For intermediate C++ learners, tackling practical projects can enhance your programming skills and deepen your understanding of concepts, such as building a simple banking system to manage user accounts.
Here's a code snippet illustrating a basic structure:
#include <iostream>
#include <string>
#include <map>
class Bank {
std::map<std::string, double> accounts;
public:
void createAccount(const std::string& name, double initialDeposit) {
accounts[name] = initialDeposit;
std::cout << "Account created for " << name << " with initial deposit: $" << initialDeposit << std::endl;
}
void displayBalance(const std::string& name) {
if (accounts.find(name) != accounts.end()) {
std::cout << "Account balance for " << name << ": $" << accounts[name] << std::endl;
} else {
std::cout << "Account not found for " << name << std::endl;
}
}
};
int main() {
Bank bank;
bank.createAccount("Alice", 1000.00);
bank.displayBalance("Alice");
return 0;
}
Getting Started with Intermediate C++ Projects
Understanding the Requirements
Before diving into C++ projects for intermediate developers, it’s essential to understand what this level entails. An intermediate developer typically has a strong grasp of key concepts, including:
- Object-oriented programming (OOP): Understanding classes, inheritance, and polymorphism.
- Memory management: Proficient use of pointers, dynamic allocation, and understanding memory leaks.
- Standard Template Library (STL): Familiarity with containers, iterators, and algorithms.
Having a solid command of these skills will empower you to tackle projects that enhance your C++ capabilities.
Selecting the Right Tools
When embarking on your project journey, selecting the right tools can make a significant difference. Here are the recommendations:
- IDEs (Integrated Development Environments):
- Visual Studio: Ideal for Windows users, offering a range of features for C++ development.
- Code::Blocks: Lightweight and cross-platform, suitable for simpler development needs.
- CLion: A robust IDE from JetBrains that supports CMake and offers powerful refactoring tools.
Utilizing libraries and frameworks, such as Qt or Boost, can elevate your projects significantly. Additionally, embracing a version control system like Git ensures that you can manage your code effectively and track changes over time.
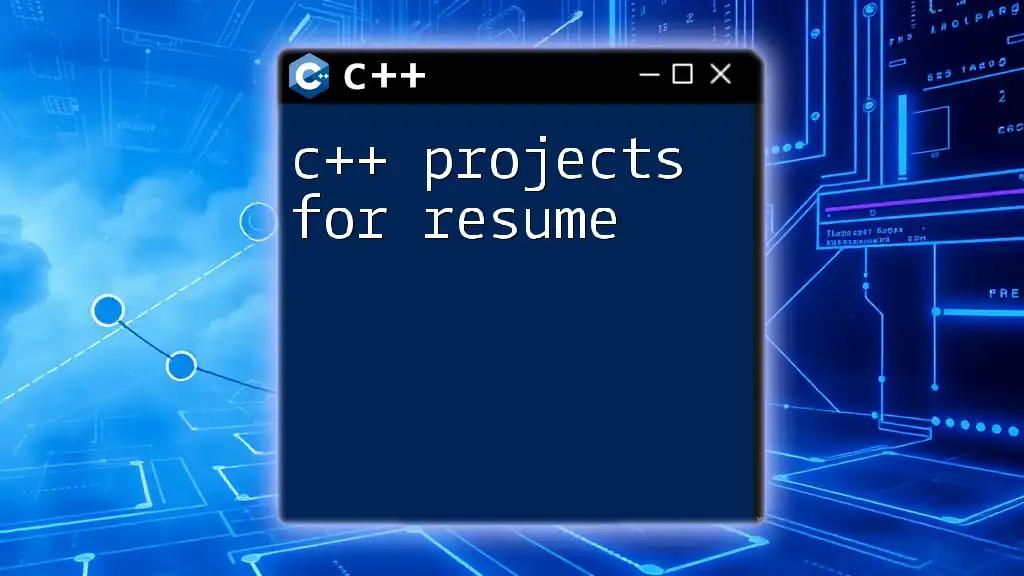
Project Ideas to Enhance Your C++ Skills
Game Development Projects
Building a Simple 2D Game
Creating a simple 2D game, such as Pong or Snake, is an excellent way to apply your C++ knowledge. This project will involve fundamental concepts like class structures and game loops.
Here's a basic structure to get you started:
class Game {
public:
void run() {
while (isRunning) {
processInput();
updateGame();
render();
}
}
};
In this snippet, the run method encompasses the core game loop, allowing for processing input, updating game states, and rendering graphics.
Creating a Text-Based Adventure Game
A text-based adventure game is another fantastic project. It challenges you to implement functions and decision-making structures effectively. With users making choices through text input, you’ll learn to manage game flow and utilize conditionals.
Consider using a `switch` statement to handle user decisions:
switch(choice) {
case 1:
// Move to the next room
break;
case 2:
// Pick up an item
break;
default:
// Invalid choice
break;
}
Data Structure Projects
Implementing a Personal Library Management System
This project allows you to create a class for books and manage a collection. You’ll leverage data structures, such as vectors, to store and manipulate your data.
Here’s an example of adding a book to the system:
class Library {
private:
std::vector<Book> books;
public:
void addBook(const Book& book) {
books.push_back(book);
}
};
This simple `addBook` method modifies the internal list of books, highlighting how you can manage collections dynamically.
Developing a Basic Contact Management System
Similar to the library management system, this project requires you to design a program storing contacts. Class structures can encapsulate all contact information and enable searching functionalities.
File Handling Projects
Creating a Budget Tracker
Developing a budget tracker will test your ability to read and write files effectively. This project will also incorporate input validation, ensuring that users do not enter invalid data.
Here’s how you can read expenses from a file:
std::ifstream inputFile("expenses.txt");
std::string line;
while (getline(inputFile, line)) {
// Process the line
}
In this snippet, the program reads from `expenses.txt`, illustrating the basics of file handling in C++.
Implementing a Simple CSV Parser
Creating a CSV parser offers a hands-on experience with file I/O. You’ll read from a CSV file, process its contents, and perhaps convert them into a user-friendly structure. Understanding delimiters and data handling will be crucial here.
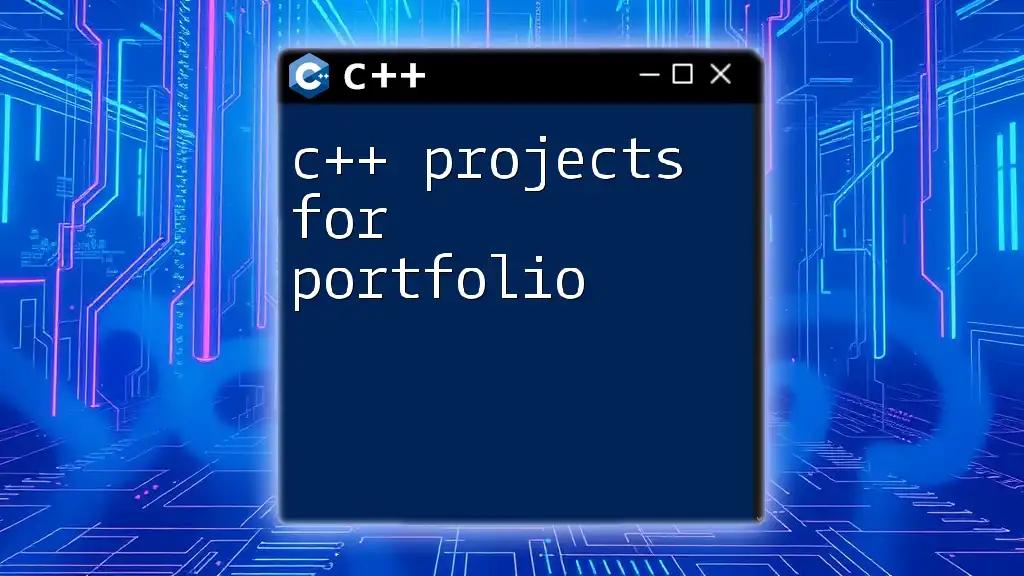
Exploring Advanced Concepts through Projects
Multithreading in C++
As you delve deeper into C++, you might explore multithreading. A concurrent file downloader project can serve as a practical implementation of this concept.
Consider a simple example demonstrating thread creation:
#include <thread>
void downloadFile(std::string url) {
// Code to download file from the URL
}
int main() {
std::thread t1(downloadFile, "http://example.com/file1");
std::thread t2(downloadFile, "http://example.com/file2");
t1.join();
t2.join();
return 0;
}
This code snippet demonstrates leveraging multiple threads to perform tasks concurrently, an advanced skill set valuable in modern programming.
Network Programming
Building a Simple Chat Application
Networking is a vital area of C++. A basic chat application can teach you about socket programming. You’ll explore client-server architecture and understand how data is transmitted over the network.
Here’s a fundamental example of establishing a TCP connection:
#include <sys/socket.h>
#include <netinet/in.h>
int main() {
int sock = socket(AF_INET, SOCK_STREAM, 0);
sockaddr_in server;
server.sin_family = AF_INET;
server.sin_port = htons(8080);
// Additional connection code
return 0;
}
This establishes a basic socket connection, the first step toward developing a chat application.
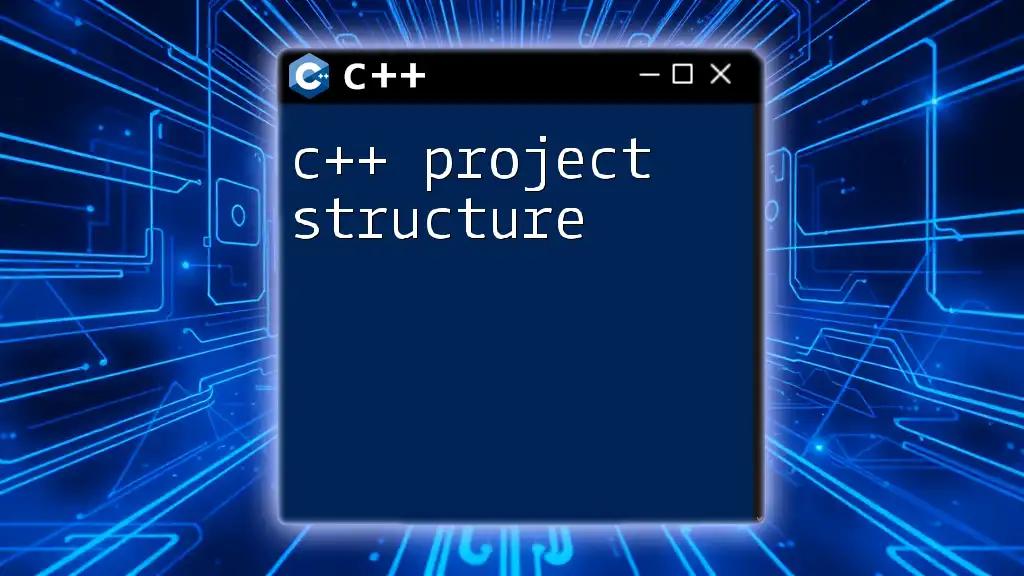
Best Practices for C++ Development
Code Quality and Readability
As you write more code, maintain code quality by following established conventions. Adopting a consistent naming scheme, appropriate indentation, and commenting on your code will significantly enhance its readability.
Here's an example showcasing good practices:
class Rectangle {
public:
Rectangle(double width, double height) : width(width), height(height) {}
double getArea() const {
return width * height;
}
private:
double width;
double height;
};
This `Rectangle` class focuses on clear naming and encapsulation, which promotes maintainability.
Debugging and Testing
Debugging is an integral part of the development process. Use tools tailored for C++ to catch errors early. Furthermore, writing unit tests with frameworks like Google Test ensures that your functionalities work correctly.
A simple unit test example could look like this:
TEST(RectangleTest, AreaCalculation) {
Rectangle rect(5.0, 4.0);
ASSERT_EQ(rect.getArea(), 20.0);
}
This code tests the area calculation of your `Rectangle` class, reinforcing the importance of testing as you build projects.
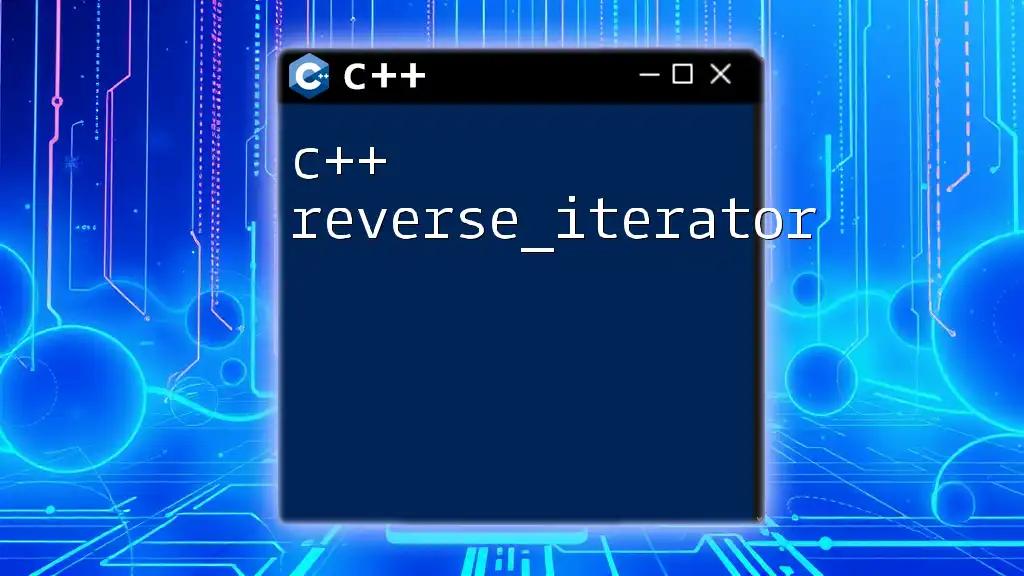
Conclusion
Engaging in C++ projects for intermediate developers helps solidify your understanding and expand your repertoire of skills. By creating games, managing data, or working with files, you will apply your C++ knowledge in practical, meaningful ways.
Don’t hesitate to tackle these projects and even add your unique spin. The skills you gain will serve you well in your journey toward mastery in C++. And as you progress, remember to share your projects and experiences with the community to inspire others and foster collaboration.
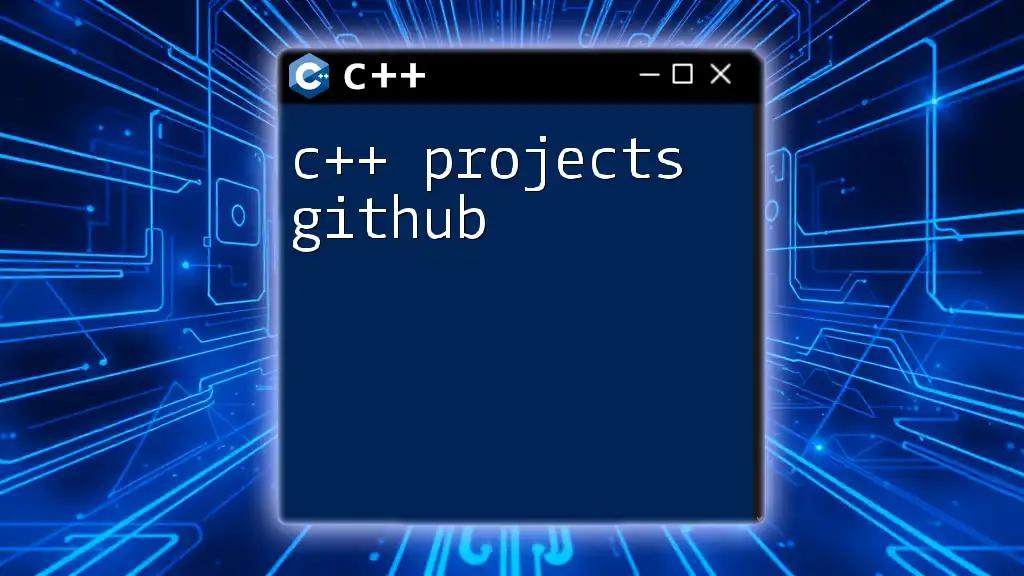
Additional Resources
When pursuing further learning in C++, consider exploring forums, tutorials, and books dedicated to deepening your knowledge. Engaging with community platforms can provide immense value as you share your projects and receive constructive feedback.