`cerr` in C++ is used for outputting error messages to the standard error stream, allowing for immediate feedback on errors in a program.
Here's a simple code snippet demonstrating its use:
#include <iostream>
int main() {
std::cerr << "Error: Invalid input!" << std::endl;
return 1;
}
What is cerr in C++?
`cerr` is a predefined output stream in C++ that is specifically designed to handle error messages. It is part of the standard library, similar to `cout` and `cin`, which are used for output and input respectively. The key distinction of `cerr` lies in its function: while `cout` delivers standard output, `cerr` allows programmers to send error messages directly to the standard error output.
Comparison between `cout`, `cin`, and `cerr`
When we look at these three streams, each serves a unique purpose:
-
`cout`: Used for standard output. It is buffered, meaning that data may not be displayed immediately as it is sent; instead, it is temporarily stored until a certain condition is triggered (like a newline).
-
`cin`: Used for standard input. It handles user input but does not relate to outputting messages.
-
`cerr`: Used for error output. Unlike `cout`, `cerr` is unbuffered. This means that any message sent to `cerr` will be displayed immediately, ensuring that errors are reported promptly.
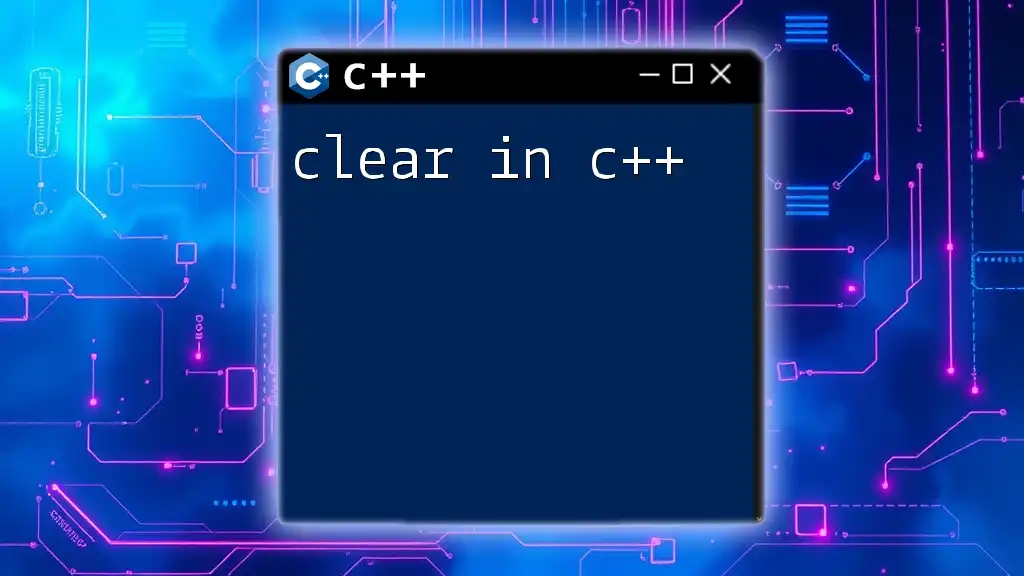
Why Use cerr for Error Handling?
Error handling is a vital component of programming, as it helps developers recognize and address problems that arise during execution. By using `cerr`, programmers can present error messages clearly and immediately.
Advantages of Using `cerr`
-
Unbuffered Output: Since `cerr` is an unbuffered stream, messages sent through it appear as soon as they are generated. This is especially important when diagnosing issues, as waiting for a buffer to flush could delay visibility of error messages.
-
Immediate Display of Error Messages: During program execution, encountering an error should trigger immediate feedback to the developer or user. This can be crucial for debugging.
Situations Where `cerr` is Preferable over `cout`
- When writing critical error messages that must be seen without delay.
- When differentiating error messages from regular outputs in applications that perform a lot of logging or user interactions. Using `cerr` ensures that error messages are not mixed with standard output.
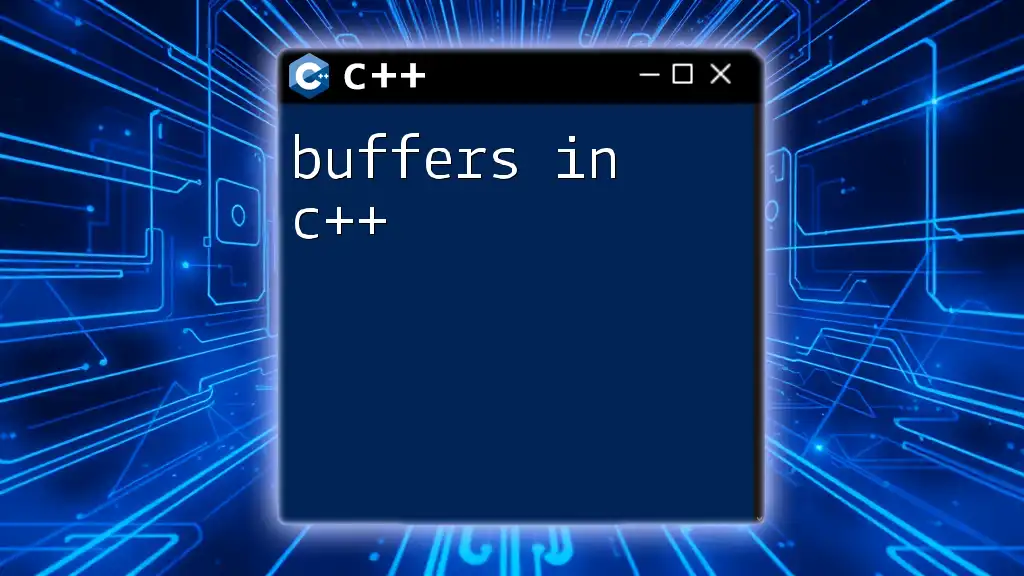
How to Use cerr in C++
Using `cerr` is straightforward and can be implemented in just a few lines of code.
Basic Syntax of `cerr`
Here’s how to use `cerr` in a simple example:
#include <iostream>
int main() {
std::cerr << "Error: Something went wrong!" << std::endl;
return 1;
}
In this example, if an error occurs, "Error: Something went wrong!" will be printed to the standard error stream immediately.
Outputting Error Messages
To effectively communicate error messages, consider the following tips:
-
Formatting Messages: Ensure that your error messages are clear and specific. Providing context can help identify the issue.
-
Using Manipulators: Just like with `cout`, you can use manipulators when working with `cerr`. For instance, `std::endl` can be used to terminate the line and flush the output stream without delay:
std::cerr << "Critical Error Occurred!" << std::endl;
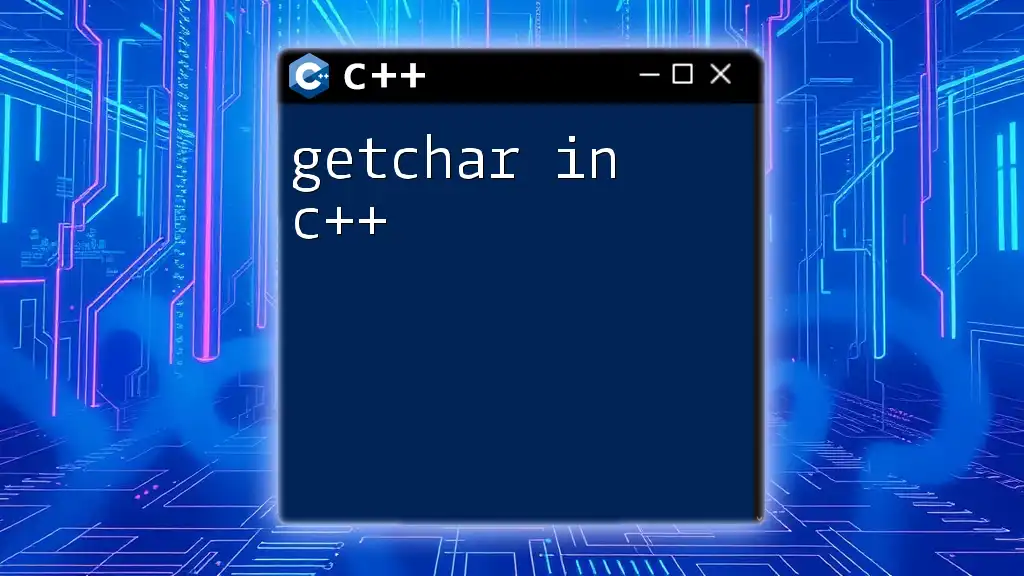
Practical Example of Using cerr
A practical application of `cerr` can be found in error handling with exceptions. Here’s a simple program that throws an exception and catches it with `cerr`:
#include <iostream>
#include <exception>
int main() {
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
In this example, when the runtime error is thrown, it is caught in the `catch` block, and the message is sent to `cerr`, ensuring you are immediately informed of the error.
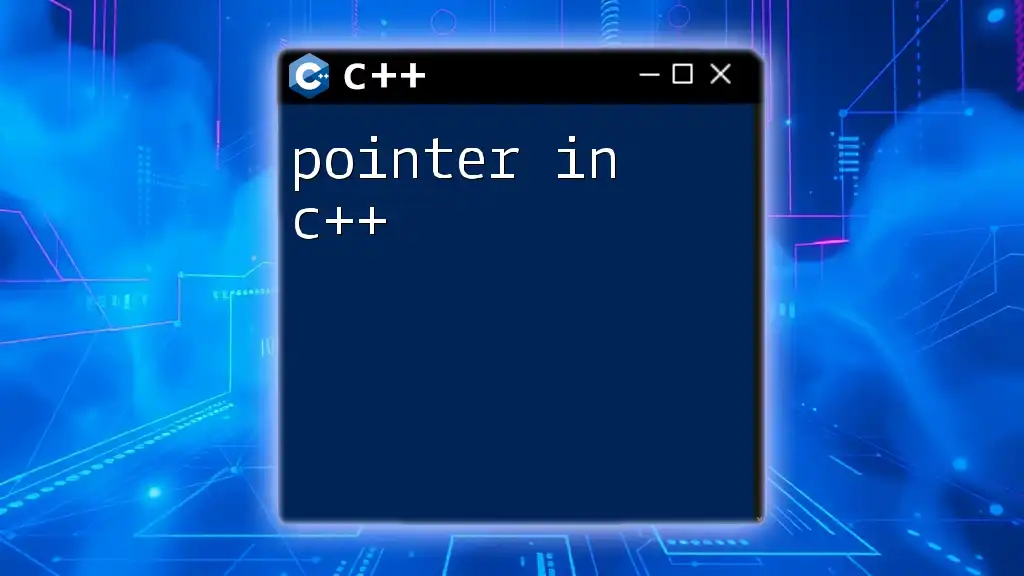
Common Mistakes When Using cerr
When working with `cerr`, developers can sometimes make several common mistakes:
-
Misunderstanding of Buffer Behavior: Many developers might expect `cerr` to operate like `cout`. However, they gain nothing from buffering in `cerr`, which can lead to confusion about output timing.
-
Failing to Distinguish Between Error Messages and Standard Output: When both `cout` and `cerr` are used, some output may be lost in translation if the messages are not clearly separated. Ensuring clear, distinct messages is vital.
-
Not Using Correct Error Handling Practices: Using `cerr` doesn't absolve a developer from needing to implement proper error handling strategies, such as structured exception management.
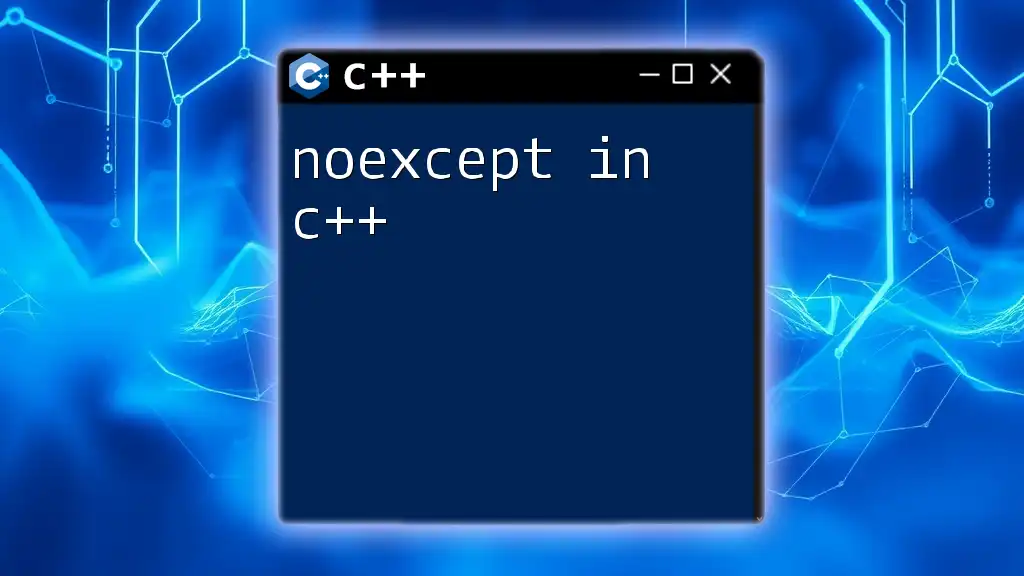
Advanced Usage of cerr
One interesting feature of `cerr` is its ability to be redirected. This allows developers to log errors into a file instead of displaying them on the console. Here’s how to accomplish that:
#include <iostream>
#include <fstream>
int main() {
std::ofstream errorLog("error.log");
std::streambuf* originalCerrBuffer = std::cerr.rdbuf(errorLog.rdbuf());
std::cerr << "This error will be logged into a file." << std::endl;
// Restore original cerr buffer
std::cerr.rdbuf(originalCerrBuffer);
return 0;
}
In this example, all messages sent to `cerr` will be written to "error.log", making it easier to maintain a history of errors for review later.
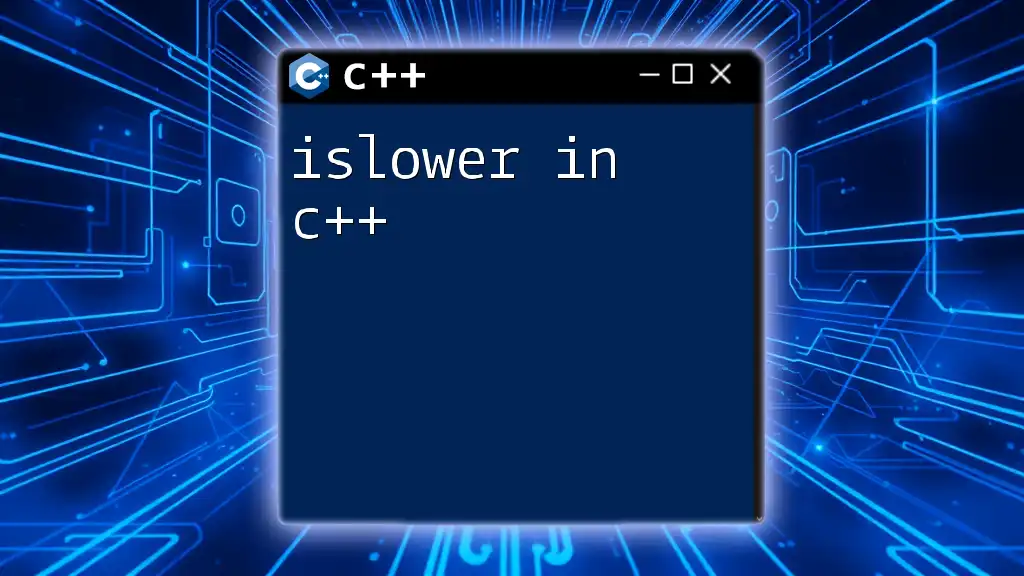
Conclusion
Using `cerr` in C++ for error handling is essential for effective debugging and user feedback. By leveraging its unbuffered output, programmers can ensure that critical error messages are displayed promptly and accurately. Understanding the proper use of `cerr` can greatly enhance your C++ programming skills, particularly in managing exceptions and providing informative feedback to users.
Take the next step in mastering C++ error handling by exploring the nuances of `cerr` and its applications in your projects!