The `cmp` command in C++ is used to compare two values or objects for equality, typically implemented using the `==` operator.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
int main() {
int a = 5;
int b = 5;
if (a == b) {
std::cout << "The values are equal." << std::endl;
} else {
std::cout << "The values are not equal." << std::endl;
}
return 0;
}
Understanding Comparison Operations in C++
In C++, comparison operations are essential for determining the relationship between two values. These operations allow developers to implement logic and control the flow of a program. Comparison operators in C++ include:
- Equality operator: `==`
- Inequality operator: `!=`
- Relational operators: `<`, `>`, `<=`, `>=`
These operators form the foundation of conditional statements and loops, enabling various functionalities such as sorting and filtering data.
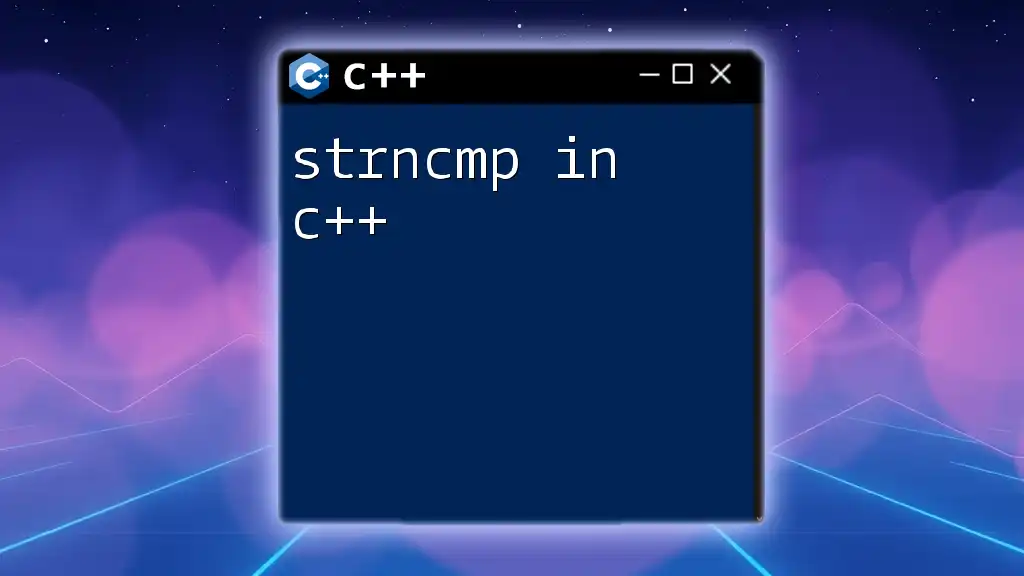
What is `cmp`?
The term `cmp` refers to a common way to implement comparison functionalities in C++. While there isn't a built-in function named `cmp`, developers often define their own `cmp` functions to facilitate custom comparisons. This is especially useful in contexts like sorting algorithms and ensuring a precise ordering of elements.
Historical Context
In the early days of C programming, comparisons were frequently done with simple functions. As C++ evolved, the need for more sophisticated comparison mechanisms led to a formalization of these functions. Custom comparison functions allow developers to encapsulate comparison logic cleanly, paving the way for improved code readability and reusability.
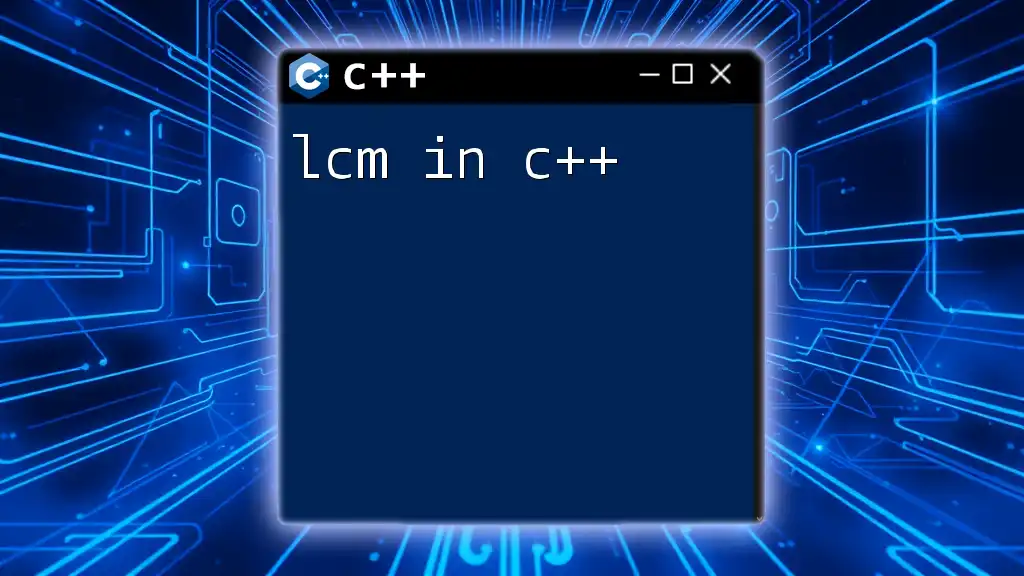
Implementing Comparison in C++
A comparison function typically returns an integer value that indicates the relationship between two items:
- Negative value: The first item is less than the second.
- Zero: The two items are equal.
- Positive value: The first item is greater than the second.
Here's an example of a simple comparison function in C++:
int compare(int a, int b) {
return (a < b) ? -1 : (a > b) ? 1 : 0;
}
This function evaluates the two integers `a` and `b`, returning `-1`, `0`, or `1` based on their comparison. This logic allows for flexible handling of different cases in larger algorithms.
Return Values of Comparison Functions
The return values of comparison functions have significant implications for their use. A return value of `-1` signifies that the first parameter is less than the second, whereas `1` indicates the opposite. Returning `0` means the two parameters are equivalent. Understanding these return values is critical when implementing functions that rely on comparisons, particularly when sorting or searching through data structures.
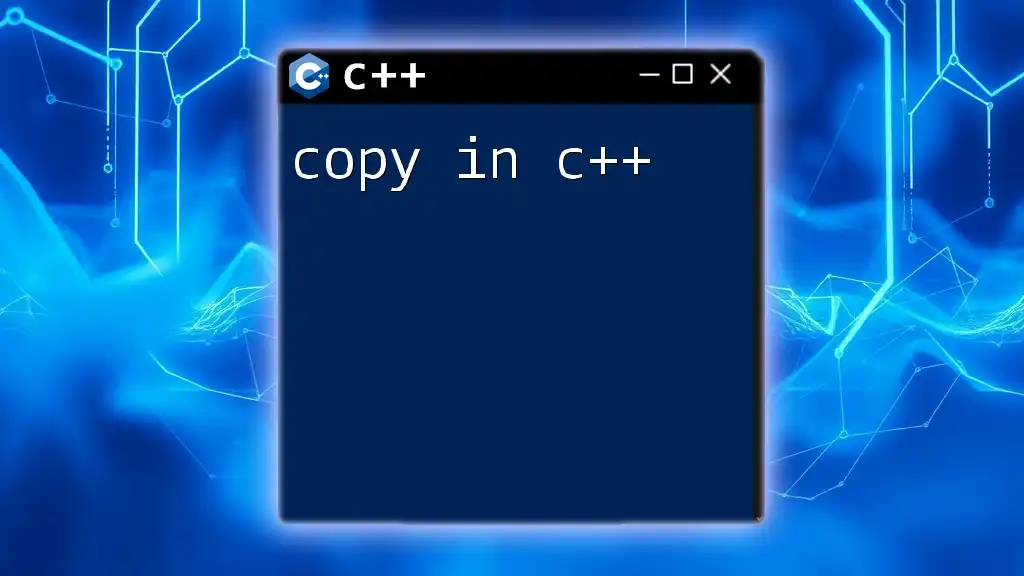
The `cmp` Function in C++ STL
The C++ Standard Library (STL) provides built-in support for sorting and comparing elements, making it easier to work with containers and algorithms. You can leverage a custom `cmp` function when using the `std::sort` algorithm.
Here's an example of using a custom `cmp` function in conjunction with `std::sort`:
#include <algorithm>
#include <vector>
bool cmp(int a, int b) {
return a < b; // Sort in ascending order
}
int main() {
std::vector<int> vec = {4, 2, 3, 1};
std::sort(vec.begin(), vec.end(), cmp);
return 0;
}
In this example, the `cmp` function defines the sorting order for the elements within the vector. By passing this function to `std::sort`, you can customize how elements are arranged.
Lambda Expressions as Alternative Comparison Tools
With the advent of modern C++, lambda expressions have emerged as powerful alternatives to traditional comparison functions. They allow for inline definition of comparison logic, enhancing readability and reducing boilerplate code. For instance, the previous sorting example can be rewritten using a lambda expression like so:
#include <algorithm>
#include <vector>
int main() {
std::vector<int> vec = {4, 2, 3, 1};
std::sort(vec.begin(), vec.end(), [](int a, int b) { return a < b; });
return 0;
}
This concise syntax minimizes the need for separate function declarations, streamlining the sorting process while maintaining the clarity of the comparison logic.
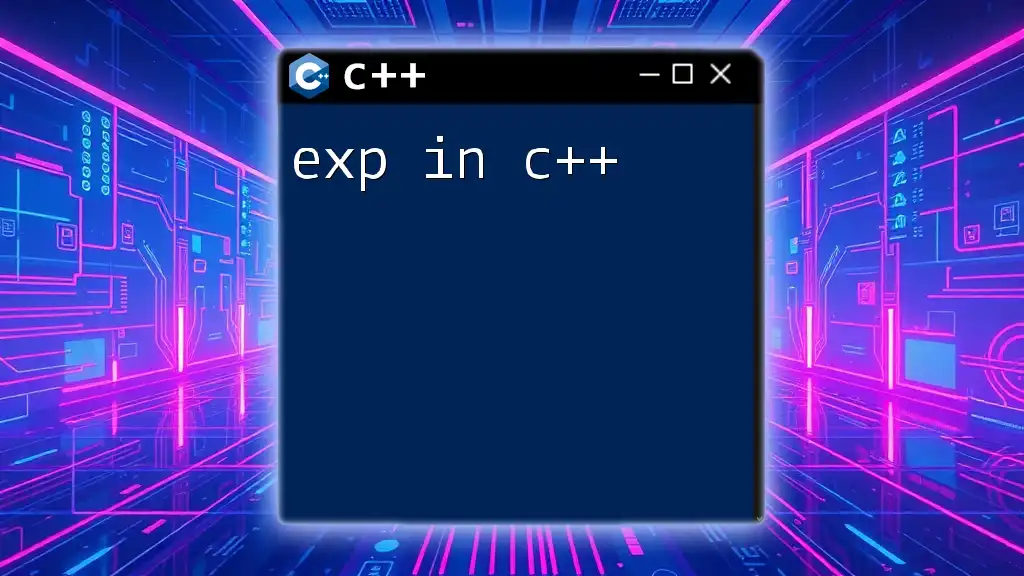
Best Practices for Writing Comparison Functions
When creating comparison functions, consider the following best practices to ensure clarity and efficiency:
-
Clarity: Keep your comparison logic straightforward and easy to understand. Avoid complex expressions that may confuse readers.
-
Ambiguity: Be mindful of scenarios where comparisons could yield ambiguous results, especially with floating-point values. It's advisable to use epsilon comparisons (`std::abs(a - b) < epsilon`) to determine equality.
-
Consistency: If you define custom comparison functions, ensure that they consistently produce the same relationship for the same inputs. This consistency is crucial for correct sorting and other algorithms.
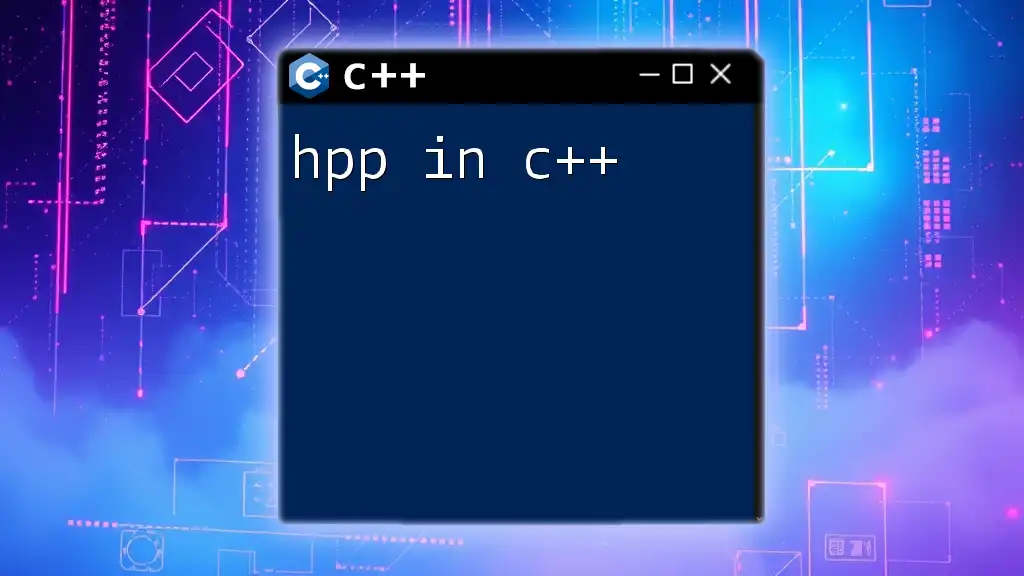
Performance Considerations
Comparison functions can significantly impact the performance of algorithms. A poorly designed comparison can lead to inefficiencies, especially in sorting and searching algorithms. Understanding the complexity of your comparison logic is essential; usually, it's best to aim for O(1) complexity for comparison functions to minimize overhead during sorting or searching operations.
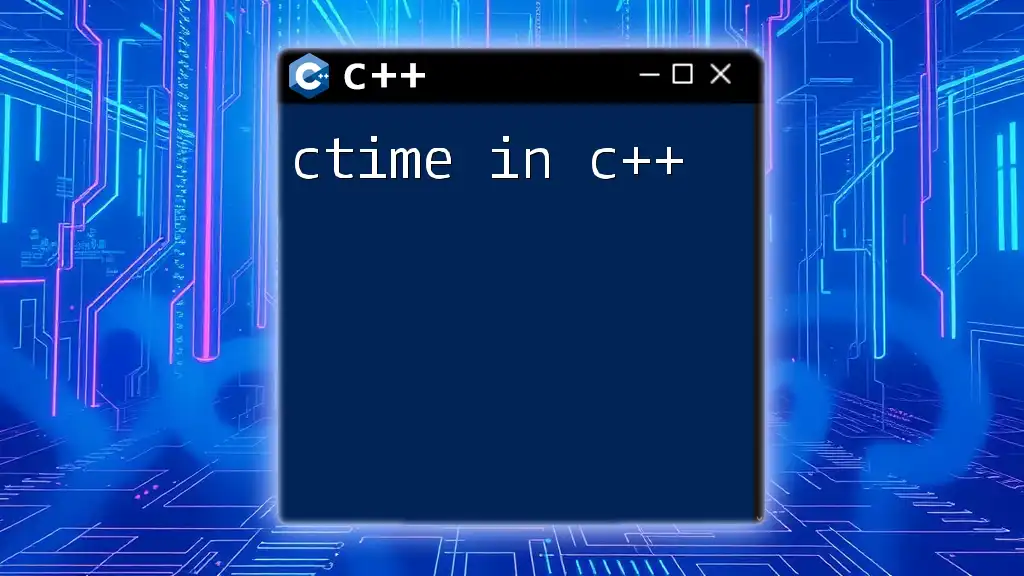
Conclusion
Throughout this guide, we've delved into cmp in C++ and its relevance in comparison operations, from understanding comparison fundamentals and implementing custom comparison functions to utilizing the C++ STL effectively. Whether using traditional functions or modern lambda expressions, having a robust grasp of comparison capabilities will enhance your coding proficiency and empower you to handle data structures efficiently.
With practice, you can master the art of writing effective comparison functions that contribute to cleaner, more performant code. Keep exploring and experimenting with different comparison methods to deepen your understanding and improve your programming skills.
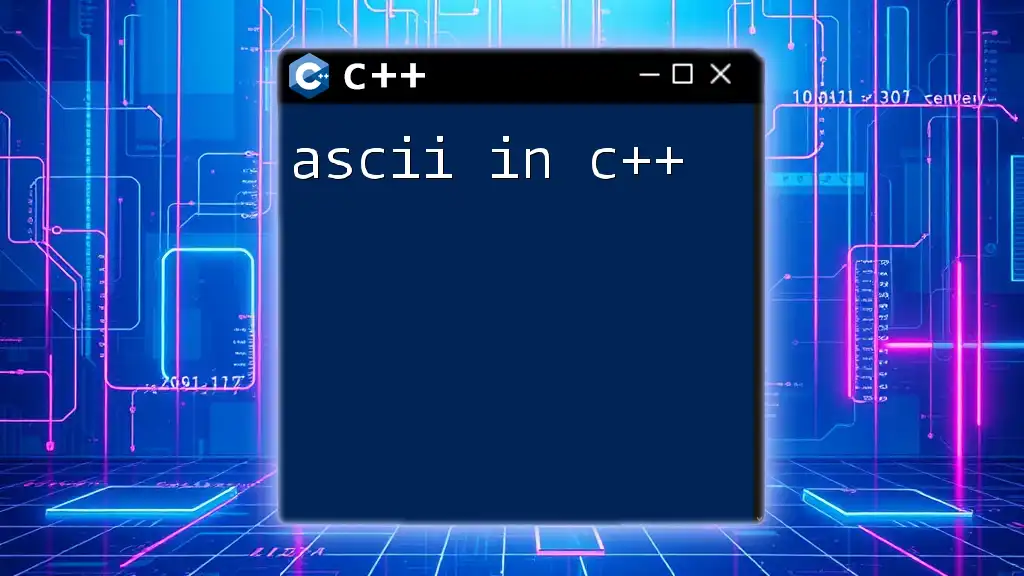
Additional Resources
For further reading on C++ comparison functions, consider exploring the official C++ documentation or online coding platforms where you can practice and refine your skills. Online forums and tutorials can also provide valuable insights and community support as you continue your journey into C++.