C++ Protocol Buffers (protobuf) is a method developed by Google for serializing structured data, enabling efficient data communication between programs.
#include <iostream>
#include "example.proto" // include the generated protobuf header
int main() {
MyMessage msg; // Create a new message
msg.set_id(1); // Set the message fields
msg.set_name("Hello Protobuf");
std::string serialized_data;
msg.SerializeToString(&serialized_data); // Serialize the message
std::cout << "Serialized data: " << serialized_data << std::endl;
return 0;
}
Introduction to Protobuf
What is Protobuf?
Protocol Buffers (Protobuf) is a language-agnostic binary serialization format developed by Google. It allows developers to define structured data in a .proto file and generates code that can read and write this data in a more efficient manner than conventional text-based serialization methods like XML or JSON. This efficiency is crucial for performance-critical applications and systems where data interchange is frequent.
Why Use Protobuf in C++?
Using Protobuf with C++ yields several advantages:
- Efficiency: Protobuf uses a compact binary format that minimizes file sizes and improves transmission speeds over networks.
- Strongly Typed: With Protobuf, you define schemas for your data. This helps catch errors early, which is vital in large-scale software development.
- Backward Compatibility: Protobuf supports field presence and backward/forward compatibility, making it easier to evolve your data structures without breaking existing applications.
Use cases include communication between microservices, configuration storage, or network protocol development, showcasing the versatility of c++ protobuf.

Setting Up Protobuf for C++
Installation Guide
To get started with c++ protobuf, you need to install the Protobuf compiler and libraries. Follow these steps based on your operating system:
-
Linux: You can install Protobuf using a package manager:
sudo apt-get install protobuf-compiler libprotobuf-dev
-
macOS: Use Homebrew for installation:
brew install protobuf
-
Windows: You might need to build Protobuf from source or use precompiled binaries available from GitHub.
Compiling Protobuf
After installation, you should be able to compile Protobuf from the command line. Verify the installation:
protoc --version
Make sure it returns the version number of the installed Protobuf compiler.

Protobuf Basics
Protobuf Language Structure
Protobuf message format relies on defining schemas in `.proto` files. These files explicitly state structures and types, making it easier to manage data formats across different languages and platforms.
Defining Protobuf Messages
Creating your first .proto file starts with a simple message structure. Here’s an example of how to define a message for storing a person's data:
syntax = "proto3";
message Person {
string name = 1;
int32 id = 2;
string email = 3;
}
In this snippet, the `syntax = "proto3";` line indicates that we are using version 3 of Protobuf. Each field has a type (`string`, `int32`) and a unique identifier (1, 2, 3), used for serialization.
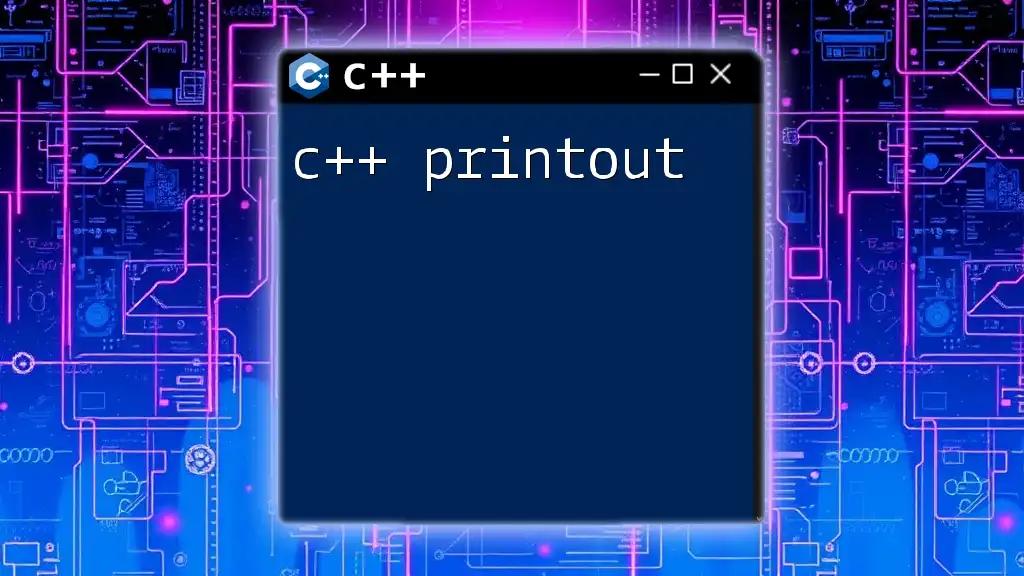
Generating C++ Code from Protobuf Definitions
Using the Protobuf Compiler
To generate C++ classes from your `.proto` definitions, you can use the Protobuf Compiler (`protoc`). This is done by running the following command in your terminal:
protoc --cpp_out=. person.proto
This command will create `person.pb.h` and `person.pb.cc`, which contain the classes needed to work with your `Person` message in C++.
Understanding Generated Files
The generated files include `Person::SerializeToString` and `Person::ParseFromString` methods, which allow you to convert your objects to and from binary format easily.

Using Protobuf in C++ Applications
Serializing Messages
To utilize c++ protobuf, you must serialize your message objects. Here's how you can do this in C++:
#include "person.pb.h"
Person person;
person.set_name("John Doe");
person.set_id(123);
person.set_email("john.doe@example.com");
std::string serialized_data;
person.SerializeToString(&serialized_data);
In this code, we create an instance of the `Person` class, set its fields, and serialize it into a string.
Deserializing Messages
To read back the serialized data, you will deserialize the string back into a `Person` object. Here’s an example of how to do that:
Person new_person;
if (new_person.ParseFromString(serialized_data)) {
// Successfully parsed the data
std::cout << "Name: " << new_person.name() << std::endl;
std::cout << "ID: " << new_person.id() << std::endl;
std::cout << "Email: " << new_person.email() << std::endl;
} else {
// Handle error
std::cerr << "Failed to parse the person data." << std::endl;
}
This snippet shows how to check if data was successfully parsed and output the fields.

Advanced Protobuf Features
Nested Messages and Enums
Protobuf allows the definition of nested fields and enumerated types. Here’s an example of a nested message structure using a `Contact` message that contains `PhoneNumber`:
message Contact {
message PhoneNumber {
string number = 1;
PhoneType type = 2;
}
repeated PhoneNumber phone = 1;
}
enum PhoneType {
MOBILE = 0;
HOME = 1;
WORK = 2;
}
Here, we define a `Contact` message that can hold multiple `PhoneNumber` entries and an enumerated `PhoneType`.
Using Protobuf with gRPC
gRPC, a high-performance RPC framework, utilizes Protobuf for defining service contracts. Here’s a simple example of how you might define a service:
service Greeter {
rpc SayHello (HelloRequest) returns (HelloReply) {}
}
When using gRPC, Protobuf not only helps define data but also the method of communication, enabling efficient service interactions.
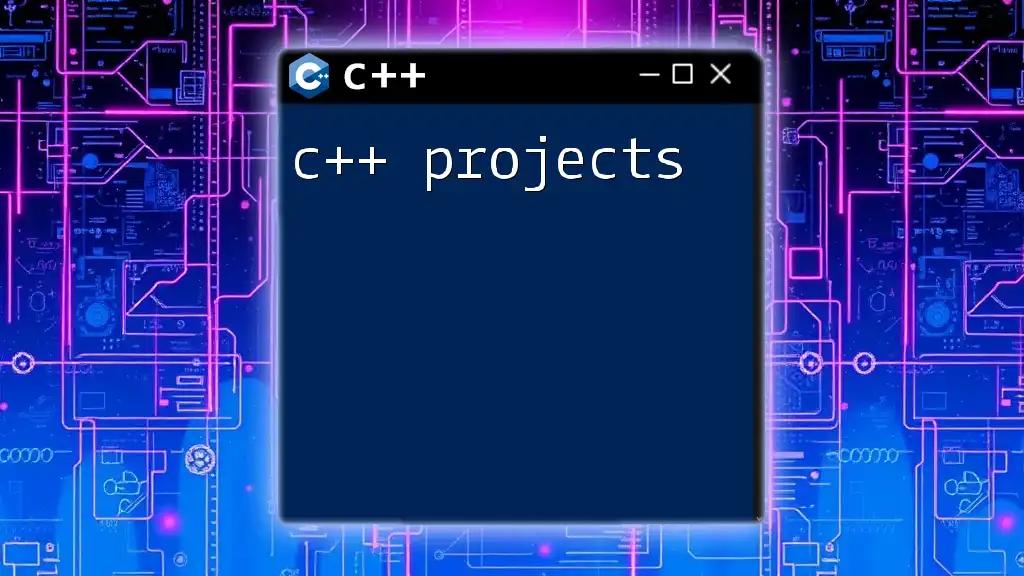
Common Issues and Troubleshooting
Common Pitfalls with C++ Protobuf
Some frequent issues encountered while working with c++ protobuf include:
- Missing Fields: If a required field is not set before serialization, it can lead to errors during parsing.
- Version Mismatch: Ensure that the Protobuf version used for compiling matches the version of the library used in your application.
Performance Tuning
To optimize Protobuf in C++, consider:
- Use of `packed` option: For repeated fields, using `packed=true` can save space and improve performance.
- Benchmarking Serialization: Regularly check the serialization and deserialization times if performance is critical for your application.
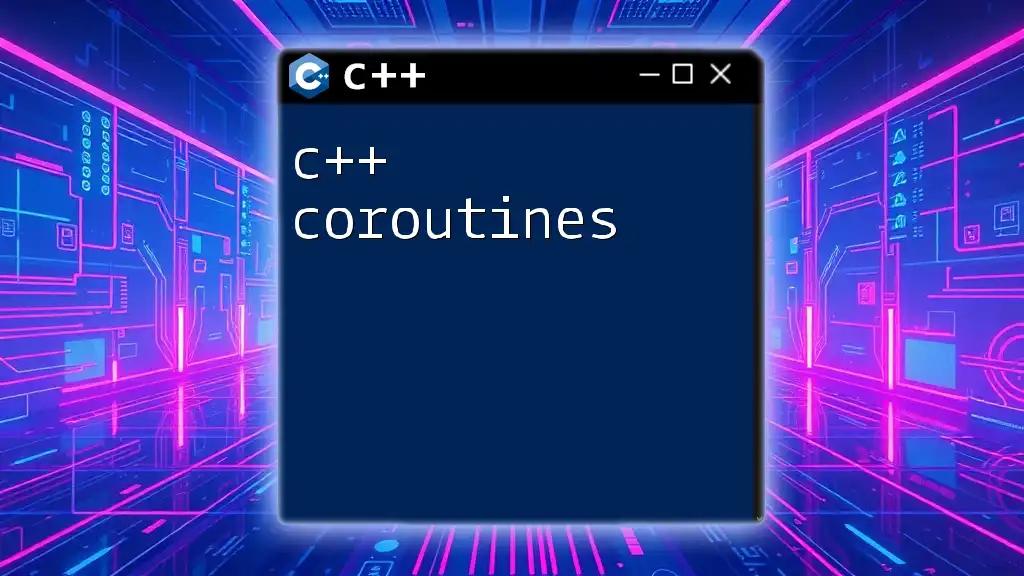
Conclusion
Recap of Protobuf in C++
In this comprehensive guide, we explored the essentials of c++ protobuf, from installation and message definition to advanced features like nested types and integration with gRPC. Protobuf provides developers with a powerful tool to serialize data efficiently while maintaining type safety.
Next Steps and Resources
To dive deeper into Protobuf, consider exploring the official [Protocol Buffers documentation](https://developers.google.com/protocol-buffers/) and experimenting with real-world applications and its integration with other libraries.

FAQs
What are the differences between Protobuf and other serialization formats?
Compared to JSON or XML, Protobuf is more compact and faster because it serializes data in a binary format rather than human-readable text. This translates to less bandwidth used and quicker storage and retrieval times.
Can Protobuf be used in cross-platform applications?
Yes! Protobuf is designed to be portable; its generated files can be used across different programming languages and platforms, allowing for seamless communication.
What are some best practices for defining Protobuf messages?
- Use descriptive names for your messages and fields.
- Always define a unique number for fields to maintain backward compatibility as your application grows.
- Consider using enums for a set of predefined values to ensure valid data entries.
With these insights and examples, you're equipped to start leveraging c++ protobuf effectively in your projects.