C++ threads allow you to run multiple tasks concurrently in a program, improving efficiency and performance by utilizing multi-core processors.
#include <iostream>
#include <thread>
void task() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(task); // Create a thread that runs the task function
t.join(); // Wait for the thread to finish
return 0;
}
What is a thread in C++?
A thread in C++ is defined as the smallest sequence of programmed instructions that can be managed independently by the scheduler. It represents a path of execution within a program. Understanding threads is crucial for developing concurrent applications, allowing for multiple operations to run simultaneously rather than sequentially.
The significance of threading lies in its ability to improve the efficiency and responsiveness of applications, especially in scenarios that involve heavy computations or need to handle multiple tasks at once. A brief history indicates that C++ introduced threading with the C++11 standard, which included a threading library to simplify thread management.
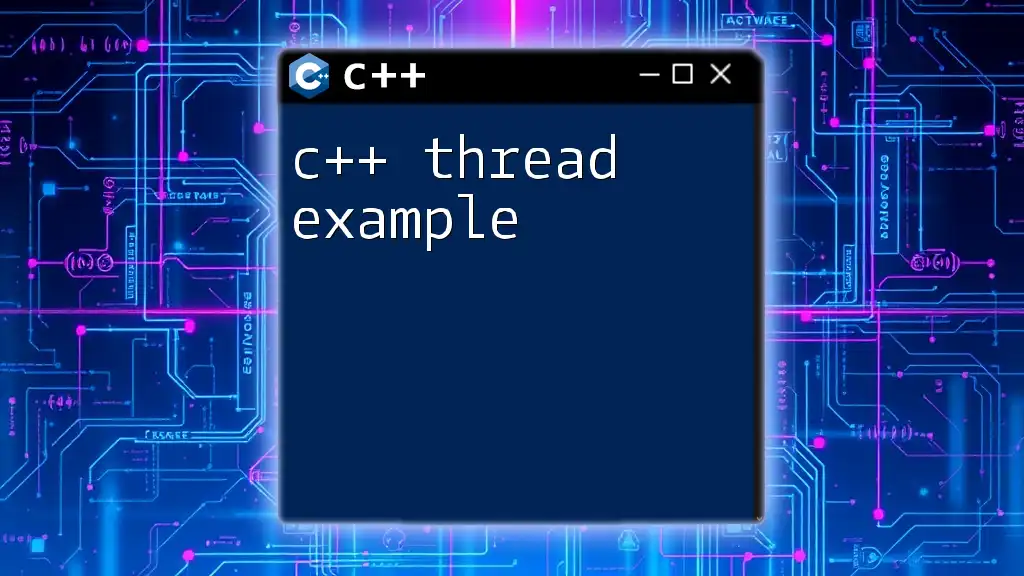
Why use threads in C++?
Using threads in C++ offers numerous advantages, such as:
- Performance Improvements: Threads can help utilize the maximum potential of multi-core processors, significantly enhancing the performance of applications.
- Increased Responsiveness: By leveraging threads, applications can remain responsive to user input, as background tasks can run without interrupting the main application flow.
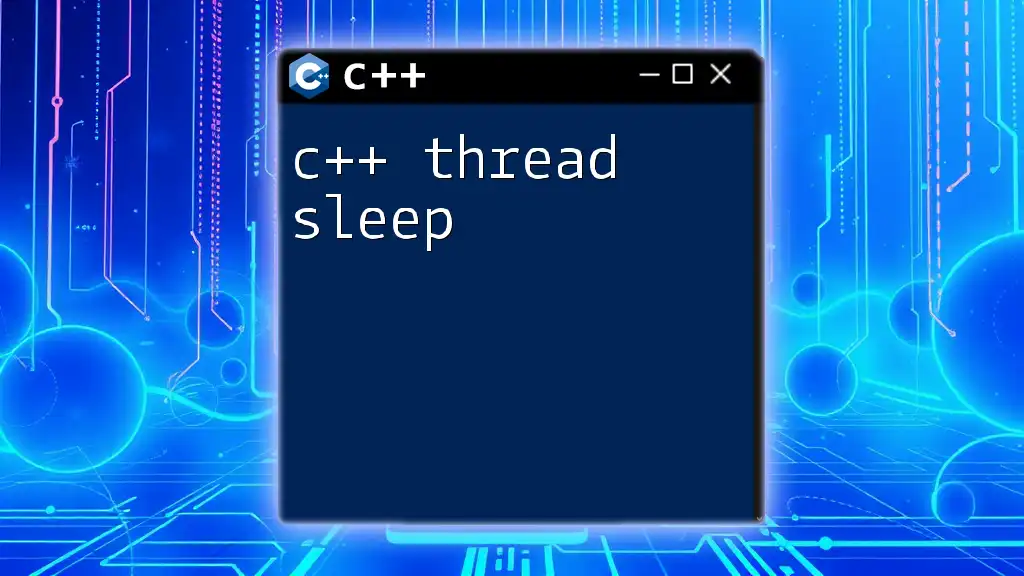
Understanding Threads in C++
What are threads?
Threads can be thought of as lightweight processes that share the same memory space. Unlike processes, which operate in isolated memory spaces with their own resources, threads share the resources of their parent process while having individual stacks.
How C++ implements threads
C++ provides a robust implementation of threading through the `<thread>` library introduced in C++11. This library facilitates thread creation, management, and synchronization, enabling developers to maximize their applications' performance through concurrent execution.
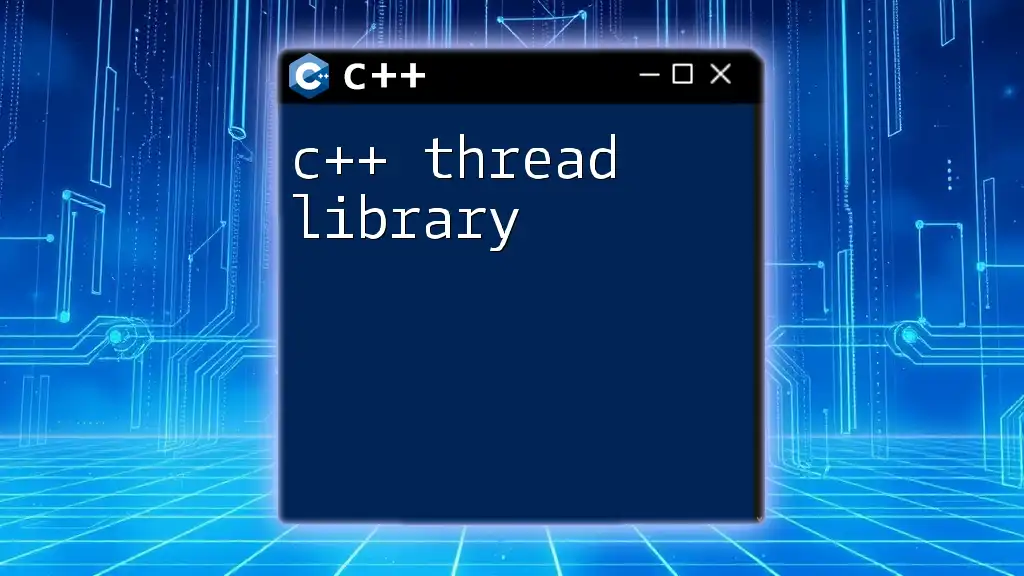
Getting Started with C++ Threads
Including the necessary headers
To work with threads in C++, you need to include the `<thread>` header file as well as the `<iostream>` library for input and output operations. The necessary header files can be included as follows:
#include <thread>
#include <iostream>
Creating your first thread in C++
Creating a thread in C++ is straightforward. Consider the following example that demonstrates how to create and execute a thread:
void function() {
std::cout << "Thread is running!" << std::endl;
}
int main() {
std::thread myThread(function);
myThread.join(); // Wait for the thread to finish
return 0;
}
In this example, we define a function that prints a message, create a thread that executes this function, and call `join()` to ensure that the main program waits for the thread to finish its task before exiting. Joining threads helps avoid undefined behavior caused by threads still executing during the program's termination.
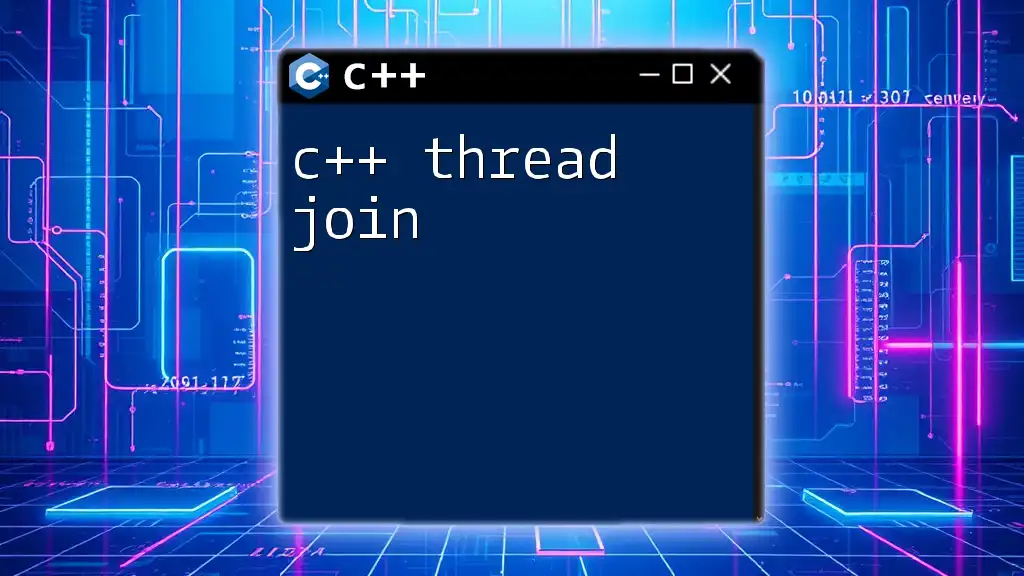
C++ Thread Lifecycle
States of a thread
Threads can exist in various states throughout their lifecycle, including:
- New: The thread has been created but not yet started.
- Runnable: The thread is eligible to be run but may be in a state waiting for CPU time.
- Blocked: The thread is waiting for a resource that is currently held by another thread.
- Terminated: The thread has completed its execution and can no longer be run.
Joining and Detaching Threads
Joining a thread means waiting for it to finish executing before continuing execution in the calling thread. In contrast, detaching a thread allows it to run independently. Here is how you can detach a thread:
std::thread t1(function);
t1.detach();
This approach allows the thread to run independently of the main thread, but it also means you won’t be able to join it later or retrieve its return value.
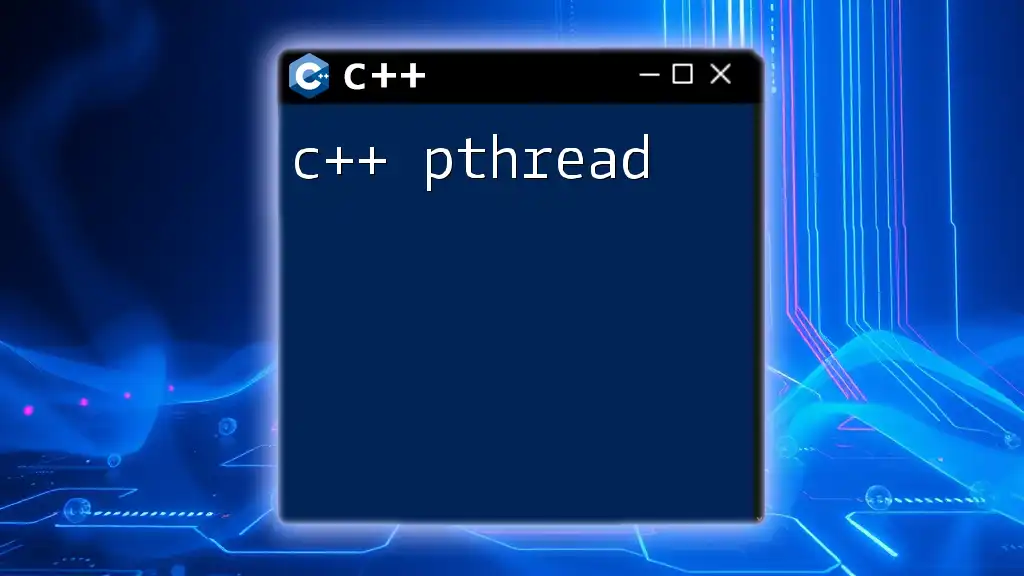
Synchronization in C++
Understanding race conditions
A race condition occurs when two or more threads attempt to modify shared data concurrently. This can lead to unpredictable results and hard-to-debug errors.
Mutexes and Locks
To protect shared resources, you can use mutexes. Mutexes ensure that only one thread can access a piece of shared data at a single time. Here’s how you can implement a mutex:
std::mutex mtx;
void threadFunction() {
mtx.lock(); // Lock the mutex
// Critical section
mtx.unlock(); // Unlock the mutex
}
In this code, before accessing the shared resource, a thread locks the mutex. Once the critical section is complete, it unlocks the mutex, allowing other threads access.
Using std::lock_guard
Utilizing `std::lock_guard` is a more efficient way to manage mutexes. It automatically handles locking and unlocking, ensuring that the mutex is released when the guard goes out of scope:
void threadFunction() {
std::lock_guard<std::mutex> lock(mtx);
// Protected code
}
This method simplifies code and reduces the risk of forgetting to unlock the mutex, making it a preferred choice in many cases.
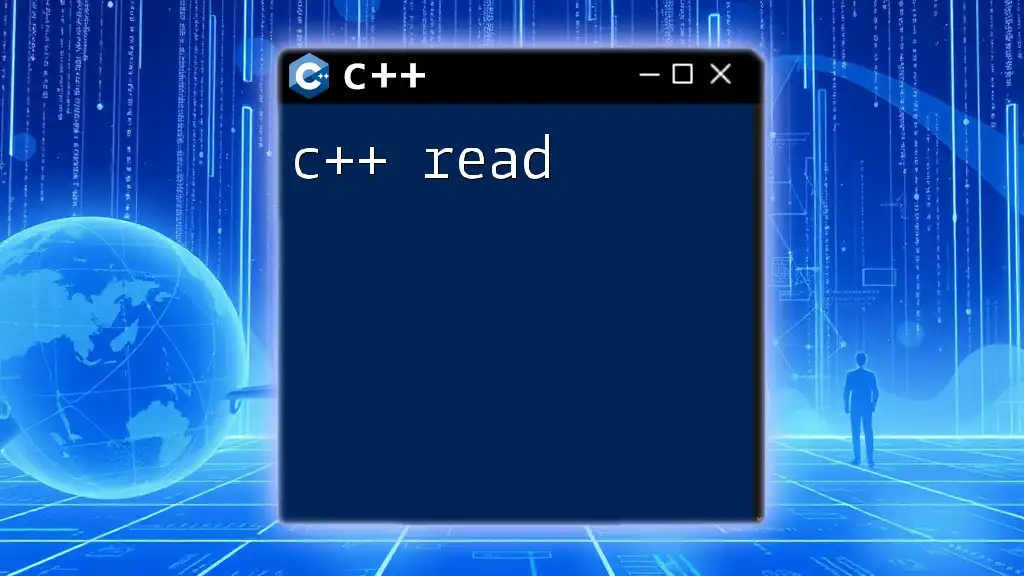
Thread Communication
Using Condition Variables
Condition variables facilitate communication between threads, allowing them to synchronize their actions based on certain conditions. Here's how to use condition variables effectively:
std::condition_variable cv;
void producer() {
// Produce data
cv.notify_one(); // Notify waiting threads
}
void consumer() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock); // Wait for notification
}
In this example, the producer thread can notify one waiting consumer thread when it has produced data, effectively coordinating their interaction.
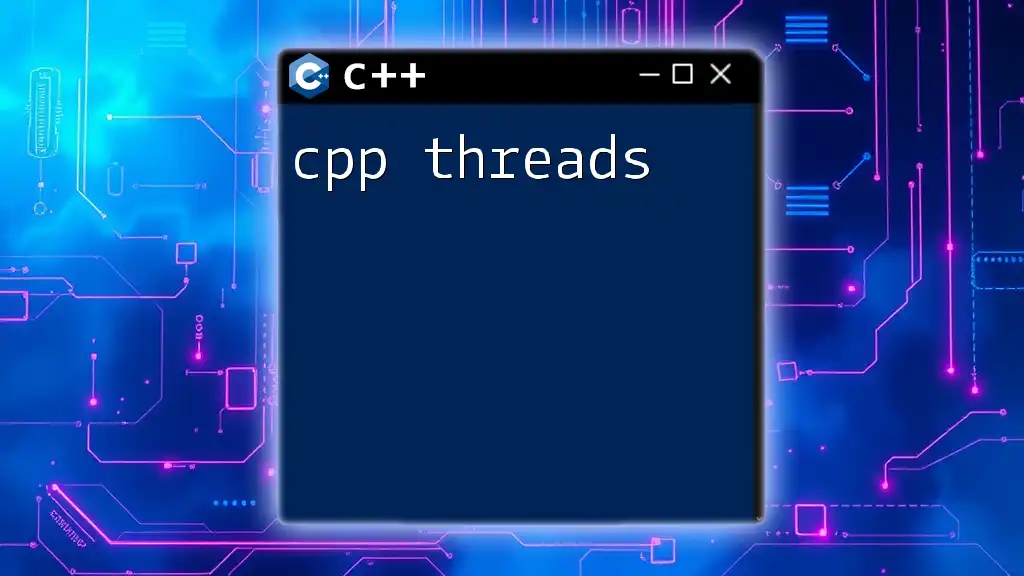
Advanced Threading Techniques
Thread Pools
Using a thread pool is a useful technique to manage multiple threads efficiently without the overhead of creating and destroying threads repeatedly. A thread pool maintains a set number of threads that can be reused for various tasks, improving application responsiveness and resource management.
Async and Future
C++ also supports asynchronous programming through `std::async` and `std::future`. This enables you to run potentially blocking operations asynchronously.
Example usage of `std::async`:
auto fut = std::async(std::launch::async, function);
fut.get(); // Retrieve the result
In this example, a task runs asynchronously in the background, allowing the main thread to continue executing until the result is needed.
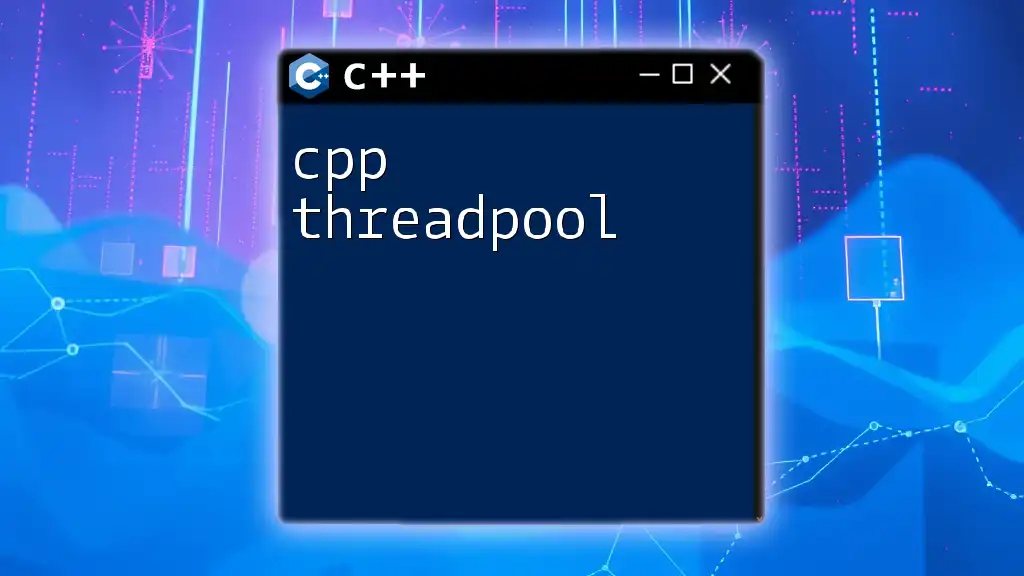
Best Practices for C++ Threading
To design efficient multithreaded applications, consider the following best practices:
- Keep threads lightweight: Minimize the work done in threads to improve context switching performance.
- Limit shared state: Reducing shared resources decreases the risk of contention and data corruption.
- Avoid thread contention: Manage how threads access resources to avoid delays caused by locked resources.
- Use RAII for managing resources: Utilizing Resource Acquisition Is Initialization (RAII) ensures that resources are managed automatically, reducing memory leaks and resource mismanagement.
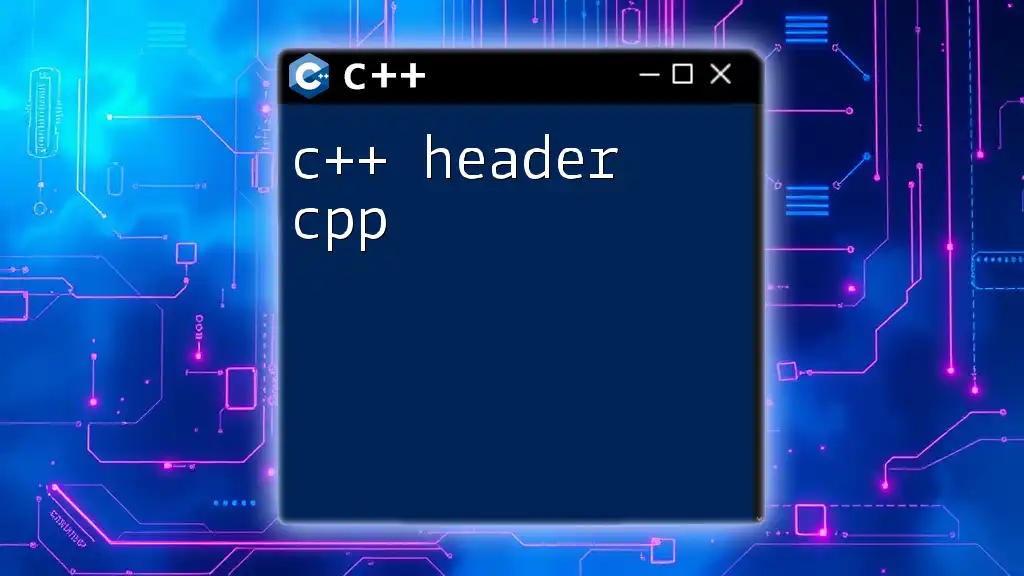
Debugging C++ Threads
Working with threads can lead to challenging issues such as race conditions, deadlocks, and livelocks. Recognizing common problems can lead to more effective debugging.
Common issues in multithreaded applications
- Race Conditions: Occurs when multiple threads modify shared data simultaneously.
- Deadlocks: Happens when two or more threads are blocking each other, waiting for resources to be released.
- Livelocks: Occurs when two or more threads continuously change states in response to each other without making progress.
Tools and techniques for debugging
Utilizing tools like Valgrind or Thread Sanitizer can significantly assist in identifying threading issues in your C++ applications, ensuring better stability and performance.
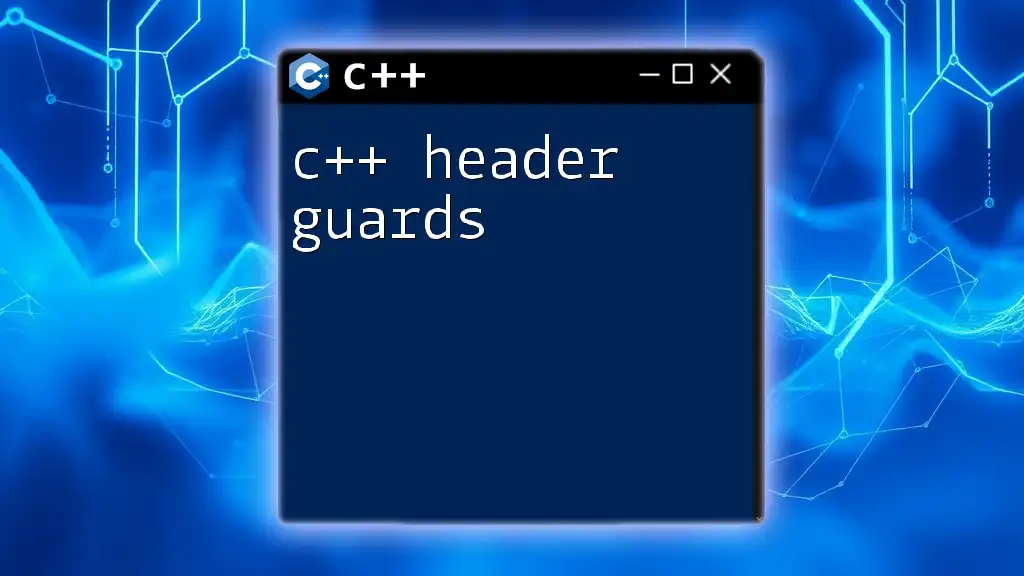
Conclusion
Understanding and effectively using c++ threads not only enhances application performance but also elevates user experience by allowing applications to handle multiple tasks simultaneously. By mastering threading concepts, synchronization techniques, and debugging strategies, developers can create robust and responsive applications that leverage the power of modern multi-core processors.
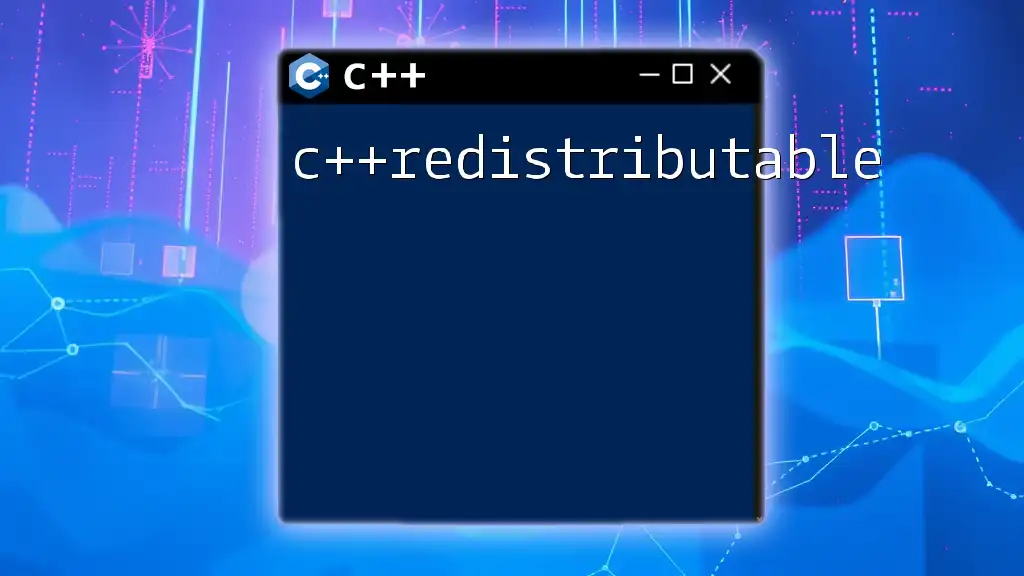
Additional Resources
Consider exploring additional books, blogs, and documentation on C++ threading to deepen your understanding. Furthermore, engaging in practical coding challenges can solidify your knowledge and skills in concurrent programming.