Buffers in C++ are contiguous memory allocations used to store data temporarily while it is being moved from one place to another, commonly used for input and output operations.
Here's a simple example of using a buffer with standard input and output:
#include <iostream>
#include <cstring>
int main() {
const int BUFFER_SIZE = 256;
char buffer[BUFFER_SIZE];
std::cout << "Enter a string: ";
std::cin.getline(buffer, BUFFER_SIZE);
std::cout << "You entered: " << buffer << std::endl;
return 0;
}
Understanding Buffer Types
Static Buffers
Static buffers are defined with a fixed size known at compile time. When declaring a static buffer in C++, you create an array that allocates memory on the stack. This is beneficial in scenarios where you know the exact amount of data you will need to handle.
When to Use Static Buffers?
- Predictable Size: Use static buffers when the size of data is known beforehand.
- Performance: Static buffers may have better performance due to stack allocation, which is generally faster than heap allocation.
Example of Static Buffer
char staticBuffer[20]; // Static buffer of fixed size
In this example, `staticBuffer` can hold up to 19 characters plus a null terminator, making it suitable for short strings.
Dynamic Buffers
Dynamic buffers are created during runtime and can grow or shrink as needed. This flexibility allows C++ programmers to manage memory dynamically, offering significant advantages over static buffers.
Advantages of Dynamic Buffers
- Resizable: Unlike static buffers, dynamic buffers can change size based on runtime requirements.
- Memory Efficiency: You only allocate the amount of memory you need, reducing waste.
Example of Dynamic Buffer
char* dynamicBuffer = new char[20]; // Dynamic buffer allocation
In this case, `dynamicBuffer` is a pointer to a character array that can be resized if needed. Remember to `delete[] dynamicBuffer;` to free the memory when done.
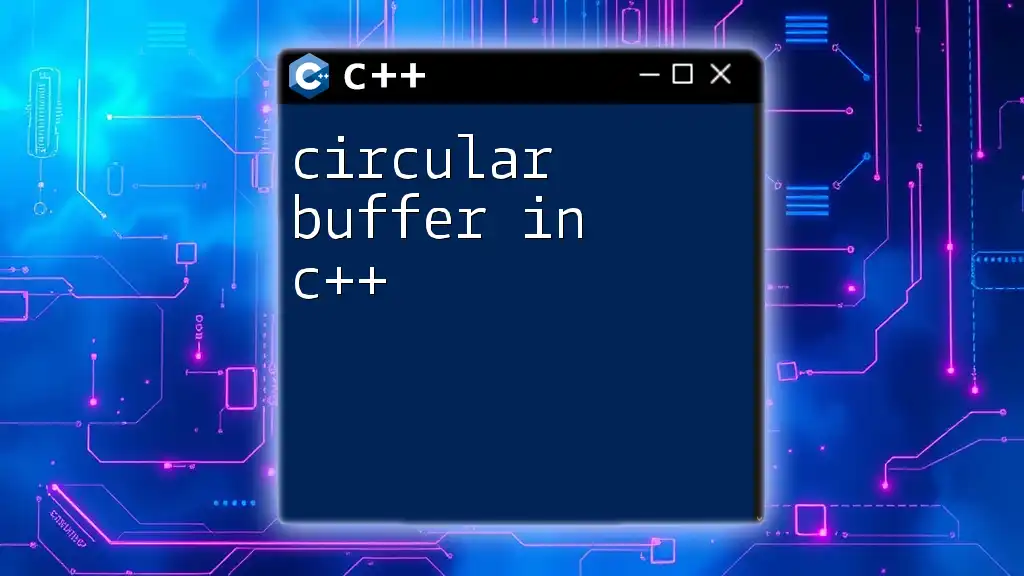
Creating and Managing Buffers in C++
Using Arrays as Buffers
Using traditional arrays as buffers is common in C++ programming. While simple and straightforward, they have limitations in size adaptability.
Example of Array Buffer
int arrBuffer[10]; // Simple array buffer
Here, `arrBuffer` quickly defines a buffer for ten integers, providing easy access to its elements.
Utilizing the Standard Template Library (STL)
std::vector
The `std::vector` class template allows for dynamic array creation. It is one of the most powerful features of the C++ Standard Library, providing automatic resizing and exceptional memory management.
Advantages of Using std::vector
- Automatic Memory Management: Vectors handle memory allocation and deallocation automatically.
- Easy to Resize: You can easily add or remove elements without complex management.
Example of Buffer Using std::vector
#include <vector>
std::vector<int> vecBuffer(10); // Vector buffer
In this example, `vecBuffer` initializes a dynamic buffer capable of holding 10 integers and can grow as needed using member functions like `push_back()`.
std::string as a Buffer
`std::string` is another powerful option for managing character buffers in C++. It simplifies string manipulation, including memory management.
Example of Using std::string
std::string strBuffer = "Hello, Buffer!"; // String buffer
In this instance, `strBuffer` serves as a flexible character buffer. Its size can adjust as you append or modify content.
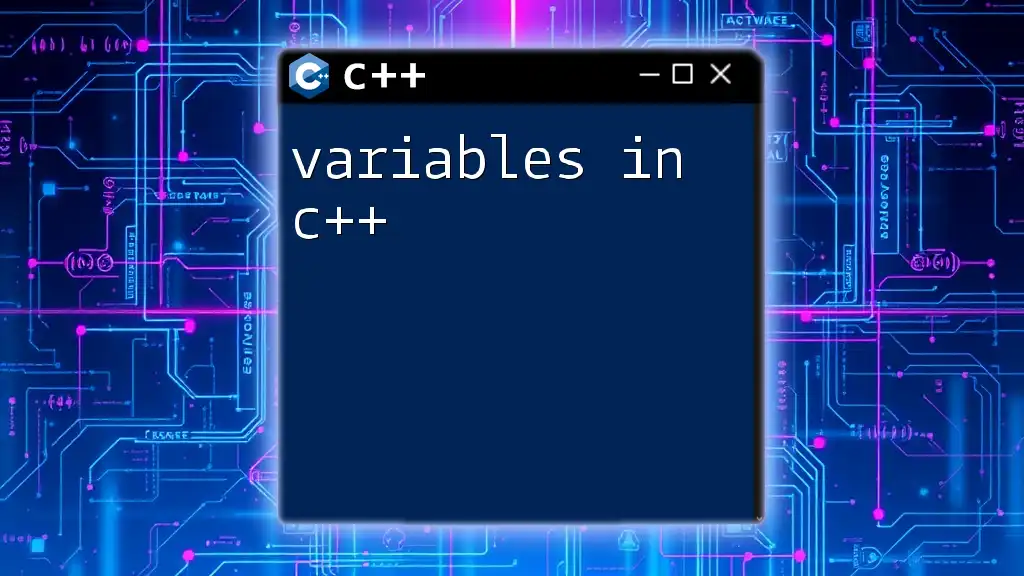
Buffer Operations
Reading from Buffers
Reading data from buffers in C++ is relatively straightforward. You can directly access elements of an array or vector or utilize member functions for STL containers.
Example of Reading from a Buffer
std::cout << dynamicBuffer << std::endl; // Print dynamic buffer contents
This snippet displays the contents of `dynamicBuffer` to the console.
Writing to Buffers
Writing to buffers varies based on the type of buffer you're using. With arrays, you may need to ensure there's enough space and consider null termination for strings.
Example of Writing to a Buffer
strcpy(staticBuffer, "Hello!"); // Writing to static buffer
In this example, we use `strcpy` to copy a string into `staticBuffer`. Always ensure that the destination buffer has enough capacity to hold the copied data.
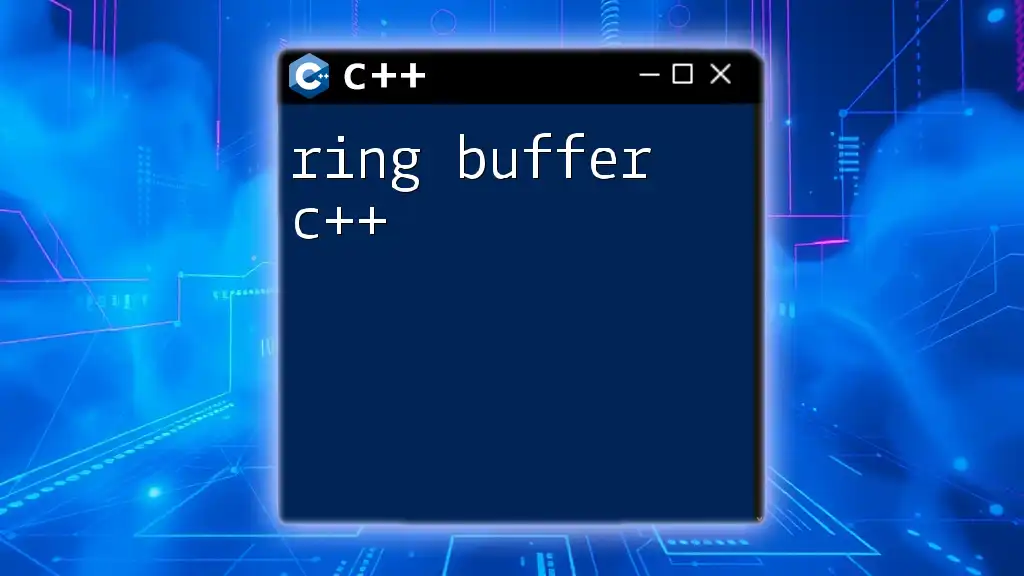
Buffer Overflow and How to Prevent It
What is Buffer Overflow?
Buffer overflow occurs when data written to a buffer exceeds its allocated size, potentially corrupting data, crashing programs, or opening security vulnerabilities. It is crucial to understand how to prevent overflow scenarios.
Consequences of Buffer Overflow
An overflow can lead to several issues, including:
- Security Breaches: Attackers may exploit vulnerabilities to execute arbitrary code.
- Program Crashes: Overwriting critical data can make programs unstable or crash unexpectedly.
Prevention Techniques
When coding, employing safe practices is vital to avoid buffer overflows. Techniques include:
- Use Safe Functions: Functions like `strncpy` and `snprintf` prevent overflows by specifying the maximum number of characters to copy.
- Validate Input Sizes: Always check the incoming data size against buffer capacity before writing.
Example of Safe Buffer Use
strncpy(staticBuffer, "Safe Copy", sizeof(staticBuffer)); // Safe copy example
By using `strncpy`, you ensure that data fits within `staticBuffer`, preventing overflow.
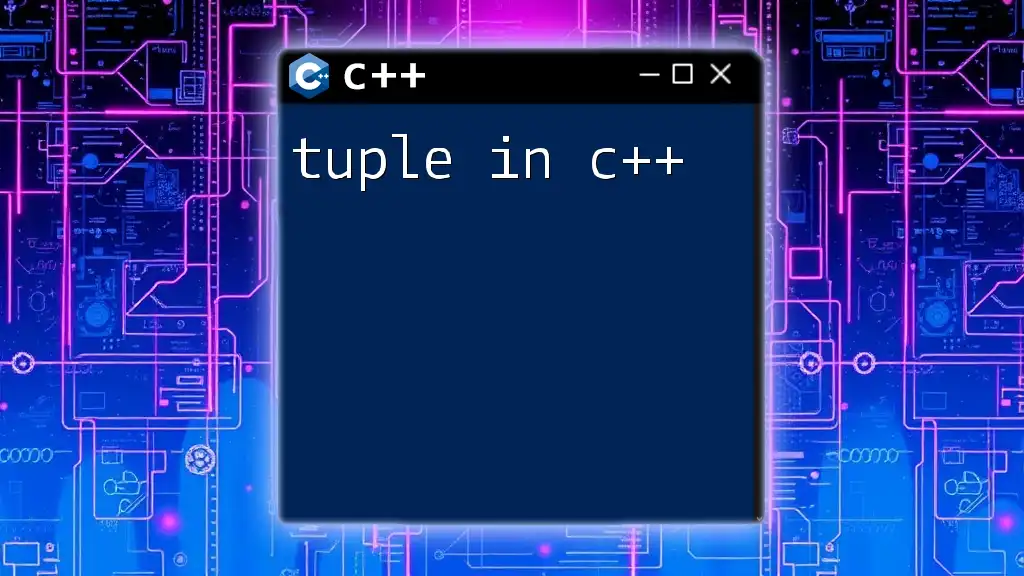
Buffer Performance
Measuring Buffer Performance
The performance of buffers can influence the efficiency of your C++ programs. Key factors to consider include:
- Latency: The time it takes to read from or write to a buffer.
- Throughput: The volume of data processed within a specific timeframe.
- Memory Access Patterns: Regular patterns contribute to better cache utilization.
Tips for Optimizing Buffer Performance
- Choose the Right Buffer Type: Depending on your use case, evaluate whether static or dynamic buffers best suit your needs.
- Cache Management: Optimize buffer access patterns to improve cache performance.
- Memory Pooling: For frequent allocations, consider memory pooling to reduce allocation overhead.
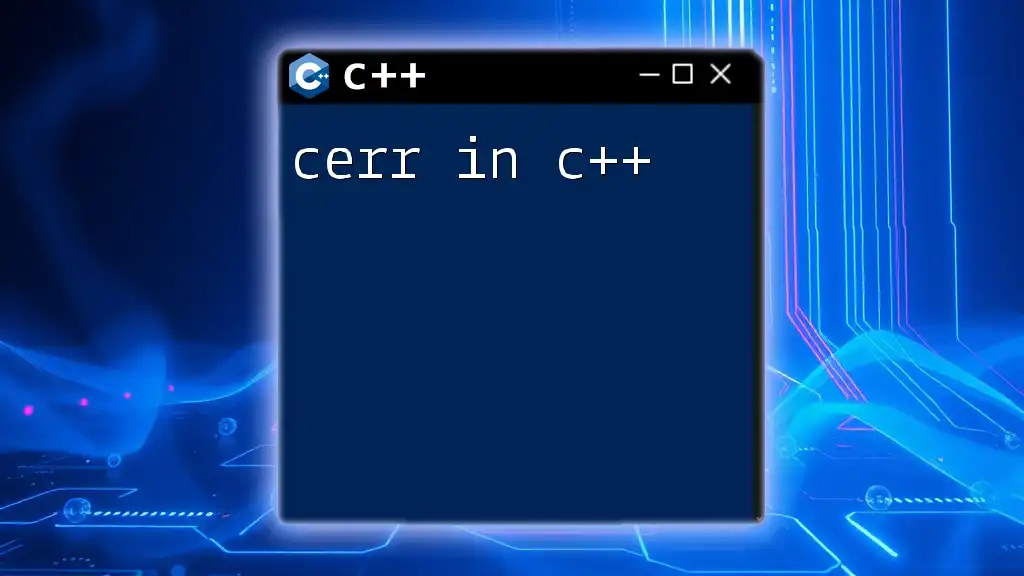
Conclusion
Buffers in C++ play a crucial role in memory management and data handling. Understanding the different types of buffers, the operations you can perform, and the importance of safety and performance will empower you as a developer. Practice implementing these techniques in your projects; mastering buffers can significantly enhance both your coding efficiency and the reliability of your applications.
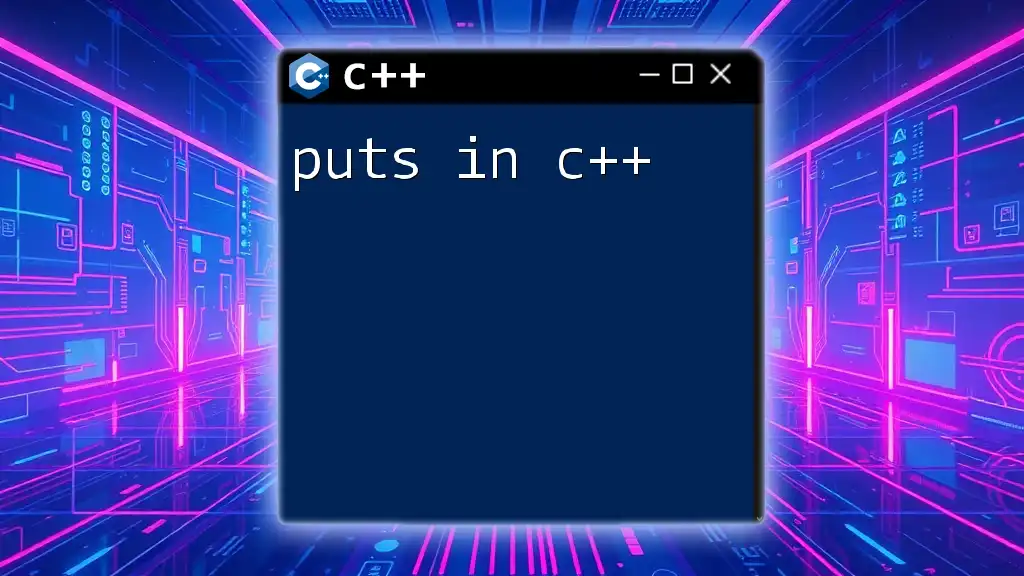
Additional Resources
For further exploration of buffers in C++, refer to the official C++ documentation and additional tutorials available online. Resources that delve deeper into buffer management practices will solidify your understanding and skill in this fundamental area of programming.