You can find the maximum element in a C++ vector using the `std::max_element` function from the `<algorithm>` header. Here's a concise example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 3, 5, 7, 2, 8};
auto maxElement = *std::max_element(vec.begin(), vec.end());
std::cout << "The maximum element is: " << maxElement << std::endl;
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can grow or shrink in size during runtime. This makes them highly versatile for various programming tasks. Unlike standard arrays, which have a fixed size, vectors allow you to add or remove elements without worrying about the array size.
The key benefits of using vectors over arrays include:
- Dynamic sizing: Vectors can adjust their size automatically.
- Ease of use: Built-in functions simplify common operations like insertion and deletion.
- STL (Standard Template Library) compatibility: Vectors work seamlessly with STL algorithms, making them a powerful choice for C++ developers.
Declaring and Initializing Vectors
To declare a vector, you need to include the `<vector>` header. The syntax for declaring a vector is as follows:
#include <vector>
std::vector<data_type> vector_name;
You can initialize a vector in several ways, such as:
- Using a list of values:
std::vector<int> numbers = {1, 2, 3, 4, 5};
- Specifying a size with a default value:
std::vector<int> numbers(5, 0); // initializes a vector of size 5 with all elements as 0
In both cases, vectors can hold various data types, including `int`, `float`, `char`, and more.
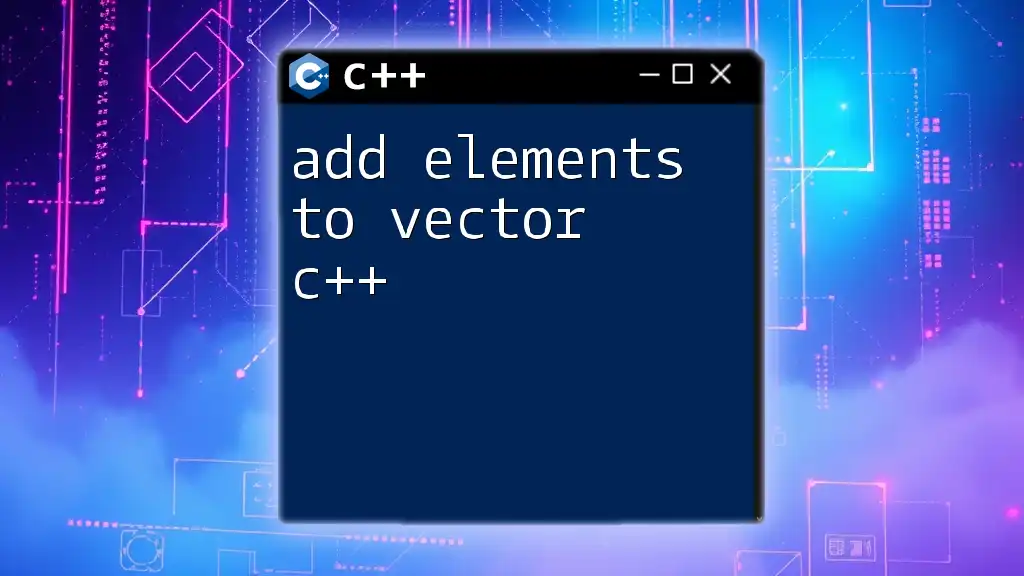
Finding the Maximum Element in a Vector
Why Find the Maximum Element?
Finding the maximum element in a vector holds significant importance in many scenarios. Applications include:
- Data analysis: Extracting insights from numeric datasets.
- Optimizing algorithms: Using maximum values to improve performance in sorting and searching algorithms.
- Game development: Determining the highest score achieved by players.
Methods to Find the Maximum Element
Finding the maximum element in a vector can be accomplished in multiple ways, primarily through iterative methods or STL functions.
Using a Loop
One straightforward method to find the maximum element is through a simple loop. This method involves iterating through the vector and comparing each element to maintain the current maximum value.
Here’s how this can be done:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> numbers = {10, 20, 5, 45, 30};
int maxElement = numbers[0]; // Assume the first element is the max
for (int i = 1; i < numbers.size(); i++) {
if (numbers[i] > maxElement) {
maxElement = numbers[i]; // Update maxElement if current is larger
}
}
cout << "Maximum Element: " << maxElement << endl; // Output the maximum
return 0;
}
In this example, we initial the `maxElement` variable with the first element of the vector. We then iterate through the vector, updating `maxElement` whenever we find a larger value.
Using the `std::max_element` Function
Another efficient method to find the maximum element is through the `std::max_element` function provided in the STL. This function simplifies the task significantly and is optimized for performance.
Here’s how to use it:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<int> numbers = {10, 20, 5, 45, 30};
auto maxElement = *max_element(numbers.begin(), numbers.end()); // Get the max using the iterator
cout << "Maximum Element: " << maxElement << endl; // Output the maximum
return 0;
}
In this example, `max_element` takes two iterators: one pointing to the beginning and the other to the end of the vector. The function returns an iterator to the maximum element, which we dereference to get the actual maximum value.
Comparison of Methods
When comparing these two methods, using a loop can be straightforward and educational, especially for beginners. However, leveraging `std::max_element` is generally more efficient and concise, especially when dealing with larger datasets.
- Loop Method: More control, educationally informative, but less efficient.
- STL Method: Optimized and simpler, best for production code.
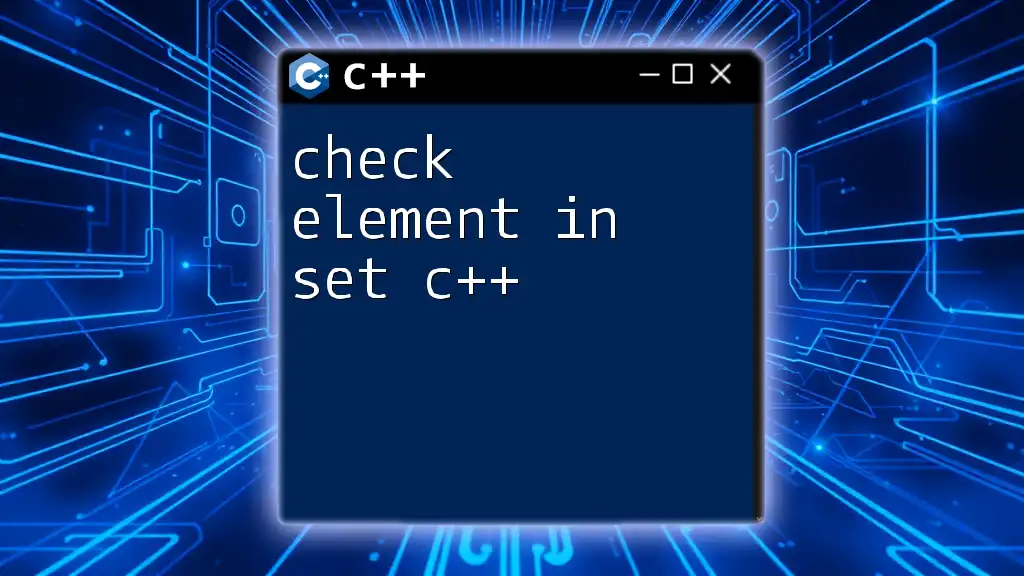
Practical Applications
Finding the Maximum Element in Various Data Types
The approach for finding the maximum element can be adapted to different data types. For example, you can find the maximum in a vector of `float` or `double` simply by replacing the `int` type.
To illustrate, here’s an example of finding the maximum in a vector of `float`:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main() {
vector<float> prices = {19.99, 29.99, 9.99, 49.99, 39.99};
auto maxPrice = *max_element(prices.begin(), prices.end());
cout << "Maximum Price: " << maxPrice << endl;
return 0;
}
Also, you can find the maximum in vectors of `string` using `std::max_element`. The comparison will be based on lexicographical (dictionary) order.
Use Cases in Real-World Applications
In real-world scenarios, identifying the maximum element can be crucial. For example, in statistical analysis, determining the maximum score in a set of exam results can directly impact evaluation methods.
In gaming, the highest score can lead to the leaderboard positions. By efficiently identifying max scores, developers can create a more engaging user experience.
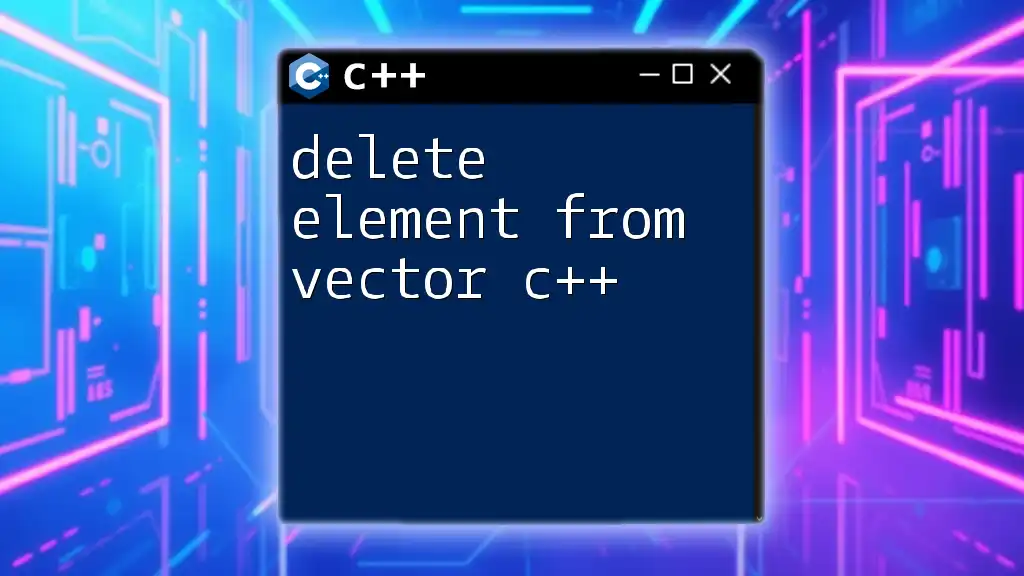
Frequently Asked Questions
What to do if the Vector is Empty?
When dealing with vectors, one important aspect to handle is the possibility of an empty vector. Attempting to find the maximum element in an empty vector may lead to undefined behavior.
To protect against this, always check if the vector is empty before proceeding:
if (numbers.empty()) {
cout << "The vector is empty." << endl;
}
Can I Use Custom Comparison Functions?
Yes, C++ allows the use of custom comparison functions with the `std::max_element` function. This enables you to determine the maximum element based on custom-defined criteria.
Here’s an example of using a custom comparator with a user-defined struct:
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Product {
string name;
float price;
};
// Comparator to find the most expensive product
bool compareByPrice(const Product& a, const Product& b) {
return a.price < b.price; // Lower price comes first, for max, reverse the order
}
int main() {
vector<Product> products = {{"Product A", 19.99}, {"Product B", 29.99}, {"Product C", 9.99}};
auto maxProduct = *max_element(products.begin(), products.end(), compareByPrice);
cout << "Most Expensive Product: " << maxProduct.name << " at $" << maxProduct.price << endl;
return 0;
}
In this case, we defined a `Product` structure and implemented our custom comparison function. The `std::max_element` function can then leverage this comparator to find the maximum product based on price.
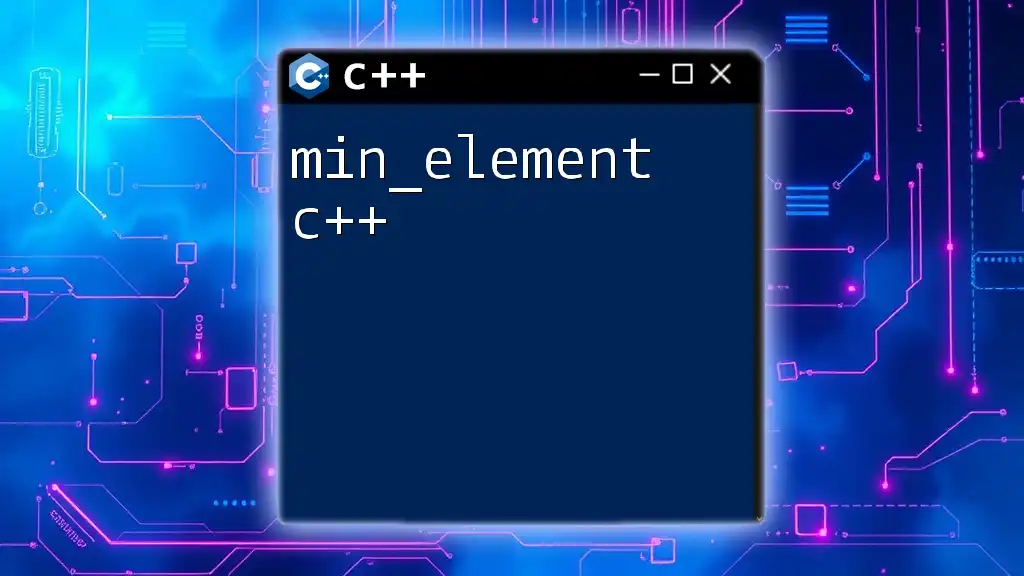
Conclusion
Understanding how to find the max element in a vector in C++ is a vital skill for any programmer. Whether using loops or leveraging standard library functions like `std::max_element`, knowing when to use each approach can improve your coding efficiency and elegance.
Practice finding maximum elements in different scenarios, and explore the rich features of C++ for effective software development. Don’t hesitate to look into further resources or courses to strengthen your knowledge and skills in C++.