In C++, the `exec` family of functions, found in `<unistd.h>`, is used to replace the current process with a new process, effectively executing a specified command in a Unix-like environment.
Here's a simple example of using `execl` to run the `ls` command:
#include <unistd.h>
#include <iostream>
int main() {
// Replace the current process with the 'ls' command
execl("/bin/ls", "ls", "-l", (char *)NULL);
// If execl returns, it has failed
perror("execl failed");
return 1;
}
What is exec in C++?
The `exec` family of functions in C++ is part of the POSIX standard, providing a way to execute a program in the context of the calling process. When you use an `exec` function, it replaces the current process with a new process that runs a specified program. This is an essential feature for system programming, particularly in Unix-like operating systems, where you may need to run different commands and operate on the system level.
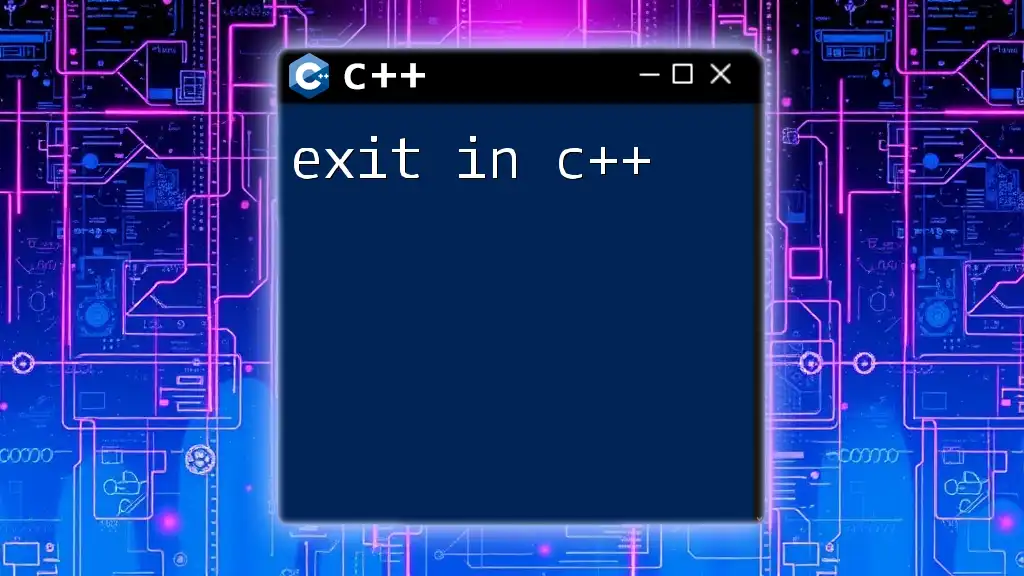
Why use exec in C++?
Using `exec` in C++ has several advantages:
- Process Management: It allows the control of new processes directly from your C++ applications, offering flexibility in process creation and execution.
- Automation: You can automate various tasks by running shell commands or other executable files, which helps in scripting and batch processing.
- Resource Management: It efficiently replaces the existing process, freeing up the resources previously occupied by the executing code.
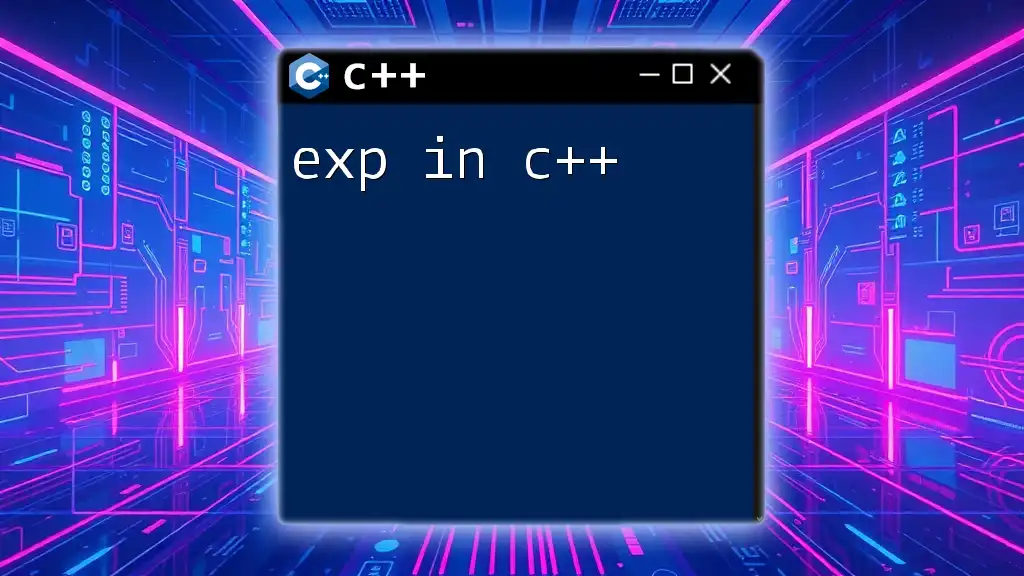
Understanding the exec Family of Functions
Overview of exec Functions
The `exec` family consists of several functions, each with minor differences suited to various use cases:
- execl: Executes a program specified by an absolute or relative pathname, taking a variable number of arguments.
- execv: Similar to `execl`, but accepts an array of pointers to null-terminated strings as arguments.
- execle: Like `execl`, but allows specifying a custom environment.
- execve: The most general form; it takes the program path, an array of arguments, and an array of environment variables.
- execlp and execvp: They look for the program in the directories listed in the `PATH` environment variable.
Common Use Cases for exec
The `exec` functions are often employed in situations such as:
- Running shell commands directly from a C++ application.
- Executing new scripts and programs without creating a separate shell.
- Implementing custom command line interfaces or command execution services.
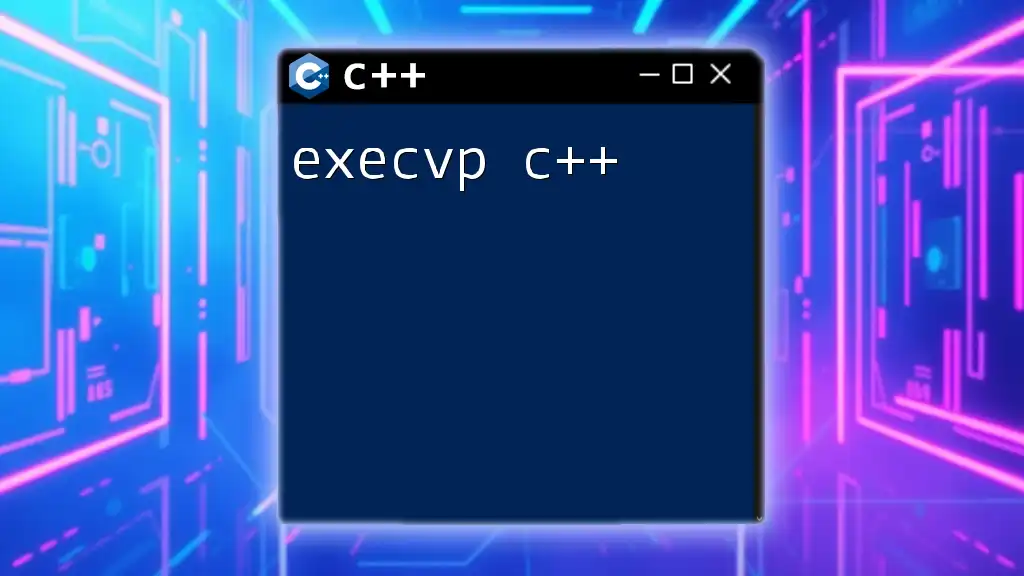
How to Use exec in C++
Setting Up Your C++ Environment
To use `exec`, you need to include the `<unistd.h>` header, which contains the necessary definitions. Ensure that you are compiling your program in an environment that supports POSIX, typically found in Unix-like systems.
Syntax and Parameters
The syntax for `exec` functions can appear complex at first. Here's a simplified structure:
exec_function(pathname, arg0, arg1, ..., argN, NULL);
- pathname: Path to the executable.
- arg0, arg1, ..., argN: Arguments passed to the program, with the first argument typically being the name of the program.
- NULL: Indicates the end of the argument list.
Examples of exec in Action
Basic Example: Using `execl`
#include <unistd.h>
#include <iostream>
int main() {
std::cout << "Executing ls command" << std::endl;
execl("/bin/ls", "ls", NULL);
std::cout << "This line will not execute if exec is successful." << std::endl;
return 0;
}
In this example, `execl` will execute the `ls` command, listing directory contents. Note that if the command executes successfully, the process is replaced and the last line will never run.
Using `execv` for Argument Lists
#include <unistd.h>
#include <iostream>
int main() {
char *args[] = {(char *)"ls", (char *)"-l", NULL};
execv("/bin/ls", args);
return 0;
}
Utilizing `execv` enables you to pass an array of string arguments, providing flexibility in argument management. The command executed here will also display detailed directory contents.
Environment Variables with `execle`
#include <unistd.h>
#include <iostream>
int main() {
char *env[] = {(char *)"PATH=/usr/bin", NULL};
execle("/bin/ls", "ls", "-l", NULL, env);
return 0;
}
`execle` allows you to specify environment variables directly for the new process. If you want to modify how the command runs depending on the environment, this function is ideal.
Exploring `execvp` and `execlp` for PATH resolution
When you want a function to find executables in your environment's `PATH`, use `execvp` or `execlp`. Here's how `execvp` can be used:
#include <unistd.h>
#include <iostream>
int main() {
char *args[] = {(char *)"ls", (char *)"-a", NULL};
execvp("ls", args);
return 0;
}
This code will search for `ls` in the directories specified in your `PATH` environment variable, making it more flexible and easier to use when you do not want to hardcode the path.
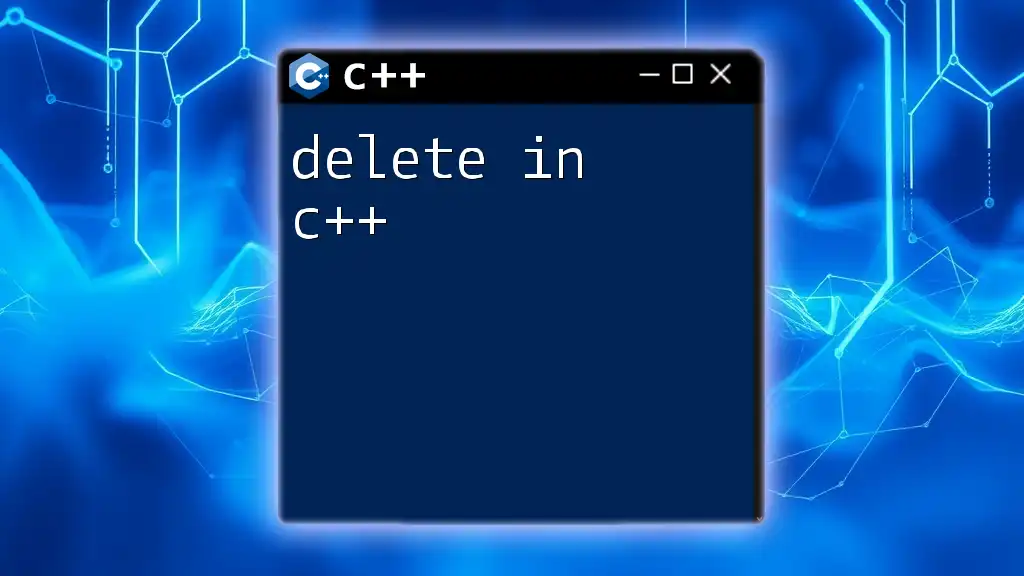
Common Mistakes to Avoid
Forgetting NULL Terminators
Each argument list must end with a NULL pointer. If omitted, the executed program may encounter undefined behavior, leading to errors or crashes.
Confusing exec with fork
While both `fork()` and `exec()` are used in process management, they serve distinct purposes. `fork()` creates a new process that is a duplicate of the calling process. Later, you can use `exec()` in the child process to replace it with another process. This can enable you to run commands concurrently or manage process hierarchies effectively.
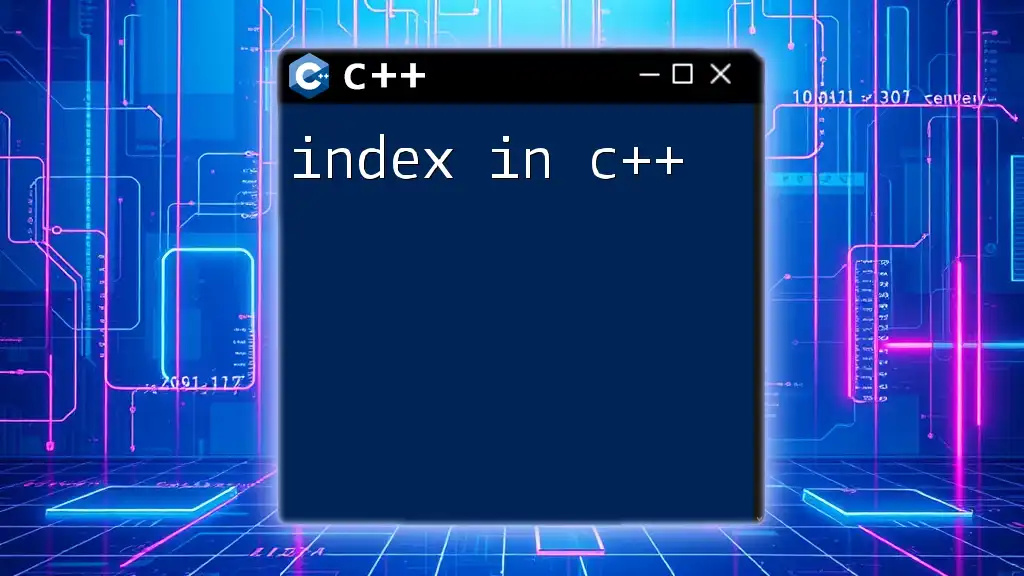
Error Handling and Debugging Tips
Checking for Errors in exec
It is critical to handle potential errors when using `exec`. All `exec` functions return only if there is an error. In such cases, you can check the output using `perror()` for user-friendly error reporting.
if (execvp("command", args) == -1) {
perror("execvp failed");
}
Debugging exec calls
Debugging is essential for ensuring that your `exec` calls function as intended. Using tools such as GDB can help trace the execution flow and identify where your process may be failing. Be sure to differentiate between execution failures and errors in your logic.
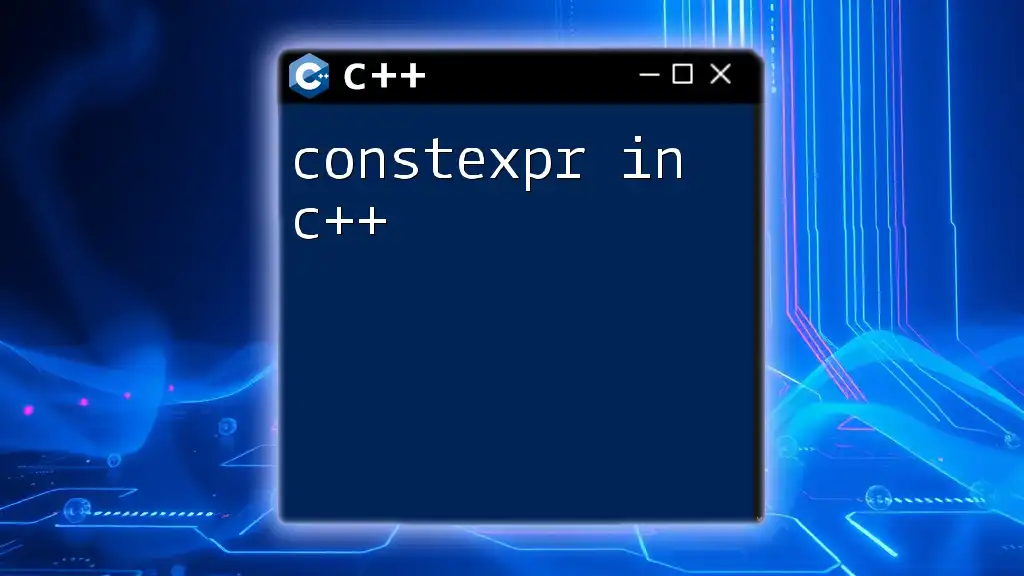
Conclusion
The `exec in C++` provides a powerful mechanism for executing external commands and processes directly from your applications. By understanding the different variations and functionalities of the `exec` family, you can manipulate processes effectively to achieve a wide range of tasks. Practice using these functions in different environments to gain confidence in your process management skills.
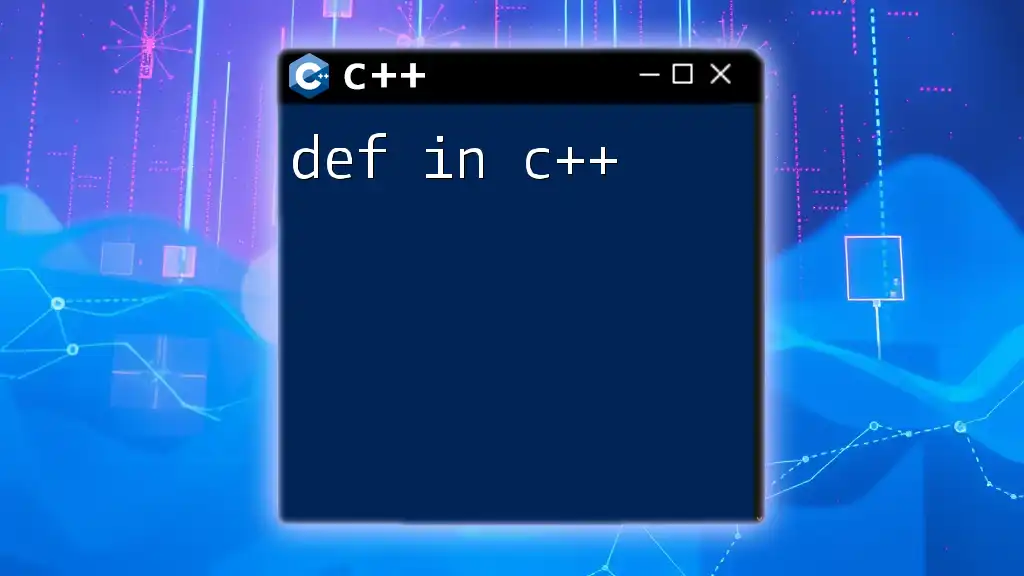
FAQs
What happens to the original process when exec is called?
When `exec` is invoked, the original process is replaced by the new program specified in the `exec` call. This means all the data associated with the original process is lost, and it cannot resume execution.
Can exec be used with C++ classes and objects?
No, `exec` does not directly support C++ classes or object methods, as it replaces the entire process, including memory and execution context. However, you can execute a separate program that interacts with your C++ application as needed.
Difference between exec and system() in C++.
The `system()` function starts a new shell to execute the command, whereas `exec` replaces the current process. This leads to fewer resources consumed when using `exec`, as it doesn’t create a new shell, which can be beneficial in optimizing your application.