In C++, the `copy` function from the `<algorithm>` header allows you to copy elements from one range to another.
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5);
std::copy(source.begin(), source.end(), destination.begin());
for (int value : destination) {
std::cout << value << " ";
}
return 0;
}
Understanding Copying in C++
What is Copying in C++?
Copying in C++ refers to the process of creating a duplicate of an object, encompassing both its data and operations. This fundamental concept is crucial in memory management and helps to ensure data integrity when dealing with complex data types. In C++, copying can occur in various contexts, such as passing objects to functions, returning objects from functions, or storing them in containers.
Types of Copies
Shallow Copy vs Deep Copy
-
Shallow Copy: This type of copy duplicates the immediate values of the object, but not the objects that the original object points to. Any objects that are shared between the original and the copied object can lead to unexpected behavior if one object is modified or deleted.
-
Deep Copy: In contrast, a deep copy duplicates all values, including any dynamically allocated memory pointed to by the original object. This ensures that the copied object is completely independent of the original object.
Example of Shallow Copy:
class Shallow {
public:
int* data;
Shallow(int val) {
data = new int(val);
}
// Shallow Copy Constructor
Shallow(const Shallow& source) : data(source.data) {}
~Shallow() {
delete data; // Potential issue: double deletion when both objects are deleted
}
};
Example of Deep Copy:
class Deep {
public:
int* data;
Deep(int val) {
data = new int(val);
}
// Deep Copy Constructor
Deep(const Deep& source) {
data = new int(*source.data);
}
~Deep() {
delete data;
}
};
Copy Constructors
A copy constructor is a special constructor in C++ used to create a new object as a copy of an existing object. It takes a reference to an object of the same class as its parameter.
Example:
class Example {
public:
int value;
Example(int v) : value(v) {}
// Copy Constructor
Example(const Example& obj) : value(obj.value) {}
};
Using a copy constructor helps to control how objects are copied and allows for customization of the copying process.
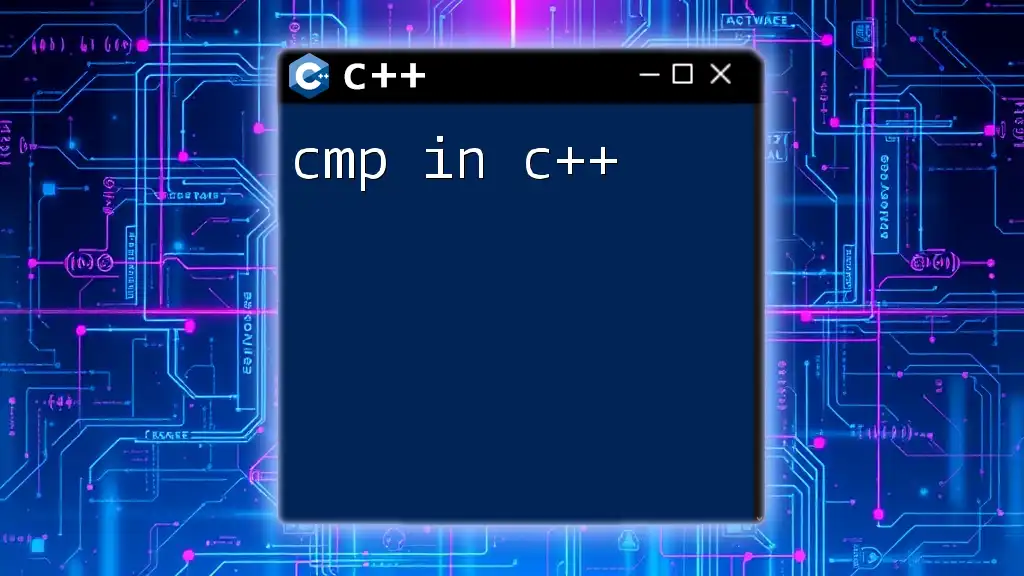
Copying Objects in C++
Default Copy Constructor
When you create an object, C++ automatically generates a default copy constructor if you do not provide one. This default copy constructor performs a shallow copy of all members.
Example:
class DefaultCopy {
public:
int value;
DefaultCopy(int v) : value(v) {}
};
DefaultCopy a(5);
DefaultCopy b = a; // Uses default copy constructor, performs a shallow copy
User-Defined Copy Constructor
Sometimes, a default copy constructor is insufficient, especially for classes that allocate memory. A user-defined copy constructor is necessary to manage complex copying behavior.
Example:
class UserDefinedCopy {
public:
int* data;
UserDefinedCopy(int val) {
data = new int(val);
}
// User-defined copy constructor
UserDefinedCopy(const UserDefinedCopy& source) {
data = new int(*source.data); // Creating a deep copy
}
~UserDefinedCopy() {
delete data;
}
};
Copy Assignment Operator
When an existing object is assigned a new value from another existing object, the copy assignment operator is called. This operator can also manage copying and resource allocation properly.
Example:
class AssignmentOp {
public:
int* data;
AssignmentOp(int val) {
data = new int(val);
}
// Copy Assignment Operator
AssignmentOp& operator=(const AssignmentOp& source) {
if (this == &source) return *this; // Self-assignment check
delete data; // Free existing resource
data = new int(*source.data); // Perform deep copy
return *this;
}
~AssignmentOp() {
delete data;
}
};
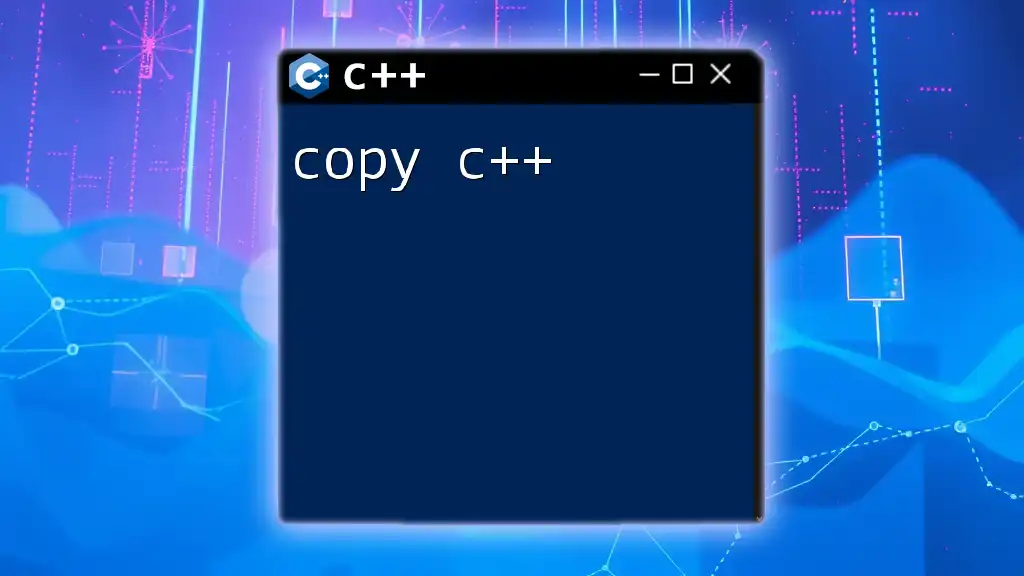
Copying with C++ Standard Library
std::copy and std::copy_if
The C++ Standard Library offers `std::copy` and `std::copy_if`, which provide powerful and efficient methods for copying data from one range to another.
Example usage of `std::copy`:
#include <algorithm>
#include <vector>
std::vector<int> source = {1, 2, 3, 4};
std::vector<int> destination(source.size());
std::copy(source.begin(), source.end(), destination.begin());
Copying with Containers
Copying Vectors
When dealing with vectors, copying can be done using the assignment operator or the `std::copy`.
Example using assignment operator:
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = vec1; // Uses the default copy constructor
Example using std::copy:
std::vector<int> vec3(3);
std::copy(vec1.begin(), vec1.end(), vec3.begin());
Copying Lists and Maps
Using `std::copy` with lists and maps follows similar principles, with the syntax tailored to the container type.
Example with std::list:
#include <list>
std::list<int> list1 = {1, 2, 3};
std::list<int> list2(list1); // Direct initialization using the copy constructor
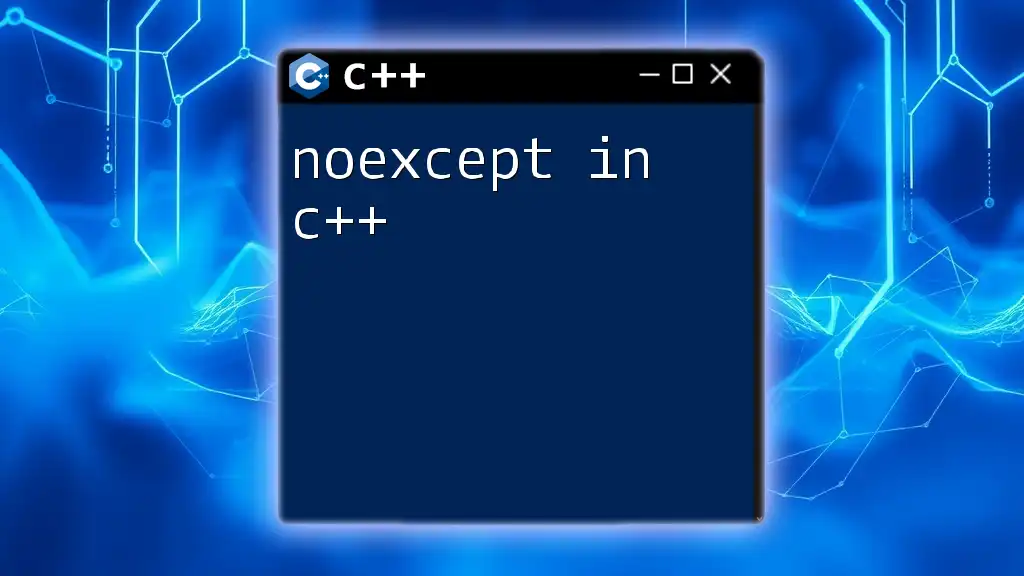
Best Practices for Copying in C++
Understanding Object Lifetimes
When implementing copy operations, understanding object lifetimes is critical. It is essential to ensure that objects remain valid throughout their usage, especially when copying dynamically allocated memory.
Ensuring Safety in Copying
Rule of Three
The Rule of Three states that if a class requires a user-defined copy constructor, copy assignment operator, or destructor, it likely requires all three. This rule ensures that classes behave predictably during copy operations and resource management.
Using Smart Pointers
Smart pointers, such as `std::shared_ptr` and `std::unique_ptr`, can simplify memory management while providing safe copying semantics. Smart pointers manage ownership automatically, preventing memory leaks.
Example with std::shared_ptr:
#include <memory>
class SmartPointerExample {
public:
std::shared_ptr<int> data;
SmartPointerExample(int val) : data(new int(val)) {}
};
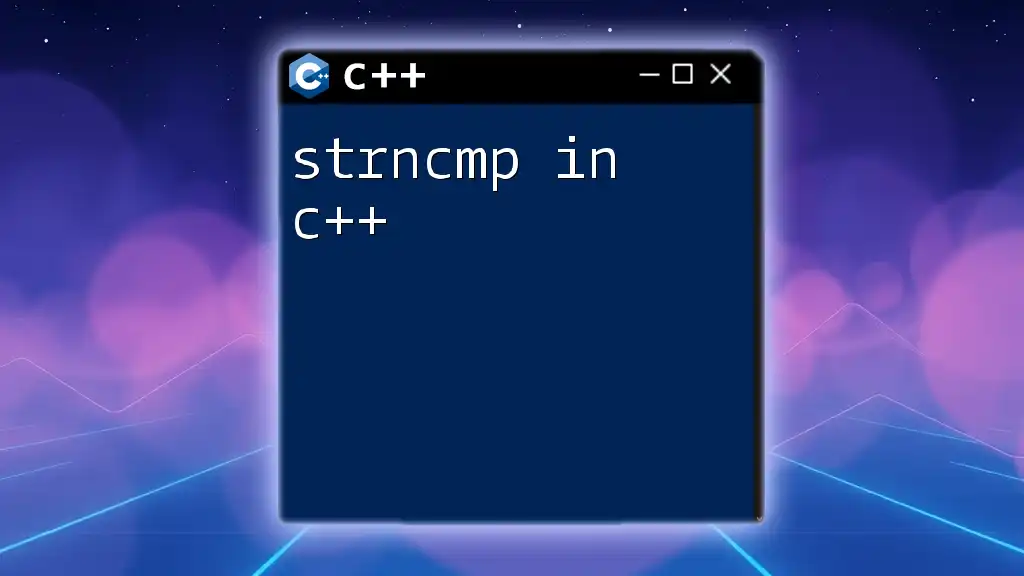
Common Pitfalls to Avoid
Memory Leaks
Memory leaks can occur when dynamically allocated memory is not properly managed during copying. Always ensure that you delete any dynamically allocated resources in destructors, copy constructors, and assignment operators.
Example of a potential memory leak:
class LeakExample {
public:
int* ptr;
LeakExample(int val) : ptr(new int(val)) {}
// This copy constructor will lead to a leak
LeakExample(const LeakExample& obj) {
ptr = obj.ptr; // Shallow copy leads to double free
}
~LeakExample() {
delete ptr; // Dangerous if both objects destroy ptr
}
};
Unexpected Behavior with Copying
Copying complex objects might lead to unintended side effects, especially if shallow copies are involved. Always verify the behavior of your classes, particularly when copying objects that contain pointers to dynamically allocated memory.
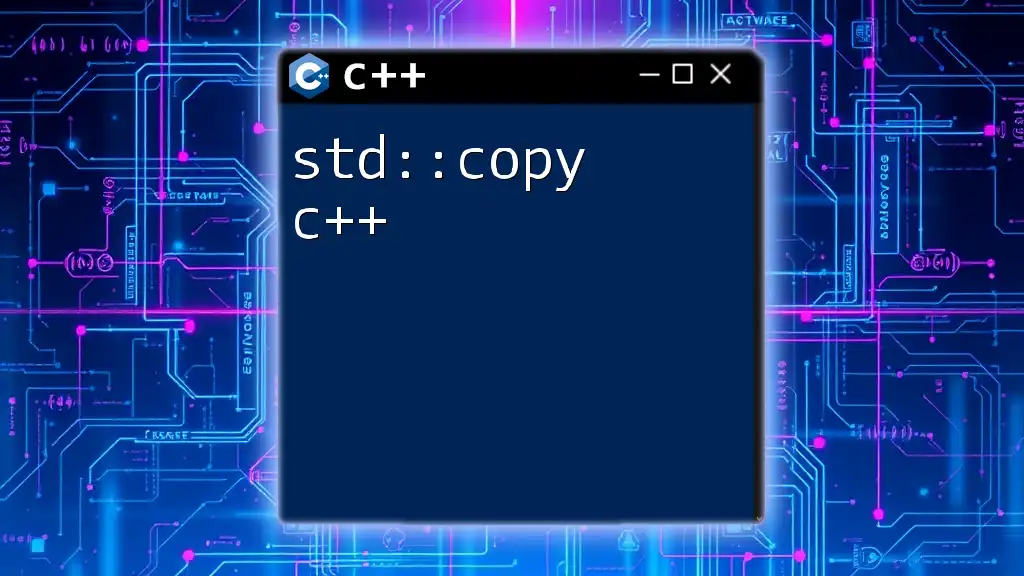
Conclusion
Summary of Key Points
Copying in C++ is an essential concept fundamental to object-oriented programming. Understanding how to use copy constructors and assignment operators correctly helps in managing resources effectively and avoiding common pitfalls, such as memory leaks and unintentional sharing of state.
Further Learning Resources
Explore more through advanced C++ texts, online courses, and documentation to deepen your understanding of copying and memory management. By practicing and experimenting, you can master the intricacies of copying in C++.
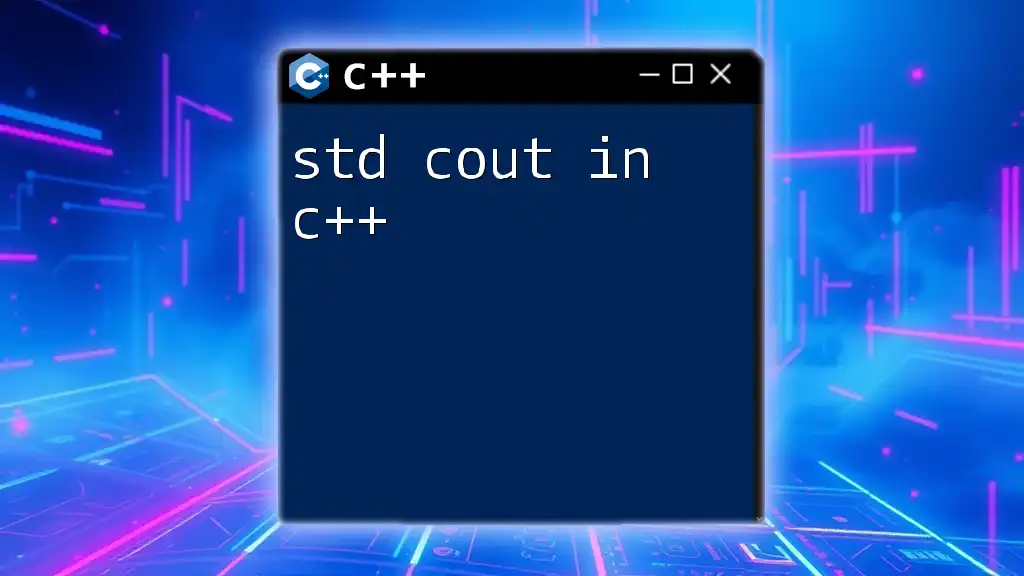
Code Repository
For complete code examples, visit the online code repository I will provide, where you can find implementations that highlight these concepts in action.