MPI (Message Passing Interface) is a powerful standard used in C++ for parallel programming, enabling processes to communicate with one another in distributed computing environments.
Here's a simple code snippet demonstrating an MPI "Hello, World!" program in C++:
#include <mpi.h>
#include <iostream>
int main(int argc, char** argv) {
MPI_Init(&argc, &argv);
int world_size;
MPI_Comm_size(MPI_COMM_WORLD, &world_size);
int world_rank;
MPI_Comm_rank(MPI_COMM_WORLD, &world_rank);
std::cout << "Hello, World! I am process " << world_rank << " of " << world_size << std::endl;
MPI_Finalize();
return 0;
}
Understanding MPI and Its Importance
What is MPI?
The Message Passing Interface (MPI) is a standardized and portable message-passing system designed to allow processes to communicate with each other in a distributed environment. MPI is crucial for achieving parallelism in computing, as it enables multiple processes to work on separate tasks while sharing data and results efficiently.
Use Cases of MPI
MPI is inherently versatile and can be applied in various domains, particularly in:
- High-performance computing (HPC): It enables massive simulations and data analytics where tasks can be parallelized across multiple nodes.
- Scientific simulations: Fields such as climate modeling, astrophysics, and molecular dynamics leverage MPI to simulate complex systems.
- Large-scale data processing: Industries dealing with vast datasets, like genomics and financial modeling, use MPI to harness the power of parallel computation for faster results.
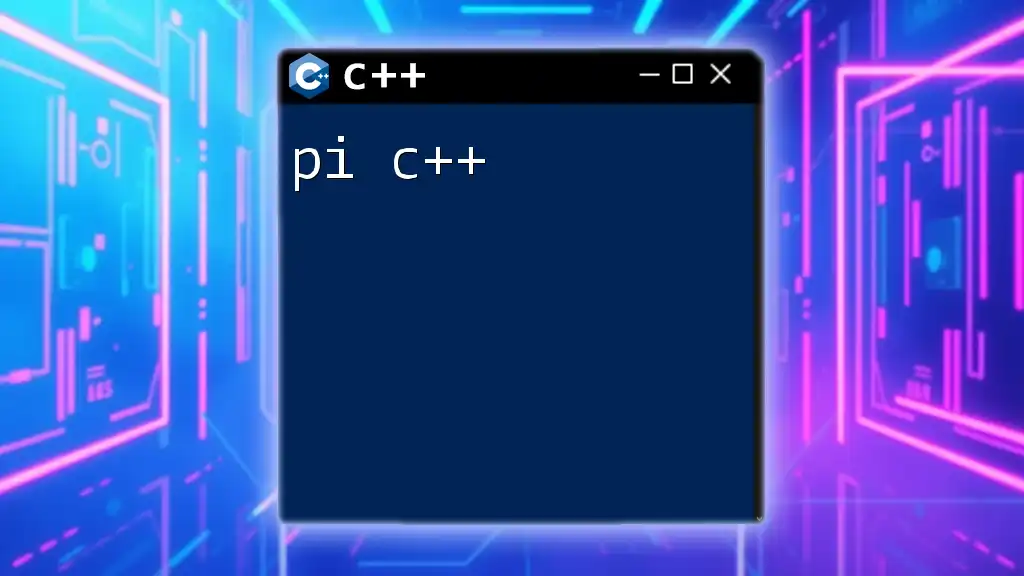
Setting Up Your Environment for MPI in C++
Installing MPI
To effectively work with MPI in your C++ projects, you need to install a suitable implementation. Two popular choices are MPICH and OpenMPI. Here's how you can set up MPI based on your operating system:
-
Linux:
- Use package managers:
sudo apt-get install mpich
- For OpenMPI, the command is:
sudo apt-get install openmpi-bin libopenmpi-dev
- Use package managers:
-
Windows:
- Download the latest binaries from the official [MPICH](https://www.mpich.org) or [OpenMPI](https://www.open-mpi.org) pages and follow the installation instructions.
-
macOS:
- Use Homebrew:
brew install open-mpi
- Use Homebrew:
Checking Your Installation
Once MPI is installed, it is essential to verify that it works correctly. You can compile and run a simple MPI program as follows:
#include <mpi.h>
#include <iostream>
int main(int argc, char* argv[]) {
MPI_Init(&argc, &argv);
int rank;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
std::cout << "Hello from process " << rank << std::endl;
MPI_Finalize();
return 0;
}
Compile the program with `mpic++` and run it using `mpirun -np <number_of_processes> ./your_program`. You'll see that each process outputs its rank.
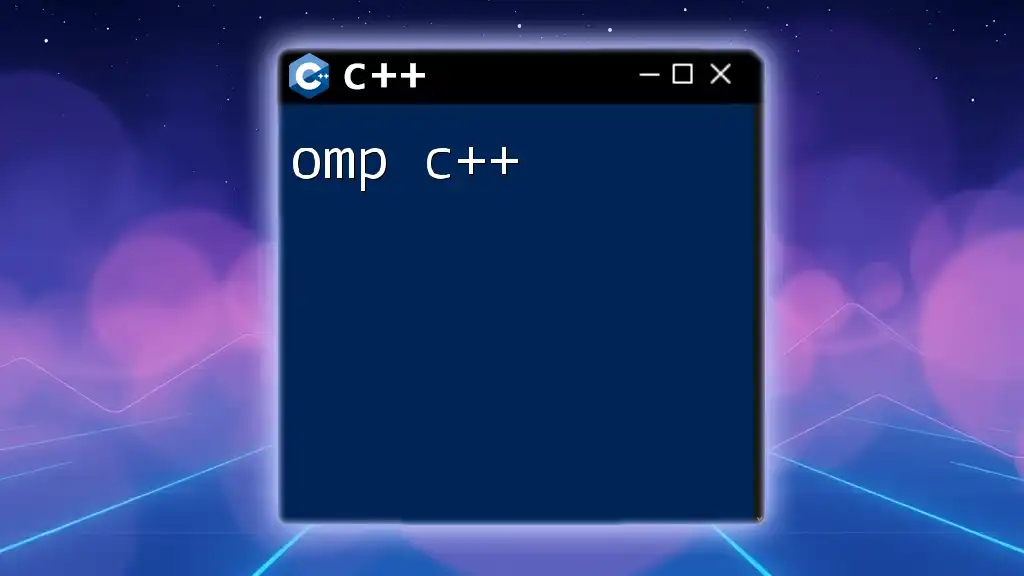
Basic Concepts of MPI in C++
Processes in MPI
In MPI, a process is an instance of a running program that can execute code independently. Each process has a unique rank that identifies it within a communicator (like `MPI_COMM_WORLD`). The size of the communicator refers to the total number of processes. Understanding these basics is key to effectively utilizing MPI.
Communication in MPI
MPI provides various means of communication. Processes can exchange information using:
- Point-to-point communication: Direct communication between two processes (e.g., `MPI_Send`, `MPI_Recv`).
- Collective communication: Involves all processes in a communicator (e.g., broadcasting messages to all processes).
It's crucial to understand the differences between blocking and non-blocking communications to manage performance effectively.
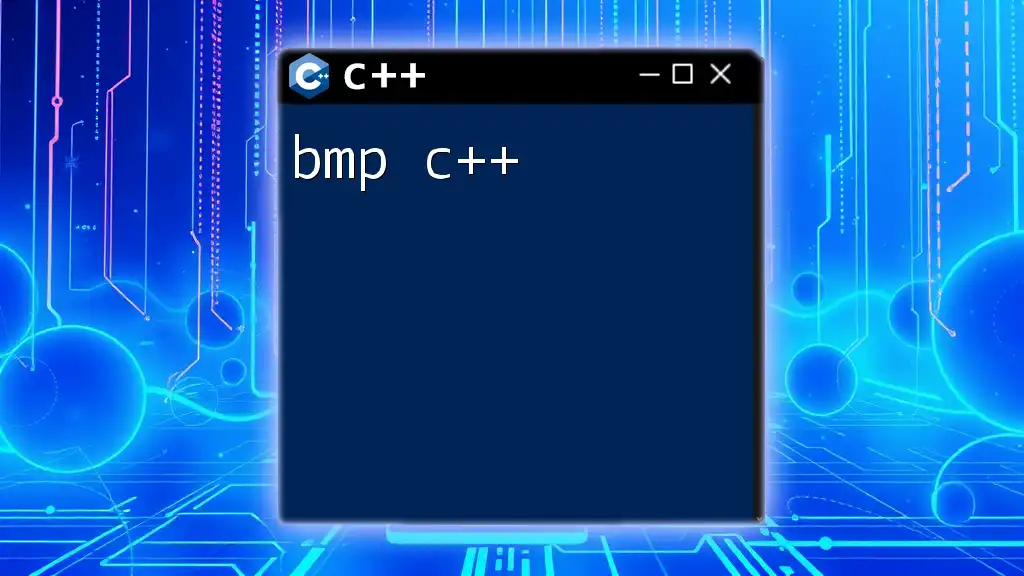
Core MPI Functions
Initialization and Finalization
Every MPI program begins with initializing the MPI environment and finishes by finalizing it. This is done using:
- `MPI_Init`: Initializes the MPI execution environment.
- `MPI_Finalize`: Terminates the MPI environment gracefully.
Here’s a starting template for an MPI program:
#include <mpi.h>
#include <iostream>
int main(int argc, char* argv[]) {
MPI_Init(&argc, &argv);
// Your code here
MPI_Finalize();
return 0;
}
Sending and Receiving Messages
To send and receive messages, MPI provides core functions:
- MPI_Send: This function sends a message from one process to another.
- MPI_Recv: This function receives a message from another process.
For example, the following code sends an integer from one process (rank 0) to another (rank 1):
int rank;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
if (rank == 0) {
int msg = 100;
MPI_Send(&msg, 1, MPI_INT, 1, 0, MPI_COMM_WORLD);
} else if (rank == 1) {
int msg;
MPI_Recv(&msg, 1, MPI_INT, 0, 0, MPI_COMM_WORLD, MPI_STATUS_IGNORE);
std::cout << "Received message: " << msg << std::endl;
}
Collective Communication
Collective operations manage data among all participating processes. They streamline communication and synchronization. Key functions include:
- MPI_Bcast: Broadcasts a message from one process to all others.
- MPI_Scatter: Distributes data from one process to all processes.
- MPI_Gather: Collects data from all processes to one.
- MPI_Reduce: Combines values from all processes and sends the result to one process.
Here’s an example using `MPI_Bcast`:
int number;
int rank;
MPI_Comm_rank(MPI_COMM_WORLD, &rank);
if (rank == 0) {
number = 42; // Say the root process sets the number
}
MPI_Bcast(&number, 1, MPI_INT, 0, MPI_COMM_WORLD);
std::cout << "Process " << rank << " received number: " << number << std::endl;
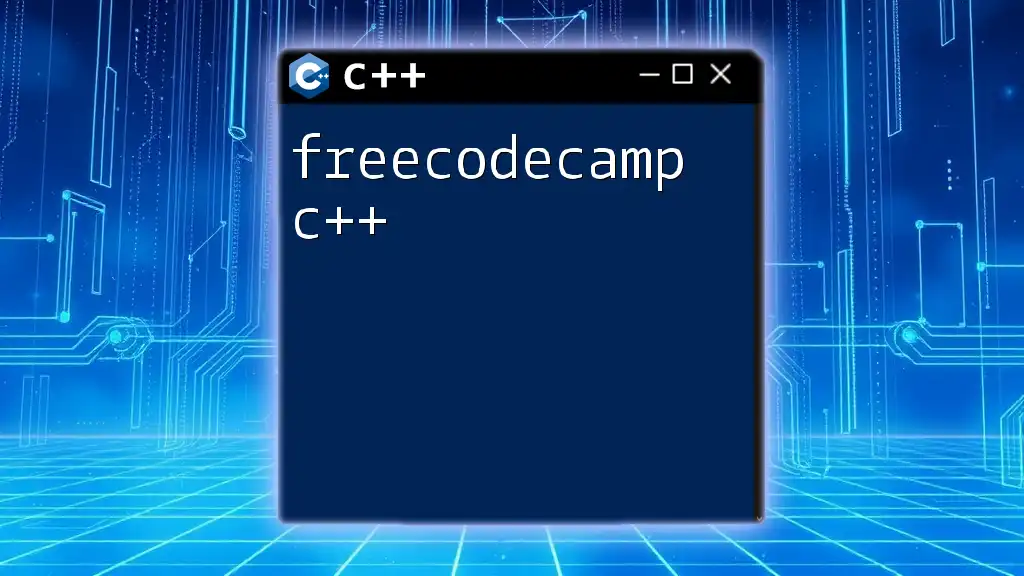
Debugging and Optimizing MPI Code
Common Issues in MPI Programming
Some common pitfalls in MPI programming include deadlocks (when processes are waiting indefinitely for each other) and race conditions (when the outcome depends on the sequence of events). Implementing proper synchronization mechanisms and ensuring robust error handling can help mitigate these issues.
Performance Optimization Techniques
To optimize MPI applications, profiling is essential. Tools like `mpiP` or `TAU` can assist in identifying bottlenecks. Consider these best practices:
- Choose the right payload size: Larger messages can reduce the overhead of communication.
- Balance computation and communication: Minimize the idle time of processors waiting for data.
- Reduce the frequency of communication: Attempt to send larger chunks of data rather than many smaller messages.
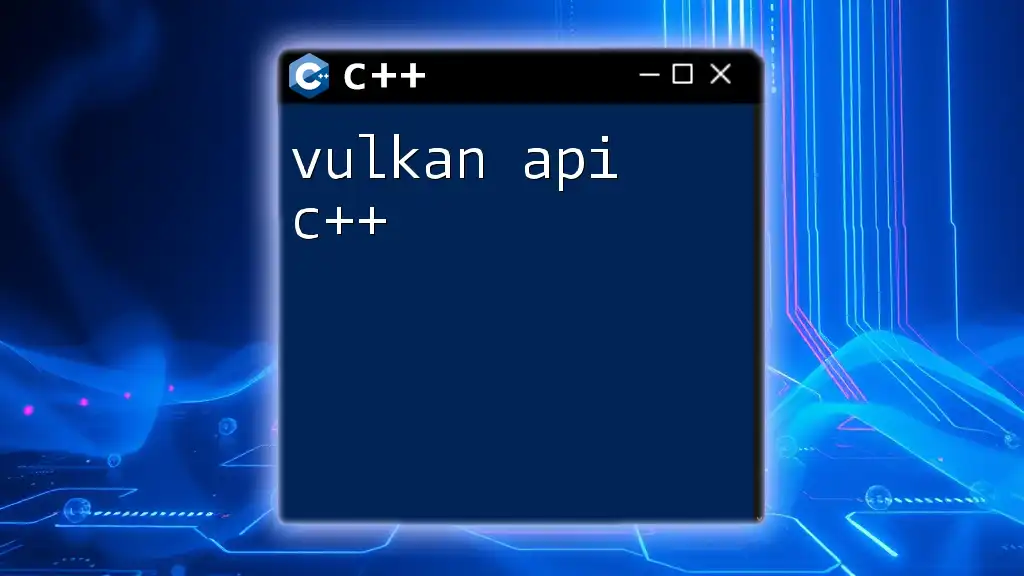
Advanced Features of MPI
Handling Errors in MPI
Error handling is vital in MPI programming. MPI provides a range of error codes, and you can use `MPI_Error_string` to convert these codes into readable messages. This aids in debugging and ensuring the reliability of distributed applications.
Creating Custom Datatypes
Sometimes, it’s efficient to define complex data structures for communication. MPI allows the creation of custom datatypes using `MPI_Type_create_struct`. This capability reduces the overhead associated with sending multiple individual pieces of data.
Example of creating a custom datatype:
typedef struct {
int a;
float b;
} mydata_t;
MPI_Datatype mydata_type;
MPI_Type_create_struct(...); // Implementation details depending on the structure
Non-Blocking Communication
Non-blocking communication functions, like `MPI_Isend` and `MPI_Irecv`, allow processes to continue their computation without waiting for communication to finish. This can lead to better resource utilization:
MPI_Request request;
MPI_Isend(&msg, 1, MPI_INT, 0, 0, MPI_COMM_WORLD, &request);
// Other work can be done here
MPI_Wait(&request, MPI_STATUS_IGNORE);
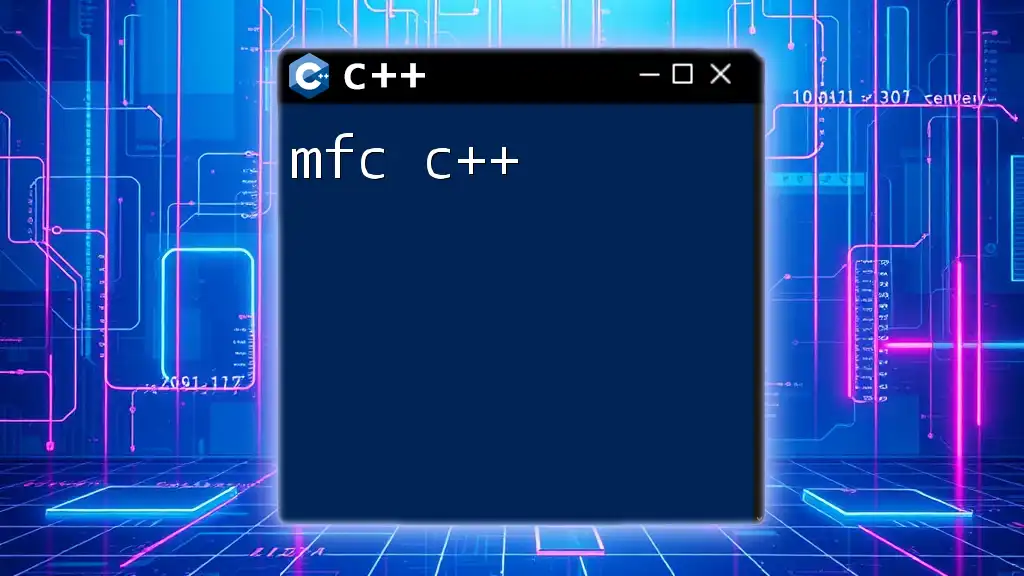
Real-World Applications of MPI
Case Studies
Several organizations leverage MPI for groundbreaking projects. For example, supercomputing centers use MPI in weather modeling, while pharmaceutical companies implement it in drug discovery simulations. The ability to handle massive datasets and complex calculations makes MPI invaluable.
Future of MPI
The future of MPI looks promising as advancements in hardware and software lead to evolving paradigms in parallel computing. Continued community development and the integration of new technologies, such as hybrid MPI/OpenMP approaches, keep MPI relevant in modern computing.
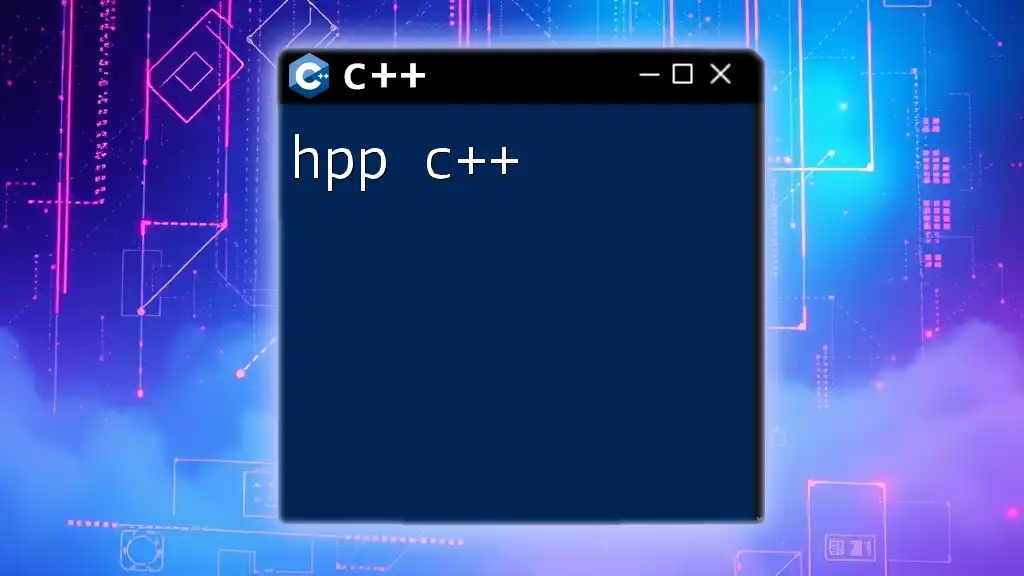
Conclusion
Summary of Key Points
MPI is a powerful tool for networked computing, enabling efficient process communication in C++. By understanding its core principles, functions, and best practices, users can harness its potential for high-performance applications effectively.
Additional Resources
For further reading and exploration, consider diving into MPI documentation, tutorials available online, and recommended books on parallel programming. Engaging with the community through forums or workshops can also enhance your understanding and application of MPI in C++.