated data of unknown length. Here's an example of reading user input into a vector:
std::vector<int> userInputs;
int input;
while (std::cin >> input) {
userInputs.push_back(input);
}
In this code snippet, we create an empty vector called `userInputs` and continuously read integers from the user until the input ends. Each value is added to the vector dynamically.
Example 2: Searching Through a Vector
You can easily search for elements in a vector using algorithms provided in the STL. Here’s a straightforward example that checks if the number `10` is in the `userInputs` vector:
bool found = std::find(userInputs.begin(), userInputs.end(), 10) != userInputs.end();
This line of code uses the `std::find` algorithm to search for the value `10`. It returns true if found, or false otherwise.
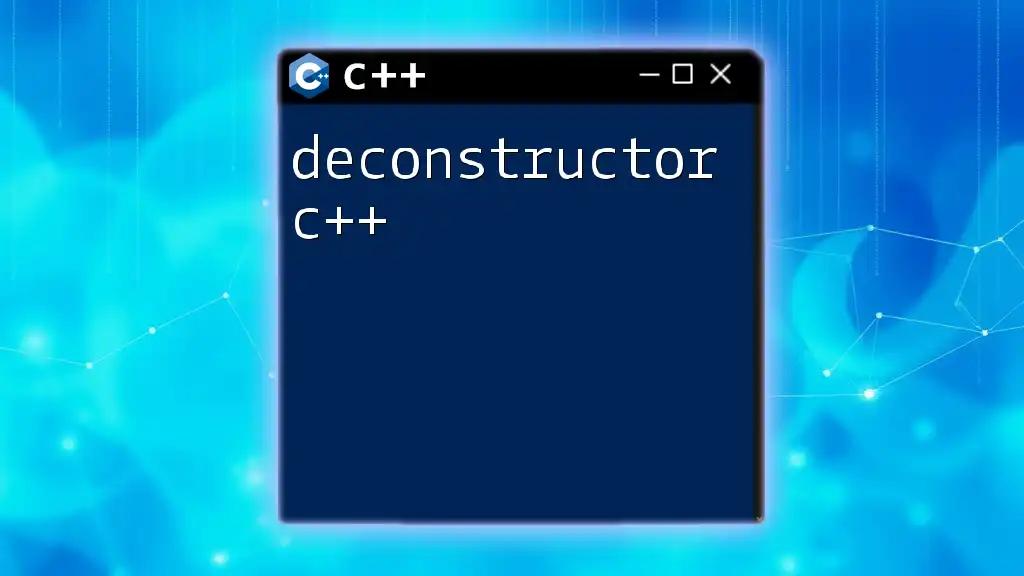
Advanced Vector Operations
Iterating Over a Vector
To access each element in a vector, you can use loops. The traditional for-loop or the range-based for-loop are both effective. Here’s an example using a range-based for-loop:
for (int num : numbers) {
std::cout << num << " ";
}
Sorting a Vector
Vectors can be easily sorted using the `std::sort()` function from the `<algorithm>` library. To sort the `numbers` vector, simply use:
std::sort(numbers.begin(), numbers.end());
Sorting rearranges the elements in ascending order by default.
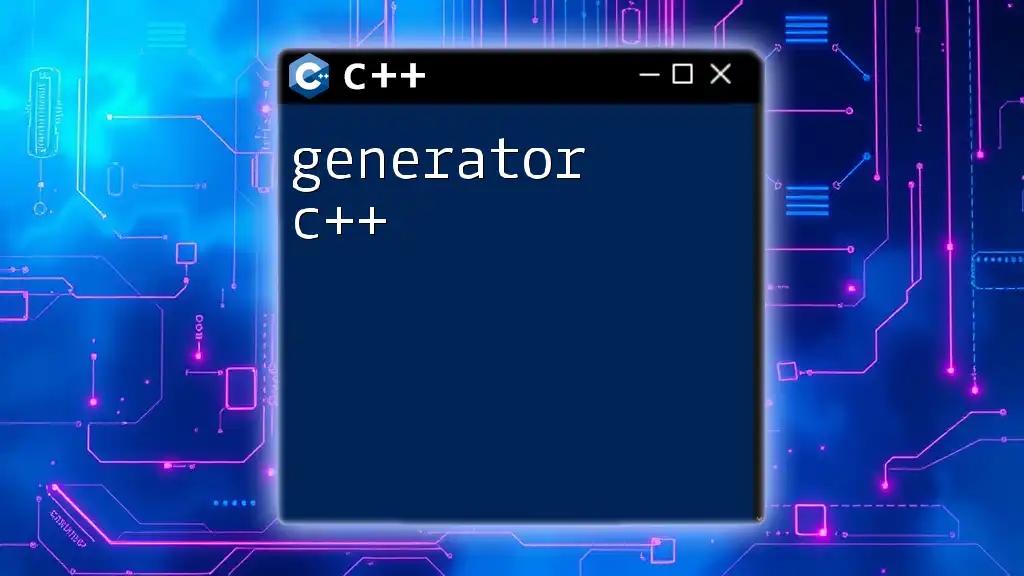
Common Use Cases for Vectors
Vectors are highly versatile and suitable for several practical applications, including:
- Dynamic Data Storage: Use vectors to handle data when the size is unknown at compile time.
- Diverse Data Structures: Implement data structures that require flexibility, like lists or queues.
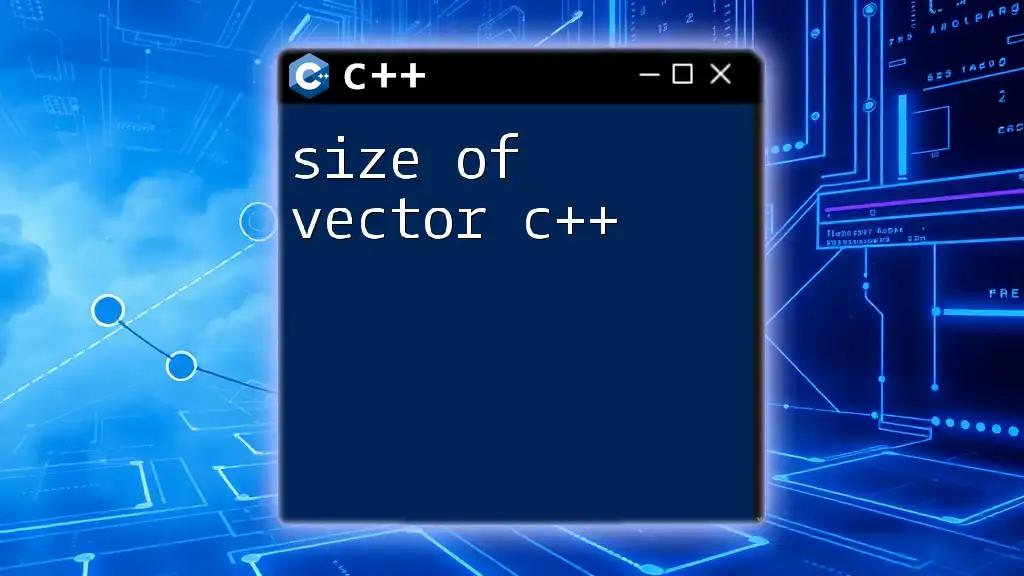
Conclusion
In summary, defining a vector in C++ opens up many possibilities for efficient data handling and manipulation. Vectors offer dynamic sizing, fast access, and versatile operations, making them an essential tool for C++ programming. By mastering vectors, you can significantly improve your ability to manage collections of data effectively.
Now, as you delve deeper into C++, we encourage you to practice using vectors through coding examples, challenges, and experimentation. Understanding their capabilities will elevate your programming skills, making your code simpler and more efficient.
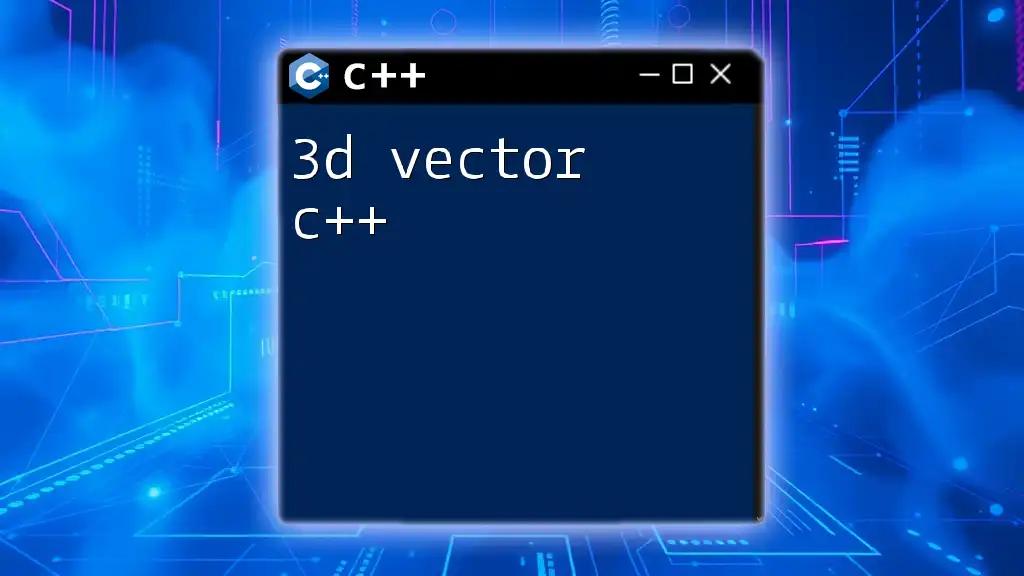
Additional Resources
For further information and a deeper understanding, consider exploring the following resources:
- C++ Standard Library Documentation: A comprehensive guide to all STL functions, including vectors.
- Recommended Books and Online Courses: Look for trusted titles specializing in C++ programming.
- Official C++ Community Forums: Engage with other developers and seek advice as you navigate your C++ journey.