"Beginner C++ projects are simple yet effective ways to practice programming skills and grasp fundamental concepts through hands-on coding experiences."
Here’s a basic example of a project that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++ Development Environment
To dive into beginner C++ projects, you first need to set up an appropriate C++ development environment. This will serve as your workspace for developing and testing your projects.
Setting Up Your C++ Development Environment
Recommended IDEs (Integrated Development Environments) can greatly enhance your coding experience by providing features like code completion and easy debugging. Popular options for beginners include:
- Visual Studio: Offers an intuitive interface and a comprehensive set of tools.
- Code::Blocks: Lightweight and flexibility make it a solid choice for simple projects.
- Online Compilers: Websites like repl.it and ideone.com are perfect if you want to start coding without downloading anything.
Next, you'll need to install a C++ compiler. The most widely used compilers include GCC and Clang. Follow the specific installation steps based on your operating system to ensure that your environment is correctly configured.
Writing Your First C++ Program
Once your environment is set up, you can try writing your very first C++ program — a classic "Hello, World!" example. This program introduces you to the basic syntax of C++. Here’s how to do it:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple line of code outputs the message "Hello, World!" to the console. It’s a fundamental first step that helps you verify that your environment is functioning as expected.
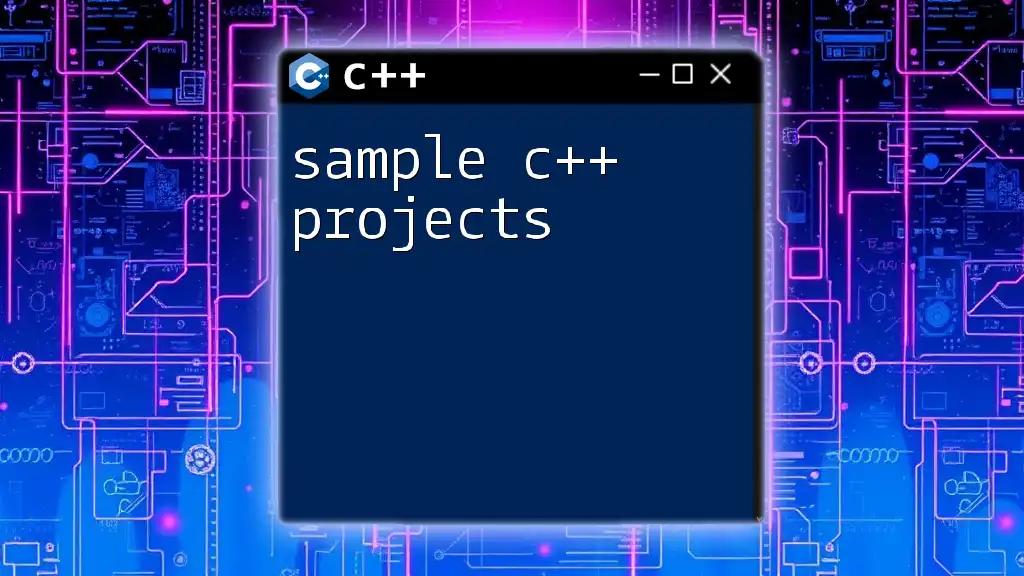
Exciting C++ Beginner Projects to Try
As you embark on your journey with C++, working on beginner C++ projects is an excellent way to apply what you've learned. Here are some practical projects to consider starting with:
Fun and Simple C++ Projects for Beginners
Basic Calculator
The Basic Calculator project is perfect for grasping basic arithmetic operations and user input. This project will help you understand operators, conditional statements, and input/output functions in C++.
Here's a simple code snippet to get you started:
#include <iostream>
using namespace std;
int main() {
char op;
float num1, num2;
cout << "Enter operator (+, -, *, /): ";
cin >> op;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
switch(op) {
case '+':
cout << num1 + num2;
break;
case '-':
cout << num1 - num2;
break;
case '*':
cout << num1 * num2;
break;
case '/':
cout << num1 / num2;
break;
default:
cout << "Invalid operator";
}
return 0;
}
Running this application will allow users to perform simple calculations, gaining hands-on experience with C++'s control structures.
Tic-Tac-Toe Game
Creating a Tic-Tac-Toe game is another excellent project that introduces more advanced concepts such as arrays and loops. This project involves user interaction and game logic to handle the game state.
A basic structure of your game might involve defining a board and a display function:
#include <iostream>
using namespace std;
void displayBoard(char board[3][3]) {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
cout << board[i][j] << " ";
}
cout << endl;
}
}
// Additional functions for game logic...
By developing this game, you’ll gain a deeper understanding of how to handle user input and manage game sequences.
Intermediate C++ Beginner Projects
As you get more comfortable, consider these initiatives to push your skills further.
Basic Banking System
Building a Basic Banking System exposes you to concepts like structs and functions. The project typically includes features such as account creation, deposits, and withdrawals. This project allows you to practice organizing code and managing user data efficiently.
Here’s how you might define an account structure:
struct Account {
string accountHolder;
float balance;
};
void deposit(Account &acc, float amount) {
acc.balance += amount;
cout << "New balance: " << acc.balance << endl;
}
This project grants you insights into managing data and conducting various operations, which is vital in software development.
To-Do List Application
A To-Do List Application is yet another valuable project. This app will help users manage tasks effectively while teaching you about dynamic data handling using vectors and basic CRUD operations — Create, Read, Update, and Delete.
For example, utilizing C++’s standard vector library can enhance your ability to manage a flexible list of tasks:
#include <iostream>
#include <vector>
#include <string>
using namespace std;
vector<string> tasks;
// Function to display tasks
void displayTasks() {
for (int i = 0; i < tasks.size(); i++) {
cout << i + 1 << ": " << tasks[i] << endl;
}
}
By developing this application, you will interact with data structures, improving your overall programming skills.
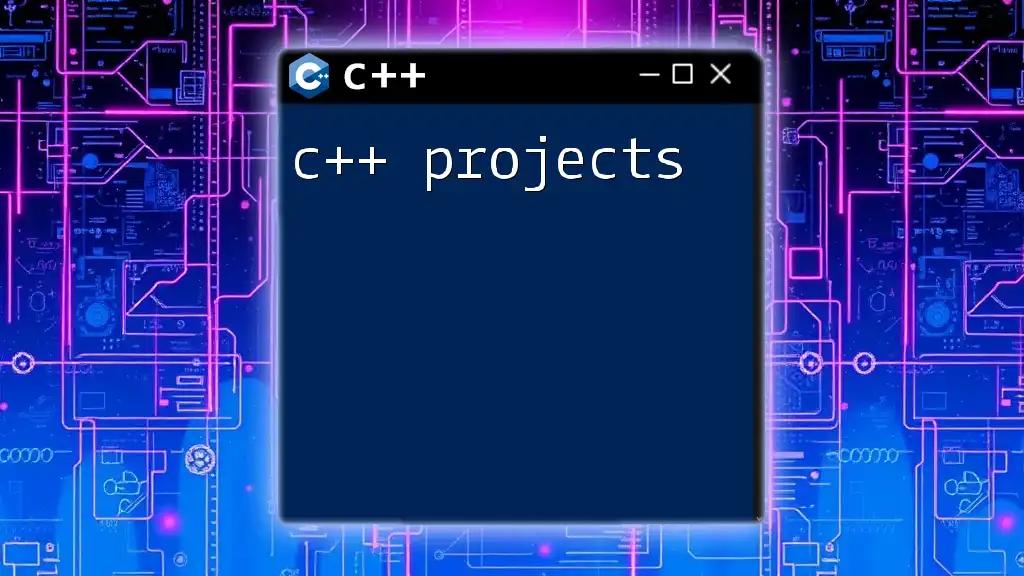
Tips for Successfully Completing C++ Beginner Projects
Structuring Your Projects
When managing projects, planning is crucial. Begin with requirement analysis — outlining what your project aims to achieve. Draft a thorough project outline that covers the features and functionalities you want to implement.
Debugging Practices
Inevitably, debugging is a significant aspect of working with C++. Familiarize yourself with common debugging techniques. Learning to identify errors in your code will save you much time and frustration. Various tools, like gdb or IDE-integrated debuggers, can aid in tracing issues effectively.
Seeking Help and Resources
No one learns programming entirely on their own. Leverage online C++ communities such as Stack Overflow and GitHub. Engaging in discussions and following tutorials can bridge gaps in knowledge. Additionally, forums and local user groups can provide invaluable support.
Benefits of Collaboration on Projects
Consider collaborating with others on projects or even contributing to open-source software. Working as part of a team exposes you to different perspectives and coding styles, enriching your learning experience.
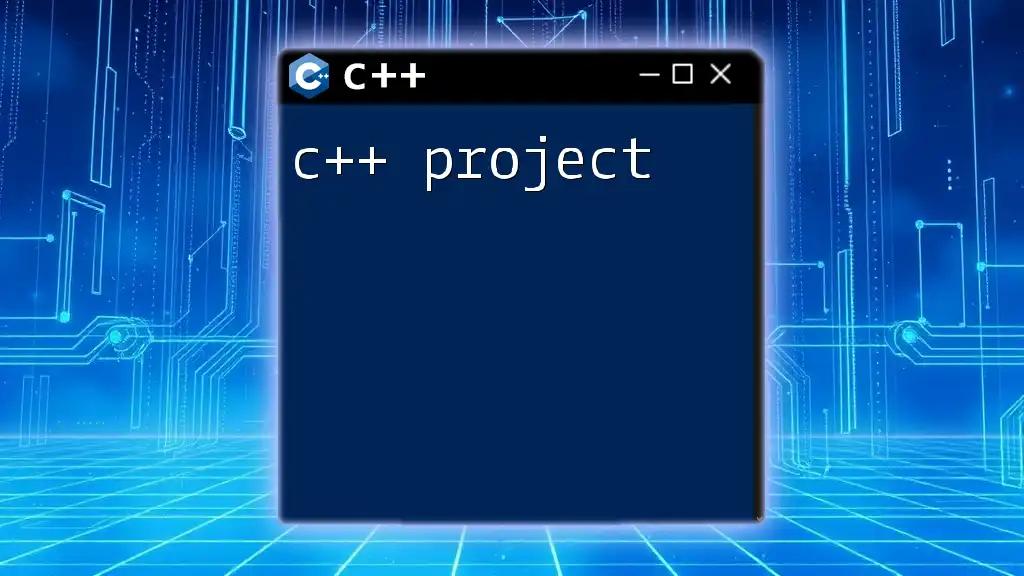
Conclusion
Embarking on beginner C++ projects is an enriching journey that will lay a solid foundation for your programming skills. Practical applications not only consolidate your understanding but also build your confidence.
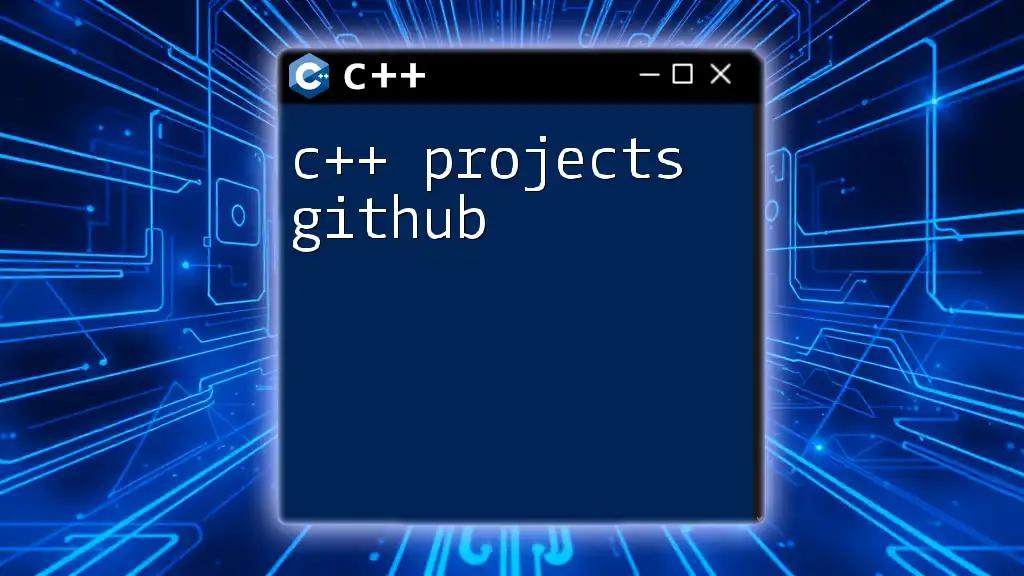
Call to Action
Join our community and keep exploring! We invite you to subscribe for more tutorials and project guides that will take your C++ skills to the next level. Share your progress in projects with others to inspire and be inspired. Happy coding!