The `not` operator in C++ is a logical NOT operator that inverts the value of a boolean expression.
#include <iostream>
int main() {
bool condition = true;
if (not condition) {
std::cout << "Condition is false!" << std::endl;
} else {
std::cout << "Condition is true!" << std::endl;
}
return 0;
}
Understanding the Logical Operator
What is a Logical Operator?
Logical operators are fundamental components of programming that allow you to control the flow of logic within your code. They enable decision-making based on boolean values—true or false. In C++, logical operators include `and`, `or`, and `not`, among others. These operators perform logical operations on operands and yield boolean results, which determine the execution of code segments.
The `not` Operator in C++
In C++, the `not` operator serves as a logical negation tool. It returns the opposite boolean value of the operand. This operator is a part of C++'s extensive list of operators and can be used as a more human-readable alternative to the traditional `!` operator, which also negates boolean values. While both achieve the same outcome, using `not` can enhance the clarity of your code and make it more expressive.
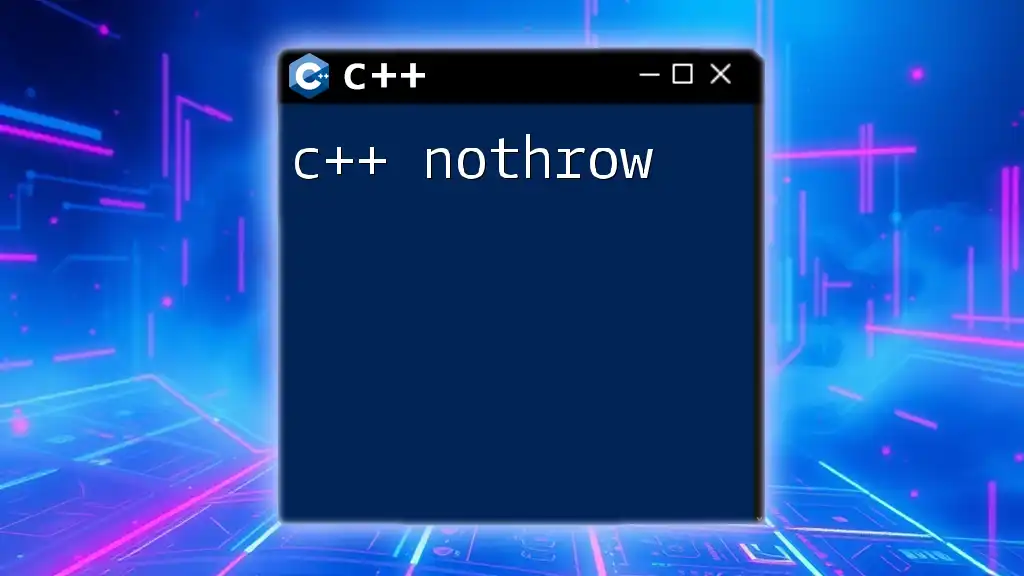
Syntax of the `not` Operator
Using the `not` operator in C++ is straightforward. Here’s the basic syntax:
bool condition = true;
if (not condition) {
// code to execute if condition is false
}
In this example, the code within the `if` statement executes only if `condition` is false. This is functionally identical to using the `!` operator:
if (!condition) {
// code to execute if condition is false
}
It’s crucial to understand that both forms are interchangeable. However, the `not` operator can make the intent of your code clearer at a glance, especially for beginners or when sharing code with team members.
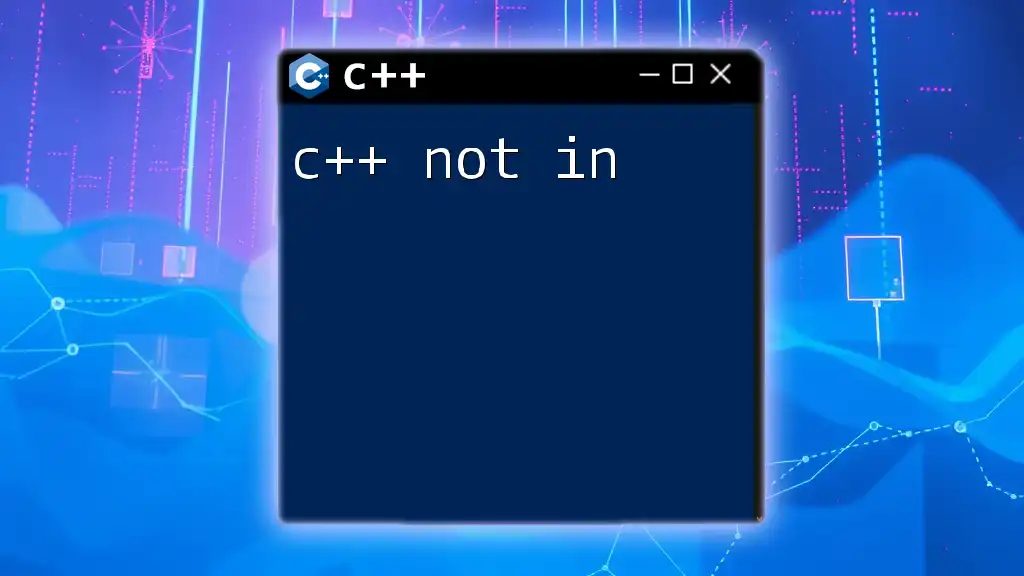
Practical Applications of the `not` Operator
Use Cases in Conditional Statements
The `not` operator excels in conditional statements, where its usage can improve clarity. For instance:
if (not condition) {
std::cout << "Condition is false." << std::endl;
}
In this code, it's easy to grasp that the message indicates a false condition. This can be especially useful in scenarios with complex logical expressions.
Combining Logical Operators
Using `not` with Other Logical Operators
You can seamlessly combine `not` with other logical operators like `and` and `or`. Here's how:
bool x = true;
bool y = false;
if (not (x and y)) {
// code for when either x or y is false
}
In this case, the `if` statement checks if not both `x` and `y` are true. If either is false, the code executes.
Consider this expanded example:
if (not (x || y)) {
// code for when both x and y are false
}
Here, the outcome occurs only when both conditions yield false, showcasing the versatility of the `not` operator in handling various logical expressions with ease.
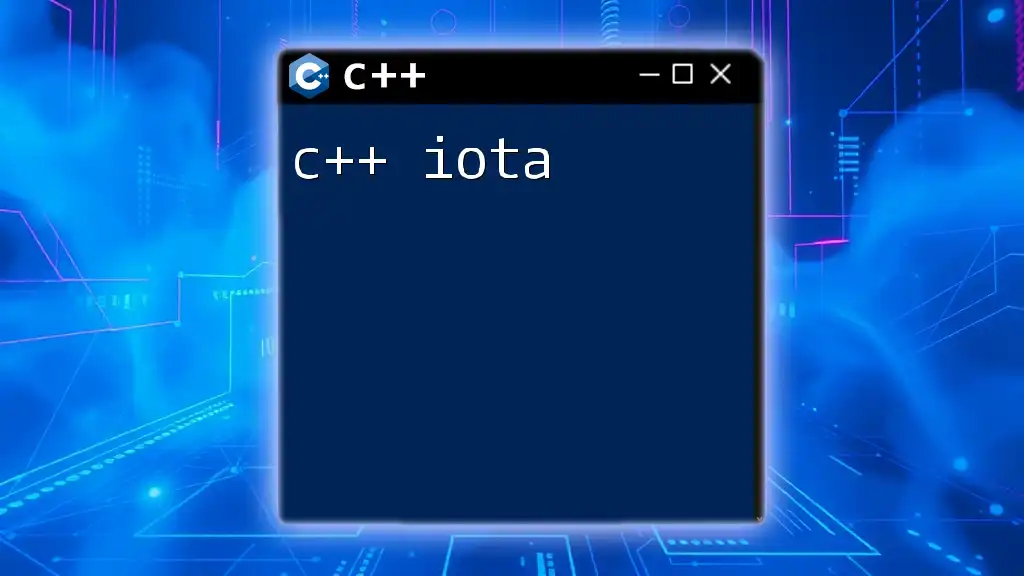
Using `not` in Loops
Application in Control Flow
The `not` operator can also be effectively utilized within loops, particularly to enhance readability. For example:
for (int i = 0; i < 10; ++i) {
if (not (i % 2 == 0)) {
std::cout << i << " is odd." << std::endl;
}
}
In this loop, the `not` operator emphasizes that the code block executes only for odd numbers. This kind of clarity can aid in understanding the logic at a glance, especially when revisiting code after a long time.
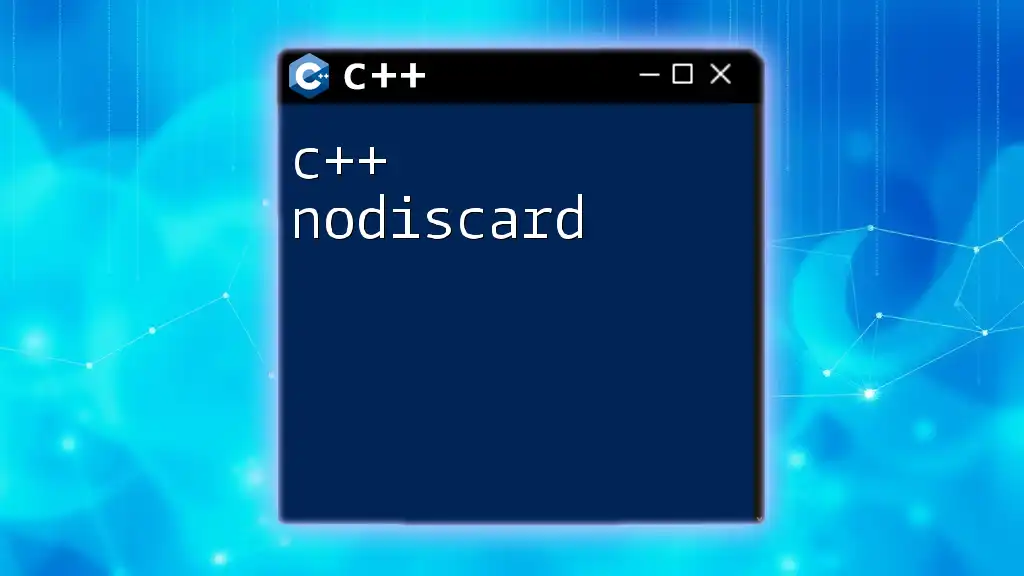
Best Practices for Using the `not` Operator
When to Use `not`
As a best practice, consider using the `not` operator in scenarios where clarity is paramount. The readability it offers is valuable in collaborative coding environments or when teaching new programmers. Here’s a notable illustration:
if (not condition) {
// Suggests a clear interpretation
}
Code Readability and Maintainability
Consistency in coding style is vital, especially within teams. By adopting the `not` operator where appropriate, teams can develop a unified approach that enhances maintainability. When colleagues see the `not` keyword, they immediately understand that negation is occurring, minimizing the cognitive load required to interpret the logic.
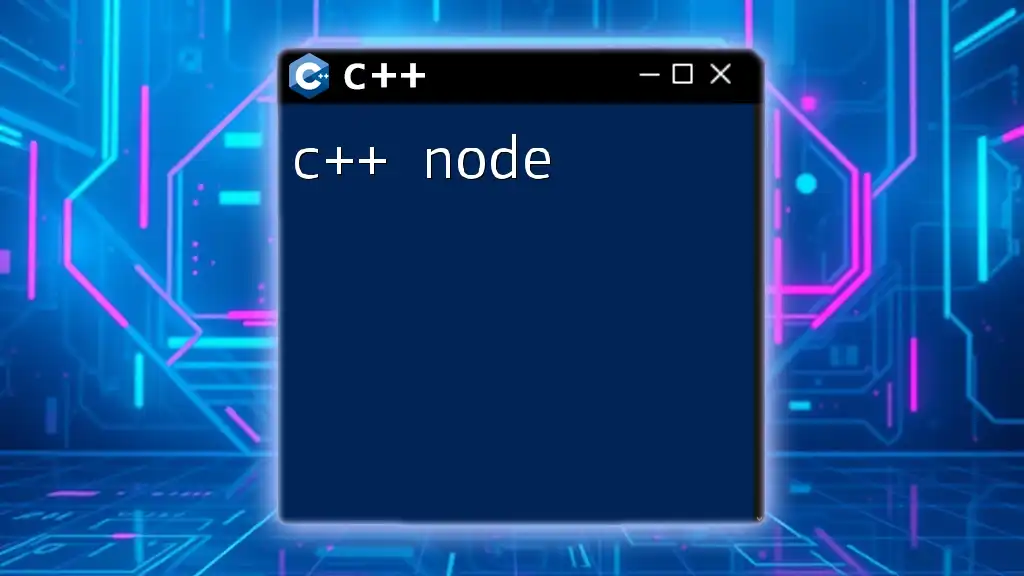
Potential Pitfalls
Misunderstandings Around the `not` Operator
While the `not` operator is straightforward, it can lead to misunderstandings if misused. One common mistake occurs when combining it with equality checks. For instance:
if (not x == true) {
// This can be confusing; it means x is false.
}
This statement may be difficult to parse, potentially leading to logic errors. Instead, consider restructuring the expression to enhance clarity.
Debugging Tips
When debugging issues related to the `not` operator, validating boolean expressions through print statements can provide insight. For example, if your `if` statement isn't behaving as expected, adding a print statement before it can help clarify the condition's value:
std::cout << "Condition (before not): " << condition << std::endl;
if (not condition) {
std::cout << "Condition is false." << std::endl;
}
This way, you can verify the actual boolean value of `condition` before applying the `not` operator, aiding in troubleshooting.
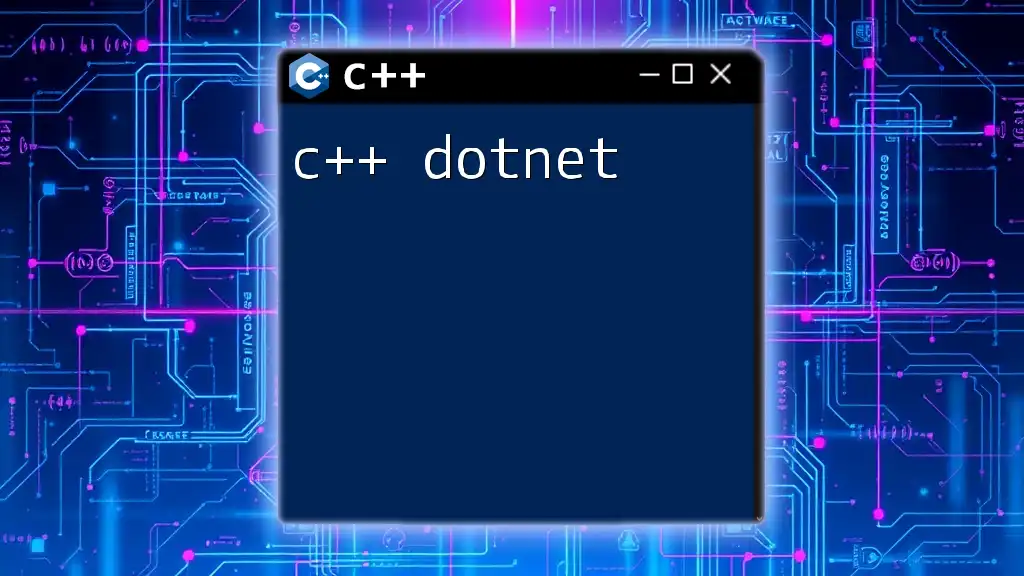
Conclusion
Recapping the usages of the `not` operator in C++, we've explored its syntax, practical applications in control flow, and best practices. The `not` operator enhances the expressiveness of your code, leading to greater readability and maintainability. By understanding its implications and applications, programmers can write more coherent and understandable code, benefiting not only themselves but their teams as well.
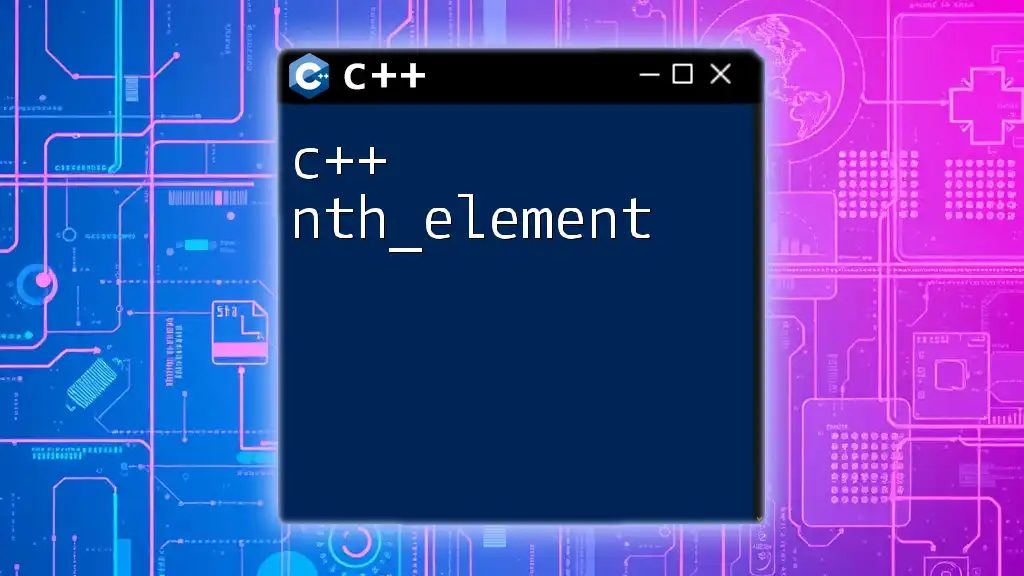
Additional Resources
For further exploration of C++ logical operators, refer to authoritative documentation and online coding platforms that reinforce these essential concepts. Engaging with these resources will solidify your understanding of `c++ not` and enhance your overall programming proficiency.