In C++, hexadecimal numbers are represented using the prefix `0x` or `0X`, allowing you to work with base-16 values efficiently.
Here's a simple code snippet demonstrating the use of hexadecimal in C++:
#include <iostream>
int main() {
int hexValue = 0x1A; // 26 in decimal
std::cout << "The decimal value of 0x1A is: " << hexValue << std::endl;
return 0;
}
Understanding the Basics of Hexadecimal in C++
The hexadecimal number system, or base-16, uses sixteen distinct symbols: the numbers 0 through 9 represent values zero to nine, and the letters A through F represent values ten to fifteen. For programmers, particularly those using C++, hexadecimal offers a more efficient way to represent binary data, as every hexadecimal digit corresponds to four binary digits (bits).
Why Use Hexadecimal?
Using hexadecimal can significantly simplify programming, especially when dealing with binary data and memory addresses. Here are a few reasons why hexadecimal is beneficial:
-
Efficiency: Hexadecimal can express large binary values in a much shorter form. For example, instead of writing `1111 1111 1111 1111`, you can simply write `0xFFFF`.
-
Ease of Use in Color Codes: In graphics and web design, colors are often represented in hexadecimal format, using `#RRGGBB`. This concise representation makes it easier to read and differentiate colors.

Using Hexadecimal in C++
Declaring Hexadecimal Values
In C++, you can declare hexadecimal literals by prefixing them with `0x` or `0X`. For example:
int hexValue = 0x1A; // This declares a hexadecimal variable
In this example, `0x1A` is the hexadecimal representation for the decimal value `26`.
Input and Output of Hexadecimal
Output Hexadecimal Using cout
To display hexadecimal values in C++, the `cout` stream can be combined with the `hex` manipulator. This changes the format of the output to hexadecimal. Here’s a simple code example:
#include <iostream>
using namespace std;
int main() {
int number = 30;
cout << "Hexadecimal: " << hex << number << endl; // Outputs: 1e
return 0;
}
In this snippet, we use the `hex` manipulator to transform the integer `30` into its hexadecimal equivalent, which is `1E`.
Input Hexadecimal
When taking input from the user, it’s also straightforward to receive hexadecimal numbers. Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
int hexInput;
cout << "Enter a hexadecimal number: ";
cin >> hex >> hexInput; // Reading hexadecimal input
cout << "Decimal value: " << hexInput << endl; // Outputs the decimal equivalent
return 0;
}
In the above code, the user enters a hexadecimal number, which is then automatically converted to its decimal form using `cin` with the `hex` manipulator.
Converting Between Number Systems
Understanding how to convert hexadecimal to decimal, and vice versa, is crucial. For instance, you can easily convert a hexadecimal string to a decimal integer using the `stoi` function.
Here’s an example explaining how to convert:
#include <iostream>
using namespace std;
int main() {
string hexNumber = "1A";
int decimalValue = stoi(hexNumber, nullptr, 16); // Convert hex to decimal
cout << "Decimal value of " << hexNumber << " is: " << decimalValue << endl; // Outputs: 26
return 0;
}
In this example, `"1A"` is converted to its decimal equivalent, `26`, using the `stoi` function with a base of `16`.

Advanced Usage of C++ Hexadecimal
Use Cases of C++ Hexadecimal
Bit Manipulation
Hexadecimal can be particularly useful when performing bit manipulation, which is a core concept in systems programming. Here’s an example of how you can use hexadecimal for setting or clearing specific bits:
#include <iostream>
using namespace std;
int main() {
int flags = 0x0F; // Represents binary 00001111
cout << "Flags: " << flags << endl;
flags |= 0x01; // Set the least significant bit
cout << "Updated Flags: " << flags << endl; // Outputs updated flags
return 0;
}
In this code snippet, the hexadecimal `0x0F` sets the initial flags. The bitwise OR operation (`|=`) is then used to set the least significant bit.
Memory Addressing
C++ programmers often use hexadecimal for memory addresses, especially when working with pointers. This is crucial for performance-critical applications such as hardware programming and system-level code. By representing memory addresses in hexadecimal format, code becomes easier to read and manage.
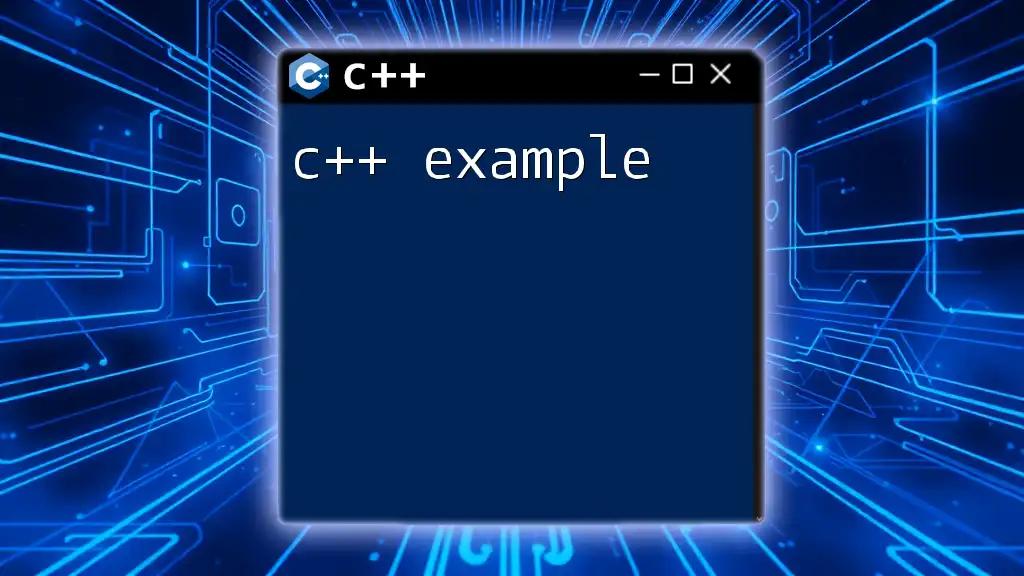
Common Pitfalls and Troubleshooting
When dealing with C++ hexadecimal, there are a few common pitfalls to watch out for:
-
Confusion Between Signed and Unsigned Integers: Ensure you understand how hexadecimal values may be interpreted differently based on the signedness of the integer type.
-
Input Misunderstanding: When reading hexadecimal input, if the user enters an invalid hexadecimal string, it may throw an error. Always validate user input to maintain robustness in your applications.
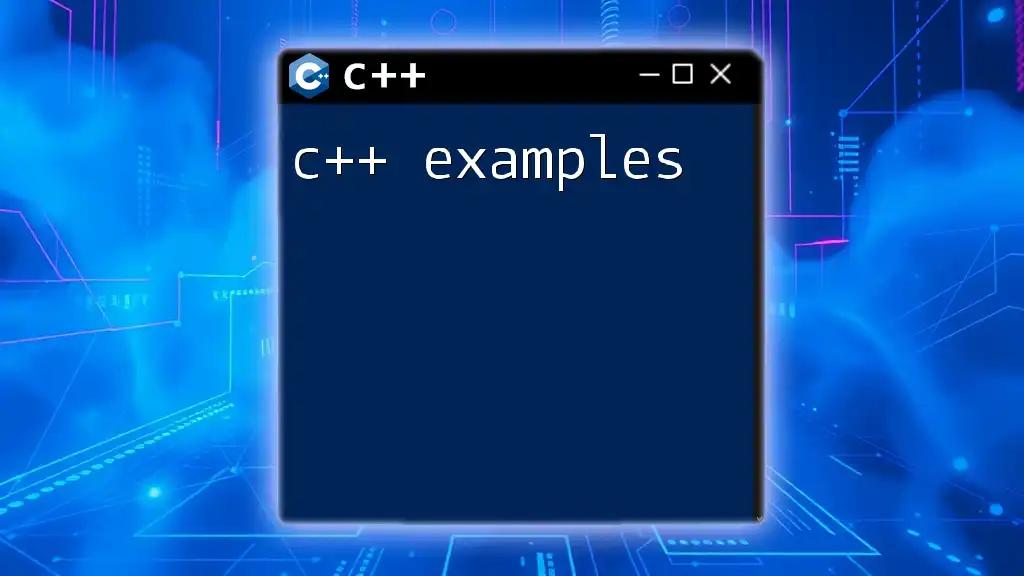
Conclusion
In summary, C++ hexadecimal is an essential tool for programmers. Understanding its structure and applications will enhance your coding efficiency, particularly in system-level programming and bit manipulation. By practicing and implementing these concepts, you will become more adept at using hexadecimal effectively in your C++ projects.

Additional Resources
For further exploration of C++ hexadecimal, consider delving into books such as "The C++ Programming Language" by Bjarne Stroustrup, or exploring online courses with a focus on advanced C++ topics. These resources will provide you with a deeper understanding of not just hexadecimal, but the entire landscape of C++ programming.