OpenMP (Open Multi-Processing) is an API that supports multi-platform shared memory multiprocessing programming in C++ by allowing developers to easily parallelize their code with simple compiler directives.
Here's a basic example of using OpenMP to parallelize a loop in C++:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel for
for (int i = 0; i < 10; i++) {
std::cout << "Thread " << omp_get_thread_num() << " is processing iteration " << i << std::endl;
}
return 0;
}
Introduction to OpenMP
What is OpenMP?
OpenMP (Open Multi-Processing) is an API that supports multi-platform shared memory multi-processing programming in C, C++, and Fortran. It simplifies the process of developing parallel applications by using compiler directives for defining parallel regions and managing the shared resources of the program. OpenMP is particularly useful as it allows developers to leverage the power of multiprocessor systems without resorting to lower-level threading methods.
Why Use OpenMP in C++?
OpenMP brings numerous benefits to C++ programming:
- Speed Up Execution Time: By executing multiple threads concurrently, you can significantly reduce the total execution time of CPU-bound programs.
- Ease of Use: OpenMP features a straightforward syntax, allowing developers to add parallelism with minimal code modifications.
- Compatibility: It can be incrementally added to existing applications, providing an easy path to optimize performance without a complete rewrite of the code.
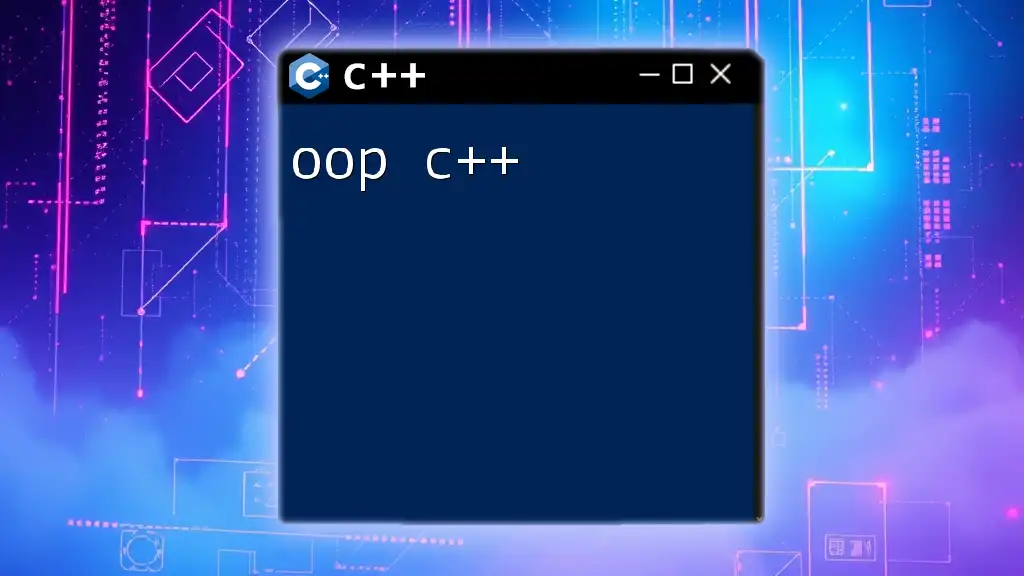
Understanding Parallel Programming
What is Parallel Programming?
Parallel programming is the technique of dividing a task into smaller sub-tasks which can be solved concurrently, leveraging multiple processors.
Key Concepts in Parallelism
In parallel programming, it’s essential to understand the distinction between threads and processes. Threads are lightweight and share the same memory space, while processes are heavier and operate in separate memory spaces. Shared data among threads can lead to race conditions, while private data ensures that each thread has its own independent instance.
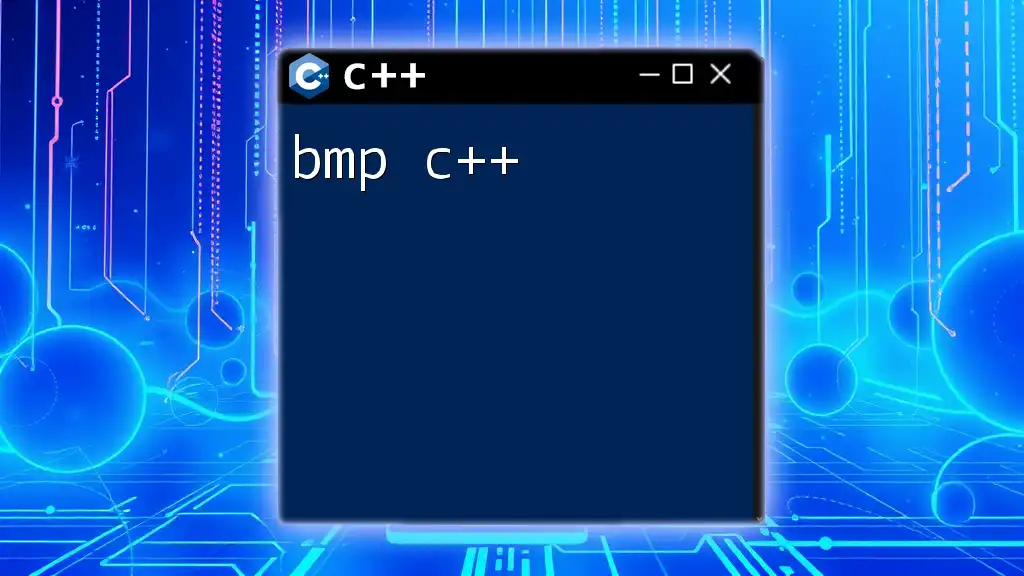
Getting Started with OpenMP in C++
Setting Up Your Environment
To get started with OpenMP in C++, you’ll need a compiler that supports OpenMP. Popular options include GCC and Microsoft Visual Studio. Often, enabling OpenMP merely requires adding a flag when compiling:
- For GCC, use the `-fopenmp` flag:
g++ -fopenmp -o my_program my_program.cpp
Basic OpenMP Syntax and Commands
A basic structure for using OpenMP features involves adding special annotations or directives in your code, initiated with `#pragma`. Here's an example showcasing a simple "Hello, World!" program using OpenMP:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel
{
std::cout << "Hello, World from thread " << omp_get_thread_num() << "!" << std::endl;
}
return 0;
}
In this example, multiple threads will print the message, showcasing the parallel execution.
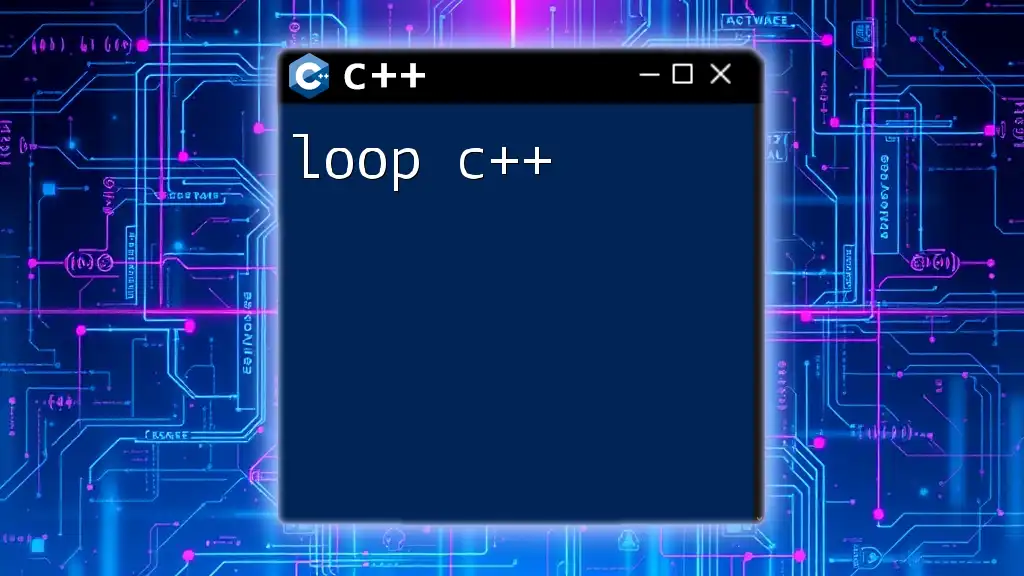
OpenMP Directives Explained
The `#pragma omp parallel` Directive
This directive creates a parallel region where threads are spawned. Each thread executes concurrently, and you can control the number of threads using `omp_set_num_threads()`. Here’s how you can implement this in code:
#include <iostream>
#include <omp.h>
int main() {
omp_set_num_threads(4); // Set the number of threads
#pragma omp parallel
{
std::cout << "Thread " << omp_get_thread_num() << " is executing." << std::endl;
}
return 0;
}
Work Sharing Constructs
`#pragma omp for`
This directive allows splitting the iterations of a loop across multiple threads, enhancing the performance drastically when you have large datasets. Here's a practical example:
#include <iostream>
#include <omp.h>
int main() {
const int N = 100;
int sum = 0;
#pragma omp parallel for reduction(+:sum)
for (int i = 1; i <= N; i++) {
sum += i; // Each thread calculates a piece of the sum
}
std::cout << "Sum is: " << sum << std::endl;
return 0;
}
In this example, each thread processes a portion of the loop, and the final sum is collected in a thread-safe manner using the reduction clause.
`#pragma omp sections`
If you have different, independent tasks that can be executed in parallel, you can use OpenMP sections. Each section represents a separate block of code executed by a different thread:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel
{
#pragma omp sections
{
#pragma omp section
{
std::cout << "Running Task 1 in thread " << omp_get_thread_num() << std::endl;
}
#pragma omp section
{
std::cout << "Running Task 2 in thread " << omp_get_thread_num() << std::endl;
}
}
}
return 0;
}
This allows for flexibility in executing completely different tasks in parallel.
Synchronization Constructs
Mutexes and Critical Sections
Race conditions can occur when multiple threads attempt to modify shared data simultaneously. To avoid this, you can use the `#pragma omp critical` directive:
#include <iostream>
#include <omp.h>
int main() {
int shared_var = 0;
#pragma omp parallel for
for (int i = 0; i < 100; i++) {
#pragma omp critical
{
shared_var += 1; // Only one thread at a time can execute this
}
}
std::cout << "Final value of shared_var is: " << shared_var << std::endl;
return 0;
}
This guarantees that increments to `shared_var` are safe from concurrent modification issues.
Barriers
Barriers in OpenMP can synchronize all threads in a parallel region. For example, after completing a task in a parallel section, you might want all the threads to reach the same point before proceeding:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel
{
std::cout << "Thread " << omp_get_thread_num() << " is working." << std::endl;
#pragma omp barrier // Wait here until all threads reach this point
if (omp_get_thread_num() == 0) {
std::cout << "All threads completed their tasks." << std::endl;
}
}
return 0;
}
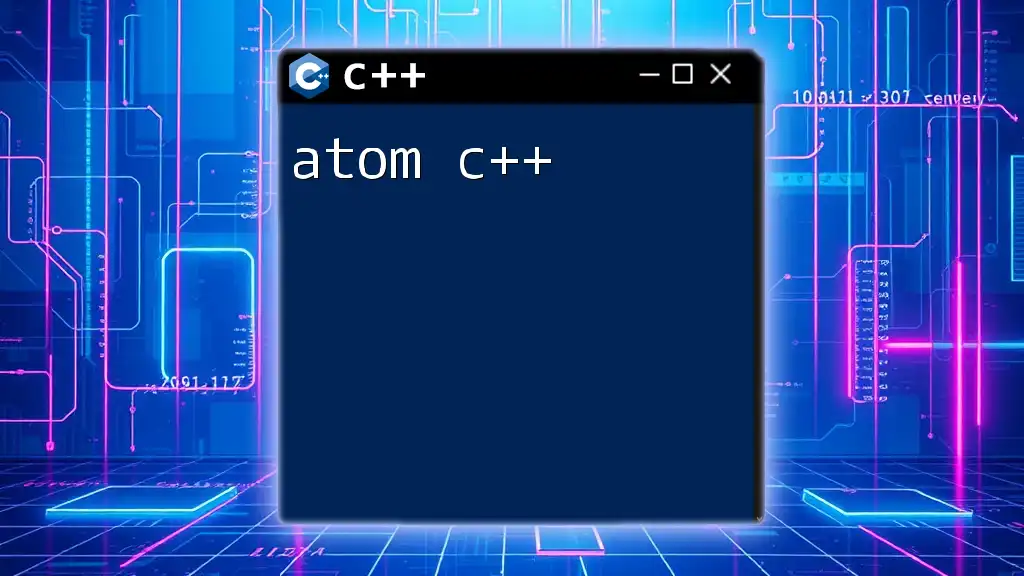
Advanced OpenMP Features
Nested Parallelism
Sometimes, you might want to create parallel regions within parallel regions, known as nested parallelism. You can enable it using `omp_set_nested(1)`:
#include <iostream>
#include <omp.h>
int main() {
omp_set_nested(1); // Enable nested parallelism
#pragma omp parallel
{
std::cout << "Outer Thread " << omp_get_thread_num() << " started." << std::endl;
#pragma omp parallel
{
std::cout << "Inner Thread " << omp_get_thread_num() << " is executing." << std::endl;
}
}
return 0;
}
This can be powerful but should be used judiciously to avoid overwhelming the system with threads.
Dynamic Thread Management
The capability to dynamically adjust the number of threads during program execution can optimize resource usage. Here’s how:
#include <iostream>
#include <omp.h>
int main() {
omp_set_dynamic(1); // Allow dynamic adjustment of thread count
omp_set_num_threads(8); // Set a maximum limit
#pragma omp parallel
{
std::cout << "Thread " << omp_get_thread_num() << " is executing." << std::endl;
}
return 0;
}
OpenMP will decide based on current workload how many threads to use, which can lead to performance improvements.
Tasking in OpenMP
Tasks are another way of managing parallel work. With `#pragma omp task`, you can create tasks that run concurrently with other tasks and threads:
#include <iostream>
#include <omp.h>
int main() {
#pragma omp parallel
{
#pragma omp single // Ensure only one thread creates tasks
{
for (int i = 0; i < 5; i++) {
#pragma omp task
{
std::cout << "Task " << i << " is being executed by thread " << omp_get_thread_num() << std::endl;
}
}
}
}
return 0;
}
This flexibility in task management can lead to more efficient parallel processing, particularly when tasks vary in duration.
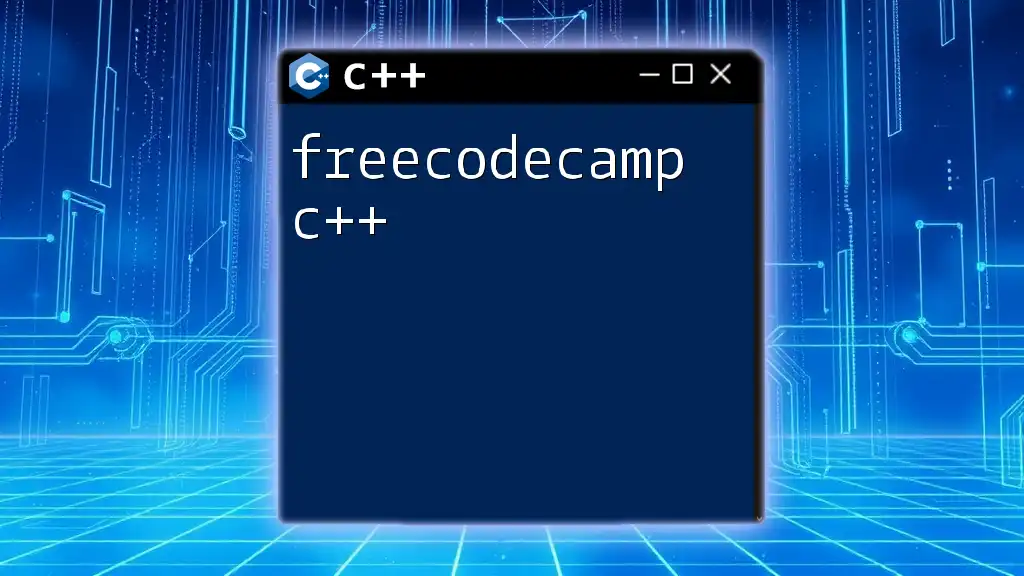
Performance Considerations
Measuring Performance with OpenMP
Validating the performance of your parallelized code is essential. Tools like gprof or valgrind can be used effectively for profiling applications to find any bottlenecks or inefficiencies in your OpenMP code.
Common Performance Bottlenecks
Parallel programming is not without its drawbacks. Common issues include:
- Overhead of Parallel Regions: Sometimes, the benefits of parallelization may be offset by the overhead of thread management.
- False Sharing: This occurs when threads on different processors modify variables that reside on the same cache line, resulting in poor performance.
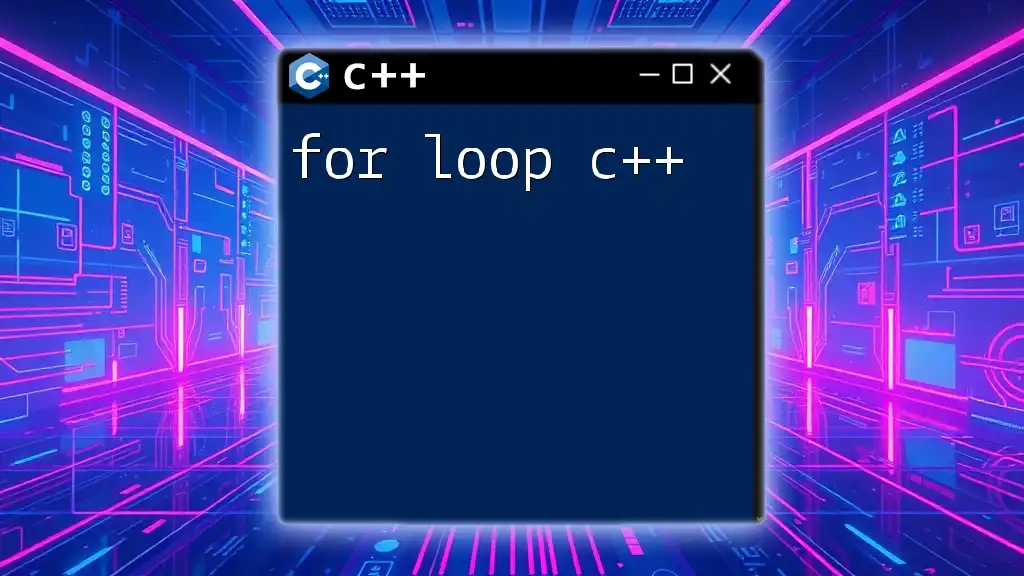
Best Practices for Using OpenMP in C++
Tips and Tricks
- Keep Workloads Balanced: Ensuring all threads have a similar amount of work helps maximize efficiency.
- Minimize Synchronization Needs: Use local data wherever possible to reduce competition for shared resources and improve performance.
Avoiding Common Pitfalls
Be aware of thread safety when modifying shared variables. Also, watch out for deadlocks that can occur if multiple threads are waiting on each other.
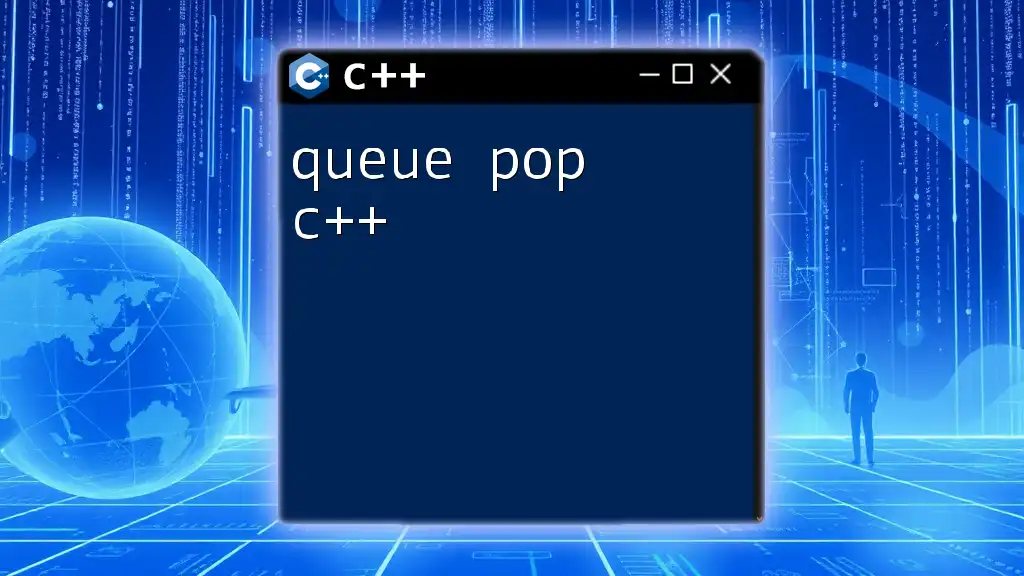
Conclusion
OpenMP provides a powerful toolkit for developers looking to harness the capabilities of parallel processing in C++. With straightforward directives and constructs, integrating parallelism into C++ programs can be both efficient and effective. As parallel programming becomes increasingly critical in developing high-performance applications, mastering OpenMP is a valuable skill for any C++ developer. Embrace the opportunity to enhance your applications and explore how OpenMP can elevate your coding practices.
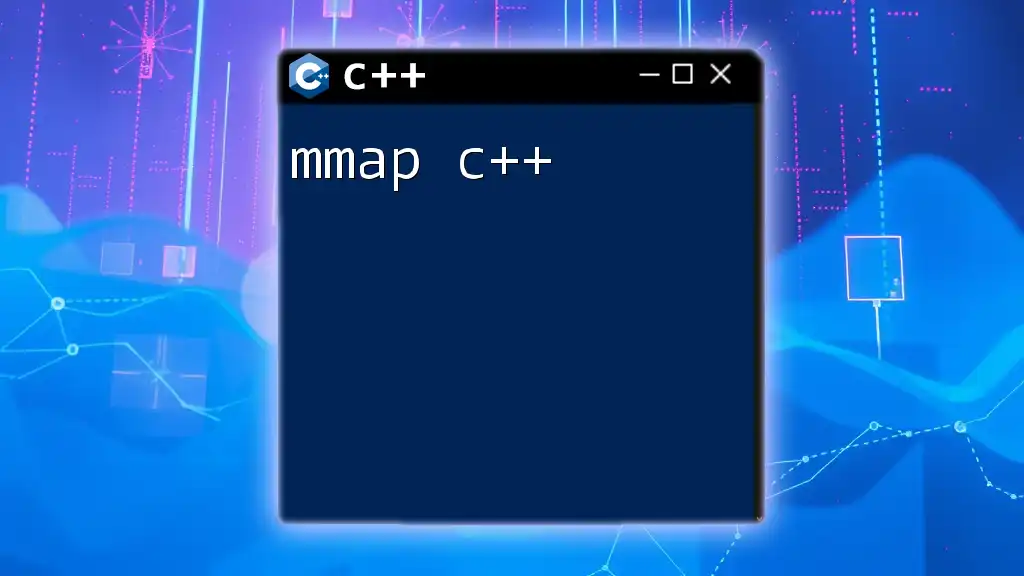
Additional Resources
- Books and Online Courses: Consider exploring comprehensive resources and courses designed specifically for OpenMP and parallel programming in C++.
- OpenMP Official Documentation: The [OpenMP official website](https://www.openmp.org) is a treasure trove of information and updates.
- Communities and Forums: Join online forums and communities to share knowledge and gain insights into best practices and advanced uses of OpenMP in C++.
By following this guide, you’ll have a solid foundation to start using OpenMP in C++, optimizing your programs for performance!