To delete an element from a vector in C++, you can use the `erase()` method along with an iterator to specify the position of the element to be removed.
Here’s a code snippet demonstrating how to delete an element from a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Deletes the element at index 2 (the value 3)
for (int i : vec) {
std::cout << i << " "; // Output: 1 2 4 5
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can resize itself automatically when elements are added or removed. Vectors are part of the Standard Template Library (STL) and offer flexibility and ease of use compared to traditional arrays. One key feature of vectors is that they maintain the order of elements and allow random access, making them a powerful choice for many applications.
Common Operations on Vectors
Vectors support a variety of operations that facilitate efficient data management:
- Adding elements: You can easily add new elements using the `push_back()` method.
- Accessing elements: Elements can be accessed directly using indexing, which gives you the ability to retrieve or modify elements quickly.
- Size and capacity: The `size()` method returns the number of elements currently stored in the vector, while `capacity()` indicates how many elements the vector can hold before needing to resize.
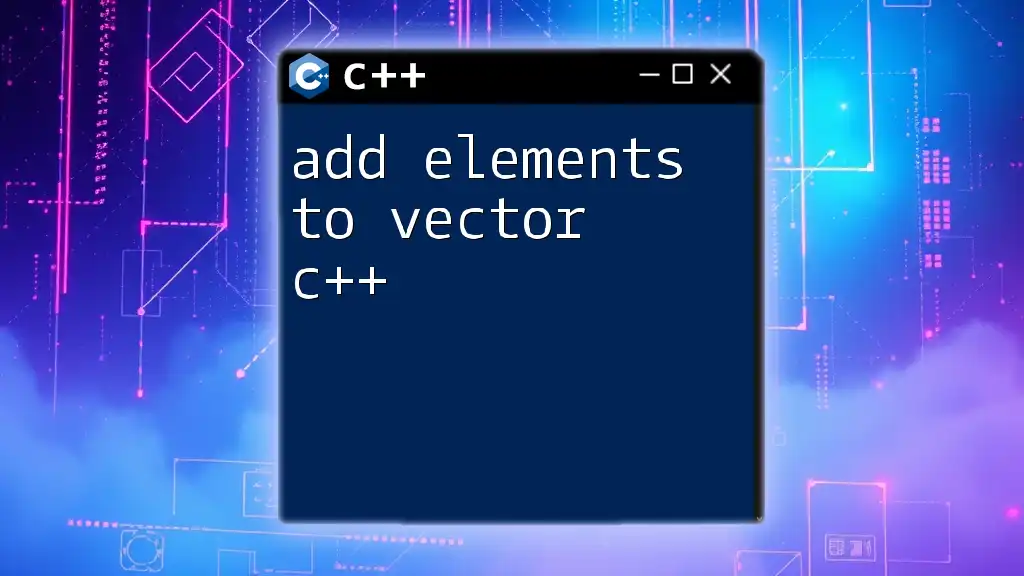
How to Remove an Element from a Vector in C++
Removing an element from a vector can be necessary when you want to ensure that only relevant data remains. Below are the most common methods for deleting elements from a vector in C++.
Deleting Elements using `erase()`
The `erase()` method is an effective way to delete an element from a vector by its position.
- Syntax:
vector_name.erase(position);
Understanding how to locate the position of the element you wish to erase is crucial. Below is a practical example:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Removes the element at index 2 (which is '3')
for (int i : vec) {
std::cout << i << ' '; // Output will be 1 2 4 5
}
return 0;
}
In this example, we demonstrate how to remove the element `3` located at index `2`. After the removal, the vector retains its order, and the output confirms the successful deletion.
Deleting by Value Using `remove()`
Figuring out how to remove elements by their value is another important aspect of managing vectors. However, `std::remove` does not actually erase elements; instead, it moves the elements to the front and returns an iterator to the new end of the "logical" vector.
-
Combining `remove()` and `erase()`: You'll typically use `remove()` in conjunction with `erase()` to achieve the desired result.
-
Syntax:
remove(vector_name.begin(), vector_name.end(), value); vector_name.erase(new_end_position, vector_name.end());
Here's an illustrative example:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 3, 5};
vec.erase(std::remove(vec.begin(), vec.end(), 3), vec.end()); // Remove all '3's
for (int i : vec) {
std::cout << i << ' '; // Output will be 1 2 4 5
}
return 0;
}
In the code above, every instance of `3` is removed from the vector. The `remove()` function effectively shifts the elements to eliminate the specified value, but you must use `erase()` to clean up the excess elements remaining.
Removing All Elements from a Vector
If your goal is to start fresh and remove all elements from a vector, the `clear()` method can help you accomplish this easily.
- Syntax:
vector_name.clear();
Consider this example:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.clear();
std::cout << "Size after clear: " << vec.size(); // Output will be 0
return 0;
}
After invoking `clear()`, all elements in the vector are removed, resulting in a size of zero. This method is often preferred when the complete data set is no longer needed.
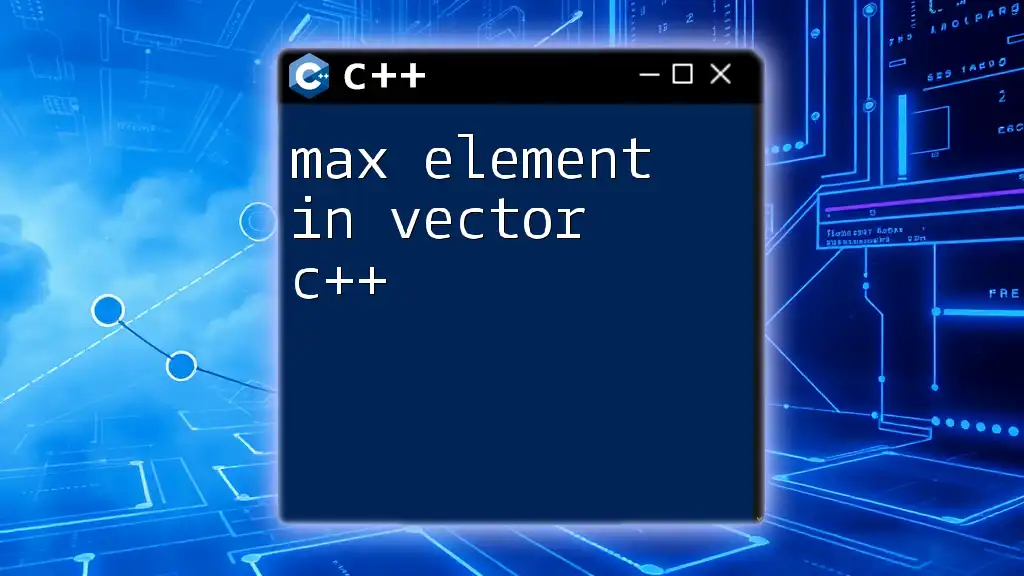
Best Practices for Deleting Elements
Avoiding Iterator Invalidations
When elements are deleted from a vector, iterators pointing to these elements may become invalid. This can lead to unexpected behavior if you continue using the invalidated iterators.
To mitigate this, always ensure that iterators are updated after a deletion operation. If you're iterating through a vector while removing elements, it's wise to consider using a loop that adjusts correctly to the changing size of the vector.
Performance Considerations
When deciding how to delete elements, it’s essential to consider the performance impacts. The complexity of the `erase()` operation is linear (O(n)), which means that the larger the vector, the longer it will take. In contrast, `remove()` is often more efficient due to not immediately resizing the vector but will still require you to use `erase()` as a follow-up step.
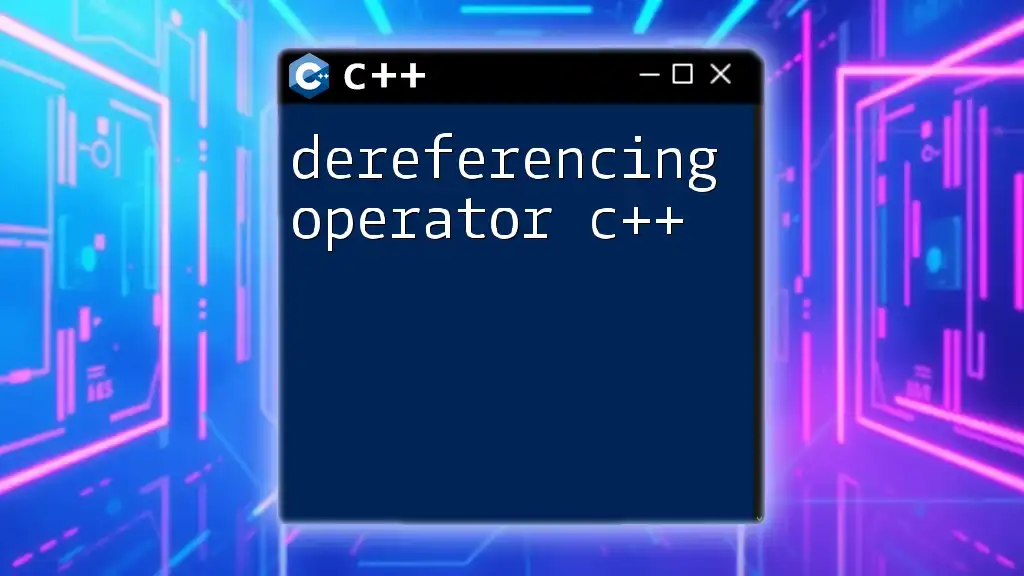
Common Errors and Troubleshooting
Compilation Errors
When working with vectors, it's crucial to ensure you include the necessary headers, such as `<vector>` and `<algorithm>`. Failure to do so will often result in compilation errors related to undefined functions.
Logical Errors
Logical errors can occur when an algorithm is not implemented correctly. For instance, using `remove()` without `erase()` will not actually remove elements from the vector, potentially causing confusion. Always verify that the desired outcome has been achieved by checking the vector's contents after performing deletion operations.
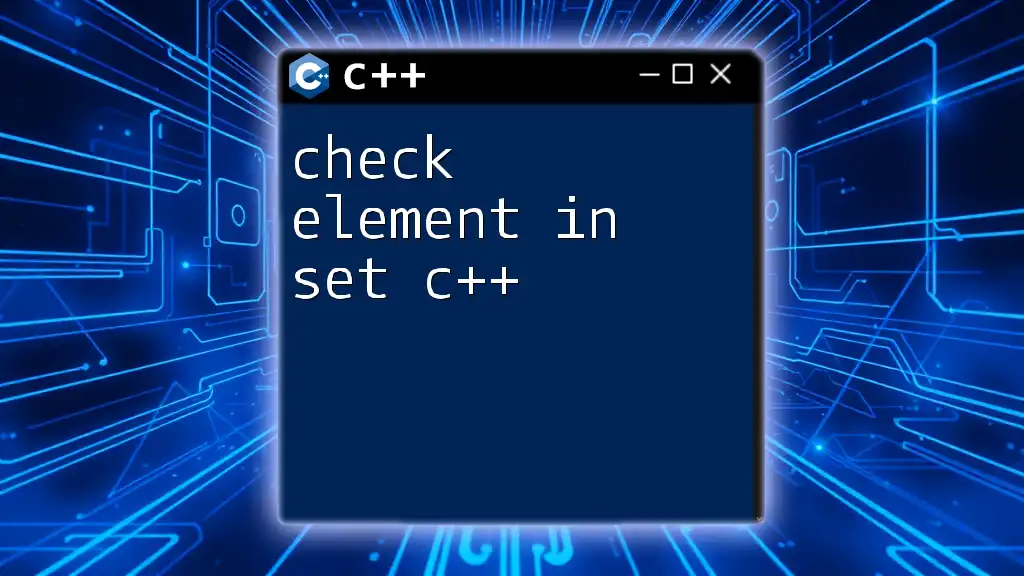
Conclusion
In summary, knowing how to delete an element from a vector in C++ is crucial for effective data management. Utilizing methods such as `erase()`, `remove()`, and `clear()`, you can maintain and manipulate your data efficiently. Practice these concepts to build fluency in working with vectors in C++, and always be mindful of best practices to avoid common pitfalls.
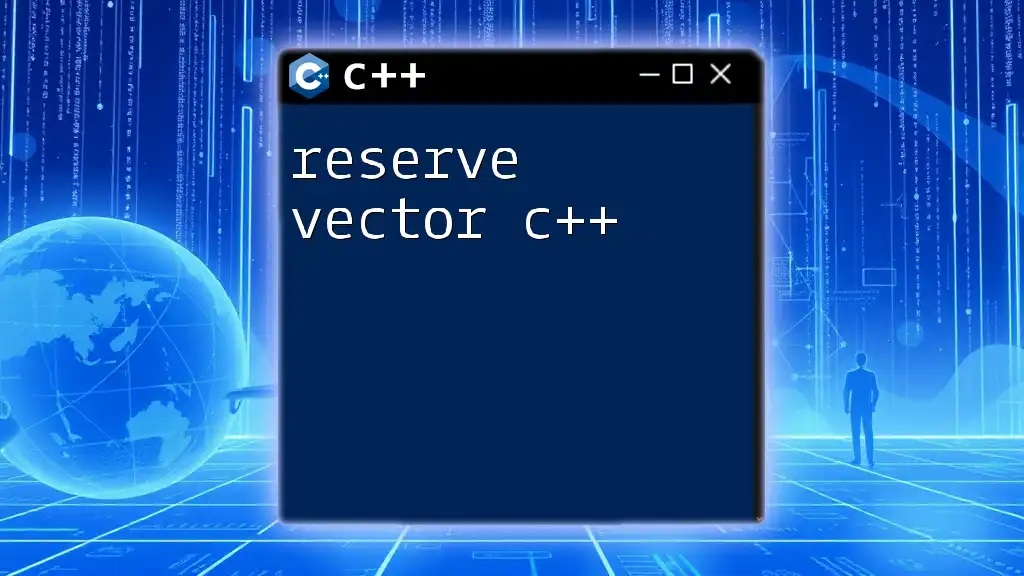
Call to Action
Share your experiences with deleting elements from vectors or ask questions about specific scenarios you are encountering. Engaging with the community can lead to a deeper understanding and better solutions. Happy coding!