ZeroMQ (0mq) is a high-performance asynchronous messaging library that allows developers to build scalable and distributed applications in C++ with ease.
Here's a simple example of a ZeroMQ publisher in C++:
#include <zmq.hpp>
#include <string>
#include <iostream>
#include <unistd.h>
int main() {
zmq::context_t context(1);
zmq::socket_t publisher(context, ZMQ_PUB);
publisher.bind("tcp://*:5555");
while (true) {
std::string message = "Hello, World!";
publisher.send(zmq::buffer(message), zmq::send_flags::none);
std::cout << "Message sent: " << message << std::endl;
sleep(1);
}
return 0;
}
What is ZeroMQ?
ZeroMQ, often abbreviated as 0MQ, is a high-performance messaging library that provides an asynchronous, message-oriented communication framework. It simplifies messaging patterns and allows developers to build scalable and distributed applications with ease. Unlike traditional message brokers and queues, ZeroMQ operates without a central message broker, enabling direct communication between distributed applications.
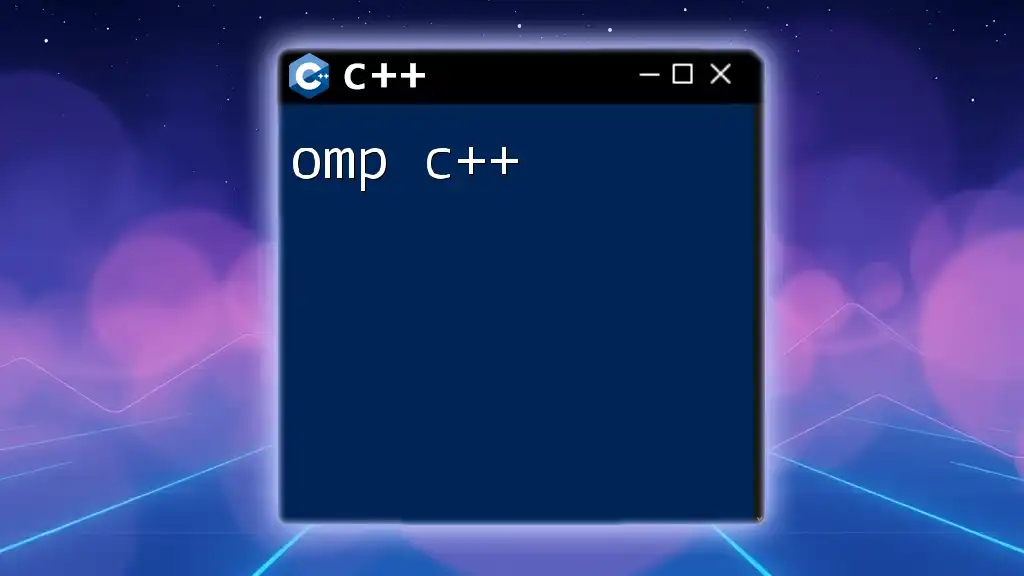
Why Use ZeroMQ in C++?
Using ZeroMQ in C++ offers several significant advantages over traditional messaging protocols.
-
Performance: ZeroMQ is designed for speed and scalability. Its lightweight nature means messages are sent with minimal overhead, making it suitable for high-throughput applications.
-
Simplicity: Developers can implement complex messaging patterns without the need to manage threads or sockets directly. The library abstracts much of the complexity, allowing you to focus on application logic.
-
Versatility: ZeroMQ supports various messaging patterns, enabling developers to implement different architectures (like publish-subscribe, request-reply, etc.) with minimal effort.
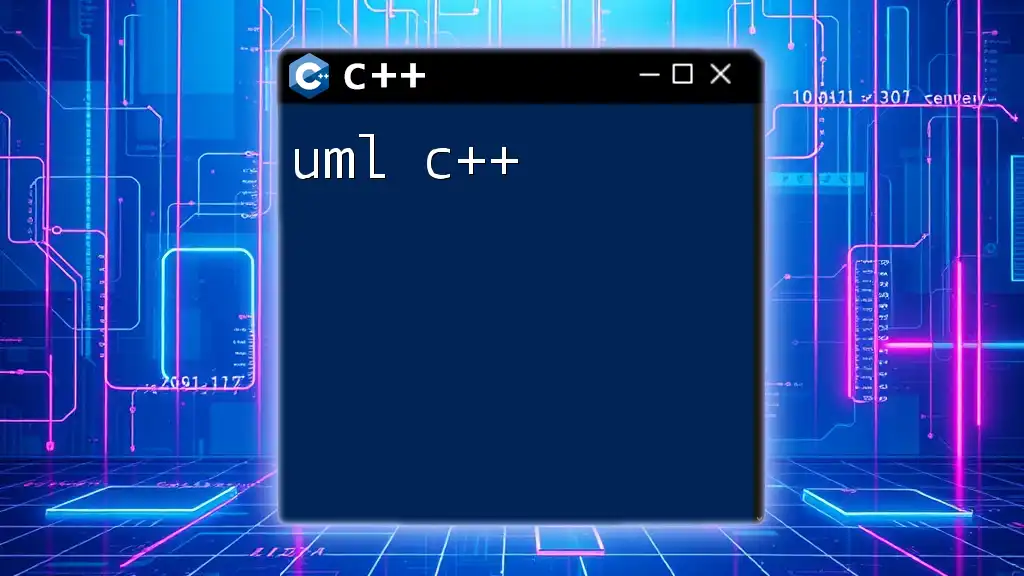
Setting Up ZeroMQ for C++
Installing ZeroMQ
Before changing your C++ applications with 0MQ, you must first install the library on your system. Here are the installation instructions based on the operating system you are using:
-
Linux: You can easily install ZeroMQ using your package manager. For example, on Debian-based systems:
sudo apt-get install libzmq3-dev
-
Windows: You can use `vcpkg` to manage installations:
vcpkg install zeromq
-
MacOS: Homebrew is the go-to package manager:
brew install zeromq
Including ZeroMQ in Your C++ Project
Once ZeroMQ is installed, you need to link the library in your C++ project. If you're using CMake, here is a basic example of how to modify your `CMakeLists.txt`:
find_package(ZMQ REQUIRED)
include_directories(${ZMQ_INCLUDE_DIRS})
target_link_libraries(your_target_name ${ZMQ_LIBRARIES})
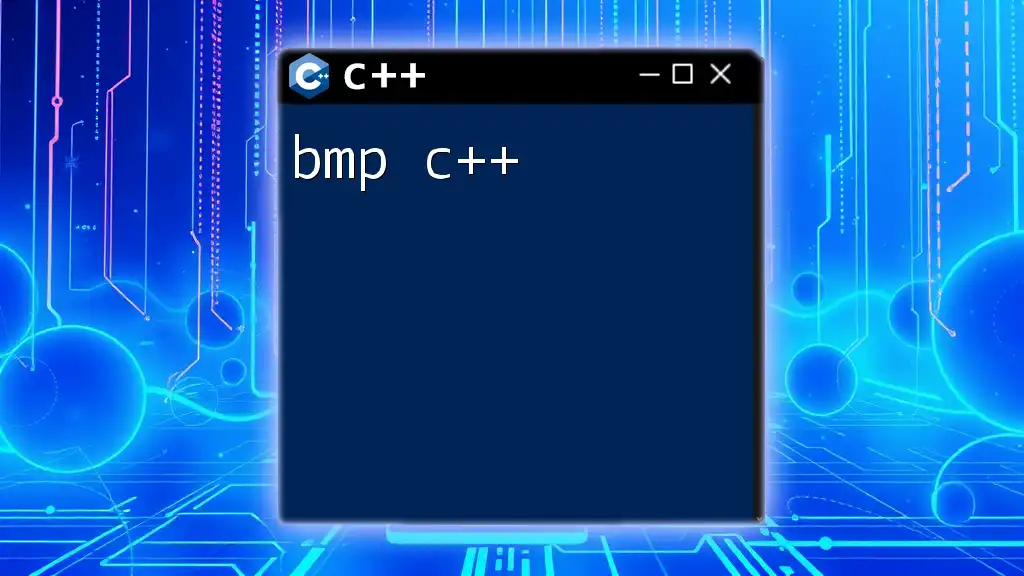
Core Concepts of ZeroMQ
Messaging Patterns in ZeroMQ
ZeroMQ supports several messaging patterns, which dictate how messages are sent and received. Understanding these patterns is crucial for effective communication in your applications:
-
Request-Reply: This pattern is suitable for client-server communications. The client sends a request and waits for a reply before continuing.
-
Publish-Subscribe: This pattern allows publishers to send messages to multiple subscribers. Subscribers only receive messages that are relevant to them, based on the topics they subscribe to.
-
Push-Pull: This is a load-balancing pattern. Messages are sent from one or more producers (pushers) to one or more consumers (pullers), evenly distributing the workload.
Understanding Sockets in ZeroMQ
In ZeroMQ, a socket is not the same as a traditional socket but is instead a high-level abstraction that simplifies messaging. Here are some common socket types you will encounter:
-
REQ (Request) and REP (Reply): Used for request-reply patterns. The REQ socket sends requests and waits for replies, while the REP socket listens for requests and sends responses.
-
PUB (Publisher) and SUB (Subscriber): Used for publish-subscribe patterns. The PUB socket broadcasts messages, and the SUB socket subscribes to receive specific messages based on topics.
-
PUSH (Push) and PULL (Pull): Used for task distribution. The PULL socket distributes messages evenly among multiple PUs (Pushers).
Example of creating a socket in C++:
zmq::context_t context(1);
zmq::socket_t socket(context, ZMQ_REQ); // Create a REQ socket
socket.connect("tcp://localhost:5555"); // Connect to a server endpoint
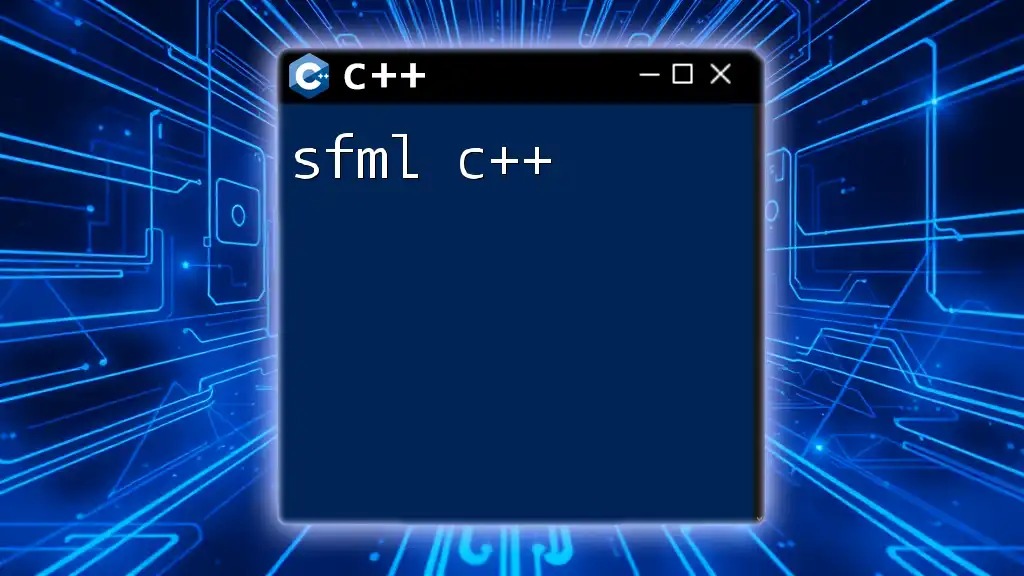
Getting Started with ZeroMQ in C++
Creating a Basic ZeroMQ Application
To illustrate how 0MQ works, let’s create a simple "Hello World" application using the request-reply messaging pattern. This example consists of both a server and a client.
Server Code
#include <zmq.hpp>
#include <string>
#include <iostream>
int main() {
zmq::context_t context(1);
zmq::socket_t socket(context, ZMQ_REP);
socket.bind("tcp://*:5555");
while (true) {
zmq::message_t request;
socket.recv(&request);
std::string received_msg(static_cast<char*>(request.data()), request.size());
std::cout << "Received: " << received_msg << std::endl;
std::string reply_msg = "Hello from server";
zmq::message_t reply(reply_msg.size());
memcpy(reply.data(), reply_msg.data(), reply_msg.size());
socket.send(reply);
}
return 0;
}
Client Code
#include <zmq.hpp>
#include <string>
#include <iostream>
int main() {
zmq::context_t context(1);
zmq::socket_t socket(context, ZMQ_REQ);
socket.connect("tcp://localhost:5555");
std::string request_msg = "Hello from client";
zmq::message_t request(request_msg.size());
memcpy(request.data(), request_msg.data(), request_msg.size());
socket.send(request);
zmq::message_t reply;
socket.recv(&reply);
std::string reply_msg(static_cast<char*>(reply.data()), reply.size());
std::cout << "Received: " << reply_msg << std::endl;
return 0;
}
Handling Messages
Sending and receiving messages in ZeroMQ is straightforward. You wrap your data into a `zmq::message_t` object, send it over the socket, and receive it using the respective socket type. Here’s an example that shows sending simple strings and serialized messages.
To handle serialized messages, you can use libraries like protobuf or flatbuffers. This ensures your messages are serialized properly, especially when sending complex data structures.
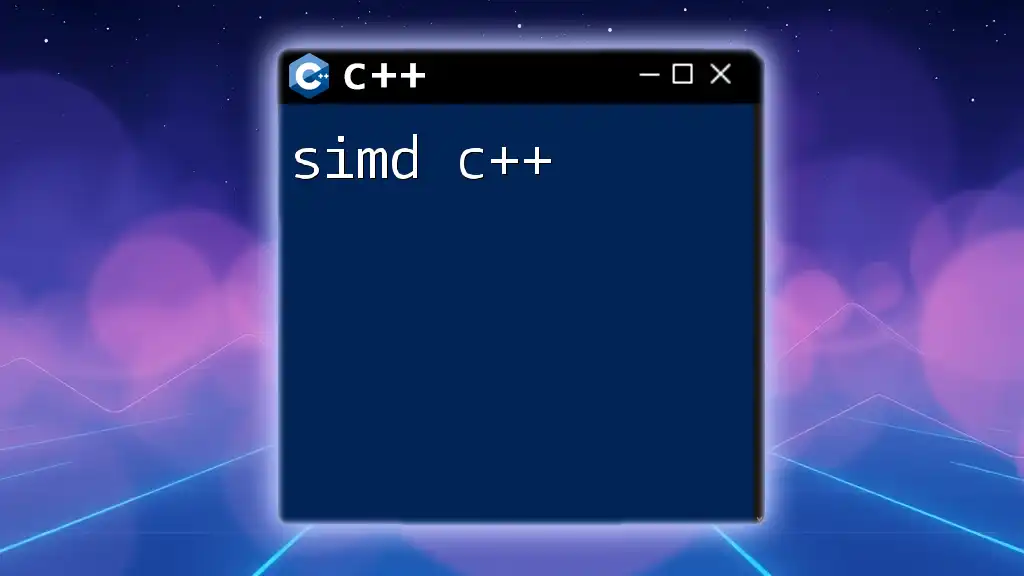
Advanced Topics in ZeroMQ
Error Handling in ZeroMQ
Effective error handling is critical in messaging applications. ZeroMQ provides several mechanisms to detect issues, such as timeouts and malformed messages. For instance, you can use a try-catch block around your send and receive operations. Here’s an example:
try {
socket.send(message);
} catch (const zmq::error_t &e) {
std::cerr << "Failed to send message: " << e.what() << std::endl;
}
Performance Optimization Techniques
To get the best performance out of ZeroMQ, consider these optimization techniques:
-
Message Batching: Instead of sending messages individually, batch them together to reduce overhead.
-
Use of Asynchronous I/O: ZeroMQ sockets can be polled for events, allowing you to react only when it's ready, keeping your application efficient.
-
Configure Socket Options: Fine-tune socket options like `SO_SNDBUF` and `SO_RCVBUF` to optimize network performance.
Integrating ZeroMQ with Other C++ Libraries
One powerful aspect of ZeroMQ is its ability to integrate with other C++ libraries. For instance, combining ZeroMQ with Boost can enhance your application's capabilities. You might use Boost for asynchronous tasks while employing ZeroMQ for messaging. Here’s how you could set that up:
#include <boost/asio.hpp>
#include <zmq.hpp>
// Use Boost's io_service for asynchronous tasks while ZeroMQ handles messaging
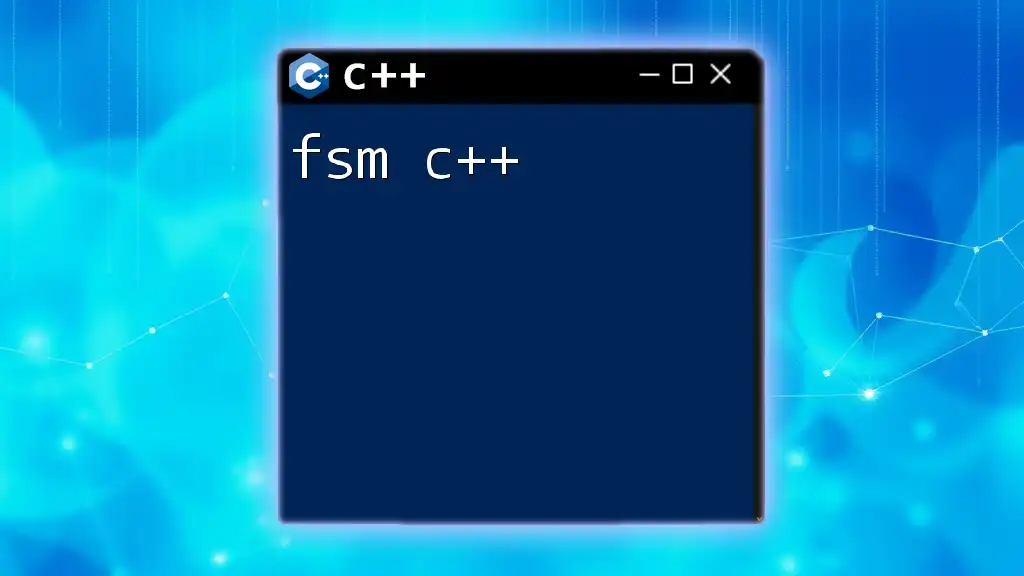
Debugging ZeroMQ Applications
Common Issues and Solutions
When developing with 0MQ, you might encounter certain issues. Some common problems include:
-
Socket connection errors: Ensure endpoints are correctly specified and services are running. If you cannot connect, verify firewall settings and network availability.
-
Message loss: If you experience missed messages, consider using QoS features or analyzing your workflow for potential bottlenecks.
Tools for Monitoring ZeroMQ
Tools such as ZeroMQ Monitor and Wireshark can provide insights into messaging operations. Monitoring the performance of your ZeroMQ applications ensures you can diagnose issues before they escalate.
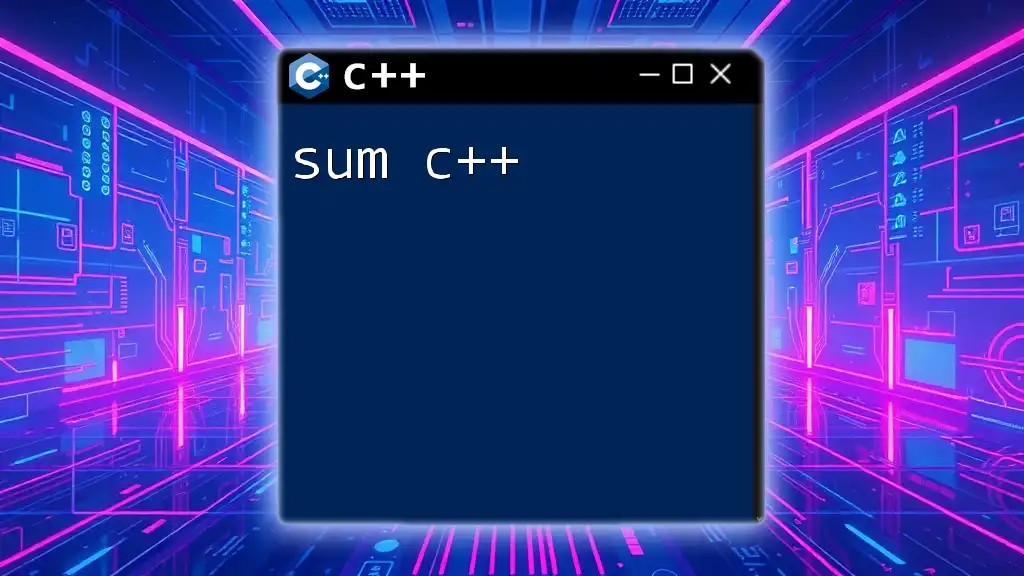
Real-World Applications of ZeroMQ in C++
Case Studies
Several companies have successfully integrated ZeroMQ into their applications. For example, ZeroMQ is widely used in financial trading platforms for low-latency message passing. By using 0MQ, these platforms can efficiently manage vast streams of pricing data and user transactions with minimal lag.
Community and Resources
The ZeroMQ community is vibrant and robust. Engaging with forums and discussions can enhance your understanding and provide solutions to challenges you might face. Key resources include:
- ZeroMQ's Official Documentation: A comprehensive guide to all features.
- GitHub Repositories: Explore various projects that implement ZeroMQ.
- Books: Look for titles specifically covering ZeroMQ and advanced messaging patterns.
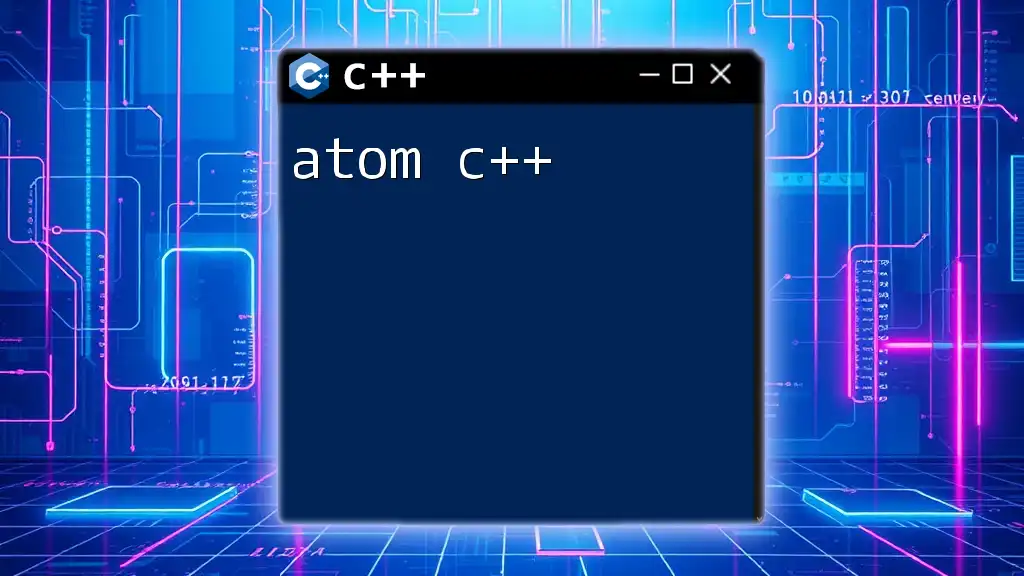
Conclusion
In summary, 0MQ C++ provides a powerful toolkit for building fast and reliable messaging systems. By utilizing its various features, you can create scalable applications that meet the demands of modern software environments.
As you embark on your journey with ZeroMQ, remember to interact with the community, leverage resources, and experiment with different messaging patterns. The possibilities are endless, and mastering ZeroMQ will certainly enhance your capabilities as a C++ developer.