C++ is a versatile, high-level programming language that supports object-oriented, procedural, and generic programming paradigms, enabling developers to create efficient and reusable code.
Here's a simple example demonstrating a property of C++ that defines a class and instantiates an object:
#include <iostream>
using namespace std;
class Car {
public:
string model;
int year;
void displayInfo() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
int main() {
Car myCar;
myCar.model = "Toyota";
myCar.year = 2022;
myCar.displayInfo();
return 0;
}
General Properties of C++
Object-Oriented Language
C++ is an object-oriented programming language that promotes a methodical approach to software development. The core concepts of Object-Oriented Programming (OOP)—such as classes, objects, inheritance, polymorphism, and encapsulation—enable developers to create modular and maintainable code.
Classes are blueprints for creating objects, encapsulating data and functions that operate on that data. Objects are instances of classes. Here is a simple code example:
class Animal {
public:
virtual void sound() { cout << "Animal sound"; }
};
class Dog : public Animal {
public:
void sound() override { cout << "Bark"; }
};
In this example, `Dog` inherits from `Animal`, demonstrating both inheritance and polymorphism. You can create new animal types later without altering existing code, enhancing code maintainability.
Platform Independence
One of the remarkable properties of C++ is its platform independence. Code written in C++ is portable across various operating systems, thanks to standardized compilers available for different platforms. By adhering to the C++ standards, developers can write code once and compile it everywhere, which saves time and effort.
Performance and Efficiency
C++ is known for its performance and efficiency. Unlike many high-level languages, C++ provides low-level memory management capabilities, allowing developers to optimize performance.
Understanding pointers and references crucially impacts performance, as it allows control over memory and CPU usage. For example, you can dynamically allocate memory when needed using the `new` keyword:
int main() {
int* ptr = new int(5);
cout << *ptr; // Output: 5
delete ptr;
}
This manual memory management feature can enhance performance but also requires care to avoid memory leaks.

Key Properties of C++ Data Types
Static Typing
C++ uses static typing, which means variable types are known at compile time. This type safety helps avoid runtime errors associated with type mismatches. For instance, if you declare an integer as follows, it cannot hold a string:
int a = 5; // Static typing
If you attempt to assign a string, the compiler will raise an error, ensuring that type inconsistencies are caught early.
Rich Set of Built-In Data Types
C++ comes with a rich set of built-in data types including primitive types like `int`, `float`, and `char`, as well as user-defined types such as structs and classes.
For example, you can define a simple structure like this:
struct Point { int x; int y; };
This ability to create new data types helps organize and manage data effectively in larger applications.
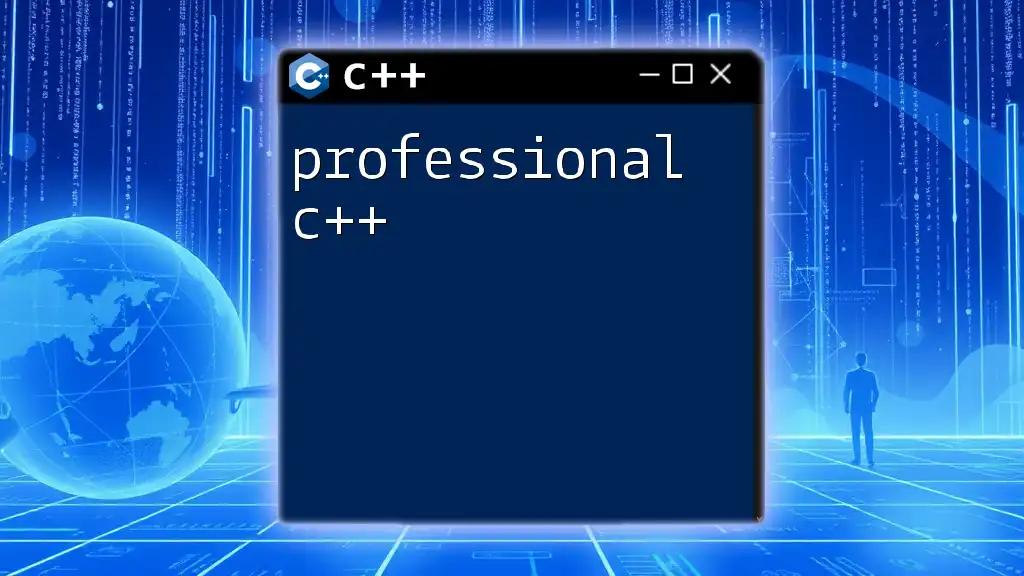
Memory Management in C++
Manual Memory Management
C++ provides manual memory management capabilities through dynamic memory allocation. By using `new` and `delete`, you can allocate and free memory at your discretion.
While this gives you powerful control, it also introduces the risk of memory leaks if you forget to `delete` allocated memory. Being prudent with memory management ensures that your applications are efficient.
int* arr = new int[10]; // dynamic allocation
delete[] arr; // freeing memory
Smart Pointers
To combat issues related to manual memory management, C++11 introduced smart pointers. Smart pointers are classes that manage object lifetime and automatically handle deallocation.
A popular variant is `std::unique_ptr`, which ensures that memory is released when it goes out of scope:
std::unique_ptr<int> ptr(new int(5));
This feature reduces the risk of memory leaks and enhances code safety and maintainability.
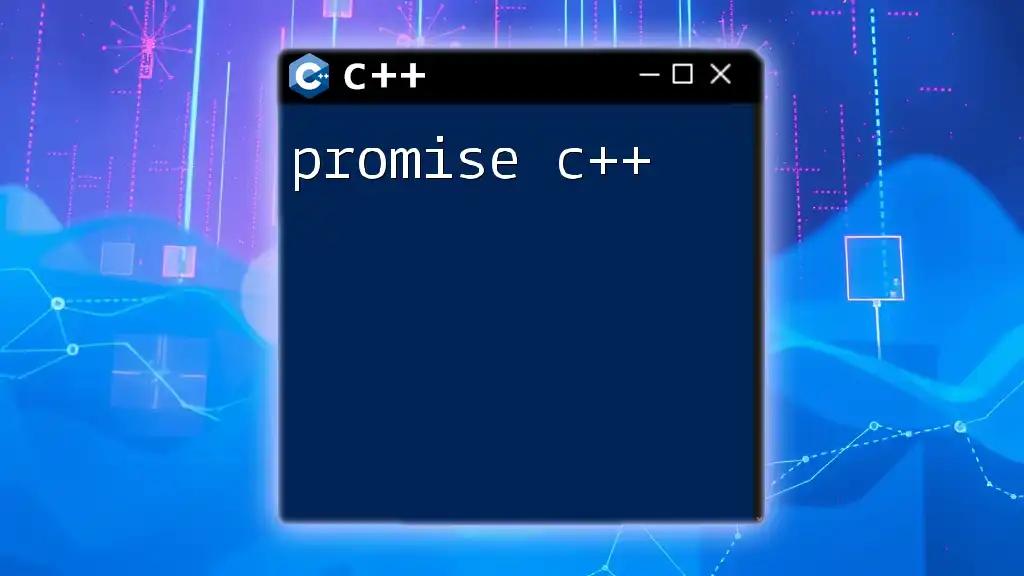
C++ Standard Library
STL Overview
The Standard Template Library (STL) is a crucial part of C++, providing a rich set of components. The STL includes algorithms, iterators, and data structures designed to simplify the development of complex systems.
Collection Types
C++ offers various built-in collection types like vectors, lists, maps, and sets. For example, you can create a dynamic array using a vector:
#include <vector>
std::vector<int> v = {1, 2, 3};
Vectors provide automatic memory management, resizing capabilities, and efficient random access, making them a popular choice among developers.
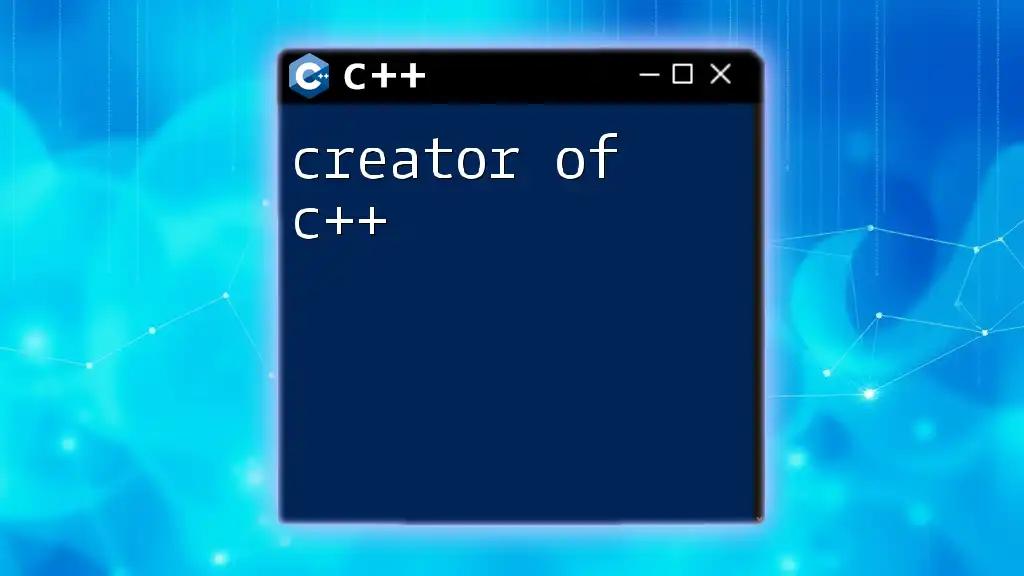
Advanced C++ Properties
Operator Overloading
One of the unique properties of C++ is operator overloading, which allows you to define how standard operators (like +, -, etc.) work with user-defined types. This provides a way to use operators in a way that is intuitive for your classes.
Here is an example of operator overloading for a simple `Complex` number class:
class Complex {
public:
int real, imag;
Complex operator + (const Complex& obj) {
return {real + obj.real, imag + obj.imag};
}
};
This capability makes code more readable and user-friendly, as you can use expressions rather than explicit method invocations.
Templates and Generics
Templates offer a way to create functions and classes that operate with any data type, which introduces powerful generic programming capabilities. This promotes code reusability and flexibility.
Here is a simple template function that adds two values:
template <typename T>
T add(T a, T b) {
return a + b;
}
You can use this template with any data type, from integers to custom classes.
Exception Handling
Robust error handling is essential in software development, and C++ offers mechanisms for exception handling to manage runtime errors gracefully. By using `try`, `catch`, and `throw`, you can provide a clear flow for error management.
Consider this example:
try {
throw std::runtime_error("Error!");
} catch (const std::exception& e) {
std::cout << e.what();
}
This encapsulates error-producing code in a `try` block while handling any exceptions that arise in the `catch` block, promoting more stable and maintainable applications.

Conclusion
Understanding the properties of C++ is crucial for any programmer looking to master this powerful language. From its object-oriented features to manual memory management and robust error handling, knowing these properties helps you write efficient, maintainable, and scalable code. As you delve deeper into C++, practicing these concepts will elevate your programming skills and prepare you for real-world challenges.
Expand your knowledge by exploring additional resources, and don't hesitate to practice coding to solidify your understanding further! Feel free to ask more questions or seek clarification on any topic you wish to learn about—curiosity is the first step toward mastery.