In C++, plotting is typically achieved using external libraries such as Matplotlib for C++ or third-party libraries like GNUplot, which allow you to visualize data points in a graphical format.
Here's a basic example using GNUplot to plot a simple graph:
#include <iostream>
#include <fstream>
int main() {
std::ofstream data("data.dat");
for (int i = 0; i <= 10; i++) {
data << i << " " << i * i << std::endl; // plotting y = x^2
}
data.close();
system("gnuplot -e \"set terminal png; set output 'plot.png'; plot 'data.dat' using 1:2 with lines;\"");
return 0;
}
Understanding Plotting in C++
What is Plotting?
Plotting is the graphical representation of data. It transforms complex numerical insights into visual formats that are easier to understand and analyze. In programming, plotting is commonly used to visualize data trends, distributions, and relationships, making it an essential tool for data scientists and engineers alike.
Why Use C++ for Plotting?
C++ is a powerful programming language that is known for its performance and efficiency. It allows developers to create high-performance applications, which is crucial when handling large datasets. Compared to other programming languages, such as Python or R, C++ offers more control over memory management and performance optimization. This makes it an excellent choice for scenarios where speed and efficiency are paramount.
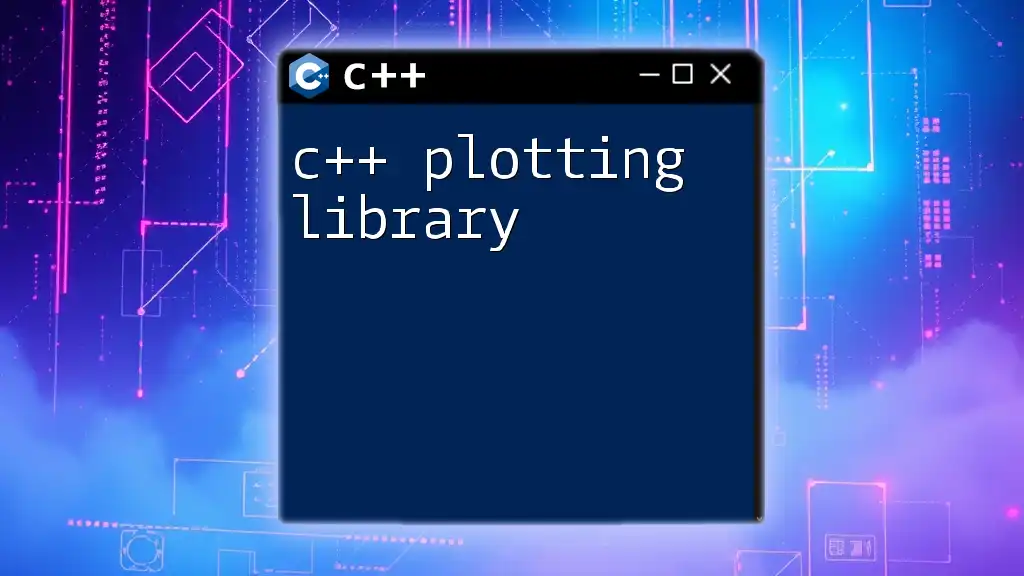
Getting Started with C++ Plotting
Setting Up Your Environment
To begin plotting in C++, you will need an Integrated Development Environment (IDE) that supports C++. Some popular choices include:
- Visual Studio: Ideal for Windows users with extensive features.
- Code::Blocks: A free cross-platform IDE that is lightweight.
- CLion: A paid option with robust support for CMake and deep code analysis.
Installing Necessary Libraries
To create plots, you must install specific libraries. Two popular choices include Matplotlib for C++, which interfaces with its Python counterpart, and SFML (Simple and Fast Multimedia Library) for graphical rendering.
To install Matplotlib for C++, follow these steps:
- Ensure you have `Python` and `pip` installed on your machine.
- Use pip to install Matplotlib by running:
pip install matplotlib
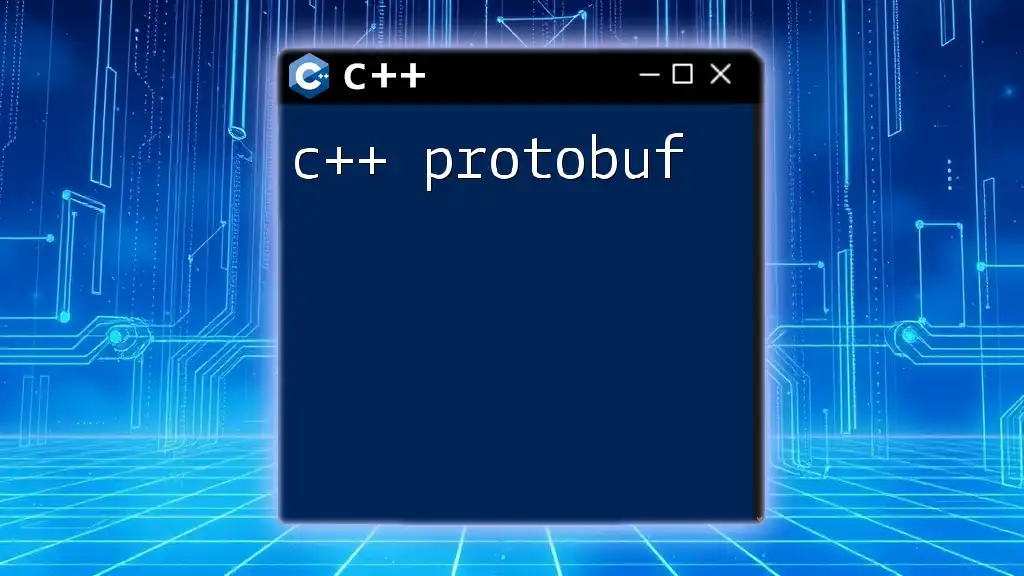
Basic Plotting Concepts
Types of Plots
There are various types of plots you can create depending on your data representation needs:
- Line Plot: Best for showing trends over a continuous variable, often time.
- Scatter Plot: Ideal for comparing two numerical values and visualizing their correlation.
- Bar Plot: Useful for displaying categorical data and comparisons amongst different groups.
Key Vocabulary and Concepts
Having terminology familiarity helps in creating effective visualizations. Here are some foundational terms:
- Axes: The reference lines for the plot, typically horizontal (x-axis) and vertical (y-axis).
- Legends: Descriptions that help identify the data series in a plot.
- Grids: The background lines that can make reading the plot easier.
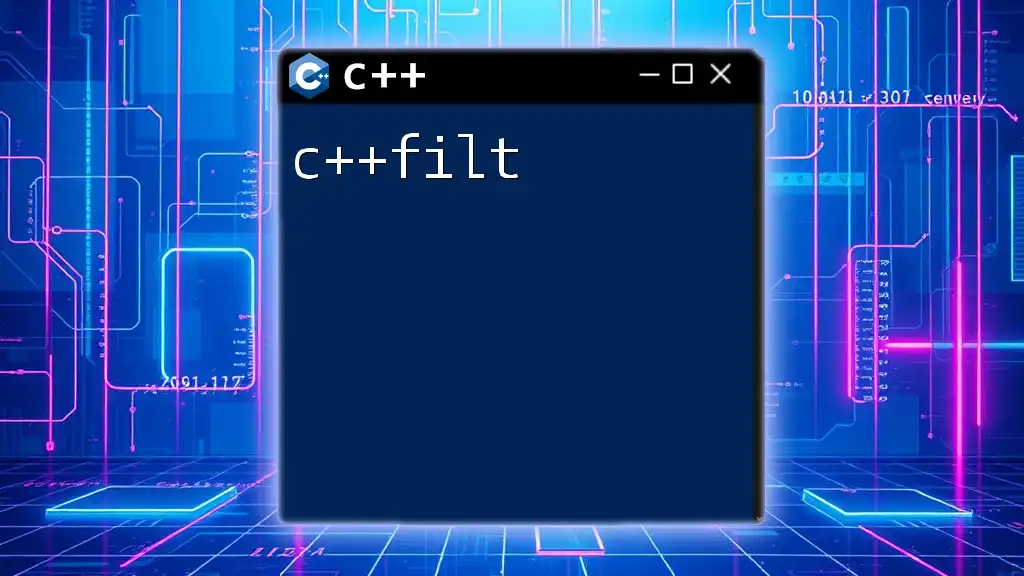
Creating Your First Plot in C++
Writing a Simple Line Plot
Creating a simple line plot is quite straightforward in C++. Below is a sample code that demonstrates how to create a basic line plot using the Matplotlib C++ library:
#include <iostream>
#include <matplotlibcpp.h>
namespace plt = matplotlibcpp;
int main() {
std::vector<int> x = {1, 2, 3, 4, 5};
std::vector<int> y = {1, 4, 9, 16, 25};
plt::plot(x, y);
plt::title("Simple Line Plot");
plt::xlabel("X Axis");
plt::ylabel("Y Axis");
plt::show();
return 0;
}
This code snippet creates a simple line plot that represents the square of each x value on the y-axis. In the code:
- The `plot()` function takes two vectors, x and y, to define plot points.
- You can customize the title and axis labels using `title()`, `xlabel()`, and `ylabel()`.
Customizing Your Plots
Enhancing your plot’s visual appearance is crucial for better comprehension. You can add titles, labels, and legends:
plt::title("Customized Plot Title");
plt::xlabel("Custom X Label");
plt::ylabel("Custom Y Label");
plt::legend();
Introducing these elements ensures clarity in your data presentation.
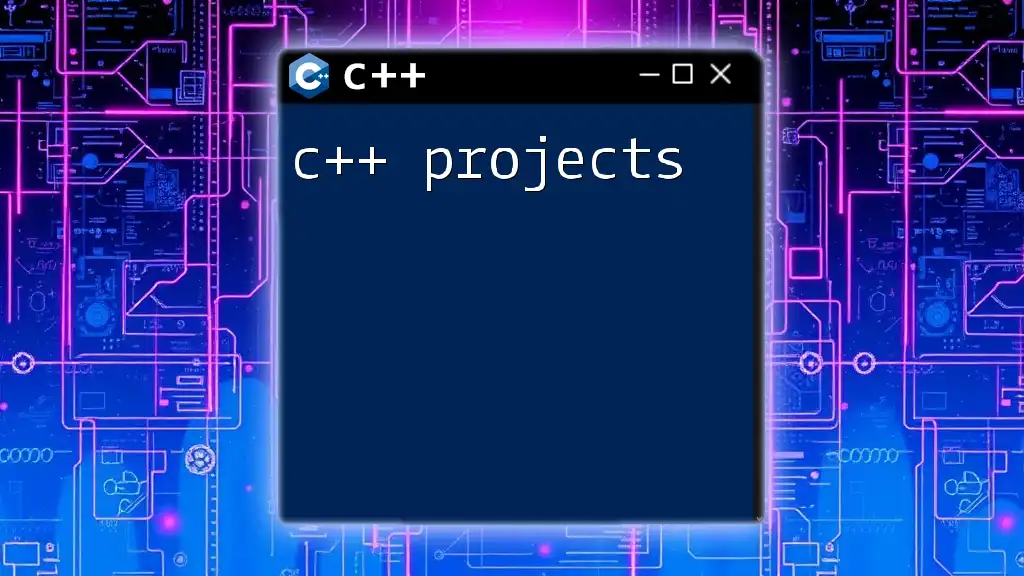
Advanced Plotting Techniques
Adding Multiple Datasets to a Single Plot
Overlaying multiple datasets can provide comparative insights. Here's how you can achieve this in C++:
plt::plot(x, y, "r-"); // Red line for y
plt::plot(x, /* Another dataset */, "b--"); // Blue dashed line for second dataset
Creating Subplots
If you want to display different plots side by side, you can create subplots. For instance:
plt::subplot(2, 1, 1);
plt::plot(x, y);
plt::subplot(2, 1, 2);
plt::plot(x, /* second dataset */);
This approach allows for effective comparisons across multiple datasets.
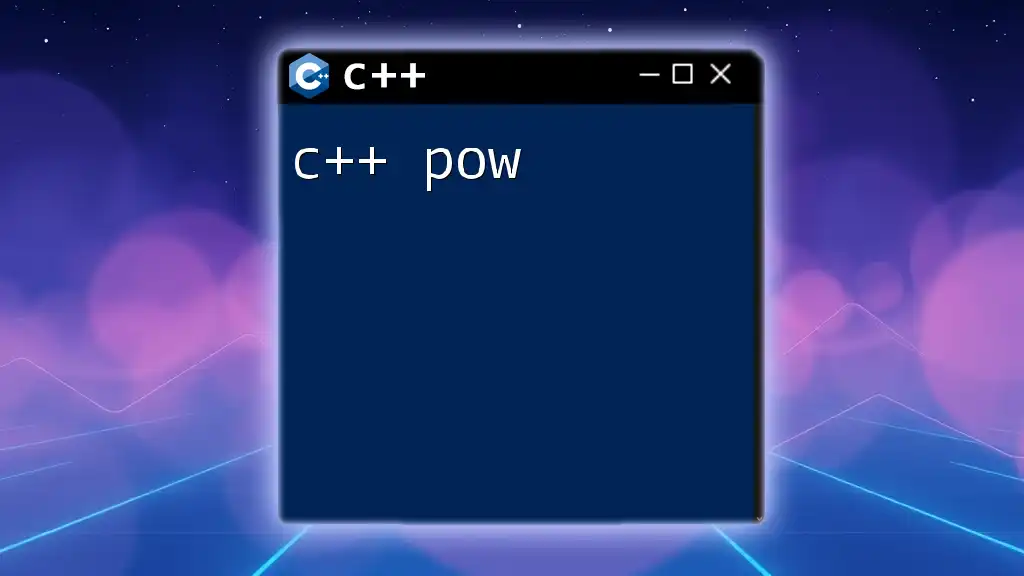
Handling Data for Plotting
Importing Data from Files
Plotting often requires data manipulation. C++ allows for reading datasets from various file formats such as CSV or TXT files. Use libraries like fstream to read your data efficiently.
Preprocessing Data for Visualization
Data often requires cleaning before visualization. This may include handling missing values, outliers, or scaling features. Preprocessed data leads to more meaningful plots.
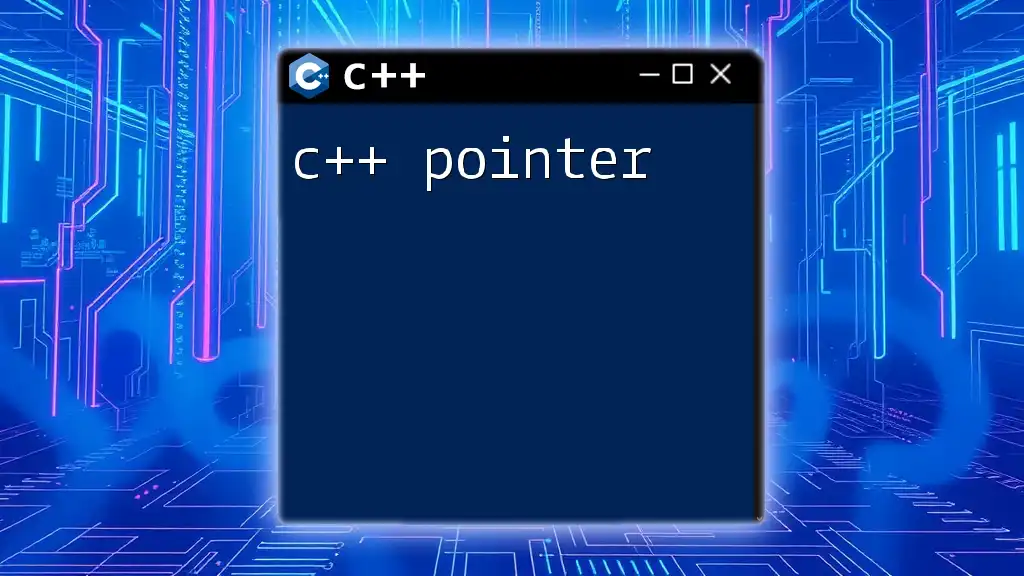
Tips for Effective Data Visualization in C++
Best Practices for Plot Design
To create informative plots:
- Maintain clarity: Avoid cluttering your plot with too much information.
- Utilize contrasting colors: Choose color schemes that are easy to distinguish.
- Filter unnecessary details: Focus on critical insights.
Common Mistakes to Avoid
- Ignoring data scaling: Ensure proper scaling for axes to avoid misleading representations.
- Overloading information: Limit the number of datasets in a single plot to maintain clarity.
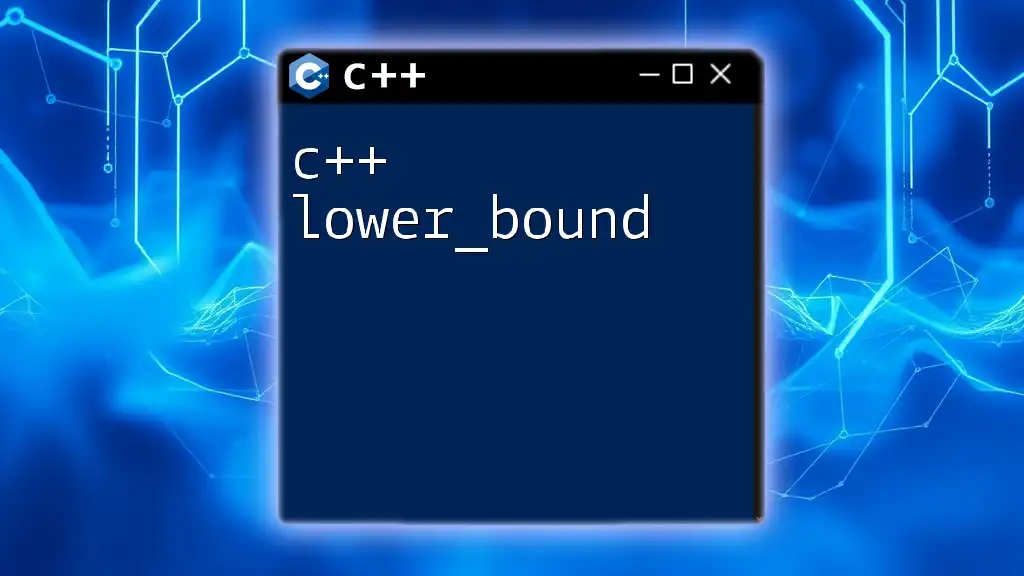
Conclusion
Recap of Key Takeaways
In this guide, you learned about the fundamentals of creating effective C++ plots, from setting up your environment to writing and customizing your plots, as well as advanced techniques for data visualization.
Next Steps for Aspiring C++ Plotters
As you gain confidence with basic plots, explore more complex data visualizations and experiment with different libraries. Engaging with the C++ community and seeking additional resources will significantly enhance your plotting skills.
Call to Action
Start plotting your data today! Experiment with different datasets and visualization techniques. Don’t forget to share your results with others to inspire improvement and learning in C++.