To check the version of C++ being used by your compiler, you can use the following preprocessor directive:
#include <iostream>
int main() {
std::cout << "C++ version: " << __cplusplus << std::endl;
return 0;
}
Understanding C++ Versions
C++ has evolved significantly over the years, with various standards introducing new features and enhancements. The primary versions you might encounter include C++98, C++03, C++11, C++14, C++17, C++20, and the upcoming C++23. Each version brought its own set of capabilities, affecting everything from performance to coding practices.
Importance of Versioning
Knowing which C++ standard you are working with is crucial for several reasons:
- Feature Availability: Different versions support different features. For instance, C++11 introduced lambda expressions and smart pointers, while C++17 brought in structured bindings and std::optional.
- Performance Improvements: Newer standards often provide optimizations that can enhance the efficiency of your code.
- Library Compatibility: Many libraries rely on specific standards, making it essential to use the correct version.
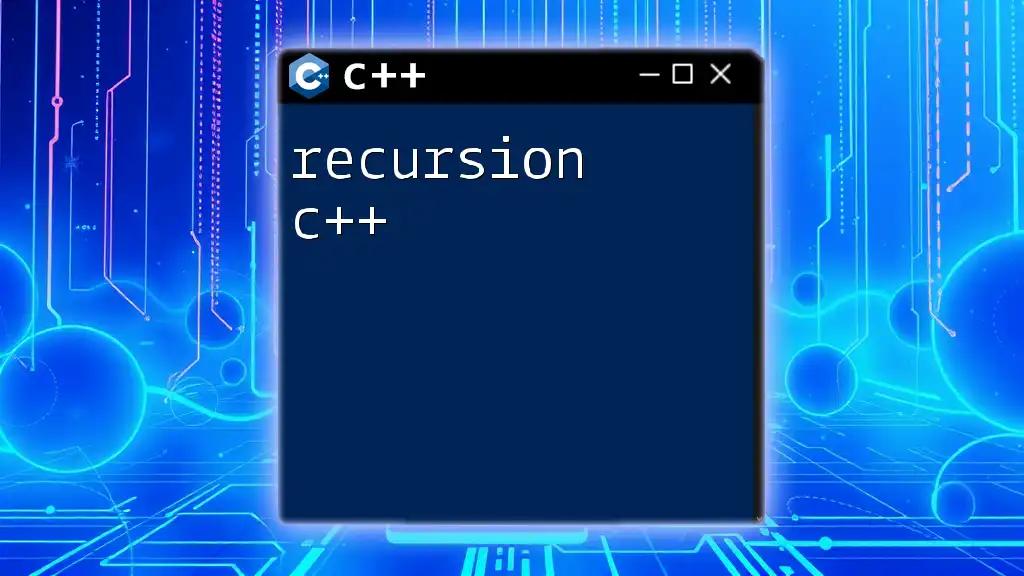
Methods to Check C++ Version
Using Predefined Macros
C++ provides predefined macros that you can leverage to identify the current version your compiler is using. The most common method is to utilize the `__cplusplus` macro.
Example: Checking Version with `__cplusplus`
You can easily check your C++ version by writing a simple program:
#include <iostream>
int main() {
std::cout << "__cplusplus: " << __cplusplus << std::endl;
return 0;
}
Explanation
When you compile and run this code, the output will be an integer that represents the C++ version, such as:
- C++98: `199711L`
- C++11: `201103L`
- C++14: `201402L`
- C++17: `201703L`
- C++20: `202002L`
Using the `__cplusplus` macro is a straightforward way to verify which version of C++ your compiler supports.
Compiler-Specific Commands
In addition to using macros, checking the version of C++ can often be done through compiler commands. Below are commands for some of the most popular compilers.
Checking with GCC
You can find out the version of GCC and its supported C++ standard using:
g++ --version
Explanation
The output will provide information about the GCC version, which is crucial because different versions offer varying levels of C++ standard compliance.
Checking with Clang
Similarly, if you are using Clang, you can run:
clang++ --version
Explanation
As with GCC, this command informs you of the version of Clang and its capabilities concerning C++ standards.
Checking with MSVC (Visual Studio)
For those working in a Visual Studio environment, checking the intended C++ version can be a bit different. You can include a specific pragma message in your code to indicate the version:
#pragma message ("Compiling with C++ version: " __cplusplus)
Explanation
This will output a message during compilation, letting you know which C++ version is being utilized.

Interpreting C++ Version Information
Understanding the output from version commands is important. For example, if the compiler reports that it supports C++14, you can leverage features from that version. If you need features from C++17 but your compiler only supports up to C++14, you have a compatibility issue that you need to address.
In addition, keep in mind the differences in compiler implementations. Not all compilers support the latest C++ standard, and some may provide partial support.
Cross-Platform Considerations
If your software needs to run across multiple platforms, it’s essential to ensure that the code is compatible with various compilers and their respective supported C++ versions. For example, code that compiles perfectly on GCC might not compile with MSVC if you're using the latest C++ features exclusive to certain standards.
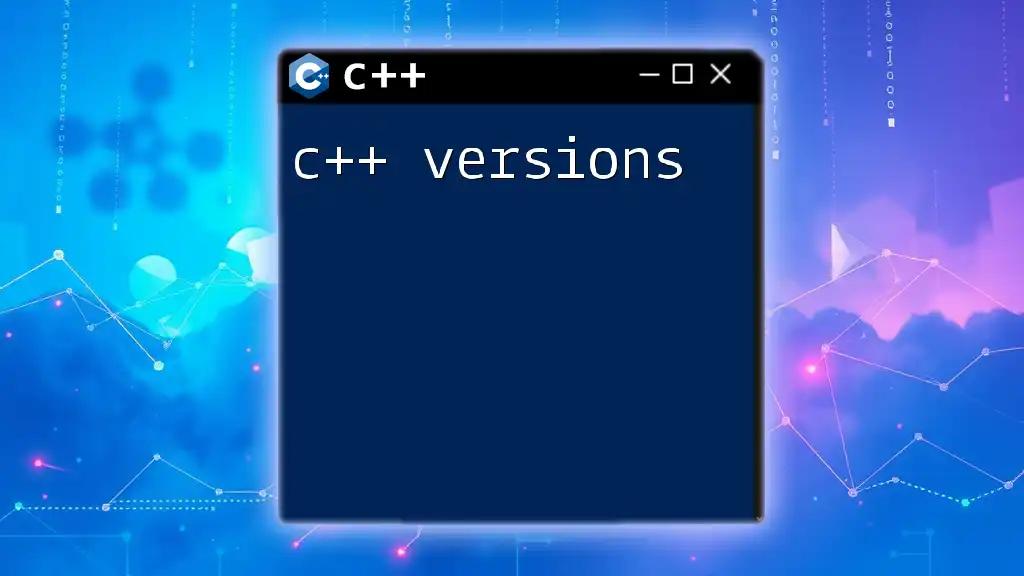
Best Practices for Managing C++ Versions
Setting Up Compiler Flags
To ensure that you can utilize the features of the correct C++ version, it’s vital to set up your compiler flags accordingly. Here’s how you can enable different C++ versions in GCC:
g++ -std=c++11 main.cpp
g++ -std=c++14 main.cpp
g++ -std=c++17 main.cpp
Explanation
These flags explicitly tell the compiler which C++ standard to use. If you omit these flags, the compiler may default to an older version, potentially leading to issues.
Documentation and Code Maintenance
Keeping your code compliant with a specific C++ version can prevent compatibility issues as your project evolves. Documenting dependencies, including the required C++ version in your project's README or documentation, can aid others (and future you) in understanding the context of the code.
Including Version Check in Code
It’s also a good practice to include checks within your code to ensure that you are compiling with the appropriate C++ standard:
#if __cplusplus < 201703L
// Legacy code handling
#endif
Explanation
This conditional compilation allows for legacy functionality if the code is compiled with an older C++ version, which can help maintain older codebases and add compatibility for newer features.
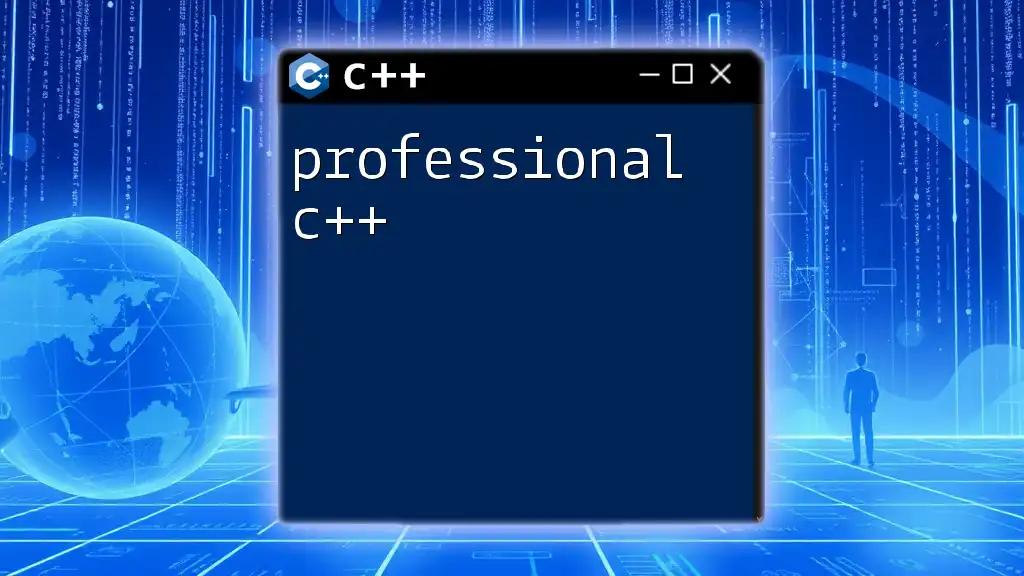
Common Issues and Troubleshooting
Version Mismatch Problems
A common issue developers encounter is a version mismatch between the code and the compiler. If your code uses a feature from C++17 but your compiler only supports C++14, you will run into errors.
To resolve these issues, make sure to do the following:
- Check your compiler version regularly.
- Update your compiler if necessary to obtain the latest C++ standards.
- Revisit your code to ensure that it only uses features available in the specified version.
Compiler Support Limitations
In some cases, you may find that certain features available in a standard are not implemented by a specific compiler. It’s beneficial to consult the documentation for your compiler to understand any limitations.
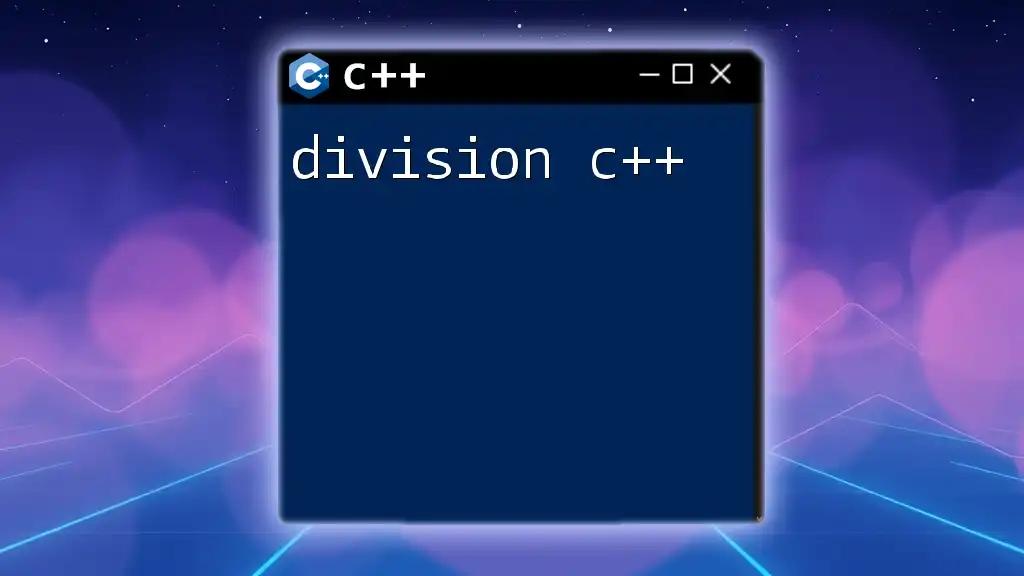
Conclusion
Being able to check the version of C++ you are working with is not only a best practice but essential for effective development. By understanding how to utilize predefined macros and compiler commands, you can ensure that you are always working with the correct features and avoid compatibility issues. Proper management of C++ versions, including setting flags and maintaining documentation, allows for cleaner and more efficient code. Utilize the methods discussed here, and you will be well-equipped to navigate the evolving landscape of C++ development.
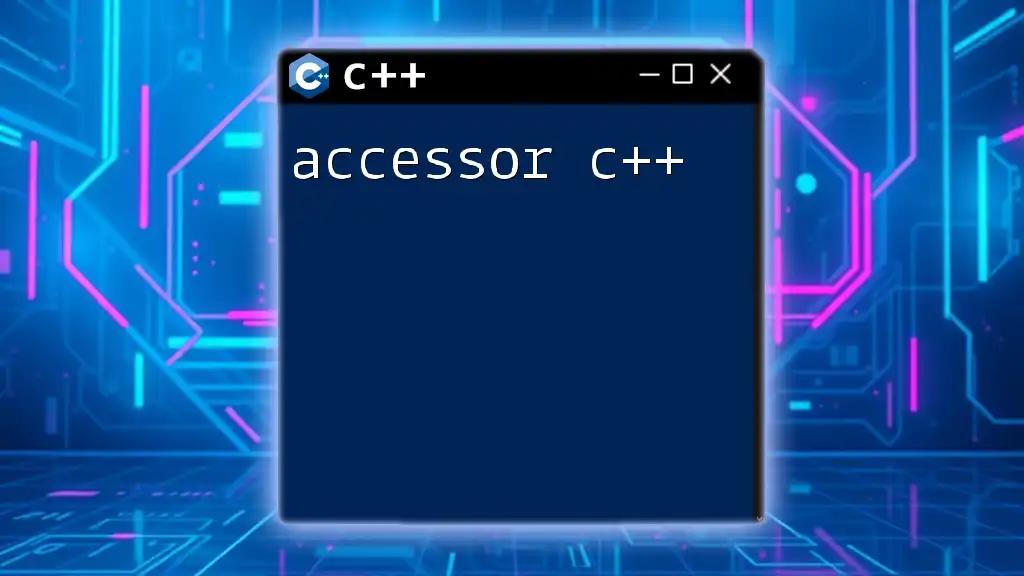
Additional Resources
For further exploration of C++ versions and features, consider checking out the official documentation for each compiler you use and reliable resources like the ISO C++ Standards website and community forums for ongoing discussions and updates.