A 2-dimensional vector in C++ is a vector of vectors that allows you to store and manipulate data in a grid-like structure, resembling a matrix.
Here's a simple code snippet demonstrating how to create and initialize a 2D vector in C++:
#include <iostream>
#include <vector>
int main() {
// Creating a 2D vector with 3 rows and 4 columns
std::vector<std::vector<int>> matrix(3, std::vector<int>(4, 0));
// Initializing the 2D vector
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
matrix[i][j] = i * j; // Example initialization
}
}
// Displaying the 2D vector
for (const auto& row : matrix) {
for (const auto& value : row) {
std::cout << value << " ";
}
std::cout << std::endl;
}
return 0;
}
What is a 2D Vector?
A two-dimensional vector (2D vector) in C++ is essentially a vector of vectors, allowing you to represent data in a grid-like structure. This structure can be particularly useful when working with matrices, grids in gaming, or any scenario where data can be organized in rows and columns. Understanding how to work with 2D vectors is essential for many applications, from scientific computing to graphical representations.
Practical Applications
2D vectors have a wide range of applications:
- Gaming: They are frequently used to represent game boards, maps, or any tile-based systems where navigational and spatial relationships are necessary.
- Scientific Computing: In mathematics, physics, and engineering, they are employed to handle matrices and multidimensional arrays, which are crucial for various algorithms and calculations.
- Graphics: 2D vectors are used in graphics programming for transformations and rendering images on screens, allowing for operations such as translations, rotations, and scaling.
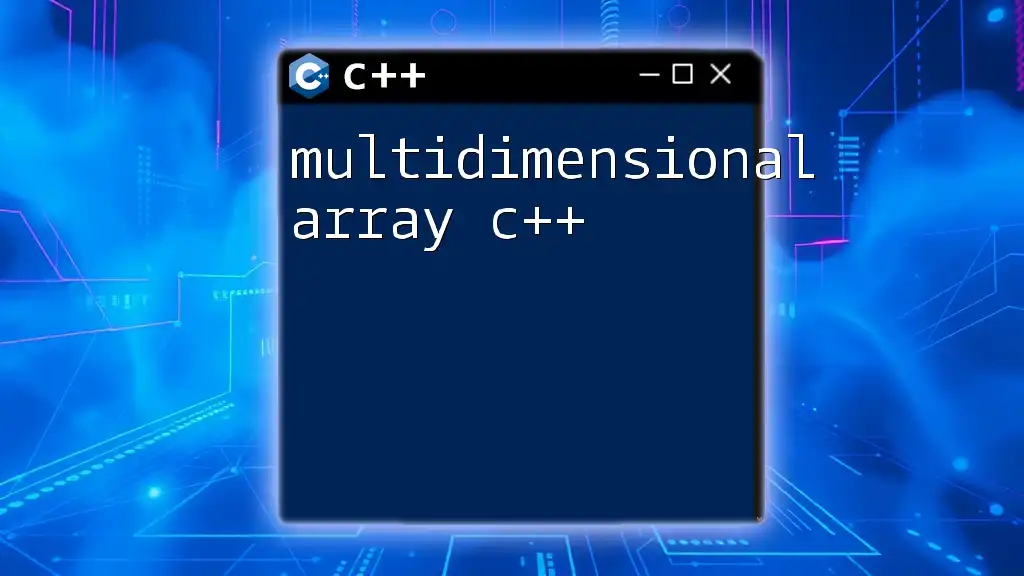
Understanding the Basics of 2D Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can resize itself automatically when items are added or removed. Unlike traditional arrays, vectors can efficiently manage their memory and easily adapt to changing data sizes. A 2D vector is effectively a collection of vectors, where each vector can represent a row of data, making it a way to create a table of information.
Syntax and Initialization
Declaring a 2D vector involves specifying two dimensions. The syntax typically resembles the following:
#include <vector>
std::vector<std::vector<int>> vec; // Declaring a 2D vector
In the example above, `vec` is a 2D vector that can hold integers. You can also initialize the vector at the time of declaration:
std::vector<std::vector<int>> vec = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; // Initializing a 2D vector
In this example, `vec` contains three rows and three columns, filled with specific integer values.
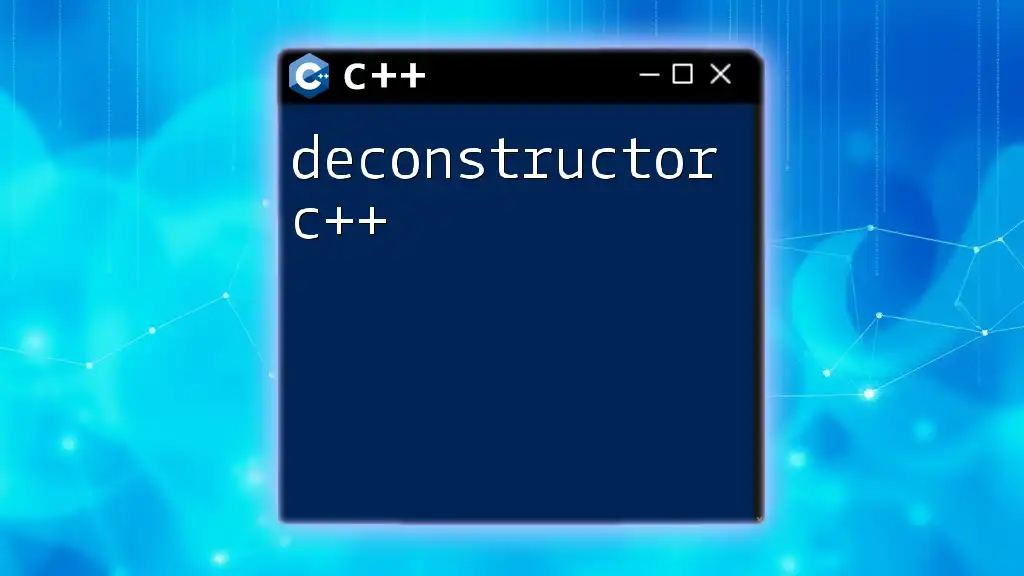
Creating and Manipulating 2D Vectors in C++
Creating a 2D Vector
To create a 2D vector, you can specify the number of rows and columns:
std::vector<std::vector<int>> vec(3, std::vector<int>(3, 0)); // Creating a 3x3 vector filled with 0s
In this case, `vec` is initialized as a 3x3 grid where every element is set to `0`. The general structure is to create a vector containing other vectors as its elements.
Accessing Elements
Accessing elements within a 2D vector is straightforward. You use the row and column indices:
vec[0][0] = 10; // Setting the first element
int value = vec[1][1]; // Accessing the element at second row, second column
In this example, the first element of the first row is set to `10`, while the second row, second column's value is assigned to the variable `value`.
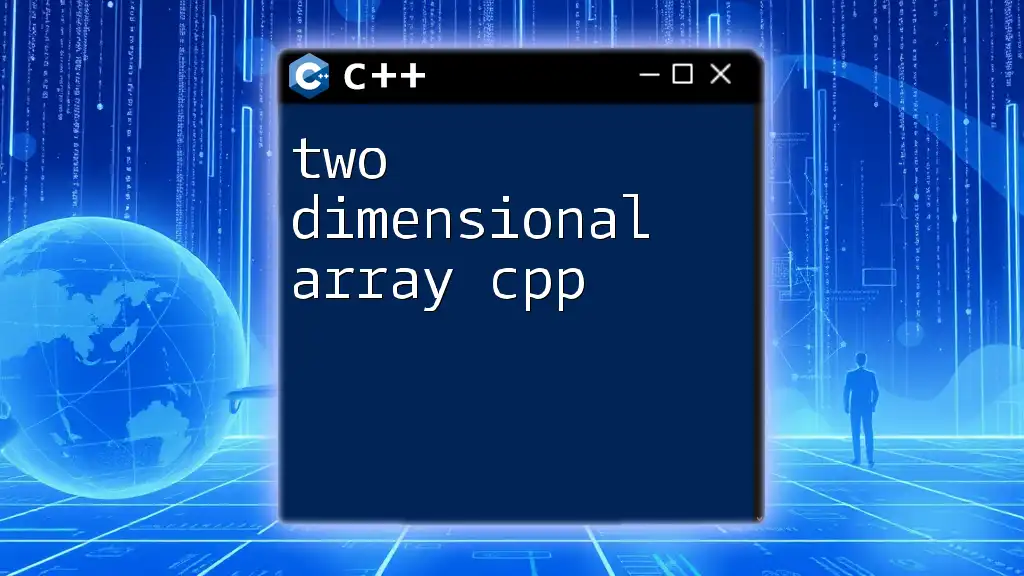
Iterating Over a 2D Vector
Using Nested Loops
To iterate over every element in a 2D vector, nested loops are typically employed.
for (size_t i = 0; i < vec.size(); ++i) {
for (size_t j = 0; j < vec[i].size(); ++j) {
std::cout << vec[i][j] << " ";
}
std::cout << std::endl;
}
This code snippet traverses each row (`i`) and each column (`j`), printing out the elements in a structured manner.
Using Range-Based For Loops
C++11 introduced range-based for loops, which simplify the iteration process by eliminating the need for index management. Here's how you can use a range-based loop:
for (const auto& row : vec) {
for (const auto& elem : row) {
std::cout << elem << " ";
}
std::cout << std::endl;
}
In this example, each row of the 2D vector is accessed directly, providing a clearer and more readable way to iterate through the elements.
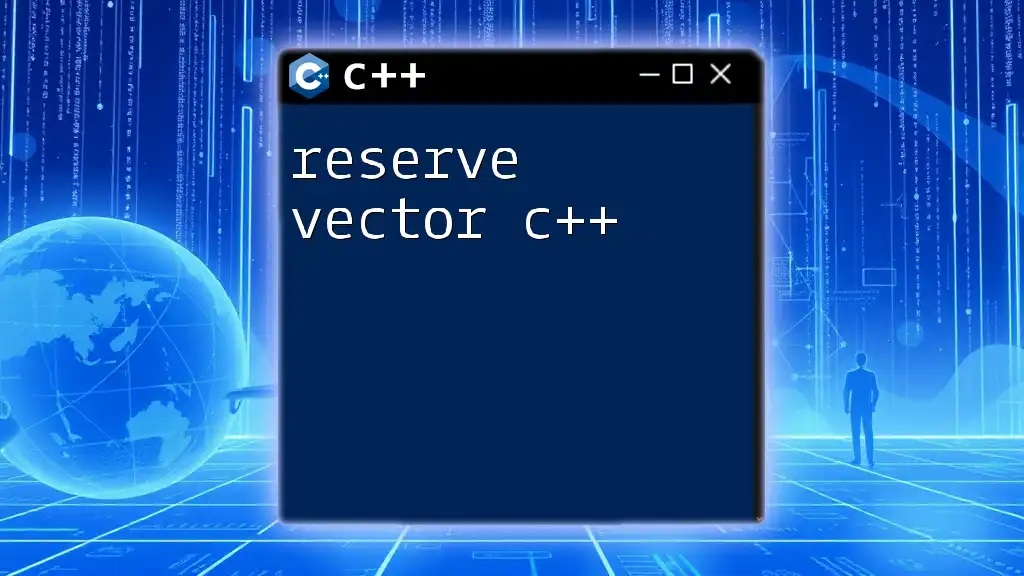
Advanced Operations on 2D Vectors
Resizing 2D Vectors
A significant advantage of using vectors is their ability to resize dynamically. To adjust the size of a 2D vector, you can use the `resize()` method:
vec.resize(4); // Add a new row
for (auto& row : vec) {
row.resize(4, 1); // Each row now has 4 columns filled with 1
}
In this case, a new row is added to the vector, and each row is resized to contain four columns, all initialized with the value `1`.
Transposing a 2D Vector
Transposing a vector involves flipping it over its diagonal, effectively converting rows to columns:
std::vector<std::vector<int>> transpose(vec[0].size(), std::vector<int>(vec.size()));
for (size_t i = 0; i < vec.size(); ++i) {
for (size_t j = 0; j < vec[i].size(); ++j) {
transpose[j][i] = vec[i][j];
}
}
This code snippet creates a new 2D vector called `transpose`, where iterations through the original vector populate it in a transposed manner.
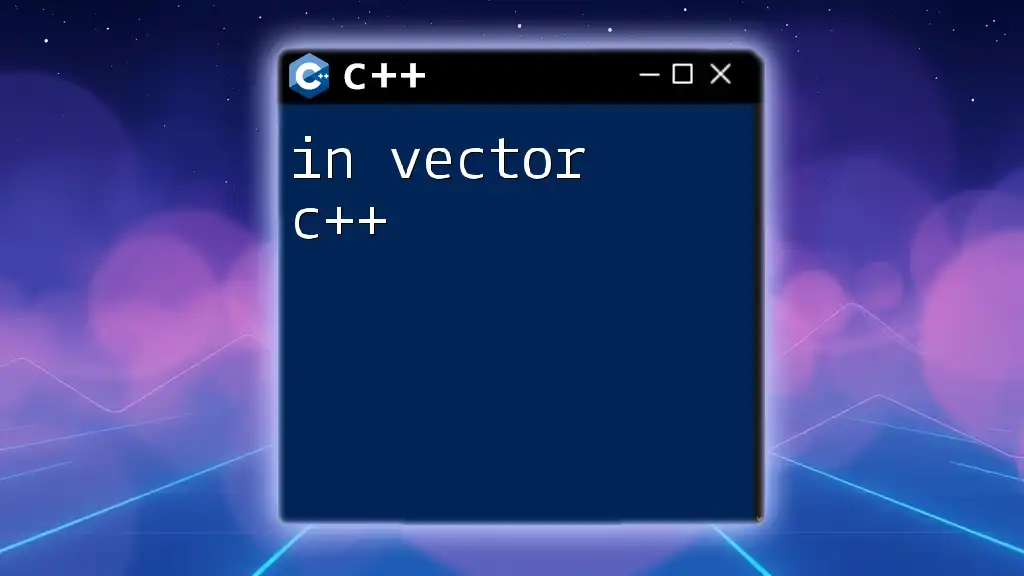
Common Challenges and Solutions
Memory Management
While 2D vectors are powerful, they can consume considerable memory when scaled up to larger sizes. It is essential to manage your memory effectively, especially in environments with limited resources. To mitigate issues:
- Avoid unnecessary copying of vectors where possible, employing references.
- Use `reserve()` for vectors if you know the expected size beforehand to minimize reallocations.
Performance Considerations
When considering performance, it's crucial to weigh the benefits of using 2D vectors against fixed-size arrays. Vectors provide flexibility and ease of use but may incur a performance penalty in scenarios demanding high-speed computations, such as real-time graphics rendering. In such cases, using raw arrays or specialized data structures may yield better performance.
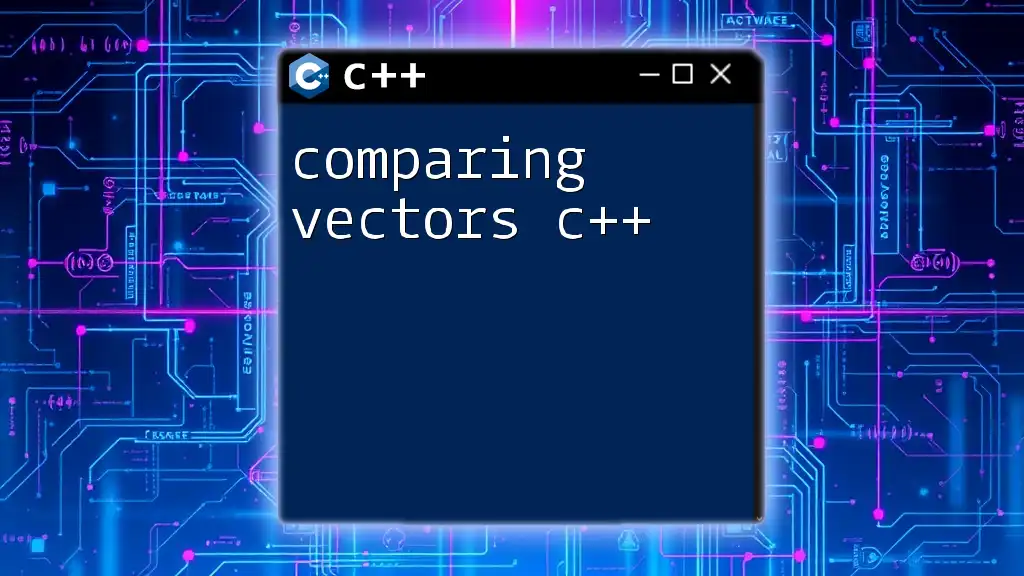
Conclusion
In this guide, we explored the intricacies of 2-dimensional vectors in C++, covering creation, manipulation, and advanced operations. By grasping how to effectively utilize 2D vectors, you can enhance your programming capabilities significantly. Practicing these concepts and applying them in various scenarios will solidify your understanding and proficiency.
Additional Resources
To dive deeper into the topic, visit C++ documentation and online tutorials focused on advanced vector usage. Engaging in C++ programming communities is also a great way to seek support and continue learning.