In C++, a header file with the `.hpp` extension is used to declare the interfaces for code components, enabling modular programming and easy inclusion in different source files.
// example.hpp
#ifndef EXAMPLE_HPP
#define EXAMPLE_HPP
void hello(); // Function declaration
#endif // EXAMPLE_HPP
What is an HPP File?
An HPP file, often referred to as a header file in C++, serves as an essential building block in modern C++ programming. It typically contains declarations for functions, classes, and variables. The primary purpose of HPP files is to provide a means to declare the interface to your code, which can then be included in various source files (CPP files) to implement the functionality.
Difference between HPP and CPP Files
While HPP files handle declarations, CPP files—also known as source files—contain the actual implementations of these declarations. This separation promotes modular programming, allowing developers to manage large projects efficiently.
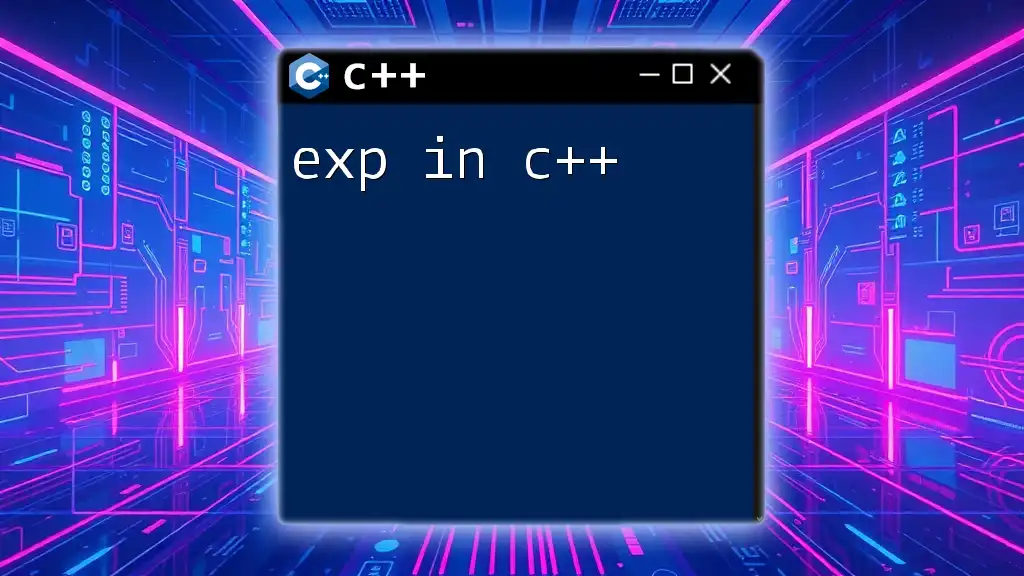
Why Use HPP Files?
Using HPP files brings several advantages:
- Modularity: By separating declarations from implementations, you can organize your code better, making it easier to maintain and update.
- Reusability: Once you've defined a class or function in an HPP file, you can reuse it across multiple CPP files without rewriting the code.
- Readability: Code becomes significantly more readable when functions and classes are clearly declared in separate header files.
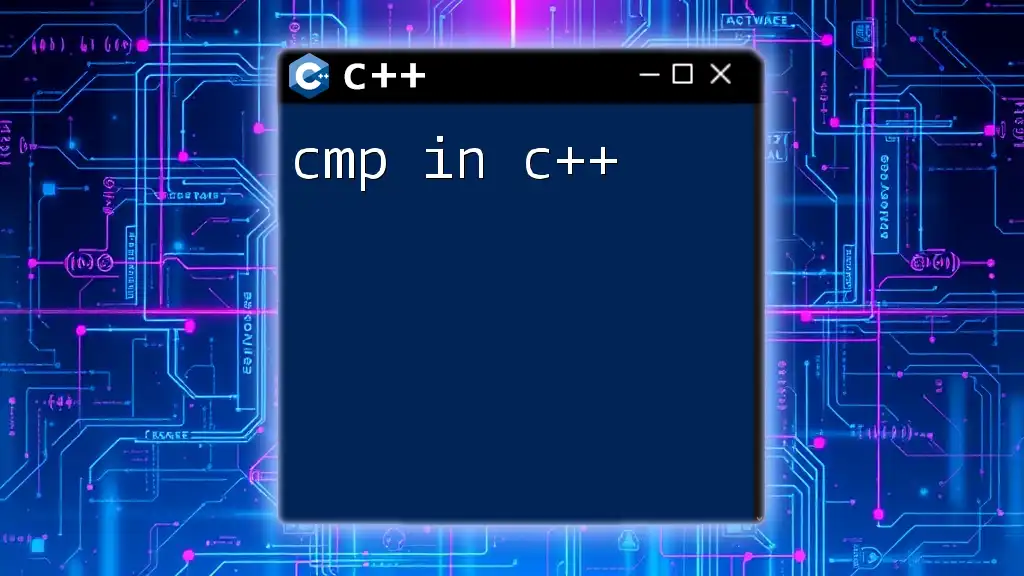
Understanding Header Files in C++
The Role of Header Files
Header files play a crucial role in organizing your C++ projects. They enable you to declare interfaces, which encompass the functions, classes, and constants used in your program. This organization is especially important when collaborating with other developers or when your project scales in complexity.
How HPP Files Work
HPP files interact with CPP files through the preprocessor. When you include an HPP file in a CPP file using the `#include` directive, the entire content of the header file is inserted into the source file at the point of inclusion. This process effectively means that declarations made in the HPP file are visible to the CPP file, enabling the compiler to check function calls and understand class structures at compile time.
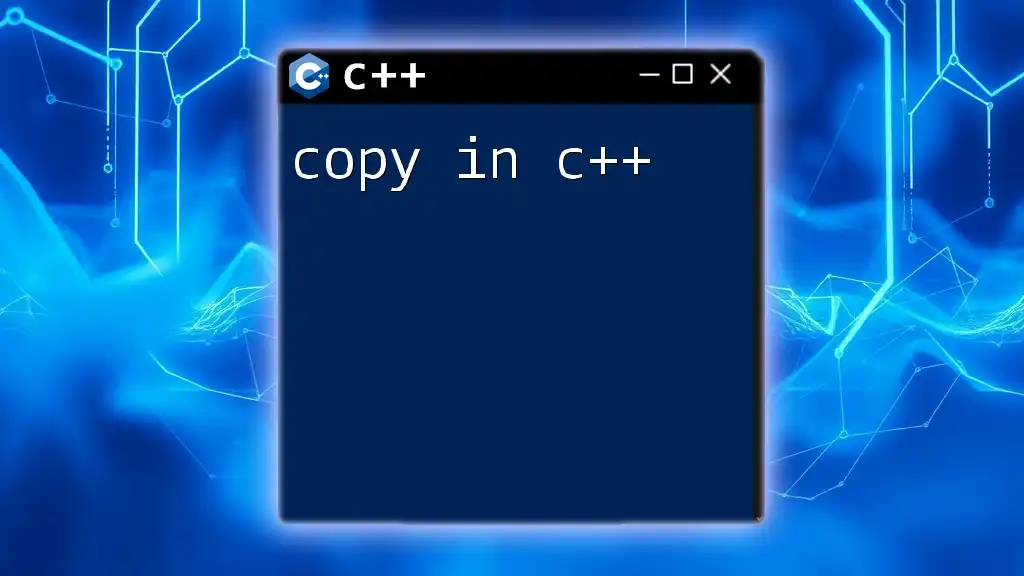
Structure of an HPP File
Basic Components of an HPP File
When creating an HPP file, it typically includes the following components:
- Include Guards: These are preprocessor directives that prevent the same header file from being included multiple times, which could lead to errors. They usually appear at the top and bottom of the HPP file.
- Namespace Declaration: To avoid naming conflicts, it's common to encapsulate your classes and functions within namespaces.
- Function and Class Declarations: This section consists of the actual declarations that other files will reference.
Example of a Basic HPP File
Here’s a simple example of a header file:
#ifndef MYCLASS_HPP
#define MYCLASS_HPP
namespace MyNamespace {
class MyClass {
public:
void myFunction();
};
}
#endif // MYCLASS_HPP
In this example, the `#ifndef`, `#define`, and `#endif` directives work together to create an include guard. The class `MyClass` contains a public method declaration `myFunction()`, which can then be defined in a corresponding CPP file.
Best Practices for Structuring HPP Files
- Naming Conventions: Use clear and descriptive names for your HPP files. A common practice is to match the HPP filename to the class or functionality it declares.
- Organizing Classes and Functions: Group related classes and functions together to enhance navigability and clarity. This practice minimizes confusion and helps other developers quickly understand your code.
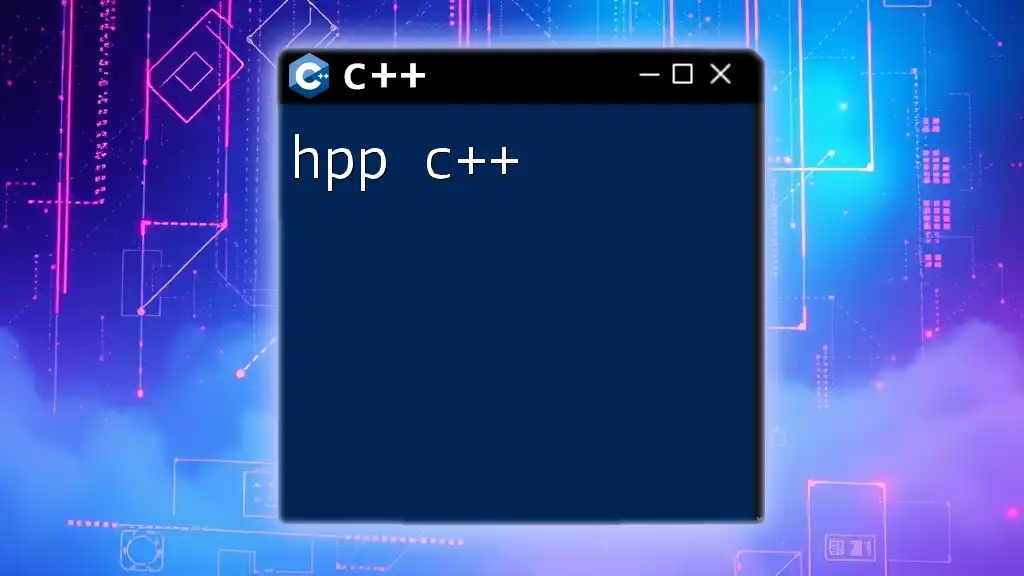
Including HPP Files in CPP Files
Syntax for Including HPP Files
To include an HPP file in a CPP file, you use the `#include` directive. Here's how to do it:
#include "MyClass.hpp"
When using quotes, the compiler looks for the HPP file in the current directory first, while angle brackets (`<>`) indicate standard library paths.
Understanding the Search Path for HPP Files
The compiler has a predefined set of directories that it searches for HPP files. Understanding how to tell the compiler where to find these files is crucial. You can add directories to the include path by specifying options in your build system or IDE.
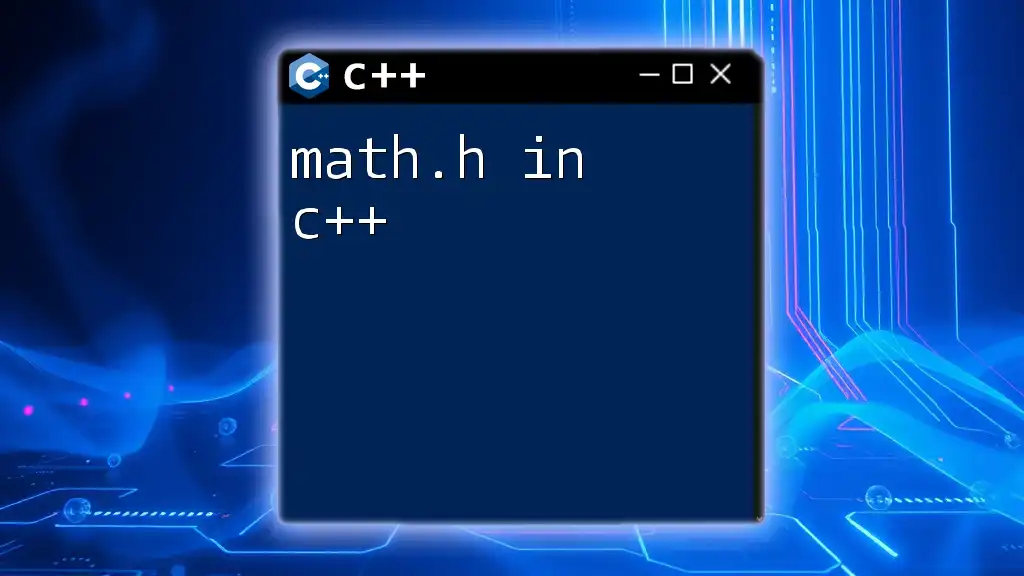
Advanced HPP Features
Templates and HPP Files
Templates provide a powerful way to create generic functions and classes. Using templates within HPP files can enhance code reusability and flexibility.
Practical Example of Templating in HPP Files
#ifndef MYTEMPLATE_HPP
#define MYTEMPLATE_HPP
template <typename T>
class MyTemplate {
public:
T add(T a, T b) { return a + b; }
};
#endif // MYTEMPLATE_HPP
In this example, `MyTemplate` is a class template that can operate with any data type `T`, allowing for versatile and generic implementations.
Inline Functions in HPP Files
Inline functions can be defined directly in HPP files to suggest to the compiler to attempt to expand the function inline rather than invoking it through the usual call mechanism. This can enhance performance, especially for small, frequently called functions.
Example of an Inline Function
inline int square(int x) {
return x * x;
}
By placing `square` in the HPP file, you provide quick access to a commonly used function without the overhead of a function call.
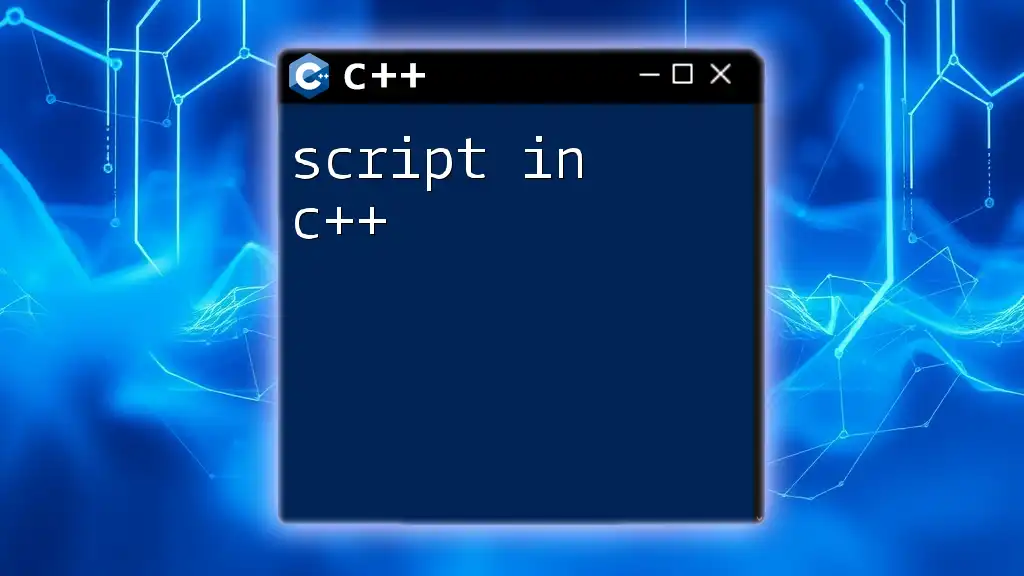
Common Pitfalls When Using HPP Files
Circular Dependencies
One of the most common issues associated with HPP files is circular dependencies. These occur when two or more header files include each other, leading to compilation errors. To avoid this, carefully architect your header inclusion dependencies and utilize forward declarations when possible.
Over-using HPP Files
While HPP files are advantageous for code organization, over-using them can lead to bloated header files. Limit the content of HPP files to declarations only; keep implementations in CPP files whenever feasible. This practice prevents unnecessary recompilation and maintains efficiency.
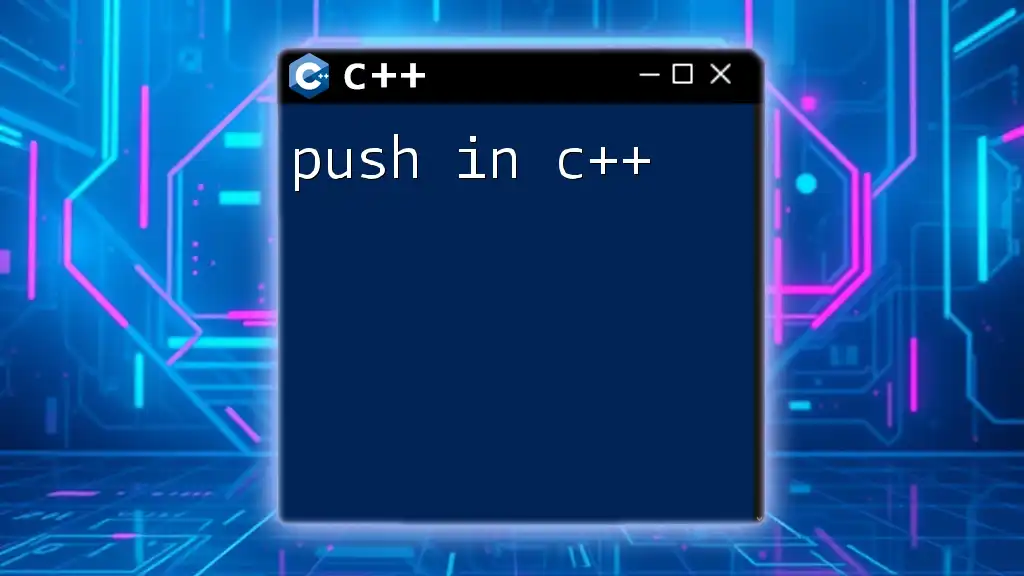
Conclusion
In summary, HPP files are a powerful tool in C++ programming that offer modularity, reusability, and improved readability. By mastering the use of HPP files, you'll be well-equipped to handle a variety of coding challenges effectively.
Encouragement to Practice
As you continue your C++ journey, don’t hesitate to create your own HPP files and experiment with the concepts discussed. The more you practice, the better you will become at organizing and structuring your codebase.
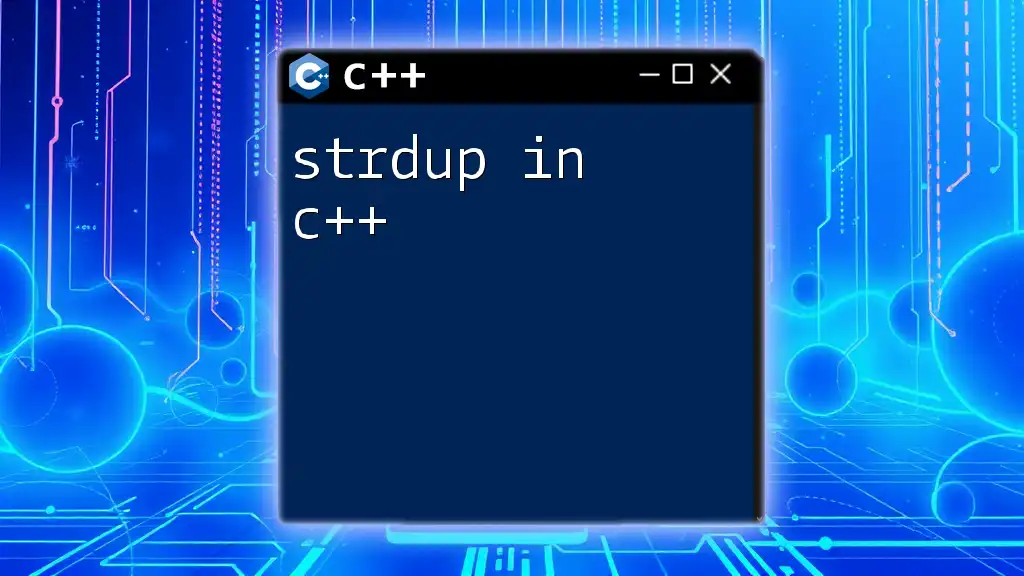
Additional Resources
Recommended Reading
For further understanding of HPP files and their applications, consider exploring authoritative C++ programming books and documentation.
Online Tutorials and Courses
Look for online platforms offering structured courses on C++ that delve deeper into the practical aspects of header files and best coding practices.