In C++, you can check if an element exists in a set using the `find` method, which returns an iterator to the element if found, or `end()` if not found. Here’s a quick example:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
int element = 3;
if (mySet.find(element) != mySet.end()) {
std::cout << "Element found!" << std::endl;
} else {
std::cout << "Element not found!" << std::endl;
}
return 0;
}
What is a Set in C++?
A set in C++ is a container that stores unique elements following a specific order. The C++ Standard Library provides the `set` class that implements a collection of objects, primarily supporting fast retrieval. Sets are characterized by their key properties:
- Unordered: The elements in a set are not stored in any particular order.
- Unique elements: A set cannot contain duplicate values, ensuring that each element remains distinct.
Understanding these fundamental properties is crucial when you need to check an element in a set because they significantly influence how we perform operations on these containers.
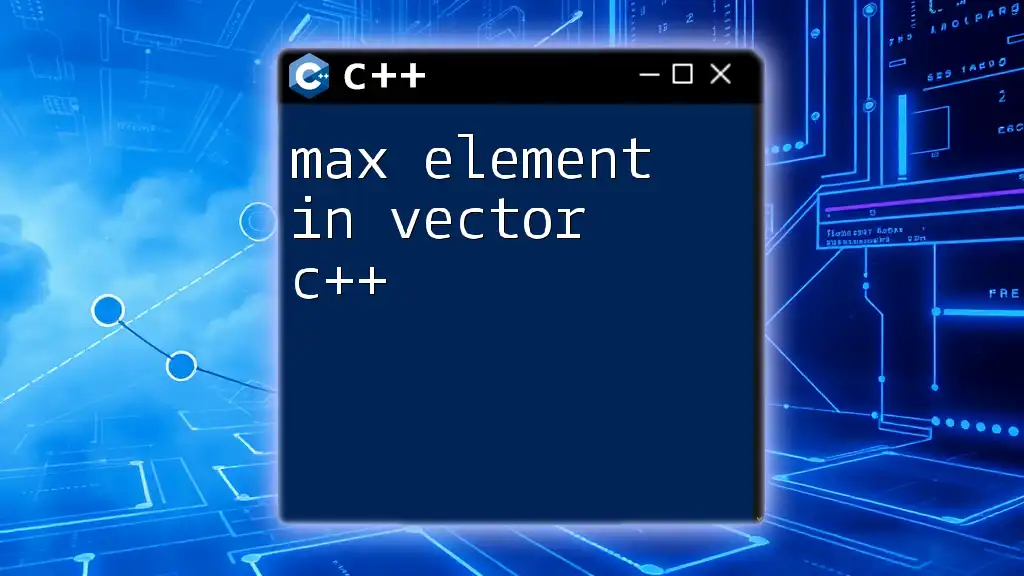
Importance of Checking Elements in Sets
Checking for the presence of an element in a set is essential in various scenarios. For instance, when developing algorithms that require quick look-up times to determine membership, sets can significantly improve performance.
When dealing with a collection of data that requires uniqueness, using sets not only simplifies your code but also enhances efficiency. Since operations on sets usually have a time complexity of O(log n) on average, they are preferable to other data structures when needing to frequently check for element presence.
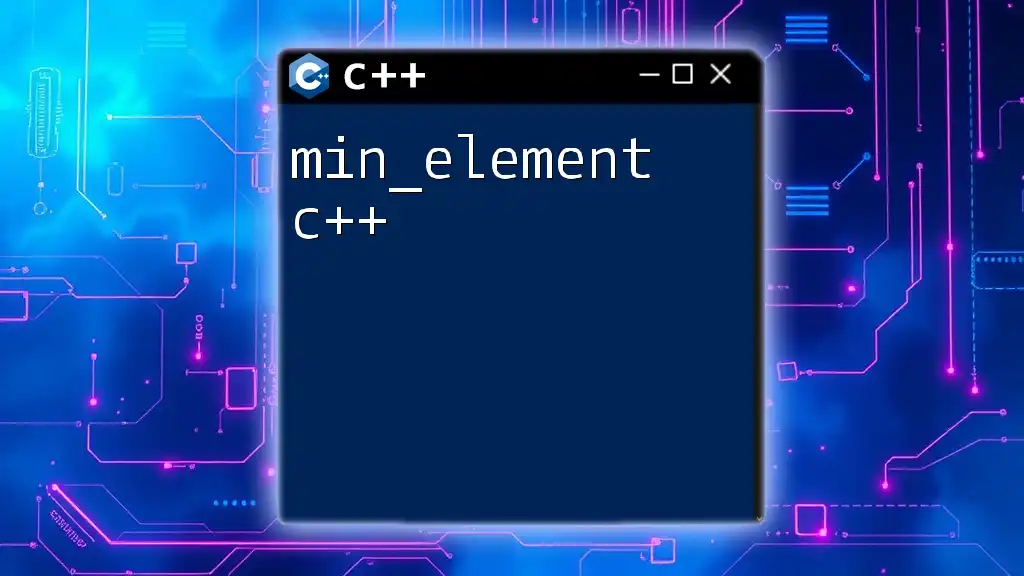
How to Declare a Set in C++
Basic Syntax for Set Declaration
To create a set in C++, you'll need to include the `<set>` header file, which provides the functionality of sets. Here’s how to declare an empty set:
#include <set>
std::set<int> mySet;
Initializing a Set with Values
You can also initialize a set with predefined values, making it easier to work with in applications needing immediate data. An example of initializing a set with integers is as follows:
std::set<int> mySet = {1, 2, 3, 4, 5};
In this example, `mySet` will contain the elements 1, 2, 3, 4, and 5, all unique inherently due to the nature of sets.
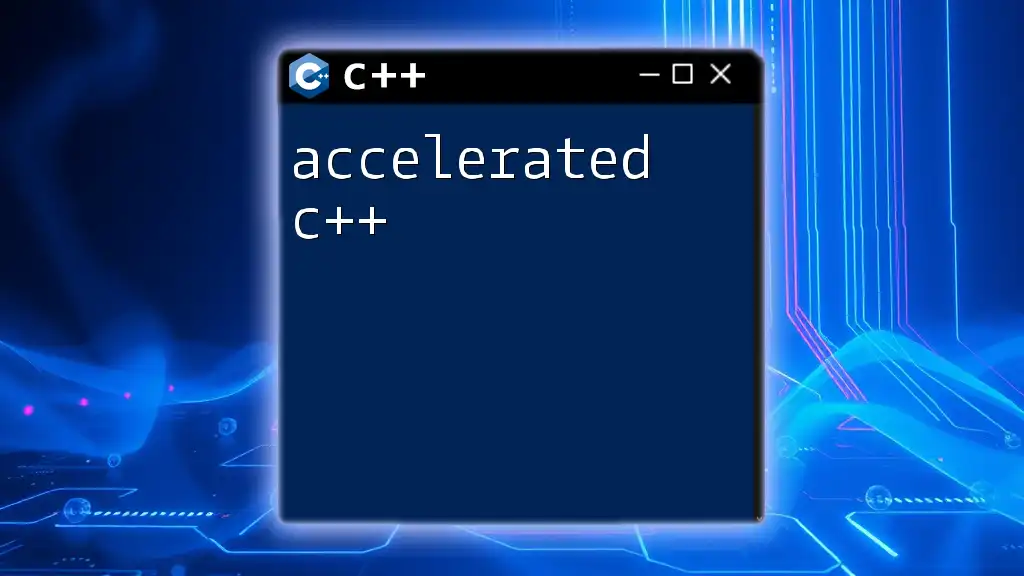
Checking for Elements in a Set
Using the find() Function
The `find` function is one of the primary methods used to check an element in a set. This function searches for the specified element and returns an iterator pointing to the element if found, or `end()` if not found.
Overview of find() Function
Here is how the `find` function can be utilized in code:
auto it = mySet.find(3);
if (it != mySet.end()) {
std::cout << "Element found!" << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
In this example, we check for the presence of the integer `3` in `mySet`. If found, the output will be "Element found!" Otherwise, it will report that the element is not present. This method provides a straightforward yet effective way to determine membership in a set.
Using the count() Function
Another way to check if an element exists in a set is by using the `count` method. This approach is particularly straightforward. The `count` function returns the number of occurrences of a specified element in the set.
Overview of count() Function
Here is how you can implement the `count` function in your code:
if (mySet.count(4) > 0) {
std::cout << "Element exists in the set." << std::endl;
} else {
std::cout << "Element does not exist." << std::endl;
}
In this case, if the integer `4` exists within `mySet`, your program will print "Element exists in the set," confirming the presence of that value. If it does not exist, the program will state that the element is missing.
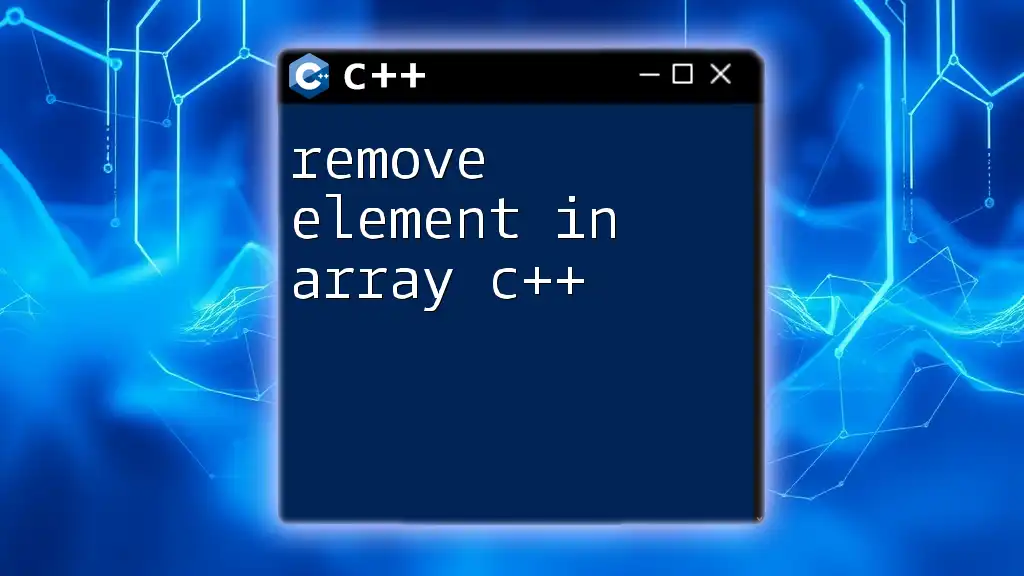
Performance Considerations
Time Complexity for Searching
When deciding to check an element in a set, understanding the performance associated with set operations is key. The average time complexity for both the `find` and `count` methods is O(log n), which ensures efficiency even with larger datasets. This efficiency is a critical reason why sets are preferred for certain applications.
Comparison with Other Data Structures
When comparing sets to other data structures such as vectors or lists, sets generally outperform in terms of lookup speed. While searching for an element in a vector often requires a linear search, resulting in a time complexity of O(n), a set's logarithmic complexity allows for faster operations under similar circumstances.
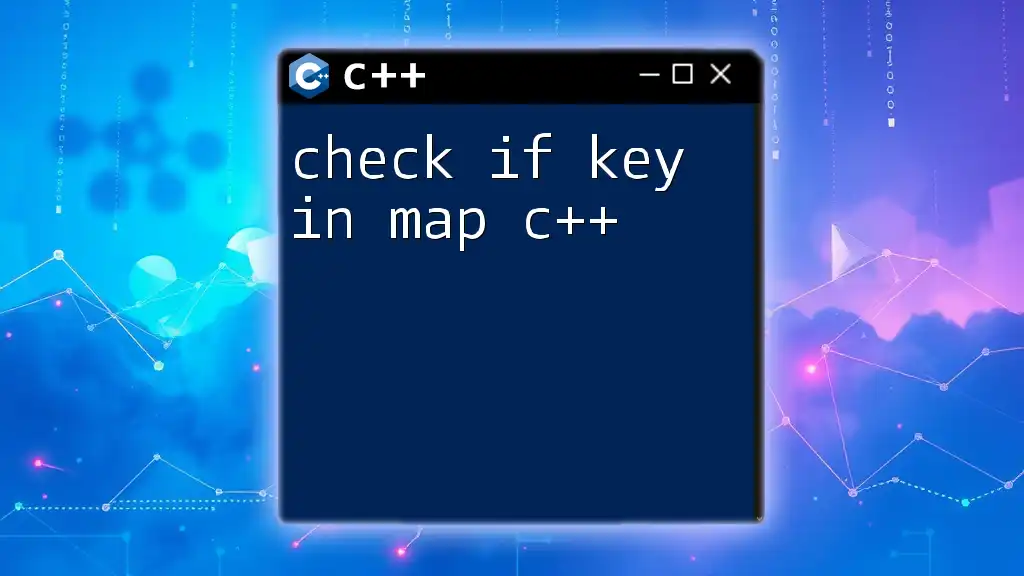
Conclusion
In this guide, we've discussed how to check an element in a set using C++. We've covered the basic structure of sets, the significance of checking element presence, and detailed methods like `find()` and `count()`. Understanding these methods not only facilitates enhanced programming efficiency but also allows developers to better manage collections of unique data types in their applications.
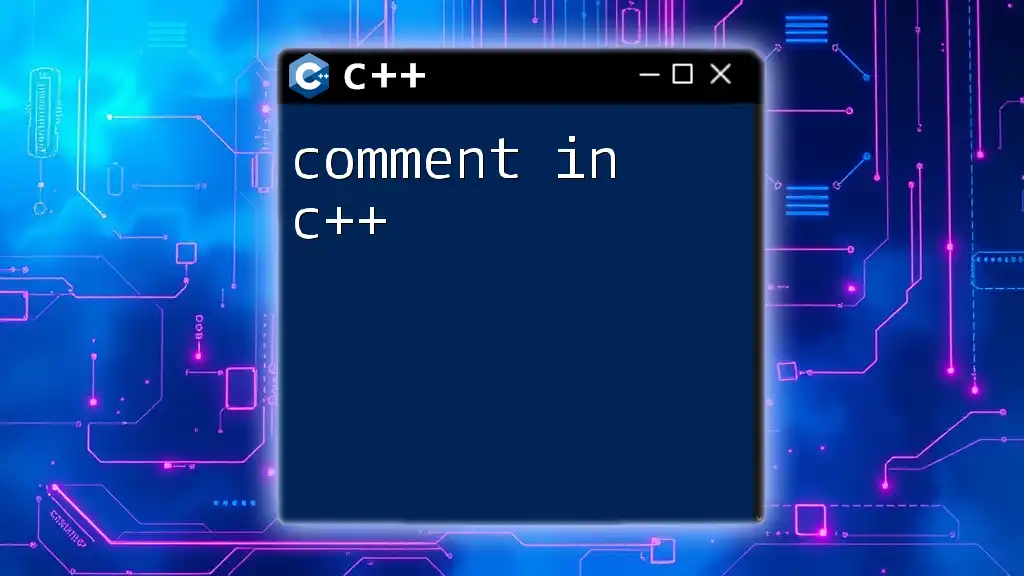
Additional Resources
For those looking to deepen their knowledge, exploring advanced topics such as the C++ Standard Template Library (STL) can offer valuable insights. Additionally, learning about advanced set operations like unions, intersections, and differences will further augment your proficiency with C++ sets.