In C++, the phrase "is num" typically refers to checking whether a variable is a number, which can be done using type checks or other validation methods, as shown in the following example:
#include <iostream>
#include <type_traits>
template<typename T>
bool is_num(T value) {
return std::is_arithmetic<T>::value;
}
int main() {
std::cout << std::boolalpha << is_num(42) << std::endl; // Output: true
std::cout << std::boolalpha << is_num("Hello") << std::endl; // Output: false
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful, versatile programming language created by Bjarne Stroustrup in the early 1980s. It is an extension of the C programming language and introduces features like object-oriented programming (OOP). C++ has gained widespread popularity for its performance, efficiency, and flexibility, making it a staple in software development across various domains, including systems programming, game development, and application software.
Overview of Data Types in C++
Data types in C++ define the types of data that can be stored and manipulated within a program. They are categorized into two primary types:
- Fundamental Data Types: These include basic types like `int`, `char`, `float`, and `double`. Each type offers different ranges and precision, allowing programmers to choose the most appropriate type for their needs.
- Complex Data Types: These include user-defined types like `structs`, `classes`, and `enums`, which enable the creation of more complex data structures tailored to specific requirements.

What Does "Num" Refer to in C++?
Numeric Data Types
In C++, numeric data types are essential for performing calculations and manipulating numerical values. They can mainly be divided into two categories:
- Integer Types: This includes `int`, `short`, `long`, and `long long`, which are used to represent whole numbers, both positive and negative.
- Floating-Point Types: This includes `float` and `double`, which allow for the representation of real numbers, incorporating fractions for more precise calculations.
Is "Num" a Standard C++ Type?
The term "num" is not recognized as a built-in type in C++. However, it can informally refer to numeric values or variables used throughout the code. Developers often use abbreviations or shorthand in naming variables, but there’s no predefined standard type called "num".
Common Practices and Conventions
In C++, developers typically follow a naming convention that helps identify the purpose of a variable. For example, using names like `numValues`, `totalSum`, or `scoreCount` can convey that the variable holds numeric information. Following these conventions enhances code readability and maintainability.

Working with Numeric Data in C++
Declaring Numeric Variables
Declaring numeric variables in C++ is straightforward. The syntax requires defining the type followed by the variable name. Here’s a simple example:
int age = 25;
double salary = 50000.50;
In this example, we defined an integer variable `age` and initialized it with the value 25, while `salary` is a double initialized to 50000.50.
Initializing and Assigning Values
Initialization refers to assigning a value to a variable at the time of declaration. Additionally, variables can be assigned values later in the program, such as through user input. The following code snippet demonstrates both:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
In this case, the user is prompted to provide a value, which is then stored in the `number` variable.
Performing Arithmetic Operations
Arithmetic operations in C++ can be performed using standard operators such as `+`, `-`, `*`, `/`, and `%`. Basic arithmetic can be illustrated with the following code snippet:
int a = 5, b = 10;
int sum = a + b;
std::cout << "Sum: " << sum;
Here, we declared two variables, `a` and `b`, then calculated their sum and displayed it.
Type Conversion
Type conversion is the process of converting data from one type to another. Implicit conversion occurs automatically, while explicit conversion requires manual instruction. An example of explicit conversion can be seen below:
float num = 7.4;
int intNum = (int)num; // explicit conversion
In this case, `num` is a floating-point variable, and we explicitly convert it to an integer type, resulting in `intNum` having a value of 7.
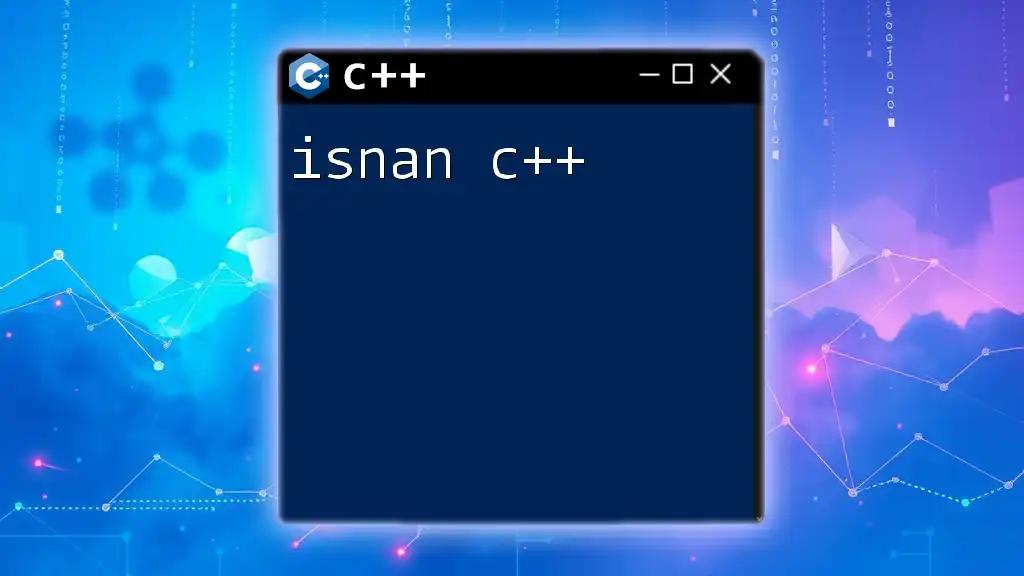
Common C++ Libraries for Numeric Operations
Using `<cmath>` for Mathematical Functions
The `<cmath>` library is invaluable for performing mathematical operations in C++. It provides various mathematical functions that can enhance the functionality of your numeric data. For example:
#include <cmath>
double squareRoot = sqrt(25); // squareRoot = 5
In this example, we use the `sqrt()` function to calculate the square root of 25.
Utilizing Standard Template Library (STL) for Numerical Algorithms
The Standard Template Library (STL) includes various algorithms and data structures that simplify many operations, especially on collections of numeric data. One particularly useful component is the `<numeric>` library, which provides a variety of algorithms. Here’s a quick look at using `std::accumulate`:
#include <numeric>
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
int total = std::accumulate(numbers.begin(), numbers.end(), 0);
In this snippet, we defined a vector of integers and calculated the sum of its elements by calling `std::accumulate()`. This is a convenient approach for processing collections of numeric values.

Conclusion
In summary, while the term "num" is not a standard type in C++, the language offers a variety of numeric data types that are integral to programming. Understanding how to declare, initialize, and manipulate numeric variables allows developers to utilize C++ effectively.

Call to Action
If you found this article helpful, consider subscribing for more C++ tutorials and insights. Dive into the examples provided, practice with the code snippets, and explore the powerful functionalities that C++ has to offer!