C++ projects on GitHub are repositories where developers share their C++ code, allowing others to learn from, contribute to, or utilize these projects for their own purposes.
Here’s an example of a simple C++ program to demonstrate basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Makes a Good C++ Project on GitHub?
Key Characteristics of Successful C++ Projects
When searching for or considering creating C++ projects on GitHub, certain characteristics can signify a well-managed and valuable project. A successful project typically features:
-
Clear documentation: This includes a comprehensive README file that details how to install, use, and contribute to the project. Good documentation serves as the project's best advocate.
-
Avoidance of anti-patterns: Adhering to best practices and design patterns is crucial in C++. Projects that exhibit common anti-patterns like "Spaghetti Code" are often harder to understand and maintain.
-
Modular design: A modular architecture enables easier testing, scaling, and maintenance. It allows developers to work on various components independently.
-
Active community and contributions: Projects that receive regular contributions show that they are being maintained and that there is a vibrant developer community behind them.
Popular C++ Project Examples
Some notable C++ projects on GitHub include:
-
OpenCV: An open-source computer vision and machine learning library. OpenCV has a wide user base and is instrumental for anyone interested in image processing.
-
LLVM: A collection of modular and reusable compiler and toolchain technologies. LLVM serves as an excellent example of a large-scale C++ project with a significant academic and industrial impact.
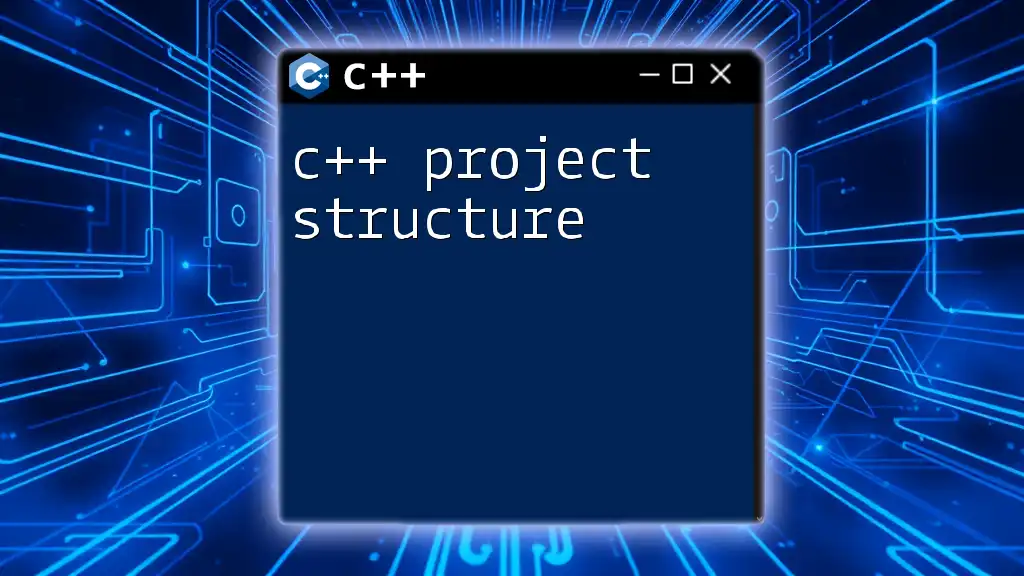
Navigating GitHub for C++ Projects
Searching for C++ Projects
GitHub provides powerful search capabilities that can streamline the process of finding C++ projects. Here are some tips:
-
Use specific keywords like "C++ Projects" or "C++ libraries" to refine your search.
-
Tags and topics: Projects often have specific tags. Looking for “C++” as a topic can lead to focused results.
Filtering and Sorting Projects
After you have performed a search, sorting the results can help you identify the most popular projects. Use the following criteria:
-
Stars: This metric indicates how many users have liked a particular project and is often a good gauge of the project’s popularity.
-
Forks: The number of forks can indicate how many developers are interested in modifying or extending the project.
-
Recent activity: Projects that have had recent commits are often maintained and are less likely to be out-of-date.
Understanding different licenses is also important. Popular licenses include:
-
MIT: Permits basically 'anything', as long as the original license is included.
-
GPL: Requires derived works to also be open-source under the same license.
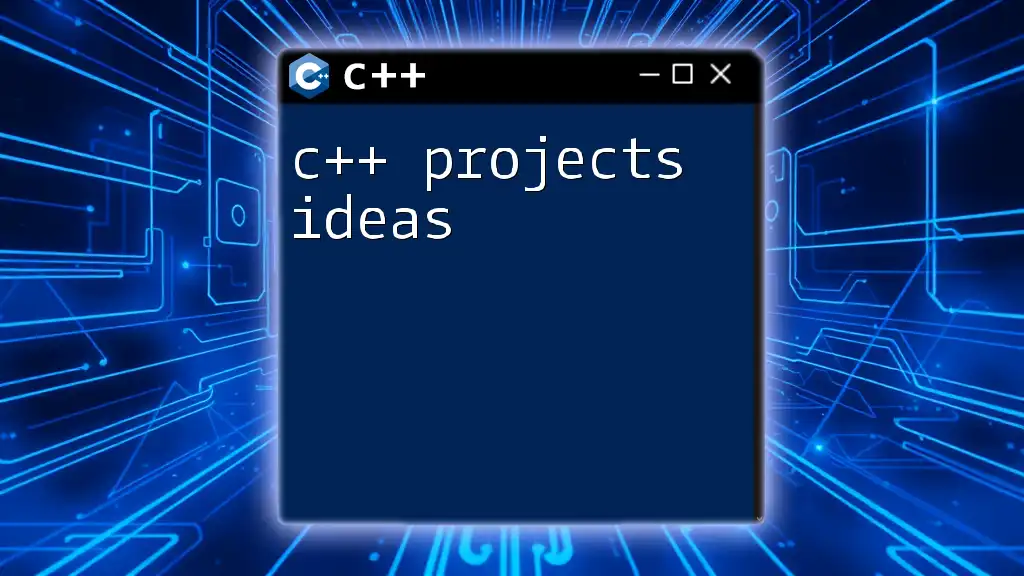
Benefits of Contributing to C++ Projects on GitHub
Skills Development
Engaging in C++ projects on GitHub is invaluable for enhancing your skill set:
-
Technical skills: By participating in projects, you’ll learn coding standards, debugging techniques, and the intricacies of version control through Git.
-
Soft skills: Collaboration fosters skills like teamwork, communication, and project management—essential in any development role.
Networking Opportunities
Working on GitHub can also provide networking opportunities. Being part of a project fosters relationships with other developers, paving the way for mentorship or collaboration on future projects.
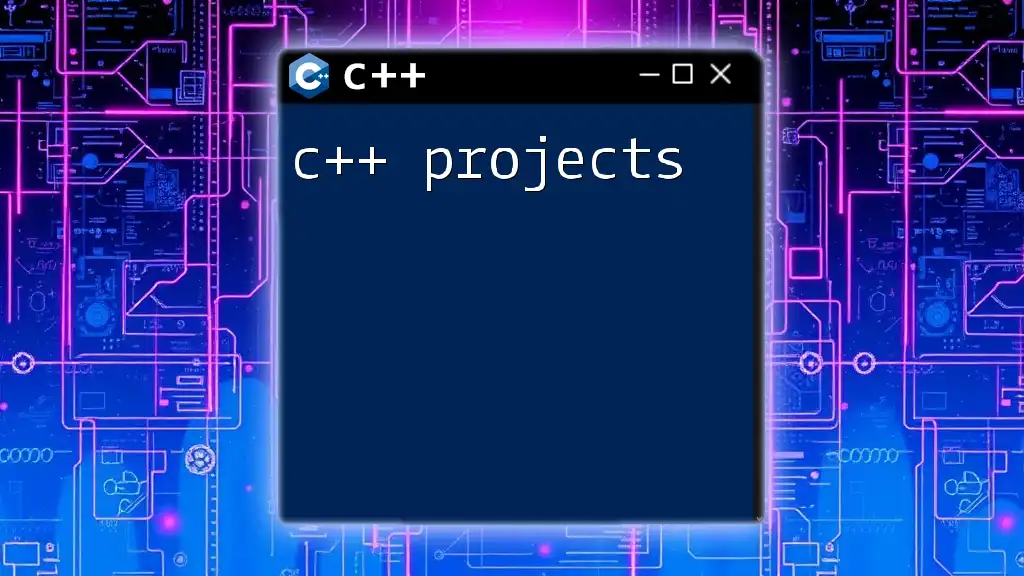
Starting Your Own C++ Project on GitHub
Setting Up Your GitHub Repository
Creating a repository for your C++ project can be straightforward:
- Go to GitHub and click on the "+" sign in the top-right corner.
- Select "New Repository". Fill in vital details such as name, description, and visibility (public or private).
The README file is essential to your repository. It should cover:
- How to install the project
- How to use it
- How other developers can contribute
Writing Great C++ Code
For effective C++ development, adhere to best practices:
-
Keep your code readable and maintainable. A common approach is to write functions that are small and specific in functionality.
-
Follow code style guidelines. This helps ensure consistency and improves code readability. Consider employing resources like Google's C++ style guide or LLVM's coding standards.
Documenting Your Project
Documentation is vital. It can alleviate onboarding challenges for new contributors and end-users. Tools like Doxygen can assist in generating documentation from annotated C++ code. Example syntax to generate documentation:
/**
* This function adds two integers.
* @param a The first integer.
* @param b The second integer.
* @return The sum of the two integers.
*/
int add(int a, int b) {
return a + b;
}
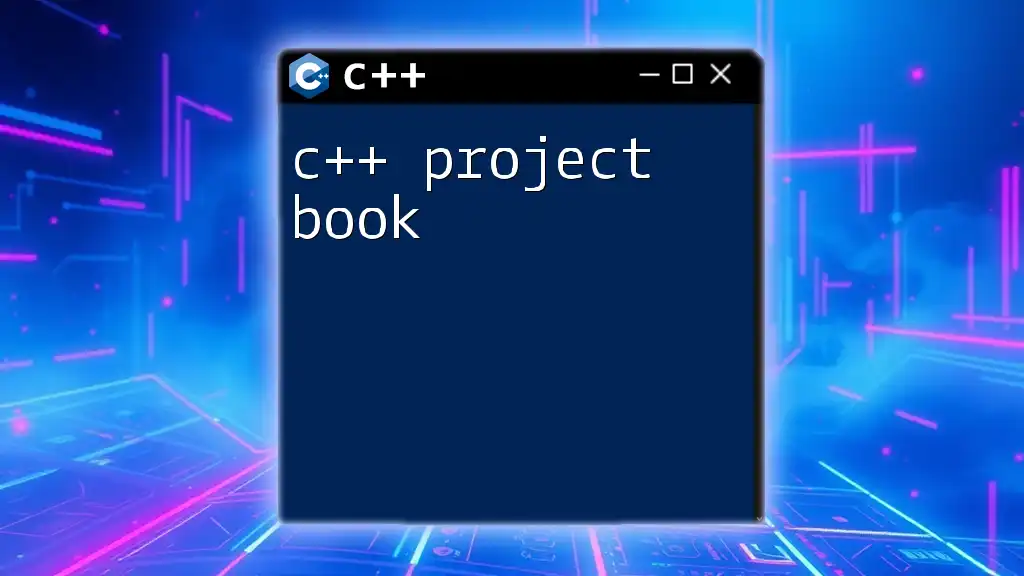
Top C++ Project Ideas to Kickstart Your GitHub Journey
Beginner-Friendly Projects
For newcomers, consider starting with:
-
Simple calculator: Create a console application that can perform basic arithmetic operations.
-
Basic file manipulation tool: Develop a program that can read from and write to files, allowing users to manipulate text data.
Intermediate Projects
If you want a bit more challenge, try these:
-
A text-based game: Building a simple game like Tic-Tac-Toe can teach you about game loops, user input, and state management.
-
Image processing tool using OpenCV: Experimenting with image filters or transformations can give you hands-on experience with libraries and algorithms.
Advanced Projects
For those ready for complex challenges, consider:
-
A game engine from scratch: Developing a basic game engine can deepen your understanding of various programming concepts.
-
Implementing data structures and algorithms: Create a library that includes common data structures (like trees or graphs) and algorithms (like sorting and searching). This can be both educational and applicable.
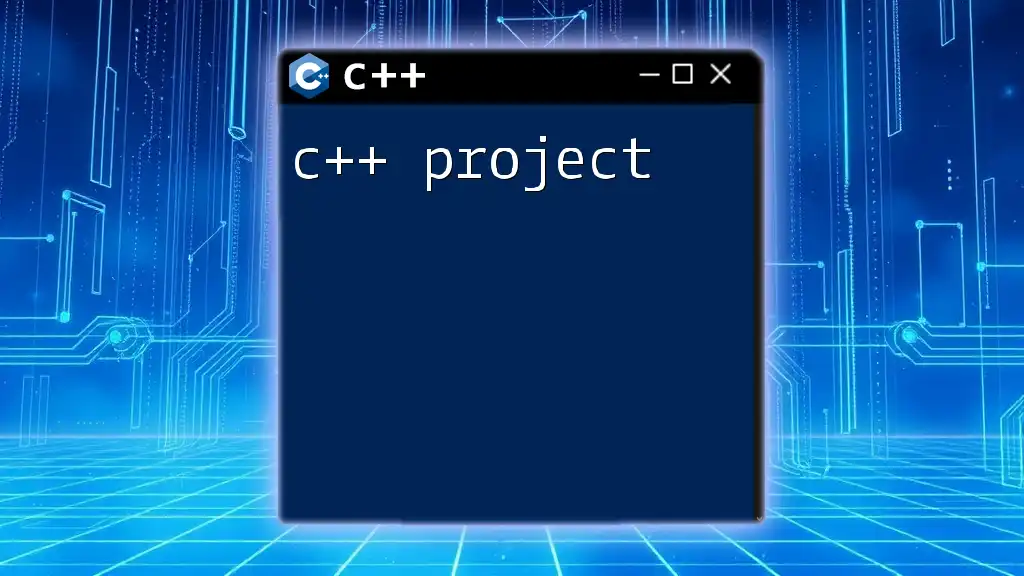
Collaborating with Other Developers
Understanding Open Source Contribution
Entering the world of open source contribution can feel daunting. Here's a breakdown:
-
Forking a project: This creates a personal copy that you can modify independently.
-
Pull requests: After making changes, you can send a pull request for the original project maintainers to review your contributions.
Finding and Joining C++ Projects
Websites like Up For Grabs or First Timers Only list projects actively seeking help. Engaging with these resources can simplify finding a place to contribute.
Reaching out to project maintainers with a concise message expressing your interest can open doors to collaboration.
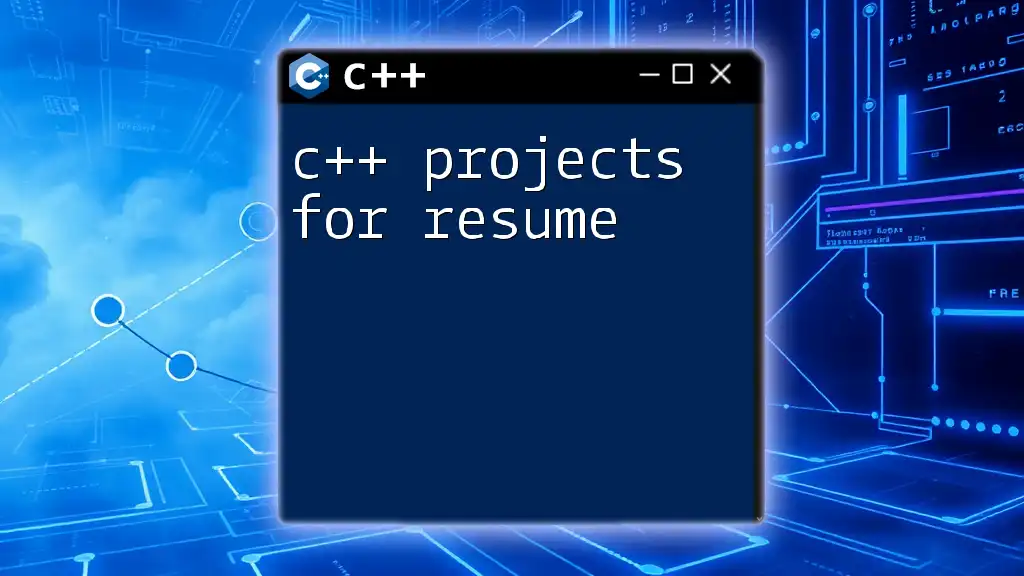
Conclusion
Engaging with C++ projects on GitHub can significantly enhance your coding skills, broaden your professional network, and deepen your understanding of software development practices. Whether you are contributing to or creating new projects, the GitHub ecosystem offers countless opportunities for growth and learning.
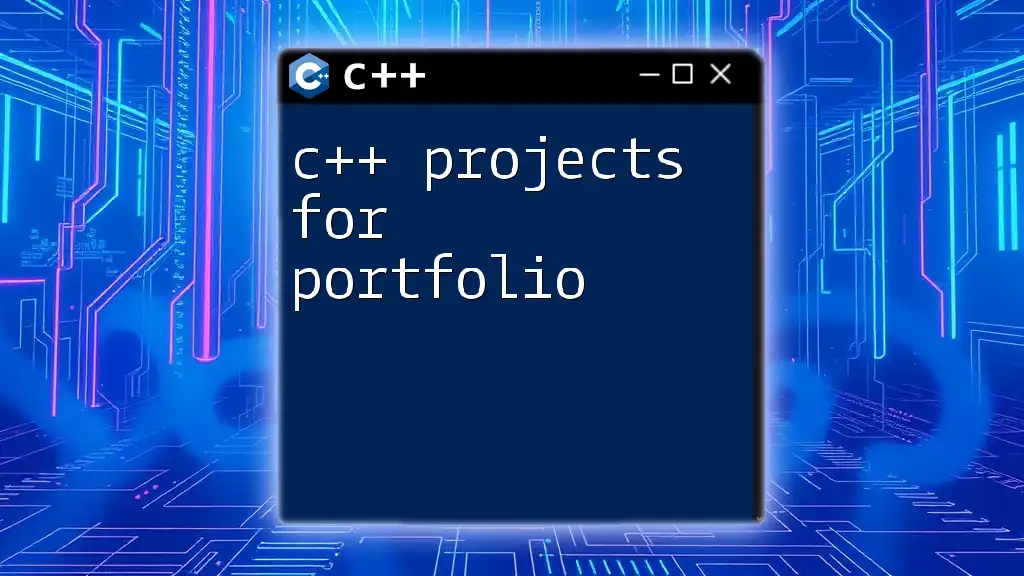
Additional Resources
For more insights, explore various C++ development blogs, contribute to forums like Stack Overflow, or dive into prominent GitHub repositories.
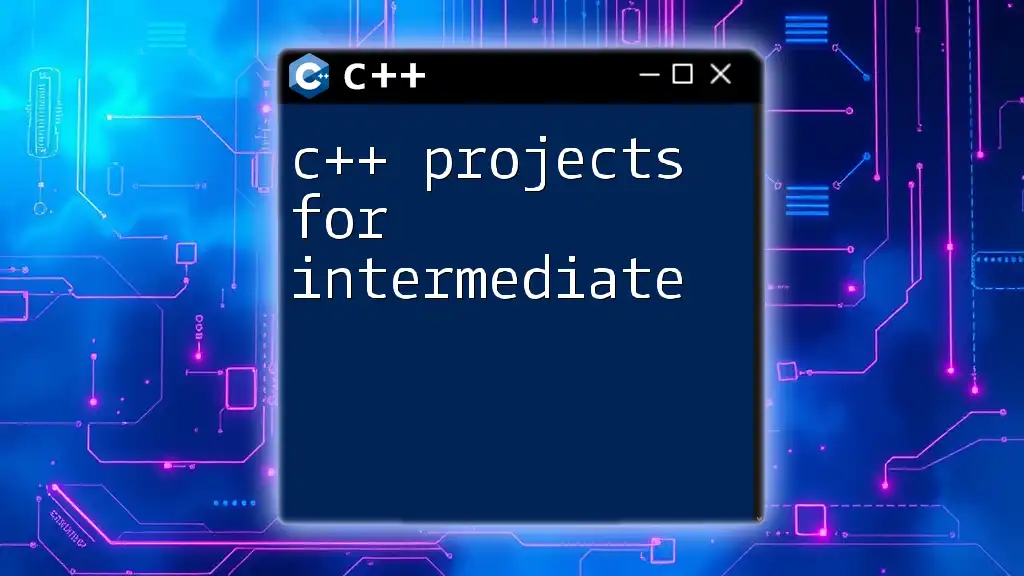
Call to Action
We’d love to hear about your experiences with C++ projects on GitHub! Share your stories or ask any questions in the comments below, and let’s build a community of C++ enthusiasts together.