The C++ Intel Compiler is a high-performance compiler that optimizes C++ code for Intel architectures, providing advanced optimizations and powerful debugging features.
Here is a simple code snippet demonstrating how to compile a C++ program using the Intel Compiler from the command line:
icpc -o hello hello.cpp
What is the Intel C++ Compiler?
The Intel C++ Compiler is a powerful tool designed to convert C++ source code into machine code, enabling it to run on various hardware architectures. This compiler stands out not only for its performance-enhancing capabilities but also for its support for numerous advanced C++ features that many developers find beneficial for high-performance applications.
Key Benefits
Using the Intel C++ Compiler can lead to significant performance optimization due to its ability to generate highly efficient code. It is designed to take full advantage of modern Intel architectures, optimizing code for parallel execution and advanced CPU features. Moreover, it fully supports advanced C++ features, allowing developers to utilize modern programming paradigms effectively. Compatibility across different platforms adds to its versatility, making it a goto tool for many C++ developers.
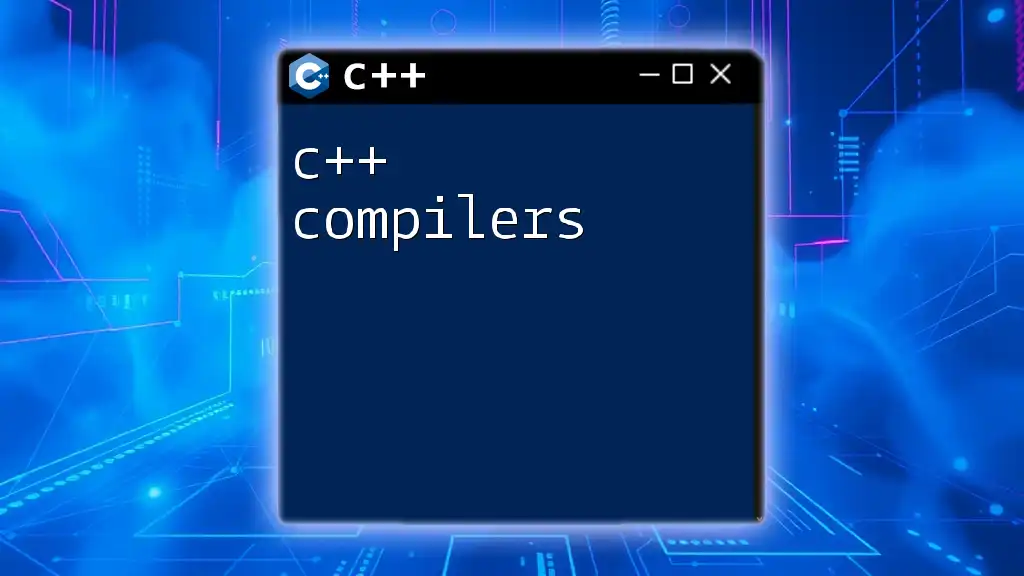
Installation and Setup
System Requirements
Before installing the Intel C++ Compiler, ensure that your system meets the following requirements:
- Hardware: Intel processors (or compatible architectures) recommended for best performance.
- Software: A compatible operating system, including Windows, Linux, or macOS versions. Ensure your OS is up-to-date for optimal functionality.
Installation Guide
To install the Intel C++ Compiler, follow these steps:
-
Download the Compiler: Visit the official Intel website and navigate to the Intel C++ Compiler section to download the appropriate version for your operating system.
-
Run the Installer: Execute the installer after download. For Windows users, this typically involves double-clicking the downloaded file and following on-screen prompts.
-
Configuration with IDE: After installation, configure your Integrated Development Environment (IDE) of choice (e.g., Visual Studio, Eclipse) to work with the Intel C++ Compiler, ensuring that paths to the compiler’s binaries are correctly set.
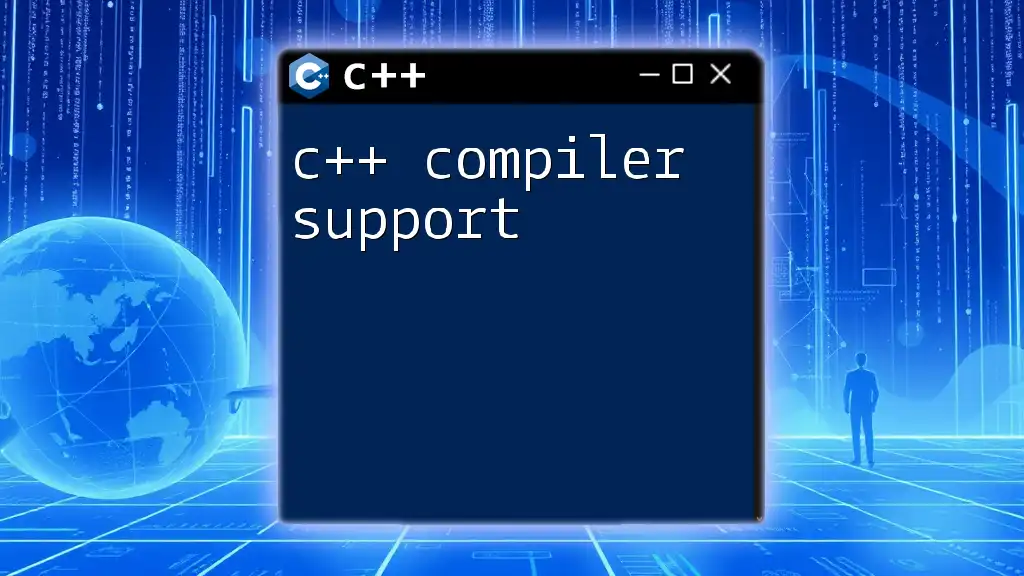
Basic Features of Intel C++ Compiler
Optimization Options
The Intel C++ Compiler provides various optimization levels that can significantly enhance the performance of your code. The common optimization flags include:
- `-O1`: Basic optimization, focusing on reducing code size and improving execution speed.
- `-O2`: Further optimizes the code, balancing performance and compilation speed.
- `-O3`: Enables aggressive optimization techniques, which can result in substantial performance improvements but may increase compilation time.
- `-Ofast`: Disables strict standards compliance for increased speed.
Here’s a simple example demonstrating the difference in performance using optimizations:
#include <iostream>
#include <cmath>
int main() {
double result = 0;
for (int i = 0; i < 1e6; i++) {
result += std::sqrt(i);
}
std::cout << "Result: " << result << std::endl;
return 0;
}
Compile this with `-O3` and compare it to compilation with no optimization. You should observe a substantial difference in runtime.
Diagnostic and Warning Features
The Intel C++ Compiler includes robust diagnostic tools and warning features which assist in identifying potential issues within your code. To enable verbose warning messages, you can use the `-Wall` flag during compilation. Here’s a snippet that generates a warning:
#include <iostream>
int main() {
int x;
std::cout << x << std::endl; // Warning: 'x' may be uninitialized
return 0;
}
The compiler will alert you that variable `x` might be used without being initialized, prompting necessary code adjustments.
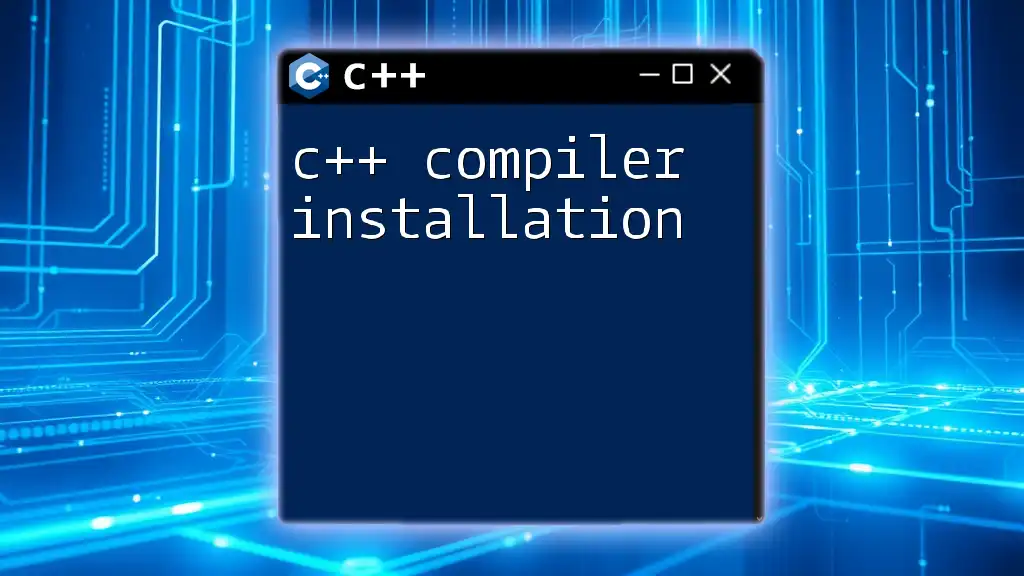
Advanced Features
Intel C++ Compiler Extensions
The Intel C++ Compiler supports several extensions that enhance C++ programming. One notable feature is intrinsics, which allow developers to access processor-specific instructions directly for performance-critical tasks.
Here’s a simple code snippet demonstrating the use of Intel intrinsics for vectorization:
#include <immintrin.h>
void add_arrays(const float* a, const float* b, float* result, size_t size) {
for (size_t i = 0; i < size; i += 8) {
__m256 vec_a = _mm256_loadu_ps(&a[i]);
__m256 vec_b = _mm256_loadu_ps(&b[i]);
__m256 vec_result = _mm256_add_ps(vec_a, vec_b);
_mm256_storeu_ps(&result[i], vec_result);
}
}
In this example, the intrinsic `_mm256_add_ps` allows for the addition of eight floats simultaneously, utilizing SIMD (Single Instruction, Multiple Data) capabilities.
Analyzing Performance
The Intel C++ Compiler comes equipped with performance analysis tools such as the Intel VTune Profiler. This tool helps identify bottlenecks in your application and provides insights into where performance can be improved. You can gather performance metrics, analyze thread activity, and understand memory usage to optimize your applications effectively.
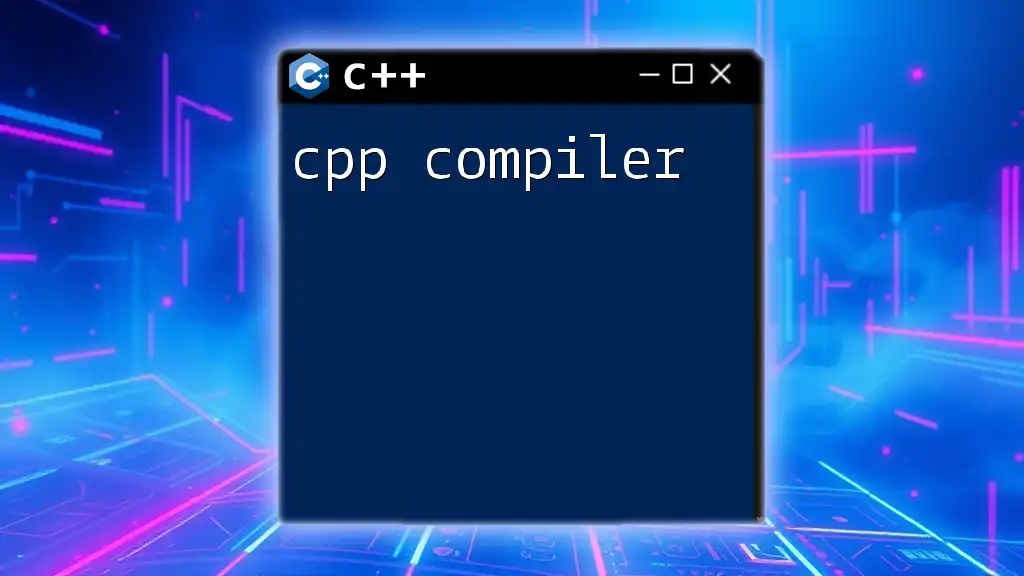
Developing with Intel C++ Compiler
Compiling a Simple Program
Compiling your first program is straightforward. Let’s create a simple "Hello World" application.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this code using the Intel C++ Compiler, save it as `hello.cpp` and run the following command:
icpc hello.cpp -o hello
Executing `./hello` (on Linux or macOS) or `hello.exe` (on Windows) will display the message.
Debugging Support
Debugging is a critical aspect of software development. The Intel C++ Compiler provides built-in support for debugging, allowing developers to step through code, inspect variables, and analyze program flow. You can compile your code with the `-g` flag to include debug information:
icpc -g hello.cpp -o hello
This ensures that your debugging tool has access to the necessary information for effective debugging.
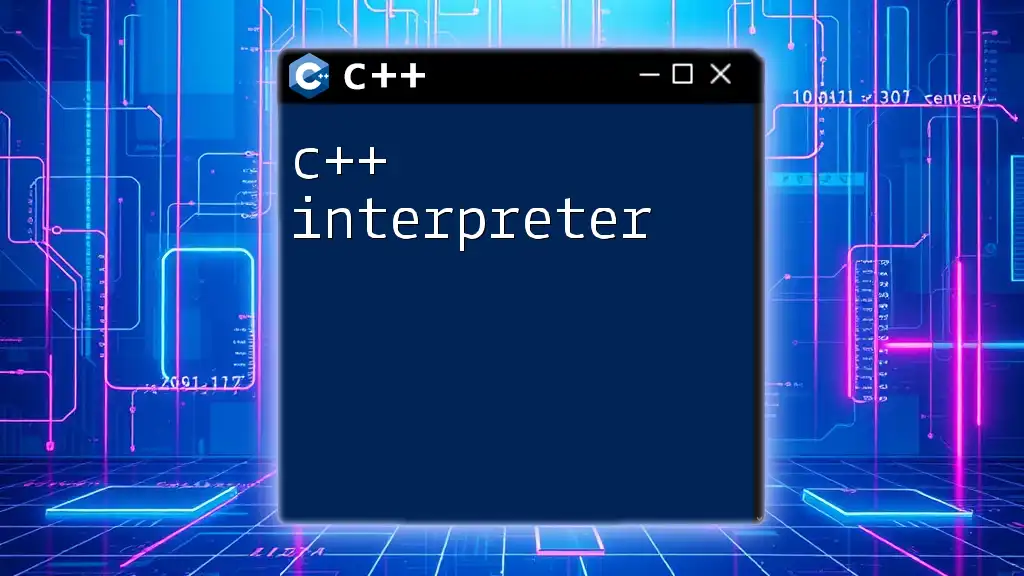
Best Practices for C++ Development with Intel Compiler
Writing Efficient Code
To take full advantage of the Intel C++ Compiler, consider the following best practices:
- Utilize Compiler Optimizations: Always compile with appropriate optimization flags for release builds. Profile your application to determine the best optimization level.
- Write Clean Code: Ensuring code readability and maintainability contributes to better performance and easier debugging.
- Leverage Multithreading: Use Intel’s threading libraries such as TBB (Threading Building Blocks) for efficient parallel programming.
Keeping Up with Compiler Updates
Compiler capabilities evolve rapidly. Regularly updating your Intel C++ Compiler ensures that you leverage the latest optimizations and features. Be proactive in checking the release notes for new enhancements and performance fixes.
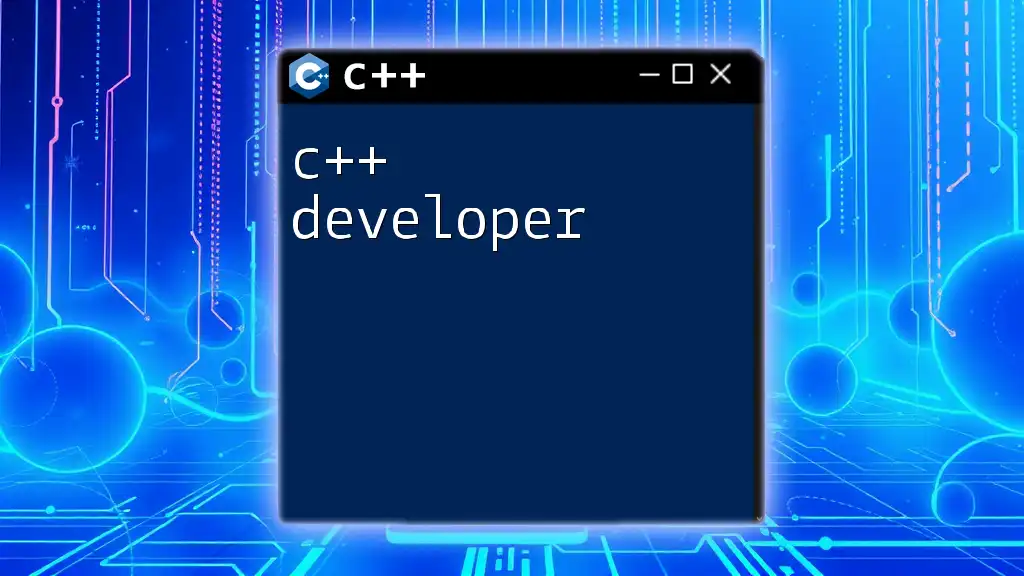
Common Issues and Troubleshooting
Compilation Errors
While working with the Intel C++ Compiler, you may encounter various compilation errors. Familiarize yourself with common messages such as "undefined reference" or "file not found." Many of these can be resolved by ensuring all relevant source files are included in the compilation command or verifying correct paths.
Performance Issues
If your application exhibits performance issues, utilize the Intel performance analysis tools to delve into your application's execution. Look for common bottlenecks, such as CPU resource contention, memory access patterns, and unnecessary context switching. The insights gained will guide optimizations to boost performance effectively.
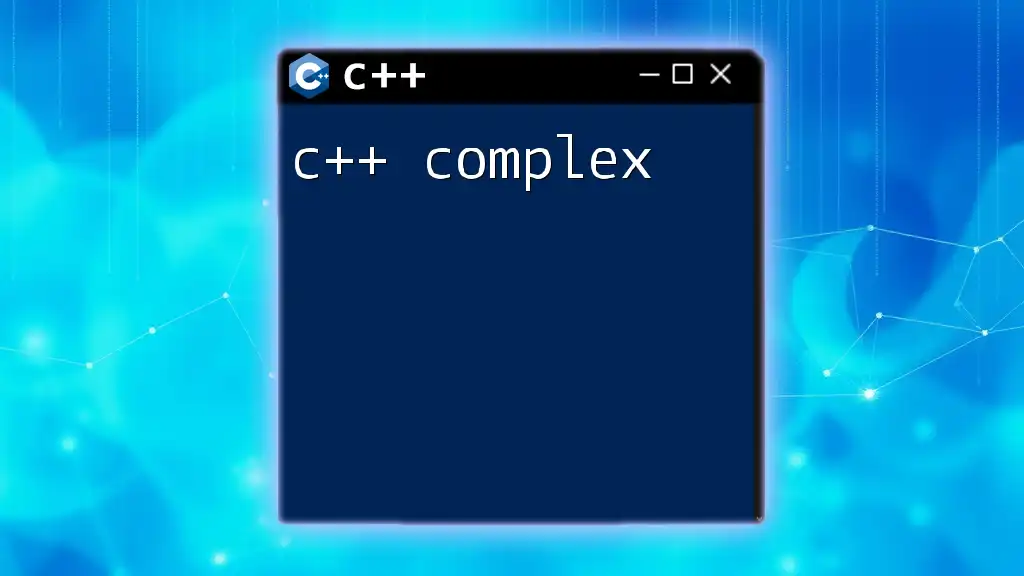
Conclusion
The C++ Intel Compiler offers a compelling package for developers seeking to write high-performance C++ applications. With its unique optimizations, extensive support for modern C++ features, and advanced profiling tools, programmers can significantly enhance their workflows. Embrace the power of the Intel C++ Compiler, experiment with its features, and continue learning from the vast resources available to elevate your programming craft.