In C++, you can resize a vector using the `resize()` member function, which changes the size of the vector to the specified number of elements, potentially adding or removing elements.
Here's a code snippet demonstrating how to use `resize()`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3};
myVector.resize(5, 0); // Resizes vector to 5 elements, filling new elements with 0
for (int num : myVector) {
std::cout << num << " ";
}
return 0;
}
Understanding C++ Vectors
What is a Vector?
A vector is a sequence container that encapsulates dynamic size arrays in C++. Unlike traditional arrays, vectors can grow or shrink in size as elements are added or removed. This feature makes them incredibly versatile for various applications, such as managing collections of data.
Why Use Vectors?
Vectors offer notable advantages over standard arrays:
- Dynamic Sizing: You do not need to declare the size of a vector in advance, making vectors highly adaptable.
- Automatic Memory Management: Vectors handle memory allocation and deallocation automatically, reducing the risk of memory leaks and dangling pointers.
- Rich Functionality: With member functions like `push_back`, `pop_back`, and `resize`, vectors provide powerful tools for managing collections of elements.

Overview of the resize Function
What is the `resize` Function?
The `resize` function is used to change the size of a vector. This function can either increase or decrease the size, allowing for dynamic adjustment of the container's capacity.
Prototype of the `resize` Function
The syntax of the `resize` function is as follows:
void resize(size_type n, value_type val = value_type());
Here, `n` specifies the new size of the vector, and `val` (optional) is the value to assign to new elements if increasing size.
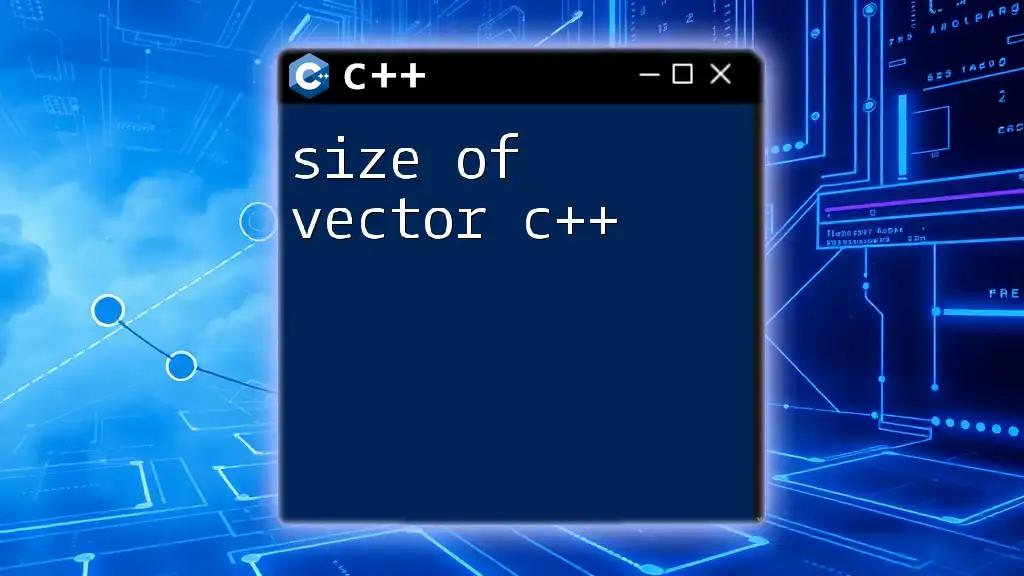
How to Use the `resize` Function
Basic Syntax and Usage
To demonstrate how to resize a vector, consider the following example that increases the size of a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec{1, 2, 3};
vec.resize(5); // Resizes the vector to contain 5 elements
for (int n : vec) {
std::cout << n << ' '; // Output: 1 2 3 0 0
}
return 0;
}
In this code, the original vector `{1, 2, 3}` is resized to contain five elements. New elements are initialized to zero by default, demonstrating how `resize` effectively maintains existing data while adding placeholders.
Resizing to a Smaller Size
Now, let's see how to handle shrinking a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec{1, 2, 3, 4, 5};
vec.resize(3); // Resizes the vector to contain 3 elements
for (int n : vec) {
std::cout << n << ' '; // Output: 1 2 3
}
return 0;
}
In this example, the vector is reduced from five to three elements. The last two elements are removed, and the output reflects this change, emphasizing how the `resize` function can also decrease the container size effectively.
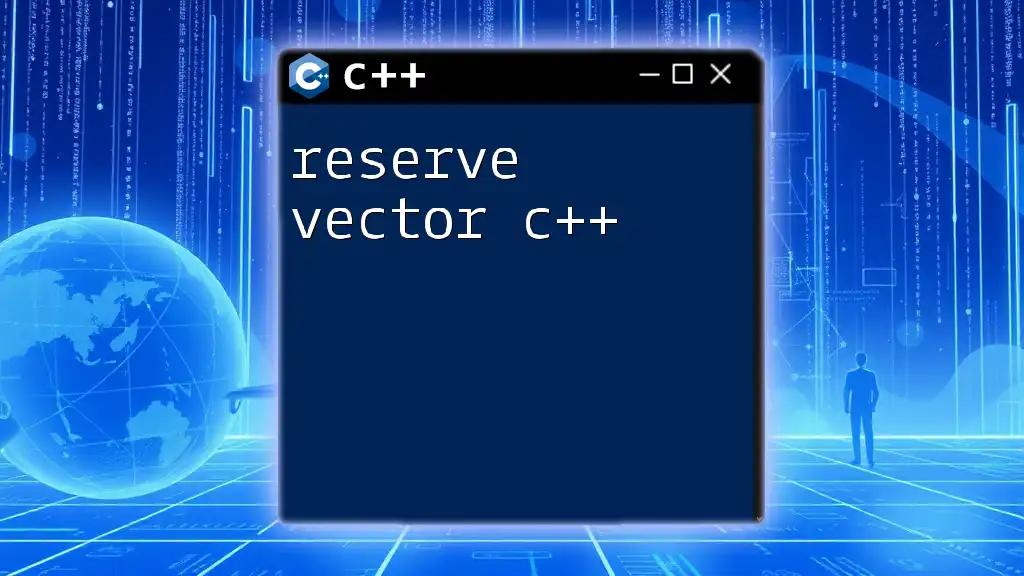
Default Values in `resize`
Setting Default Values While Resizing
You can also specify a default value when resizing. The following example adds a value of 9 to new elements:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec{1, 2, 3};
vec.resize(5, 9); // Any new elements will be initialized to 9
for (int n : vec) {
std::cout << n << ' '; // Output: 1 2 3 9 9
}
return 0;
}
This snippet shows that the vector's size is increased to five, and any new elements are initialized to `9`, illustrating the flexibility offered by the `resize` function.
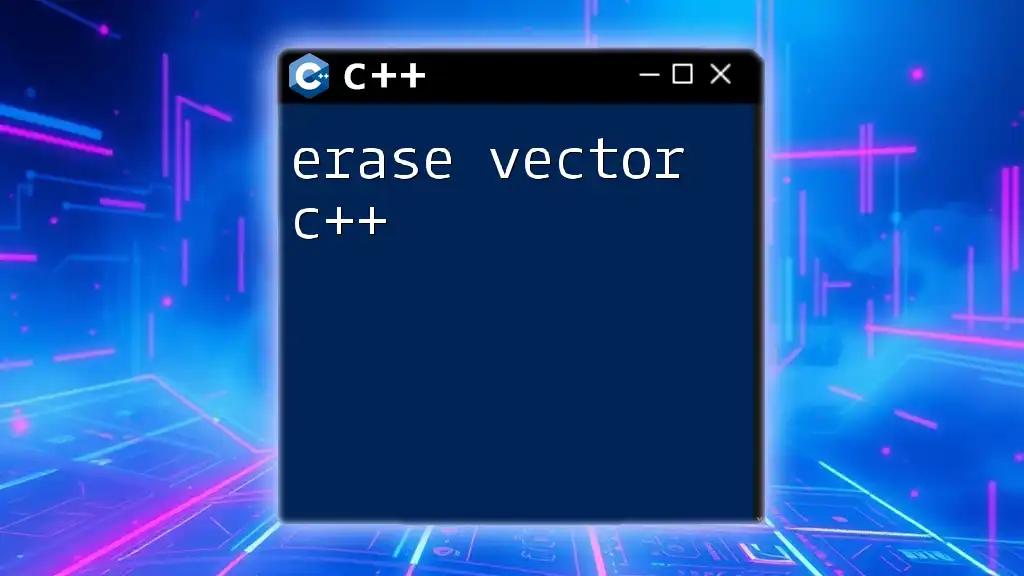
Performance Considerations
Memory Management When Resizing
When you resize a vector, particularly when increasing its size, the vector may need to allocate new memory to hold additional elements. This can lead to performance costs due to memory allocation and copying existing elements to the new location.
Best Practices for Resizing
To optimize performance:
-
Use `reserve` for Uncertain Sizes: If you anticipate needing multiple elements, use `reserve` to allocate memory without changing the size, avoiding unnecessary reallocations when elements are added.
-
Resize Wisely: Only resize vectors when necessary, and prefer using `push_back` or `emplace_back` for adding individual elements to minimize overhead when working with manageable sizes.

Practical Examples of Resizing Vectors
Using `resize` in Real-World Applications
Here’s an application of the `resize` function that allows user input to determine the size of a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<std::string> names;
int n;
std::cout << "Enter number of names: ";
std::cin >> n;
names.resize(n); // Set the size according to user input
for (int i = 0; i < n; ++i) {
std::cout << "Enter name " << (i + 1) << ": ";
std::cin >> names[i];
}
std::cout << "You entered: ";
for (const auto& name : names) {
std::cout << name << ' ';
}
return 0;
}
In this snippet, the program asks the user for the number of names and resizes the vector accordingly. It demonstrates the dynamic nature of vectors and how `resize` can be crucial in interactive programs.
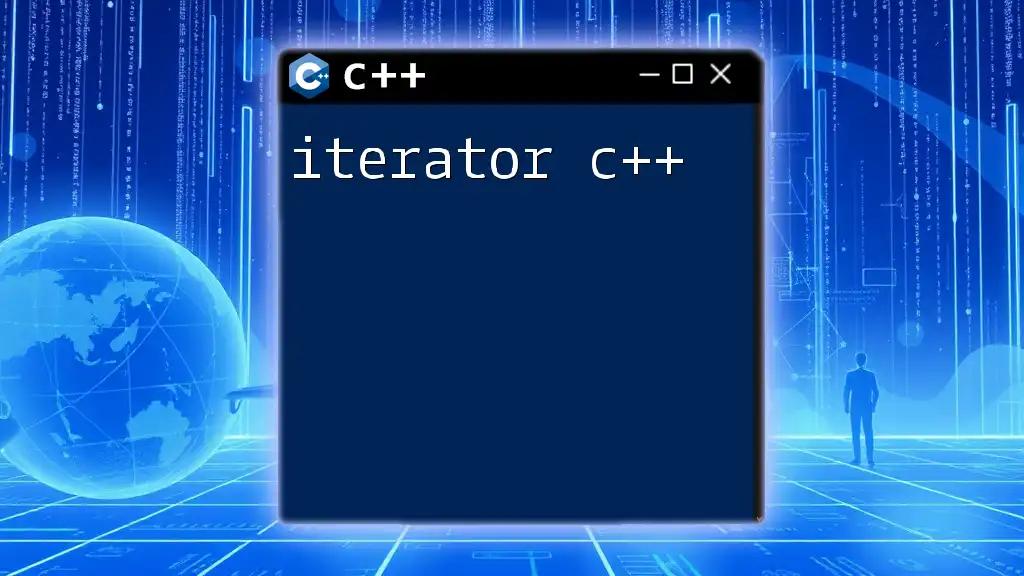
Common Mistakes to Avoid
Misuse of `resize` Function
Several pitfalls can occur when working with `resize`:
-
Forgetting to Initialize: Always ensure the vector is initialized before calling `resize`, or else it may lead to unintended behavior or logical errors.
-
Misunderstanding Effects: Be mindful of how both increasing and decreasing vector sizes work, particularly in how they handle existing data.

Conclusion
In summary, the ability to resize a vector in C++ is a powerful feature that enhances the flexibility and efficiency of your programs. Understanding how to use the `resize` function effectively allows for better data management while avoiding common pitfalls. Experiment with these concepts in your code, and consider following resources for more in-depth tutorials on C++ programming. By mastering vector manipulation, you will significantly improve your coding fluency in C++.