A Linux C++ compiler transforms C++ source code into executable programs, allowing developers to efficiently write and compile software applications on a Linux operating system.
Here's a simple example of how to compile a C++ program using the `g++` compiler:
g++ -o my_program my_program.cpp
Understanding Compiling in C++
Compiling is the critical process that transforms human-readable code into machine code that can be executed by a computer. In the context of C++, this involves translating your source code written in C++ syntax into a binary executable that a Linux operating system can understand and run. The compiler acts as a translator, ensuring that your code follows the syntactical rules of C++ and checking for any errors before generating the executable output.
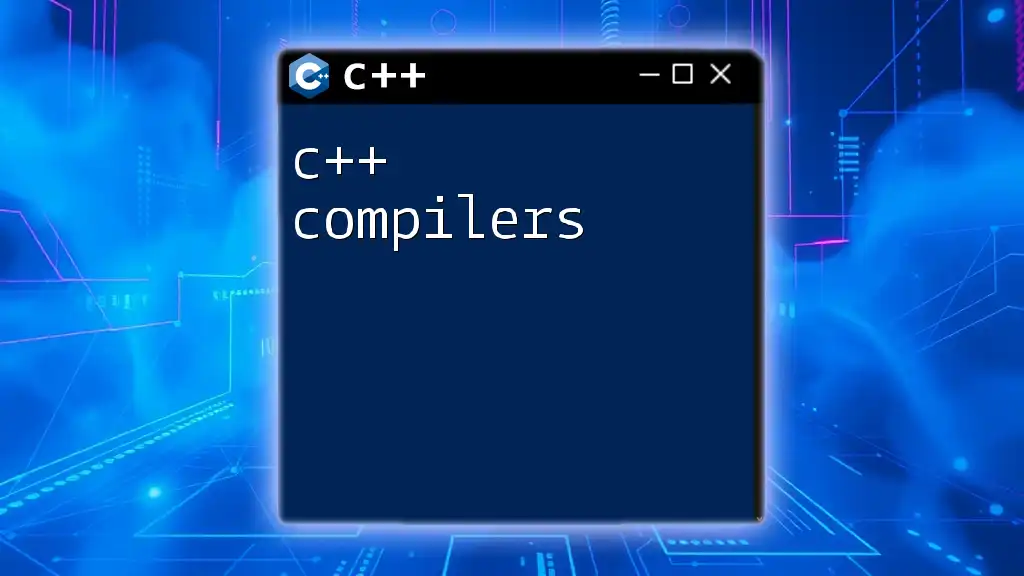
Overview of Linux Compilers
When working with a Linux C++ compiler, the most prominent ones you will encounter are:
-
GCC (GNU Compiler Collection): This is the most widely used compiler in the Linux environment. It supports various programming languages, including C and C++.
-
Clang: Known for its fast compilation and excellent diagnostic messages, Clang is a highly optimized compiler that many developers prefer for performance and clarity.
-
Others: There are additional compilers, like the Intel C++ Compiler, which are designed for specific environments and performance optimizations.
Choosing the right compiler can impact your development workflow, so it’s essential to understand the pros and cons of each.
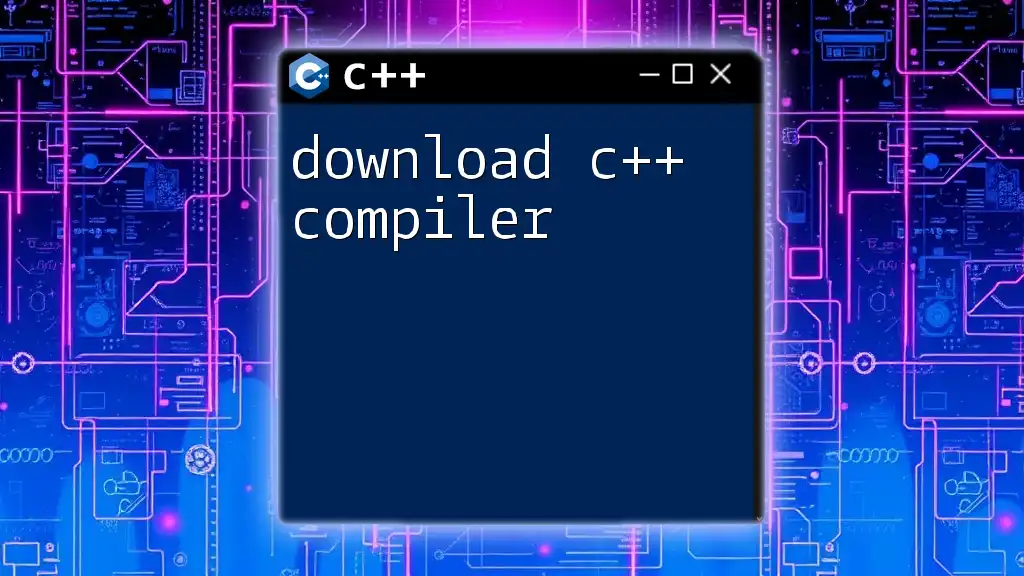
Setting Up Your Development Environment
Installing Essential Tools
To start coding and compiling C++ programs, you need to install a compiler on your Linux machine. Below are steps to install the two most popular compilers:
Installing GCC: You can easily install GCC using your package manager. Run the following command in the terminal:
sudo apt install g++
Installing Clang: If you prefer Clang for its speed and powerful features, you can install it with:
sudo apt install clang
Verifying the Installation: Once your compiler is installed, ensure it’s set up correctly by checking its version:
For GCC:
g++ --version
For Clang:
clang --version
Choosing the Right Editor/IDE
The text editor or Integrated Development Environment (IDE) you choose can significantly affect your productivity. Here are some recommended options:
- Visual Studio Code: A versatile editor with vast extension support and powerful debugging capabilities.
- Code::Blocks: An open-source, customizable IDE specifically for C/C++ programming.
- Eclipse: A versatile IDE that supports various programming languages, including C++.
Each of these tools helps streamline your development process through features like syntax highlighting, debugging, and project management.
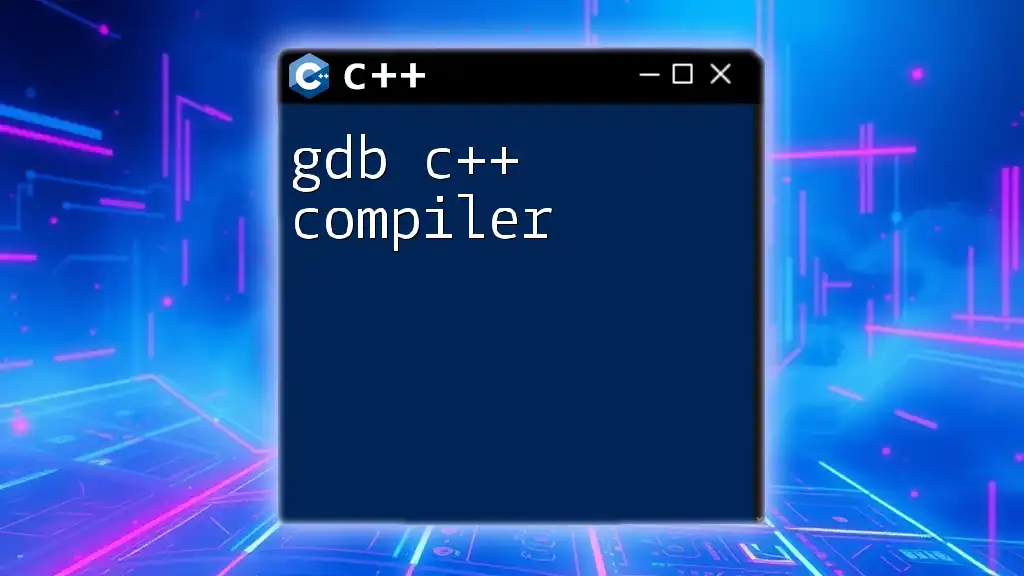
Compiling C++ Programs in Linux
Writing Your First C++ Program
Creating a simple "Hello World" program is a great way to dive into C++. Here’s a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Save this code in a file named `hello.cpp`.
Compiling Your Program
With your program written, it's time to compile it. Using GCC, you can compile your C++ file with the following command:
g++ hello.cpp -o hello
In this command:
- `g++` invokes the GNU C++ compiler.
- `hello.cpp` is the source file you want to compile.
- `-o hello` tells the compiler to output the compiled program as `hello`.
Running Your Compiled Program
After successfully compiling your program, you can run it by executing:
./hello
This command runs the `hello` executable you just created, and if everything is configured correctly, you should see “Hello, World!” printed in your terminal.
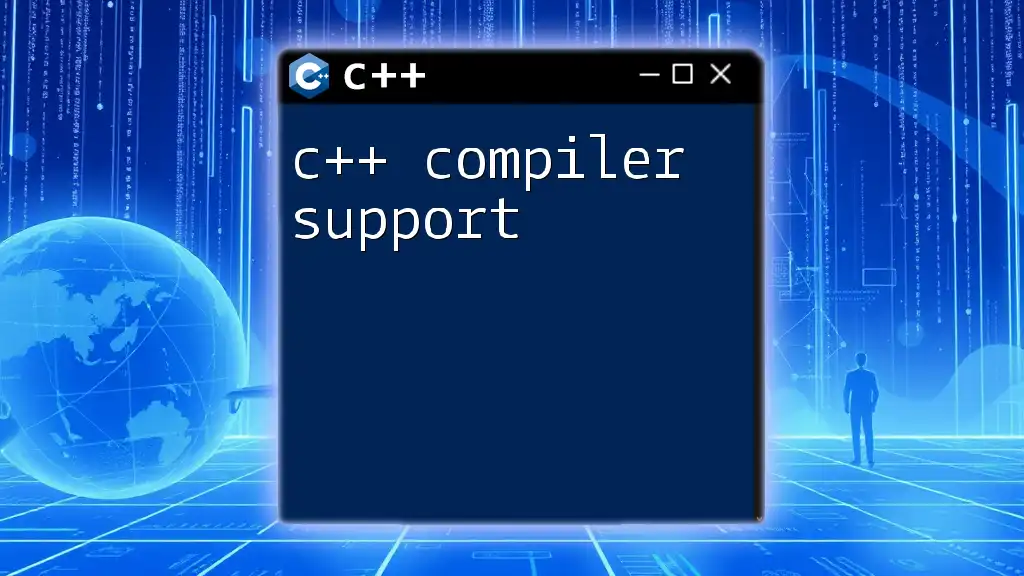
Advanced Compilation Techniques
Using Compilation Flags
C++ compilers come equipped with various flags that customize the compilation process. Here are some commonly used flags:
- `-Wall`: Enables all compiler’s warning messages.
- `-O2`: Optimizes the code for speed.
- `-g`: Includes debug information in the executable.
You can combine these flags while compiling your program to enhance your build process. For example:
g++ -Wall -O2 hello.cpp -o hello
This command will generate a more efficient executable while also providing warnings about potential issues in your code.
Working with Multiple Source Files
For larger projects, you may have multiple C++ files that need to be compiled together. Suppose you have a `main.cpp` file and a `module.cpp` file. You can compile both like so:
g++ main.cpp module.cpp -o my_program
This command compiles both files into a single executable named `my_program`.
Creating Makefiles for Efficient Compilation
When working on bigger projects, using Makefiles can help you manage your build process more efficiently. A Makefile outlines the dependencies and commands required for building your program. Here’s a simple example:
all: my_program
my_program: main.o module.o
g++ -o my_program main.o module.o
%.o: %.cpp
g++ -c $<
To use this Makefile, save it in the same directory as your source files and simply run:
make
This command will compile your project according to the specifications in the Makefile, simplifying the process, especially as the number of source files increases.
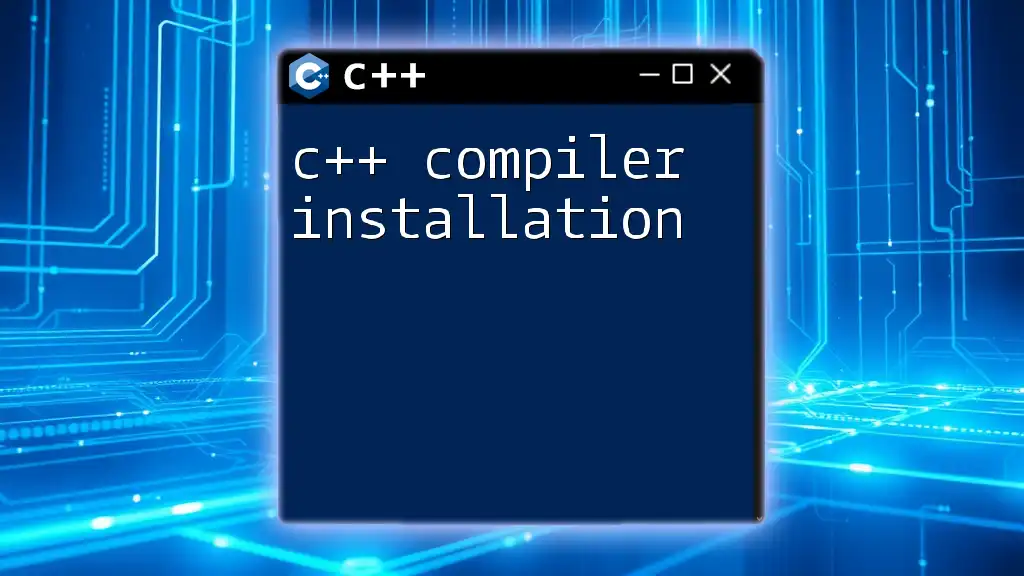
Troubleshooting Common Compilation Errors
As you write more C++ code, you’ll encounter errors during the compilation process. Some common errors include:
- Syntax Errors: Errors in your code due to incorrect syntax (e.g., missing semicolons, mismatched parentheses).
- Linker Errors: Occur when the compiler cannot find the implementation of a function or variable.
Reading and interpreting compiler error messages is crucial for debugging. Pay attention to the line numbers and descriptions provided by the compiler to pinpoint the issue.
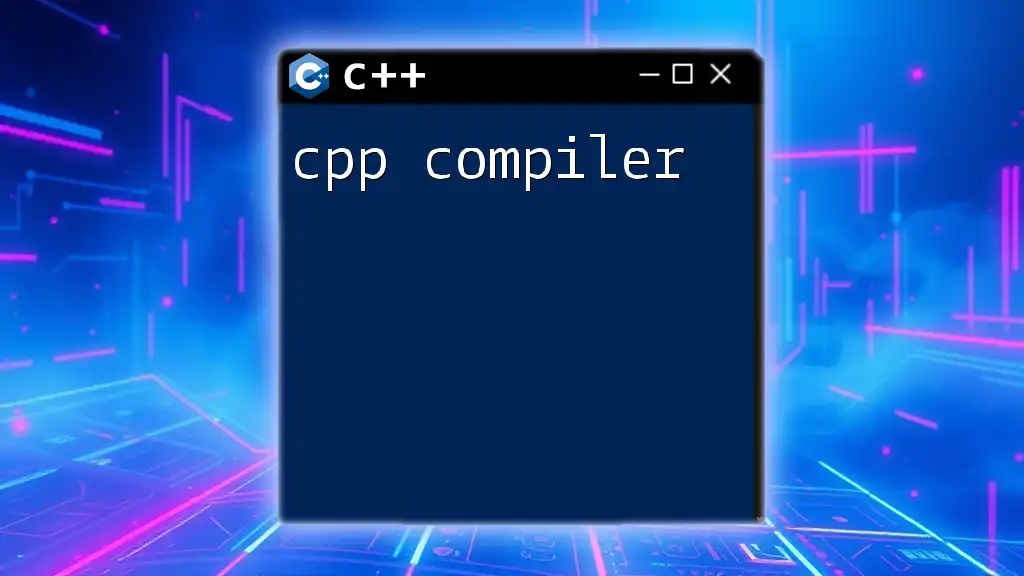
Best Practices for C++ Development in Linux
To create clean, efficient, and maintainable code, consider the following best practices:
- Consistent Coding Standards: Adhere to a set of coding conventions to enhance readability and maintainability.
- Version Control: Use systems like Git to manage code changes and collaborate effectively with others.
- Regular Review: Frequently reviewing your code helps catch potential issues early and encourages improvements.
By following these practices, you can develop high-quality C++ applications on Linux.
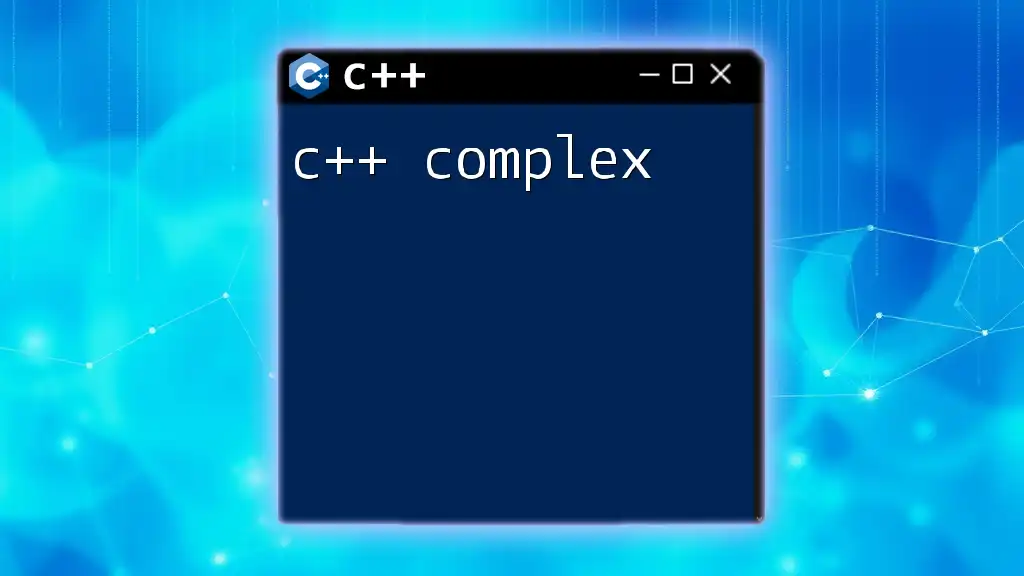
Additional Resources
For further exploration, consider checking out the official documentation for GCC and Clang, which provides in-depth information on all the features and options. Online platforms offer a wealth of tutorials tailored to various skill levels, and community forums can be invaluable for troubleshooting and guidance.
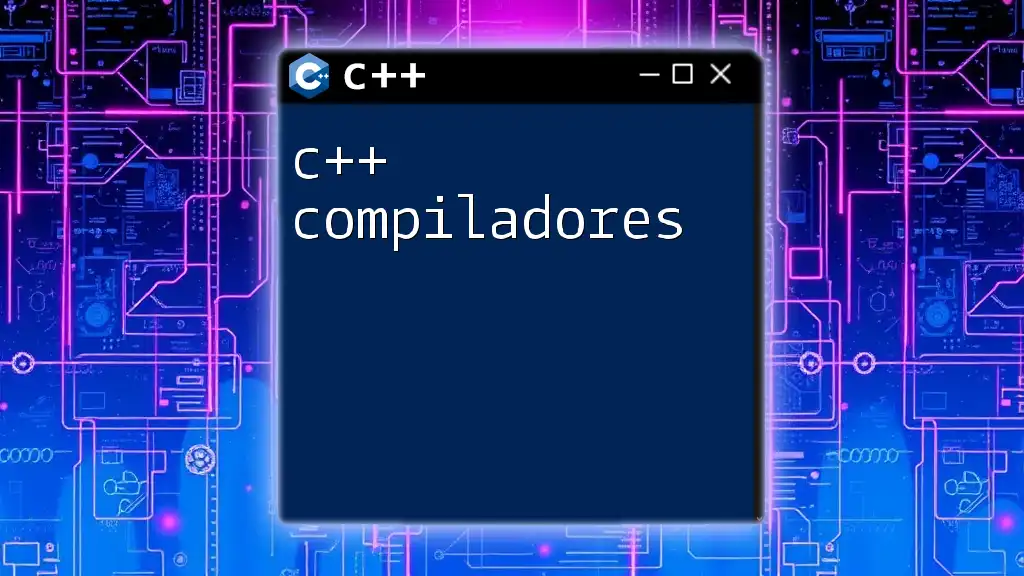
Conclusion
By grasping the fundamentals of using a Linux C++ compiler and following best practices in your development processes, you’ll be well on your way to becoming proficient in C++. The skills you develop will empower you to tackle more complex projects and enhance your programming journey.
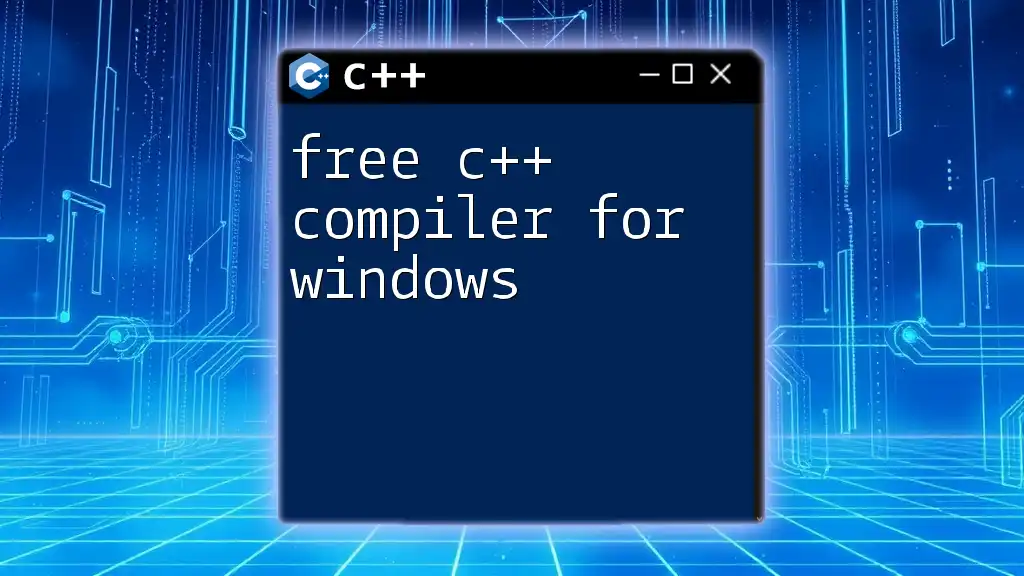
Call to Action
Stay tuned for more insightful posts and consider joining a course focused on mastering C++ commands and development in Linux. Expand your knowledge and elevate your coding skills to the next level!