C++ is primarily a compiled language, meaning that its source code is transformed into machine code by a compiler before execution, rather than being interpreted line by line at runtime.
Here’s a simple C++ example demonstrating a basic program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What It Means to Compile and Interpret
Definition of Compilation
Compilation is the process of translating high-level source code into machine code or bytecode that a computer’s processor can execute directly. This transforms human-readable code into a format that is understandable by machines.
Common examples of compiled languages include C, Rust, and, of course, C++. When you write a C++ program, the code must be transformed into a binary executable file before it can be run. This transformation occurs through several stages, providing multiple opportunities for error checking and optimization.
Definition of Interpretation
In contrast, interpretation involves executing code line-by-line or statement-by-statement. An interpreter reads source code and executes it directly rather than compiling it into a standalone binary first. Languages that are purely interpreted include Python and JavaScript, where the interpreter allows you to run programs without the overhead of a separate compilation step.
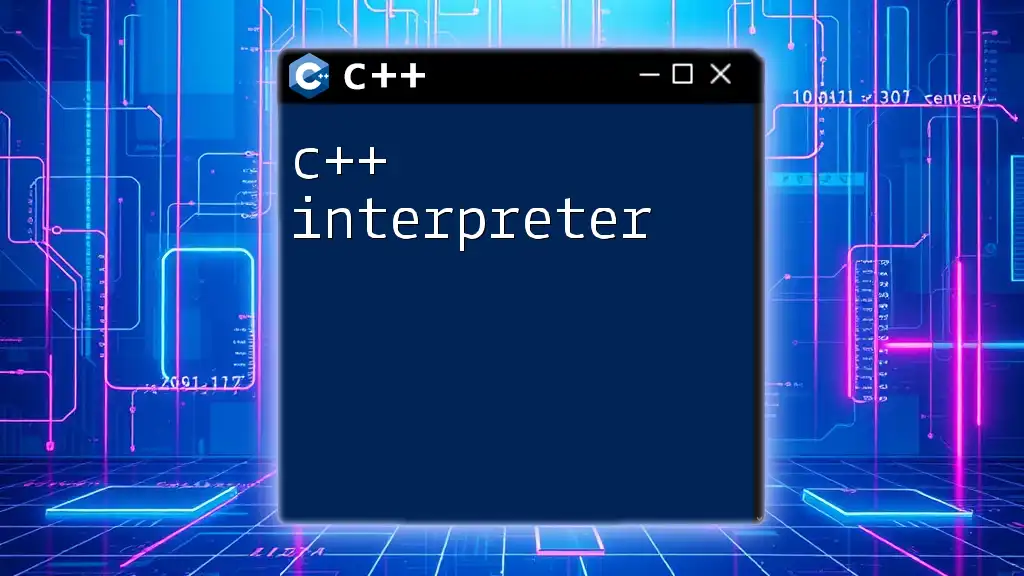
C++: A Compiled Language
How C++ Compilation Works
The process of compiling C++ code is intricate and comprises several key stages:
-
Preprocessing: This initial stage involves handling directives (like `#include` and `#define`) to prepare the source code for compilation.
-
Compilation: Here, the preprocessed code is translated into assembly language, optimized for performance.
-
Linking: Finally, the compiler links this assembly code with libraries and other object files to create a complete executable.
To illustrate the concept, consider this simple C++ program:
#include <iostream>
#define PI 3.14
int main() {
std::cout << "The value of PI is " << PI << std::endl;
return 0;
}
In the preprocessing stage, the `#include` directive pulls in the iostream library, while the `#define` creates a constant named `PI`. This makes the final compiled output efficient and optimized for execution.
Compilation Tools
Several tools assist in the compilation of C++ programs:
- GCC (GNU Compiler Collection): A powerful and widely-used compiler supporting multiple languages.
- Clang: Known for its fast compilation and advanced features like static analysis.
- Microsoft Visual C++: A popular tool for Windows development, offering an integrated development environment with robust debugging capabilities.
Benefits of Compiling C++
The act of compiling C++ code comes with several advantages:
-
Performance: Compiled code generally results in faster execution speeds when compared to interpreted code. This is due to the extra processing performed during the compilation phase to optimize the code for the target architecture. For example, a benchmark test showing the execution time for calculating the Fibonacci sequence will typically favor compiled languages over interpreted ones significantly.
-
Type Checking: C++ is statically typed, meaning that errors related to type mismatches can be detected during the compilation phase. This feature is beneficial because it reduces runtime errors and enhances reliability.
Drawbacks of Compilation
However, there are also drawbacks associated with compiling C++ code. The most notable include:
-
Longer Development Times: Each time you make changes to the code, you must recompile the entire program before you can evaluate the results. This can slow down the iterative development process.
-
Platform Dependence: Without additional tools (like Docker), compiled C++ code may not be as portable across platforms compared to interpreted languages. This can lead to complications when trying to run the same code on different operating systems.
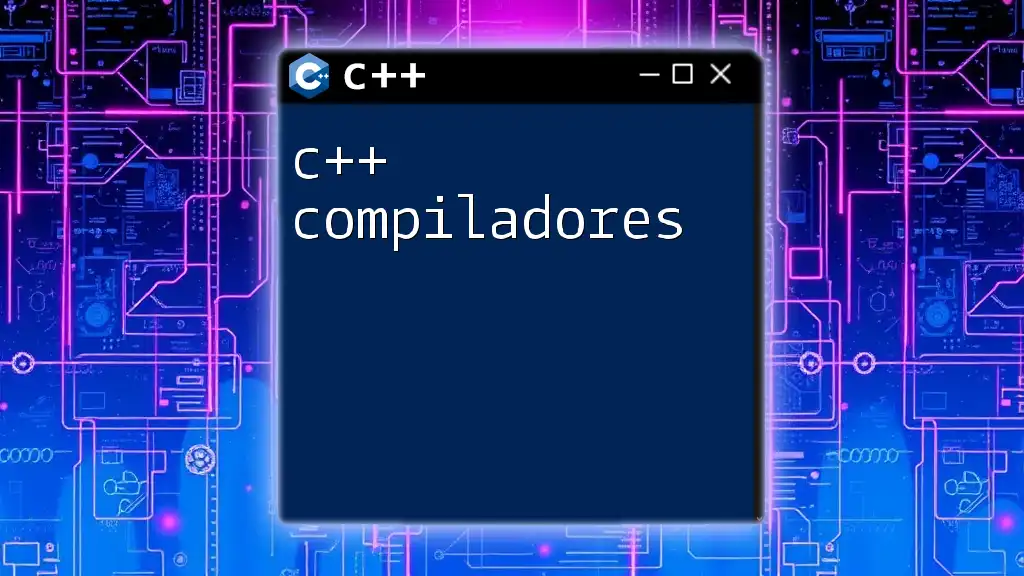
The Role of Interpreted Code in C++
Just-In-Time (JIT) Compilation
While C++ is predominantly a compiled language, there are methods to incorporate interpretation through Just-In-Time (JIT) compilation. JIT combines the advantages of both compilation and interpretation by compiling code at runtime—meaning it translates code into machine language only when it is needed.
Languages such as Java use JIT compilation, allowing for optimized performance while maintaining dynamic features. In C++, there are libraries like LLVM that facilitate a JIT compilation approach, allowing developers to leverage runtime code generation.
Example of JIT in C++
For instance, using LLVM, you could write a simple function that performs calculations at runtime:
#include <llvm/ExecutionEngine/ExecutionEngine.h>
#include <llvm/IR/IRBuilder.h>
// Include other necessary LLVM headers
// Note: This snippet is illustrative; real implementation involves more setup.
Although more complex, the use of JIT in C++ could potentially improve performance for certain applications, especially in scenarios involving runtime-generated code.
Hybrid Approaches and Performance
Hybrid approaches that blend compiled and interpreted paradigms can unlock unique opportunities for enhanced performance. By utilizing JIT compilation, developers can create responsive applications that adapt to varying user inputs and run efficiently in real-time. This aspect is crucial in modern computing environments, where responsiveness and user experience are paramount.
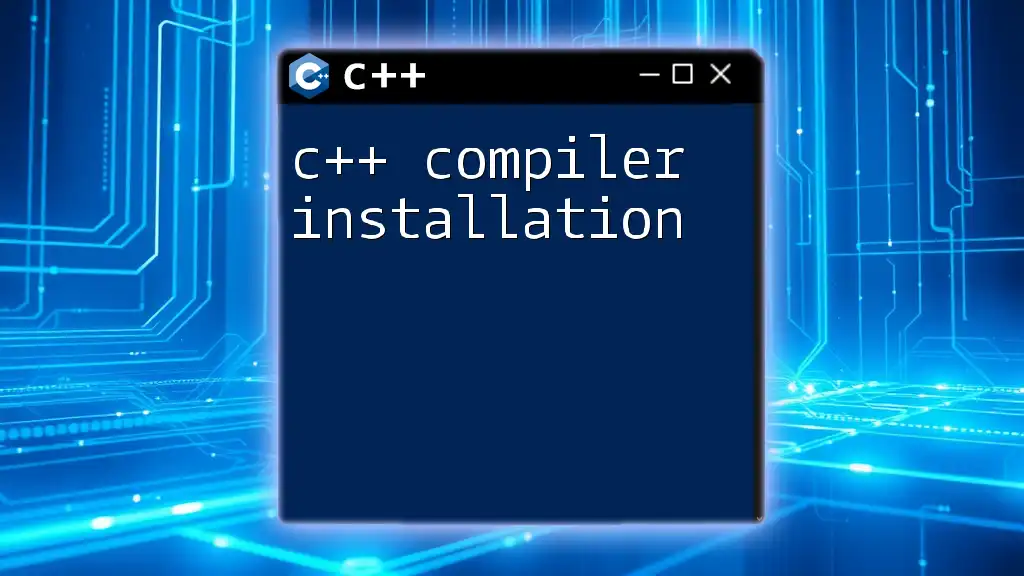
Comparing Compiled vs Interpreted Languages
Performance Comparison
Performance is often the primary differentiator between compiled and interpreted languages. Compiled languages like C++ generally outperform interpreted languages in execution speed due to the optimized machine code they generate. For example, consider running a sorting algorithm. A C++ implementation compiles to efficient binaries, while an interpreted language executes each line of code sequentially, resulting in slower performance.
Development Cycle Comparison
The development process also varies significantly between the two paradigms. In a compiled language like C++, debugging and testing require running the compilation step each time a change is made. In contrast, interpreted languages typically allow for immediate feedback, as changes can be tested in real time without the overhead of compilation.
To summarize, a brief table could capture the essence of both languages:
Feature | Compiled Language (C++) | Interpreted Language (Python) |
---|---|---|
Execution Speed | Fast | Slower |
Development Cycle | Longer (compilation needed) | Shorter (immediate feedback) |
Error Detection | Early (compile time) | Later (run time) |
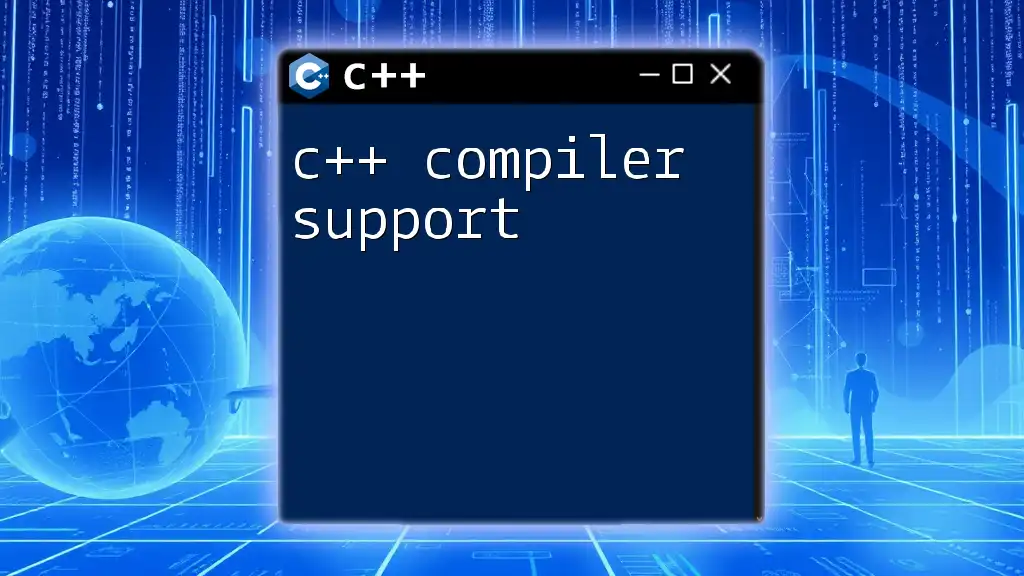
Conclusion
In conclusion, C++ is compiled, and understanding this fundamental aspect of the language is vital for any developer seeking efficiency and performance in their coding endeavors. Compiled languages, particularly C++, provide unparalleled speed advantages, thorough type checking, and overall robustness compared to interpreted languages.
When choosing a programming language for your projects, it's essential to weigh these characteristics of C++. For cases requiring real-time performance and tighter control over system resources, C++ shines as the ideal choice.
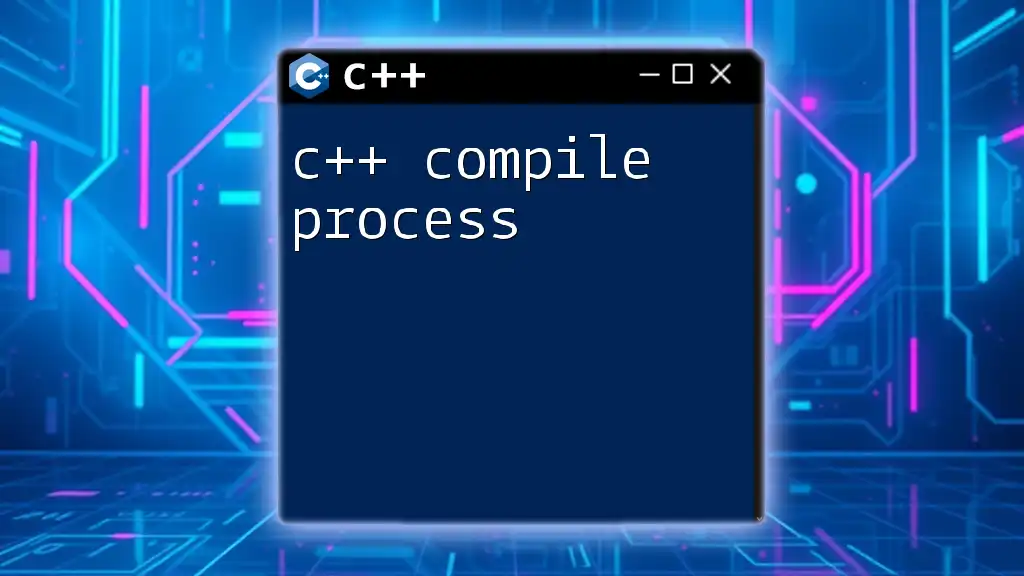
Call to Action
To deepen your knowledge of C++, explore additional resources, coding tutorials, and practice programs. Subscribe to our updates for more concise programming tips and insights. Additionally, download our free compilation guide to further boost your proficiency in C++ programming.
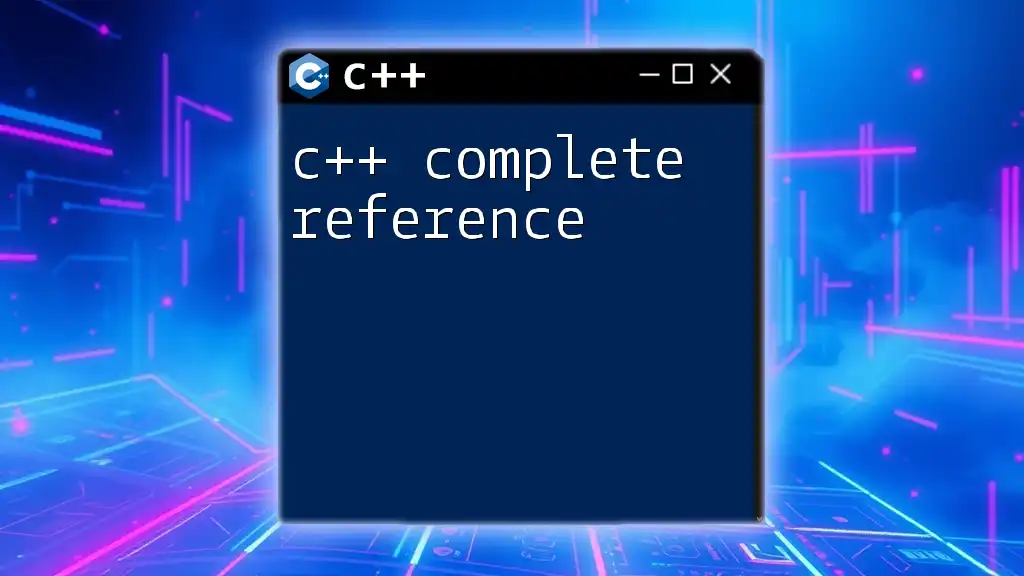
Additional Resources
Recommended Reading
- "The C++ Programming Language" by Bjarne Stroustrup.
Useful Tools
- [GCC Compiler](https://gcc.gnu.org/)
- [Clang Compiler](https://clang.llvm.org/)
- [Microsoft Visual C++](https://visualstudio.microsoft.com/)