In C++, you can compute the sum of two integers using a simple function that takes the integers as parameters and returns their sum. Here's a quick example:
#include <iostream>
int sum(int a, int b) {
return a + b;
}
int main() {
std::cout << "Sum: " << sum(5, 3) << std::endl; // Outputs: Sum: 8
return 0;
}
Understanding the Basics of Addition in C++
What is Summation?
Summation is the process of adding numbers together to obtain a total. In programming, it has applications in various scenarios, such as calculating totals, performing statistical analysis, and managing numerical data. It is a fundamental operation within any arithmetic system and is essential for algorithms that compute numerical results.
Basic Syntax for Addition in C++
In C++, the addition operation is done using the `+` operator. This operator can be utilized on different data types, including integers, floating-point numbers, and even strings (when concatenating). The basic syntax is straightforward as shown in the following example:
int a = 5;
int b = 10;
int sum = a + b; // sum equals 15
In this code snippet, we declare two integer variables `a` and `b`, assign values to them, and then calculate their sum.
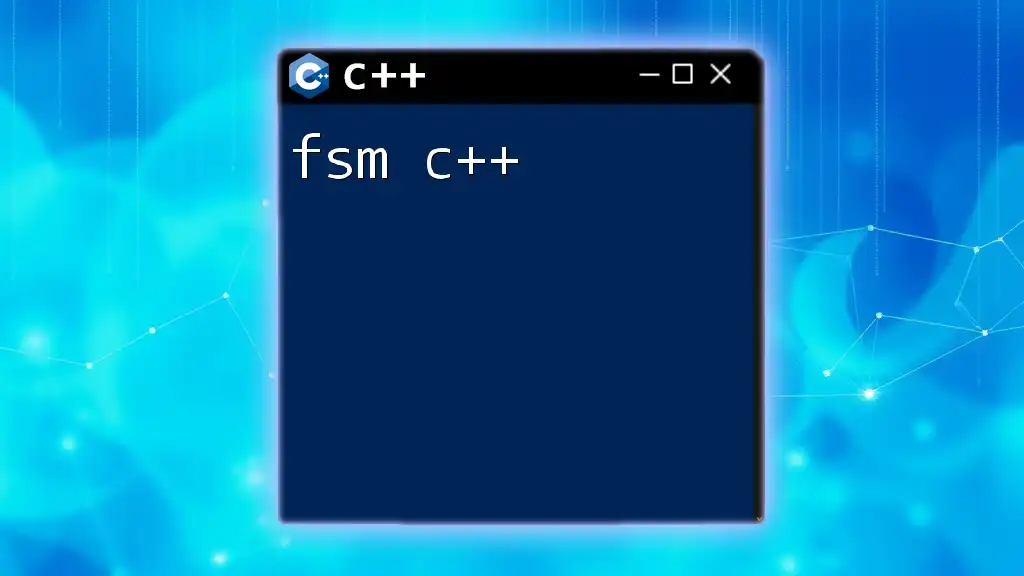
Different Ways to Compute Sum in C++
Summing Two Numbers
Summation in C++ can be performed with just two variables, making it a simple and common task. Here's how you can sum two numbers stored in variables:
int a = 12;
int b = 8;
int result = a + b; // result will be 20
In this snippet, we define two integers, `a` and `b`, and calculate the `result` using the addition operator. This forms the basis of summation in C++.
Summing an Array of Numbers
C++ uses arrays to store collections of data, which makes it easy to perform operations like summation on multiple values. Here’s an example that demonstrates how to sum an array of integers:
int arr[] = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < 5; i++) {
sum += arr[i]; // summing elements of the array
}
In this code, we initialize an array `arr` with five integer elements and use a `for` loop to iterate through each element, adding its value to the `sum` variable. This method showcases how to perform summation efficiently over a collection of numbers.
Using Functions to Calculate Sum
As your program grows in complexity, it becomes more efficient to use functions. By defining a function specifically for summation, you enhance code reusability. Here’s how to create a simple function that sums two integers:
int sum(int x, int y) {
return x + y; // function that returns sum of two integers
}
You can call this `sum` function elsewhere in your code, passing different integer values as arguments, which streamlines the addition process without repeating code multiple times.
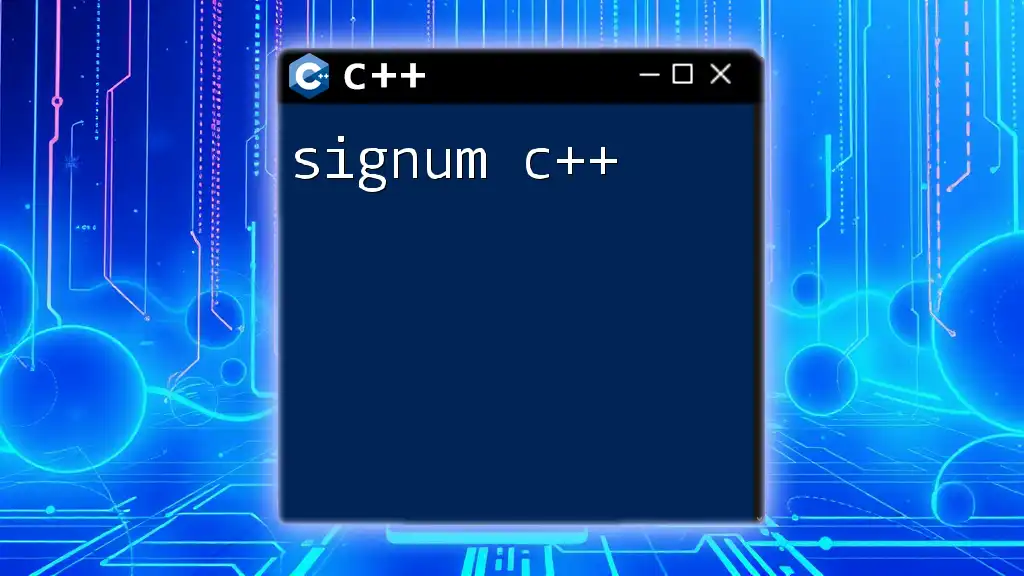
Advanced Summation Techniques
Summing with Loops
Loops are a powerful feature in C++ allowing for repetitive operations. You can sum numbers using different types of loops. Here’s an example using a `for` loop to sum the first ten natural numbers:
int total = 0;
for (int i = 1; i <= 10; i++) {
total += i; // summing numbers from 1 to 10
}
This code initializes a counter `i` and adds it to the `total` during each iteration until it reaches 10, illustrating how loops can simplify repetitive summation tasks.
Recursion for Summation
Recursion is a technique where a function calls itself to solve a problem. It can be particularly useful for summation. Here’s an example illustrating recursive summation:
int recursive_sum(int n) {
if (n == 0) return 0;
return n + recursive_sum(n - 1); // recursive call
}
In this function, `recursive_sum`, we check if `n` is zero. If it is, we return zero, thereby terminating the recursion. Otherwise, we return `n` added to the result of `recursive_sum(n - 1)`, allowing us to build the sum as we unwind the recursive calls.
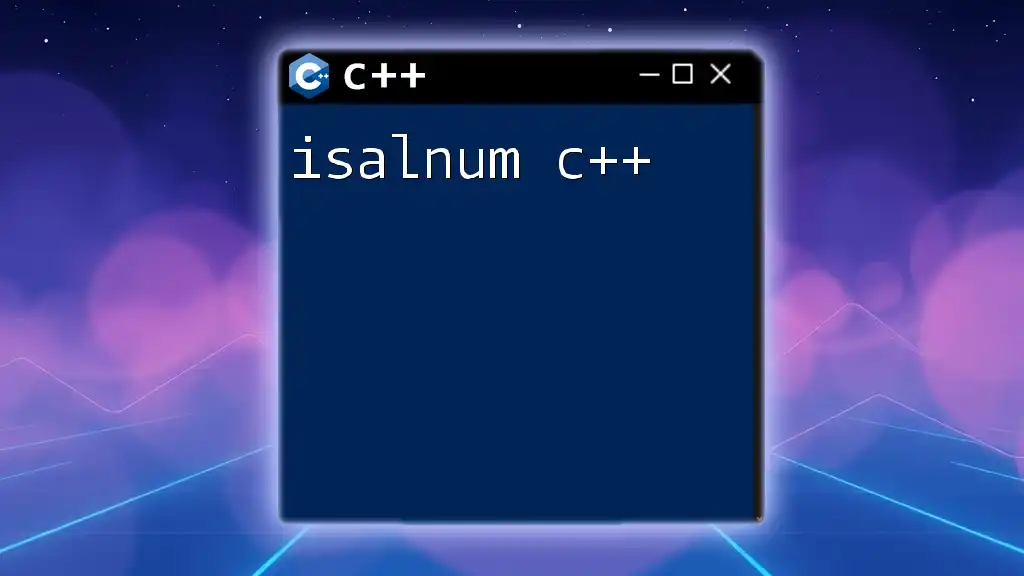
Practical Applications of Summation in C++
Summing User Input
User interaction is vital in many applications, and summing user input can illustrate dynamic processing. Here’s an example where the program reads integers from user input and calculates their total:
#include <iostream>
using namespace std;
int main() {
int n, sum = 0;
cout << "Enter the number of elements: ";
cin >> n;
for (int i = 0; i < n; i++) {
int num;
cout << "Enter number " << (i + 1) << ": ";
cin >> num;
sum += num;
}
cout << "The sum is: " << sum << endl;
}
In this program, we prompt the user to enter a count of numbers they wish to sum. We then read each subsequent number into `num` and add it to `sum`. Finally, we display the total. This showcases not only summation but also how input can affect program behavior.
Real-world Use Cases of Summation
Summation has extensive applications in real-world scenarios, such as data analysis, accounting practices, and game development. For instance:
- Data Analysis: Summation allows analysts to compute totals, averages, and other statistics essential for decision-making.
- Financial Transactions: Financial software leverages summation to aggregate values across ledgers, ensuring accuracy in reporting.
- Game Development: In gaming, summation is crucial for calculating scores, managing resources, and determining statistics that influence gameplay.
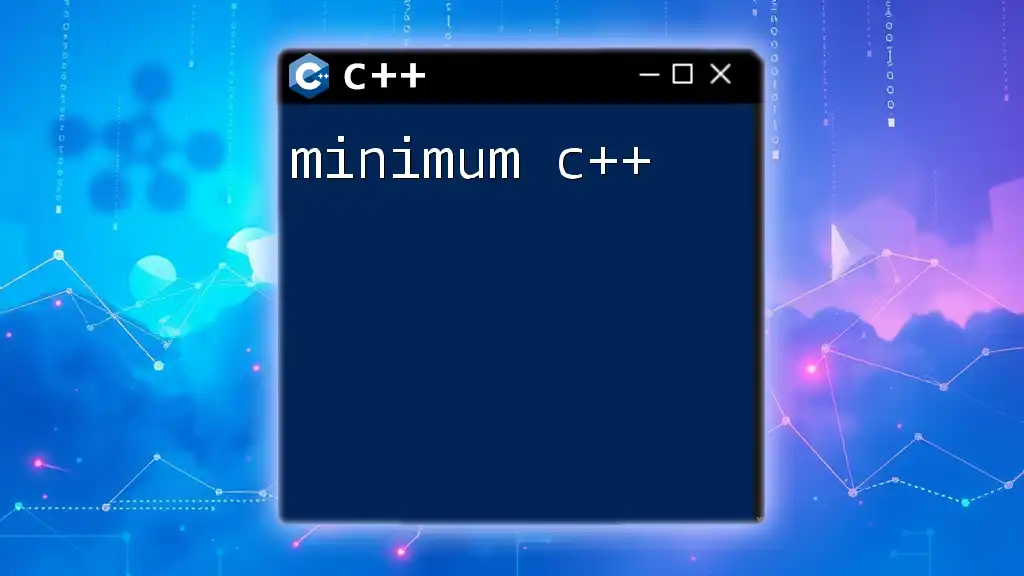
Conclusion
In this guide, we explored the various aspects of computing sums in C++, from basic addition to advanced techniques like recursion and user input handling. The versatility of summation in programming highlights its importance in numerous applications. Practicing these concepts through examples will further deepen your understanding, paving the way for more complex programming challenges.
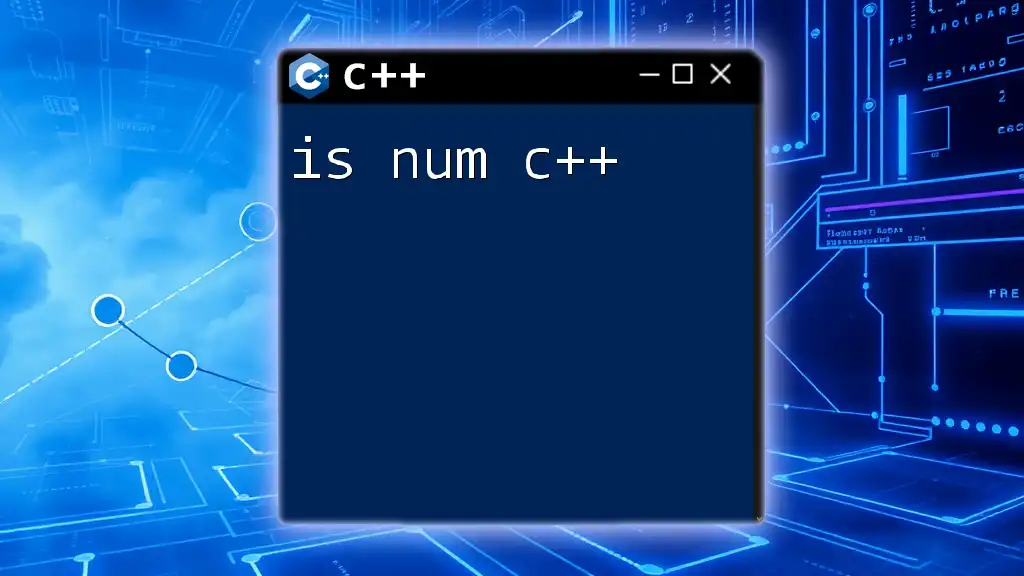
Additional Resources
To continue your learning journey, consider exploring C++ documentation, enrolling in online courses, or participating in coding challenges. Engaging with community forums can also provide additional insights and practical experiences.