A C++ three-dimensional array is an array of arrays that allows you to store data in a three-dimensional grid format, making it useful for applications like mathematical modeling and 3D game development.
Here's a simple code snippet demonstrating the declaration and initialization of a three-dimensional array in C++:
#include <iostream>
int main() {
// Declare a 3D array
int arr[2][3][4] = {
{
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
},
{
{13, 14, 15, 16},
{17, 18, 19, 20},
{21, 22, 23, 24}
}
};
// Accessing an element
std::cout << arr[1][2][3]; // Outputs: 24
return 0;
}
Understanding Three Dimensional Arrays in C++
What is a Three Dimensional Array?
A three dimensional array in C++ is an array of arrays of arrays, allowing the storage of data in a three-dimensional space. Think of it as a cube where you can store values for each coordinate in three dimensions: width, height, and depth.
For example, a 3D array can be useful in situations such as storing RGB color values for pixels in an image, where each pixel is represented in a three-component format.
Syntax of Three Dimensional Arrays in C++
The syntax for declaring a c++ three dimensional array is straightforward. Here's how you can declare a 3D array:
data_type array_name[size1][size2][size3];
For example, if you want to create an integer 3D array with dimensions 4x5x6, your declaration would look like this:
int my3DArray[4][5][6];
When handling three dimensional arrays, it's essential to understand memory allocation. Multi-dimensional arrays are stored in contiguous memory. Thus, when you create an array like `my3DArray`, C++ allocates enough memory to hold all 120 integers (4x5x6).
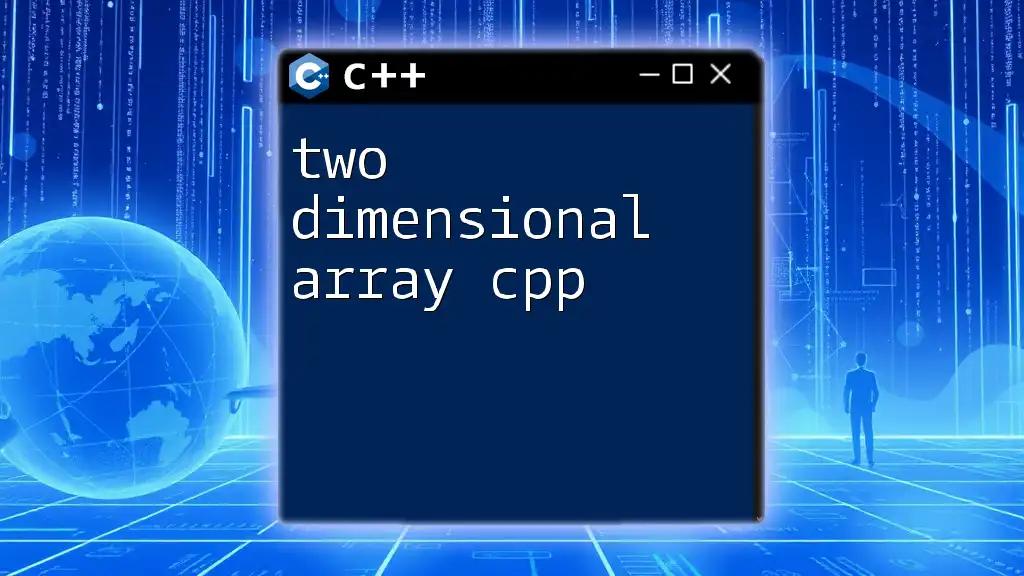
How to Access and Manipulate 3D Arrays in C++
Accessing Elements in a Three Dimensional Array
Accessing elements in a three dimensional array follows the standard indexing format. Each element is accessed via its coordinates inside the array:
array_name[i][j][k];
Here’s an example that demonstrates accessing and printing elements of a 3D array:
#include <iostream>
int main() {
int my3DArray[2][2][3] = {
{
{1, 2, 3},
{4, 5, 6}
},
{
{7, 8, 9},
{10, 11, 12}
}
};
std::cout << "Element at (1, 1, 2): " << my3DArray[1][1][2] << std::endl;
return 0;
}
In this example, the output will be `12`, demonstrating access through 3D coordinates.
Iterating Over a 3D Array
To manipulate every element within a three dimensional array, nested loops are utilized. Here is how you can iterate over and display a 3D array:
#include <iostream>
int main() {
int my3DArray[2][2][3] = {
{
{1, 2, 3},
{4, 5, 6}
},
{
{7, 8, 9},
{10, 11, 12}
}
};
for (int i = 0; i < 2; ++i) {
for (int j = 0; j < 2; ++j) {
for (int k = 0; k < 3; ++k) {
std::cout << "Element at (" << i << ", " << j << ", " << k << "): "
<< my3DArray[i][j][k] << std::endl;
}
}
}
return 0;
}
The nested loops allow you to explore every element in the three dimensional space and print its coordinates along with the value.
Modifying Elements in a Three Dimensional Array
Modifying elements can be done similarly to accessing them. Here’s how you can update specific elements inside a `3D array`.
#include <iostream>
int main() {
int my3DArray[2][2][3] = {
{
{1, 2, 3},
{4, 5, 6}
},
{
{7, 8, 9},
{10, 11, 12}
}
};
my3DArray[0][1][2] = 20; // Modifying the element at (0, 1, 2)
std::cout << "Updated Element at (0, 1, 2): " << my3DArray[0][1][2] << std::endl;
return 0;
}
In this example, the value at `(0, 1, 2)` is modified to `20`, illustrating how easily an element in a three dimensional array can be updated.
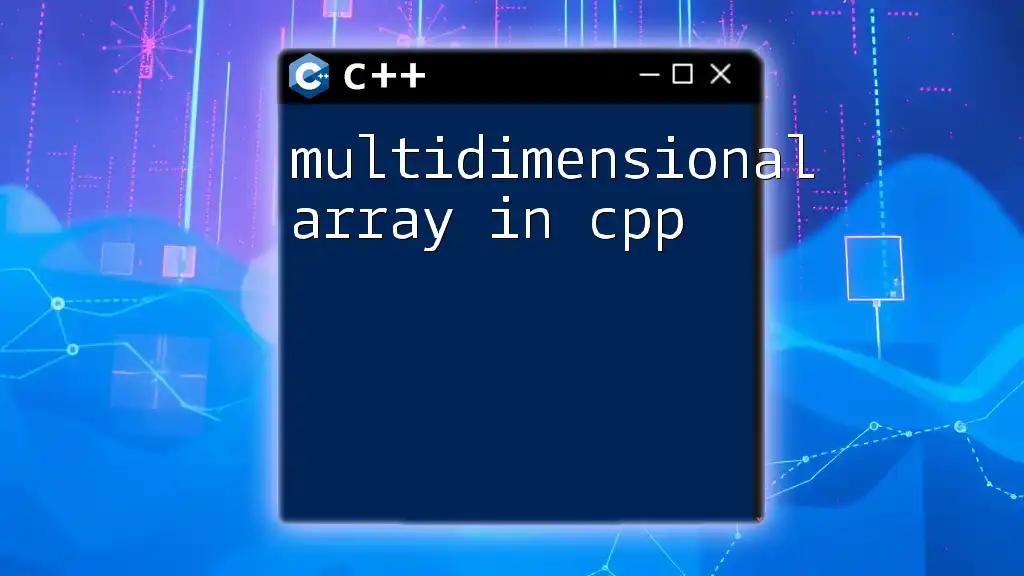
Common Operations on 3D Arrays in C++
Copying a 3D Array
Copying a three dimensional array involves accessing each element individually, particularly if it is a multi-dimensional static array. Here’s a simple technique to perform a copy:
void copy3DArray(int source[2][2][3], int destination[2][2][3]) {
for (int i = 0; i < 2; ++i) {
for (int j = 0; j < 2; ++j) {
for (int k = 0; k < 3; ++k) {
destination[i][j][k] = source[i][j][k];
}
}
}
}
This code snippet illustrates how to copy elements from one 3D array to another using nested loops.
Resizing a 3D Array
Unlike typical arrays, resizing a 3D array in C++ requires dynamic memory allocation. A common way to manage this is through pointers and `new` keyword. Here’s an example of resizing:
#include <iostream>
int*** create3DArray(int x, int y, int z) {
int*** array = new int**[x];
for(int i = 0; i < x; i++) {
array[i] = new int*[y];
for(int j = 0; j < y; j++) {
array[i][j] = new int[z];
}
}
return array;
}
This function dynamically allocates a 3D array based on the specified dimensions, allowing flexibility in usage.
Summing All Elements in a Three Dimensional Array
To perform summation across all elements in a 3D array, you can use a nested loop structure. Here’s a code snippet demonstrating this operation:
#include <iostream>
int main() {
int my3DArray[2][2][3] = {
{
{1, 2, 3},
{4, 5, 6}
},
{
{7, 8, 9},
{10, 11, 12}
}
};
int sum = 0;
for (int i = 0; i < 2; ++i) {
for (int j = 0; j < 2; ++j) {
for (int k = 0; k < 3; ++k) {
sum += my3DArray[i][j][k];
}
}
}
std::cout << "Sum of all elements: " << sum << std::endl;
return 0;
}
This will compute the total of all values in the 3D array, demonstrating a common operation in data manipulation.
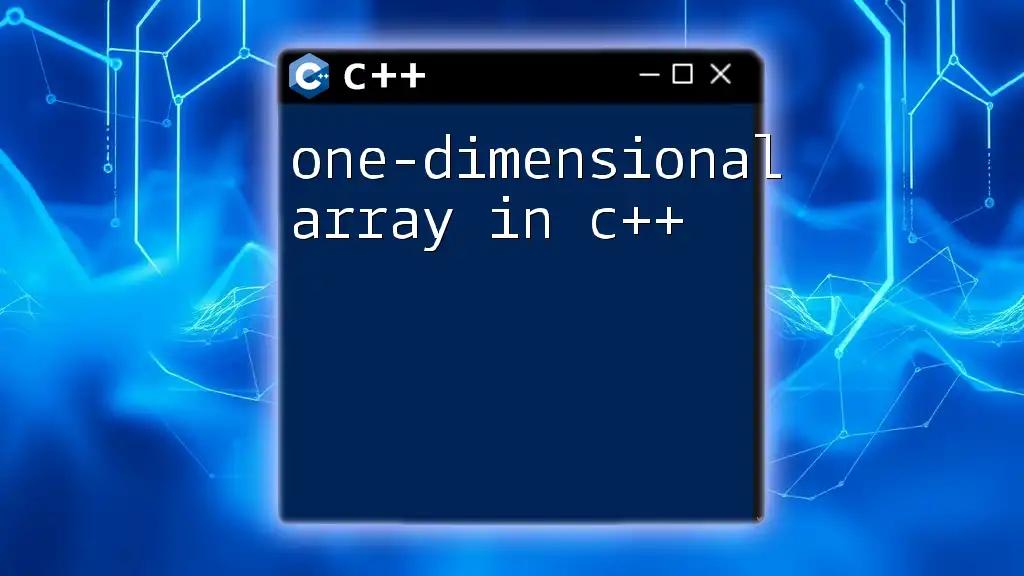
Practical Applications of Three Dimensional Arrays in C++
3D Game Development
In the realm of 3D game development, 3D arrays can serve as a framework for managing the state of a game world or level. For example, you can structure game elements like terrain or objects in a grid that utilizes height, width, and depth.
Here’s a code snippet outlining a simple grid data structure for a game level:
struct GameLevel {
int levelData[10][10][10]; // A 10x10x10 grid for level design
};
This structure provides a foundational approach to organizing game elements within a 3D space.
Scientific Computing
3D arrays find utility in scientific computing where multi-dimensional data representation is essential. For example, in weather simulations, you can use a 3D array to represent data points over a geographical grid, where each axis corresponds to latitude, longitude, and altitude.
Image Processing Techniques
In image processing, 3D arrays are used to manage pixel data efficiently. Each pixel in a color image can be represented using three values for its RGB components. Here’s an example of a simple implementation that represents an RGB image:
unsigned char image[100][100][3]; // A 100x100 image with RGB values
This structure allows for direct manipulation of colors, essential for tasks like filtering, transformation, and rendering.

Best Practices for Using Three Dimensional Arrays in C++
Memory Management with 3D Arrays
When working with c++ three dimensional arrays, especially those allocated with `new`, it’s crucial to monitor and manage memory effectively. Always ensure that dynamically allocated arrays are released properly to avoid memory leaks:
for (int i = 0; i < x; i++) {
for (int j = 0; j < y; j++) {
delete[] array[i][j];
}
delete[] array[i];
}
delete[] array; // Deallocate top-level pointer
Debugging Common Issues
A common challenge when working with three dimensional arrays is indexing errors. It's essential to ensure that all indices used are within the declared dimensions to avoid accessing out-of-bounds memory. Tools like Valgrind can assist in tracing and resolving memory-related issues.
When to Avoid 3D Arrays
Although powerful, there are scenarios where using a three dimensional array may not be the best approach. Consider exploring alternative data structures, like std::vector<std::vector<std::vector<T>>>, for dynamic sizes or when performance is a concern. Additionally, always compare the performance implications of 3D arrays against other configurations or data handling strategies.

Conclusion
In summary, a c++ three dimensional array provides a powerful way to store and manipulate multi-dimensional data structures. With numerous applications ranging from gaming to scientific calculations, understanding how to effectively use and manage three dimensional arrays is essential for programmers. By mastering this concept, you equip yourself with the tools to handle complex data more elegantly in C++.