In C++, `std::iota` is a function that fills a range with sequentially increasing values, starting from a specified initial value.
#include <iostream>
#include <numeric>
#include <vector>
int main() {
std::vector<int> vec(5);
std::iota(vec.begin(), vec.end(), 10); // Fills vec with 10, 11, 12, 13, 14
for (int n : vec) {
std::cout << n << ' ';
}
return 0;
}
What is Iota in C++?
The iota function in C++ is a powerful tool provided by the Standard Template Library (STL) that simplifies the process of generating consecutive sequences of values. By utilizing the `<numeric>` header, this function enables programmers to efficiently fill ranges with sequentially increasing values, making it easier to structure data without manual effort.
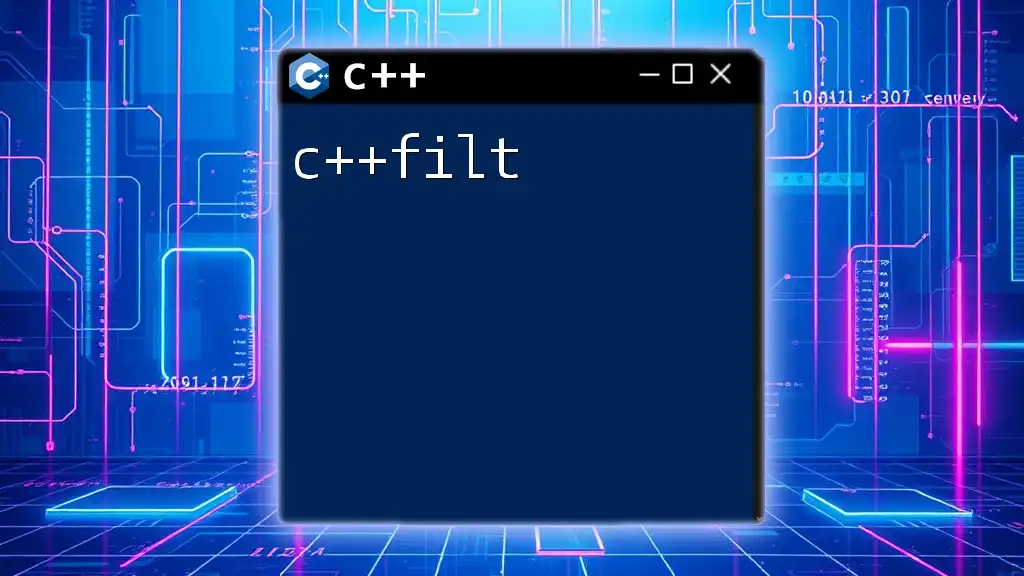
Why Use Iota?
There are several compelling reasons to use iota in your C++ programs:
- Simplicity: It provides a concise way to populate containers with sequences, eliminating the need for loops.
- Efficiency: Compared to traditional methods of filling arrays or vectors, iota is often more resource-efficient, as it directly operates on iterators.
- Readability: By using iota, the intent of your code becomes clearer. Instead of writing complex initialization loops, a single line of code captures the action.
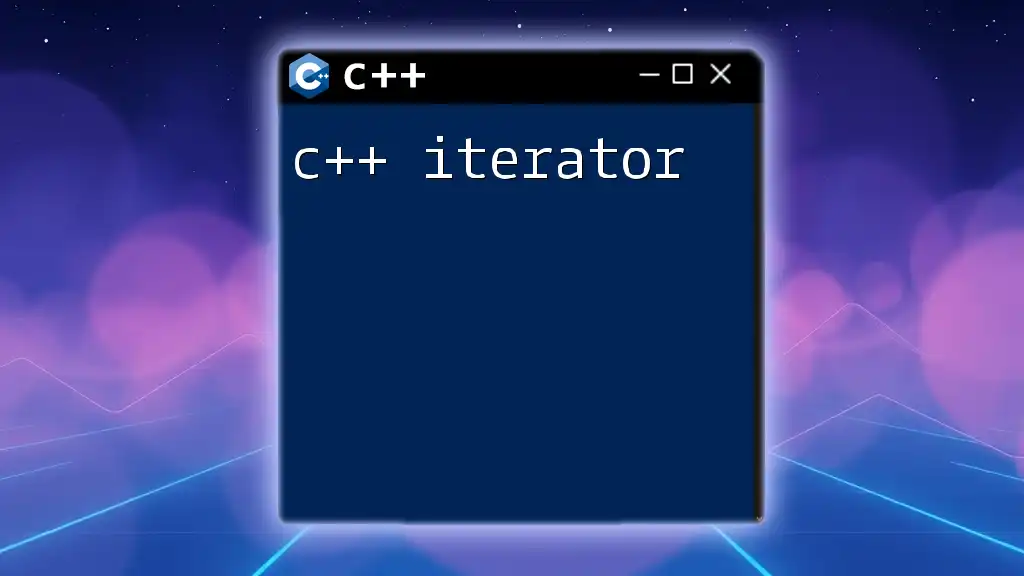
Setting Up Your Environment
To get started with C++ iota, ensure you have a compatible C++ compiler installed:
Installation Requirements
Make sure you have one of the following compilers installed on your machine:
- GCC: Version 5.0 or later
- Clang: Version 3.4 or later
- MSVC: Visual Studio 2015 or later
Additionally, it’s essential to configure your development environment effectively. Popular IDEs like Visual Studio and Code::Blocks typically handle this without manual intervention, but ensure that your project settings accommodate C++11 or higher, as iota was introduced in C++11.
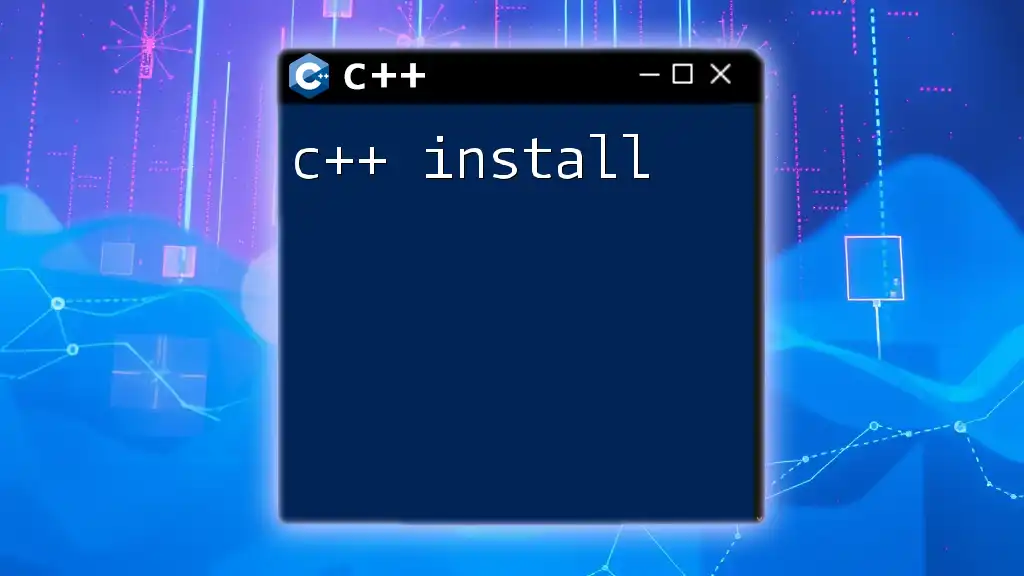
Understanding the Iota Function
Syntax of Iota
The basic syntax of the iota function is straightforward. In a code snippet:
#include <numeric>
#include <vector>
std::vector<int> vec(10);
std::iota(vec.begin(), vec.end(), 1);
Explanation of Parameters:
- `vec.begin()`: This points to the starting iterator of the vector where filling begins.
- `vec.end()`: This marks the end of the range where values are filled.
- `1`: This is the initial value from where the sequential filling starts.
How Iota Works
iota simplifies the process of filling containers by automatically incrementing the initialized value for each subsequent element. When you call `std::iota(vec.begin(), vec.end(), 1)`, it fills the vector `vec` with values starting from 1 up to the size of the vector. Each element in the range receives its value sequentially, computed as `initial_value + index`.
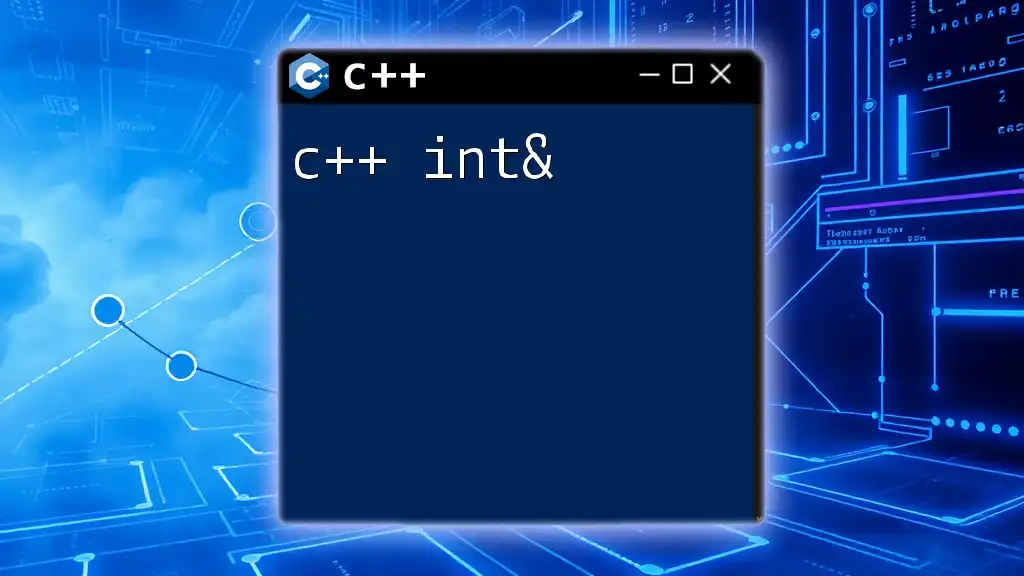
Using Iota with Different Containers
Iota with Vectors
One of the most common applications of iota is initializing a vector. For example:
#include <iostream>
#include <numeric>
#include <vector>
int main() {
std::vector<int> vec(5);
std::iota(vec.begin(), vec.end(), 10);
for (int num : vec) std::cout << num << " "; // Outputs: 10 11 12 13 14
return 0;
}
In this example, a vector of size 5 is filled with integers starting from 10. Each subsequent value is automatically incremented by 1.
Iota with Arrays
iota can also be applied to raw arrays, though there are certain limitations. Consider this example:
#include <iostream>
#include <numeric>
int main() {
int arr[5];
std::iota(arr, arr + 5, 1);
for (int num : arr) std::cout << num << " "; // Outputs: 1 2 3 4 5
return 0;
}
In this case, the iota function fills a raw array. However, since raw arrays do not provide iterators directly, you must specify the start and end pointers explicitly.
Iota with Other STL Containers
While vectors and arrays are common, iota works seamlessly with other STL containers, too. For instance, using iota with a list can be illustrated as follows:
#include <iostream>
#include <numeric>
#include <list>
int main() {
std::list<int> myList(5);
std::iota(myList.begin(), myList.end(), 1);
for (const auto &num : myList) std::cout << num << " "; // Outputs: 1 2 3 4 5
return 0;
}
The same approach works with deques or other iterable containers, demonstrating the versatility of iota across different types.
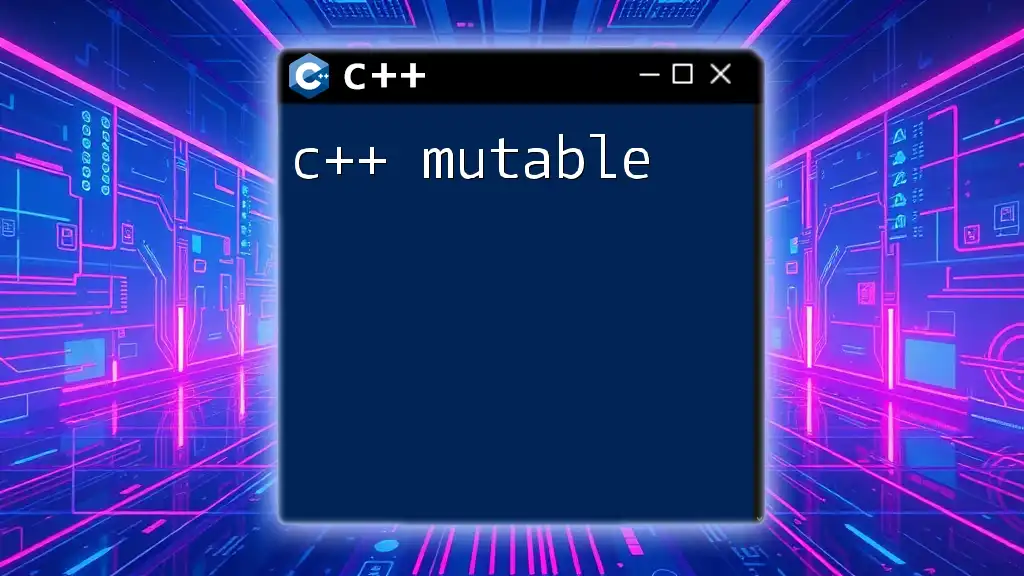
Practical Examples of C++ Iota
Filling a Range of Integers
Using iota to fill a range of integers is one of its simplest and most effective applications. For instance, to generate a sequence of integers from 1 to 10, you can write:
#include <iostream>
#include <numeric>
#include <vector>
int main() {
std::vector<int> vec(10);
std::iota(vec.begin(), vec.end(), 1);
// Outputs the generated sequence
for (int num : vec) std::cout << num << " "; // 1 2 3 4 5 6 7 8 9 10
return 0;
}
This establishes a clean and efficient method of filling a container with sequential integers.
Generating Custom Sequences
The initial value in iota can be customized to generate different sequences. For example, if you wish to initialize a vector starting from 5, you can do the following:
#include <iostream>
#include <numeric>
#include <vector>
int main() {
std::vector<int> vec(5);
std::iota(vec.begin(), vec.end(), 5);
for (int num : vec) std::cout << num << " "; // Outputs: 5 6 7 8 9
return 0;
}
By modifying the initial value, you obtain a sequence that fits specific needs, which may be especially useful in simulations or data generation.
Advanced Use Case: Filling with Complex Numbers
iota's versatility extends beyond primitive types. Consider using it to initialize a vector of complex numbers:
#include <iostream>
#include <numeric>
#include <vector>
#include <complex>
int main() {
std::vector<std::complex<double>> vec(5);
std::iota(vec.begin(), vec.end(), std::complex<double>(1.0, 1.0));
for (const auto &num : vec) std::cout << num << " "; // Outputs: (1,1) (2,1) (3,1) ...
return 0;
}
In this scenario, iota continues to function effectively by constructing a sequence of complex numbers by establishing an increment in the real part while keeping the imaginary part constant.
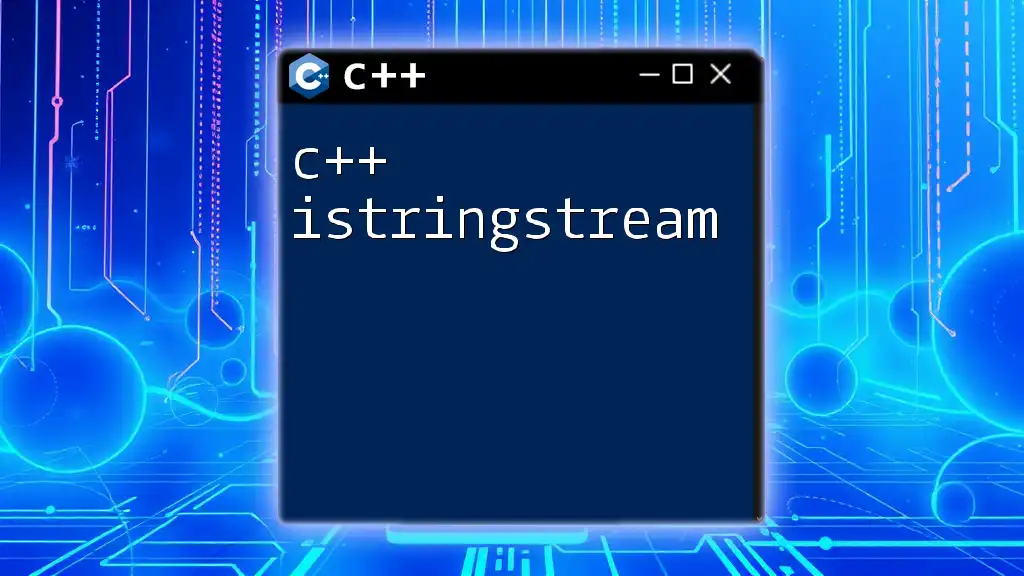
Common Mistakes and How to Avoid Them
Forget to Include `<numeric>`
A frequent issue developers encounter is failing to include the `<numeric>` header before using iota. This oversight results in compilation errors indicating that the function is undefined. Always ensure that you include the necessary headers to avoid such pitfalls.
Incorrect Iterator Types
Users sometimes confuse iterator types, particularly when using raw arrays compared to STL containers. Ensure you are using the correct iterator methods (`begin()` and `end()`) for containers and correct pointer syntax for raw arrays.
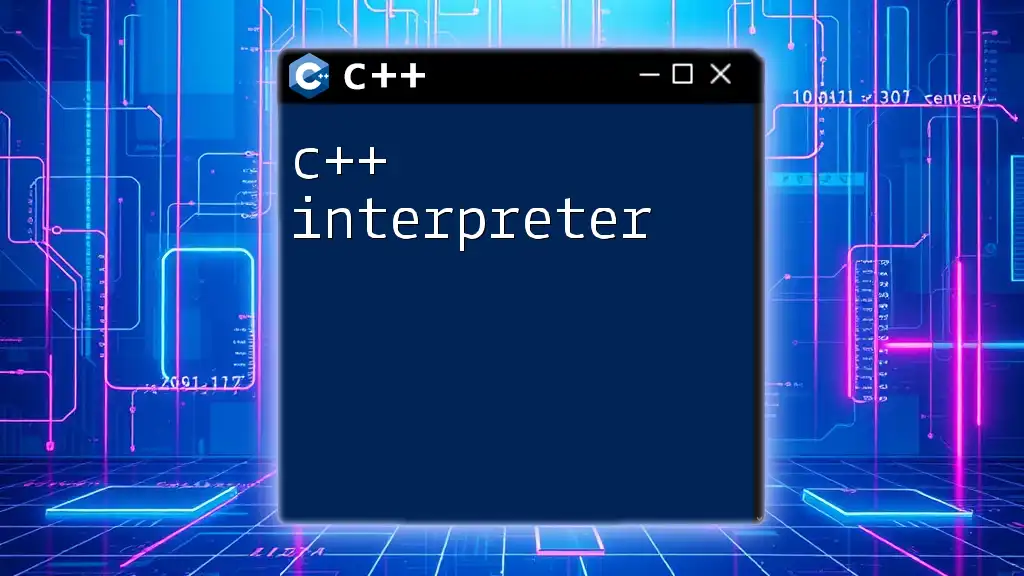
Performance Considerations
Efficiency of Iota
When examining the efficiency of iota, performance comparisons with manual filling methods reveal that iota is often faster and requires less boilerplate code. Using a loop to fill a container can lead to longer and less readable code, whereas iota does the same job in one concise statement.
Memory Considerations
While iota itself is efficient in terms of memory usage, awareness of temporary storage pop-ups can help prevent unnecessary overhead. It does not require additional storage other than the container itself, which leads to better memory management in the context of your entire program.
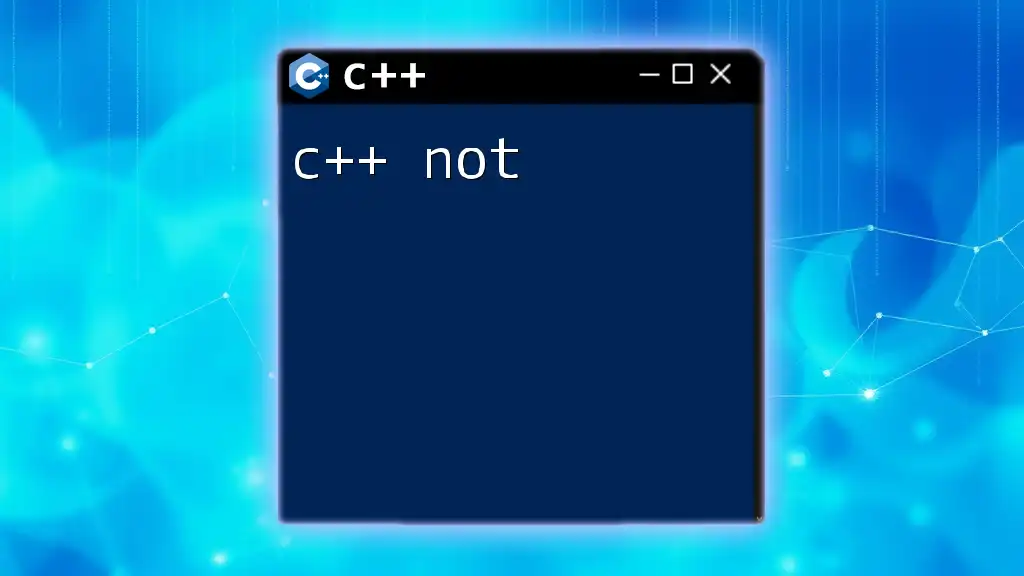
Recap on the Benefits of Using Iota
In summary, iota offers a simple, efficient, and clear approach to populating containers with sequential values. It minimizes code clutter while maximizing performance and readability, establishing it as a go-to method for C++ developers.
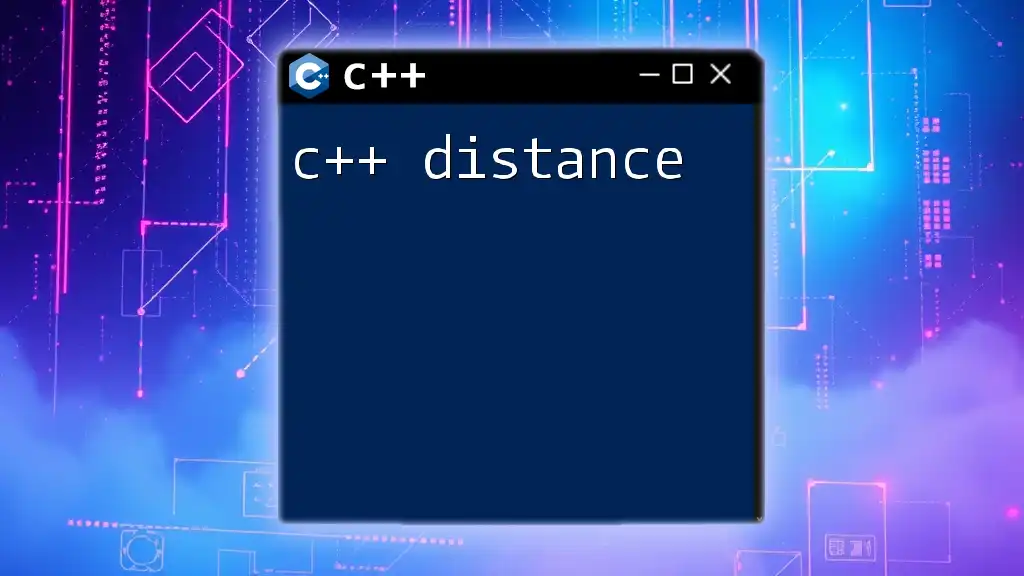
Encouragement to Experiment with Iota
As you delve deeper into C++, consider experimenting with iota in your own projects. Challenge yourself to find creative uses that can simplify your code, enhance overall performance, and improve the clarity of your programming approach.
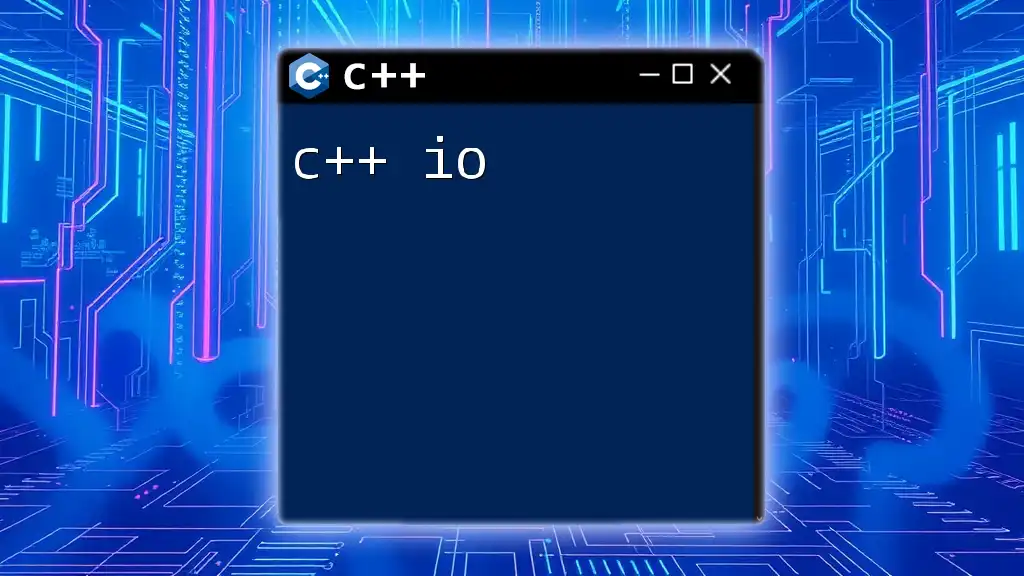
Further Reading and Resources
Official C++ Documentation
For comprehensive details and updates on the iota function, consult the official C++ documentation, which serves as a valuable resource.
Recommended Books and Tutorials
To expand your knowledge of C++ and the STL, consider exploring recommended books and online tutorials, which provide in-depth insights and practical exercises.
By understanding and implementing c++ iota, you can take significant strides towards writing cleaner, more efficient code in your programming endeavors.