A project in C++ typically involves creating a structured application or program that leverages various C++ commands and libraries to solve specific problems or perform tasks.
Here's a simple code snippet that demonstrates a basic C++ project structure with a "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++ Projects
Setting Up Your Development Environment
To embark on your journey with a project in C++, it is crucial to establish a proper development environment. Start by choosing an Integrated Development Environment (IDE) that suits your needs. Some popular choices include Visual Studio for Windows users and Code::Blocks or CLion for cross-platform capabilities. Once you've settled on an IDE, you will need a C++ compiler. Options like GCC for Linux and MinGW for Windows will enable you to compile your projects smoothly.
Basic C++ Project Structure
Understanding the layout of a C++ project lays the groundwork for effective programming. A simple C++ project typically consists of:
- Source Files (.cpp): These files contain the implementations of your functions.
- Header Files (.h): These files declare your functions and classes, helping you organize your code.
Here’s how you can create your first simple C++ project:
- Create a New Project in Your IDE: Name it something relevant, like "HelloWorld".
- Add a Source File: Create a file named `main.cpp`.
- Write Your First C++ Program:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
Run your program to see "Hello, World!" printed on the console!
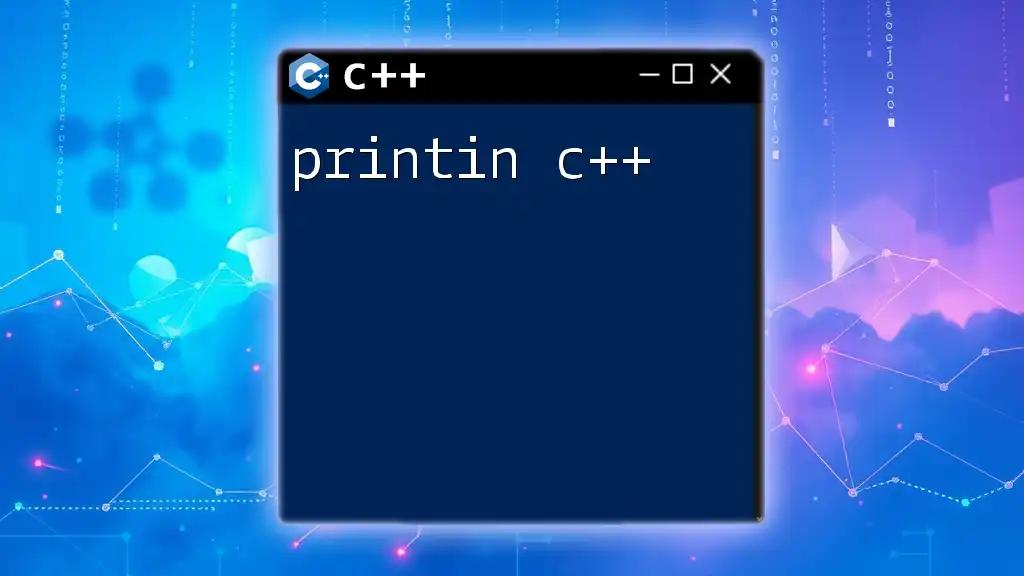
Types of Projects in C++
Beginner Level Projects
Starting with beginner-level projects allows you to build a solid foundation in C++. Here are a couple of ideas:
-
Simple Calculator: This project will help you grasp user input, operations, and control flow.
Sample code for a calculator might look like this:
#include <iostream> int main() { char operation; double num1, num2; std::cout << "Enter operation (+, -, *, /): "; std::cin >> operation; std::cout << "Enter two numbers: "; std::cin >> num1 >> num2; switch (operation) { case '+': std::cout << num1 + num2; break; case '-': std::cout << num1 - num2; break; case '*': std::cout << num1 * num2; break; case '/': std::cout << num1 / num2; break; default: std::cout << "Invalid operation."; break; } return 0; }
-
To-Do List Application: This helps you work with arrays and loops, allowing users to add and remove tasks.
Intermediate Level Projects
Once you feel comfortable with the basics, you can tackle intermediate level projects.
-
Banking System Simulation: Here, you’ll delve into object-oriented programming concepts. Define a `BankAccount` class:
class BankAccount { private: double balance; public: BankAccount(double initialBalance) : balance(initialBalance) {} void deposit(double amount) { balance += amount; } void withdraw(double amount) { if (amount <= balance) { balance -= amount; } else { std::cout << "Insufficient funds." << std::endl; } } double getBalance() const { return balance; } };
-
Basic Text-Based Game: This project can incorporate variables, control structures, and possibly file handling to save game state.
Advanced Level Projects
For those wanting a challenge, consider diving into advanced projects.
-
Chat Application: This will introduce you to networking, where you'll learn how to send and receive messages between users. You'll commonly use socket programming, which may require understanding POSIX libraries.
-
Game Development using SFML or SDL: In this project, you'll explore graphics programming. Begin with creating a game loop:
while (window.isOpen()) { sf::Event event; while (window.pollEvent(event)) { if (event.type == sf::Event::Closed) window.close(); } window.clear(); // Draw your game objects here window.display(); }

Enhancing Your C++ Project
Best Practices to Follow
To elevate your project in C++, adhere to best practices.
-
Code Readability and Documentation: Comment on your code generously, making it easier for others (and yourself) to understand the logic behind your programming decisions.
-
Optimizing Performance: Evaluate your algorithms and make efficiency a priority. Learn about time and space complexities to improve your code further.
Using Libraries and Frameworks
Integrating powerful libraries can enhance your C++ projects significantly. Familiarize yourself with libraries such as Boost for extended functionality and the Standard Template Library (STL) for efficient data structures and algorithms. Here’s how to use the STL vector:
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
nums.push_back(6);
for (int num : nums) {
std::cout << num << ' ';
}
return 0;
}
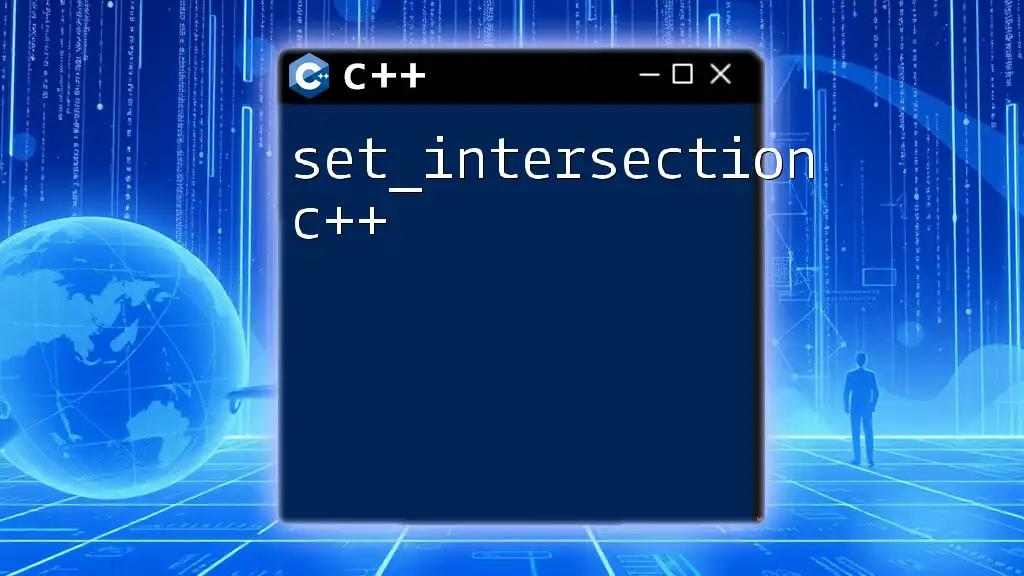
Debugging and Testing Your C++ Project
Common Debugging Techniques
As you work on your project in C++, debugging is a vital skill. Utilize the debugging tools provided by your IDE, which often allow you to set breakpoints and inspect variable values during execution. If you're using GCC, familiarize yourself with GDB (GNU Debugger) for command-line debugging.
Unit Testing in C++
Testing ensures your code behaves as intended. Implementing unit tests can be done using frameworks like Google Test. Here’s a simple test setup:
#include <gtest/gtest.h>
TEST(BankAccountTest, Deposit) {
BankAccount account(100);
account.deposit(50);
EXPECT_EQ(account.getBalance(), 150);
}
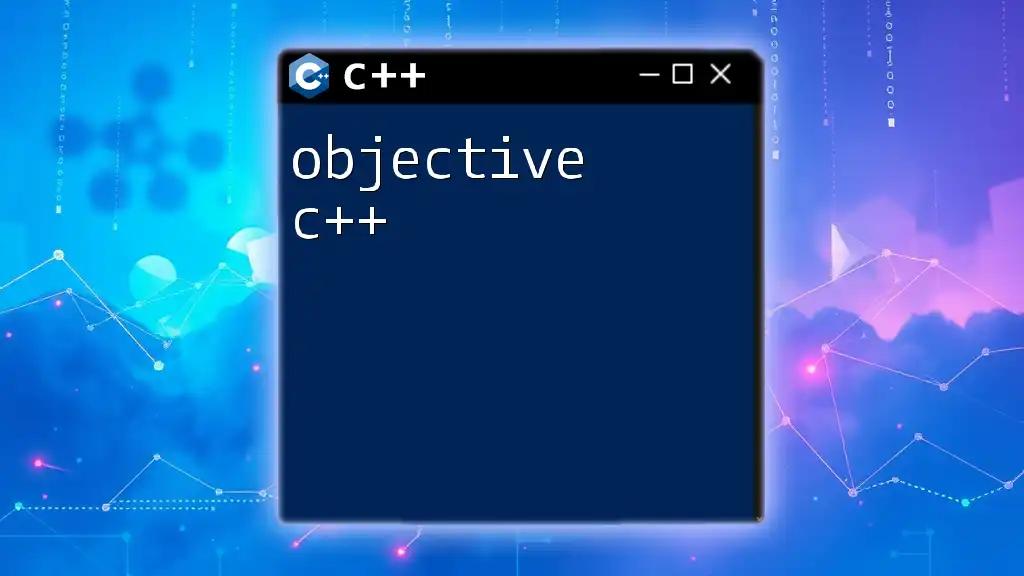
Deploying Your C++ Project
Packaging Your Application
When your project in C++ nears completion, consider how to package your application. Create an executable that users can run without installing additional software. Remember to check for compatibility across different operating systems.
Version Control with Git
Utilizing Git for version control is essential. It enables you to track changes, collaborate with others, and revert to previous states of your code. Familiarize yourself with basic commands like `git init`, `git add`, and `git commit` to manage your projects efficiently.
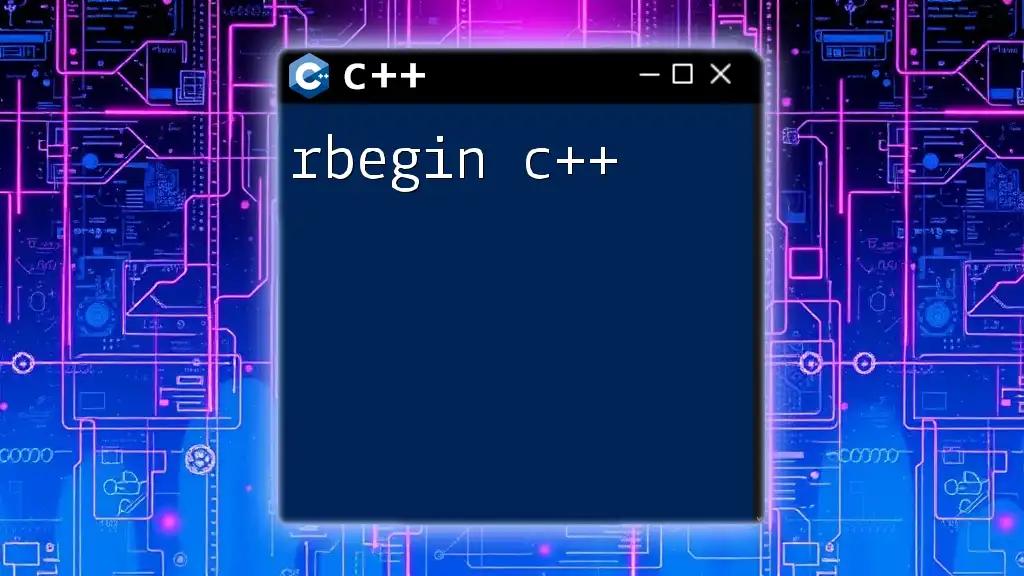
Learning Resources for C++ Projects
Books and Online Courses
To further hone your skills, explore resources like “The C++ Programming Language” by Bjarne Stroustrup and online courses from platforms such as Coursera or Udemy. These can guide you from the fundamentals to advanced concepts.
Active C++ Community and Support
Engaging with communities can provide you with invaluable support. Websites like Stack Overflow and specialized C++ forums will enable you to ask questions, share experiences, and find mentorship opportunities.

Conclusion
Implementing a project in C++ not only strengthens your programming skills but also enhances your problem-solving capabilities. Keep challenging yourself with different projects as you grow your expertise in this powerful language. Start your journey by choosing a project idea from above and bring it to life!