In C++, an exam typically involves testing the understanding of fundamental concepts, such as defining a simple program that calculates the average of an array of numbers. Here's a code snippet demonstrating this:
#include <iostream>
using namespace std;
int main() {
int numbers[] = {10, 20, 30, 40, 50};
int sum = 0;
for (int num : numbers) {
sum += num;
}
double average = sum / 5.0;
cout << "Average: " << average << endl;
return 0;
}
Understanding C++ Language Fundamentals
Basic Syntax and Structure
C++ is known for its powerful syntax, which is essential for defining the structure and flow of programs. The basic structure includes the `main` function, which serves as the entry point for execution, and can include header files such as `<iostream>` for input and output operations.
Here's a simple example that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, we include the iostream header to use the `std::cout` function, demonstrating how to output data.
Data Types and Variables
C++ supports several fundamental data types, including:
- int: for integers
- float: for floating-point numbers
- char: for characters
- bool: for boolean values
Understanding data types is crucial for memory management and performance optimization. Here’s an example of declaring different data types:
int age = 30;
float height = 5.9;
char initial = 'A';
You declare variables by specifying their type followed by the variable name and assignment.
Operators in C++
Operators are symbols that specify operations to perform on operands. They are categorized into different types:
- Arithmetic Operators: for basic mathematical operations
- Relational Operators: for comparisons
- Logical Operators: for true/false operations
Here's an example demonstrating an arithmetic operator:
int a = 5, b = 2;
std::cout << "Sum: " << (a + b) << std::endl; // Arithmetic operator
This code snippet shows how to perform addition and output the result.
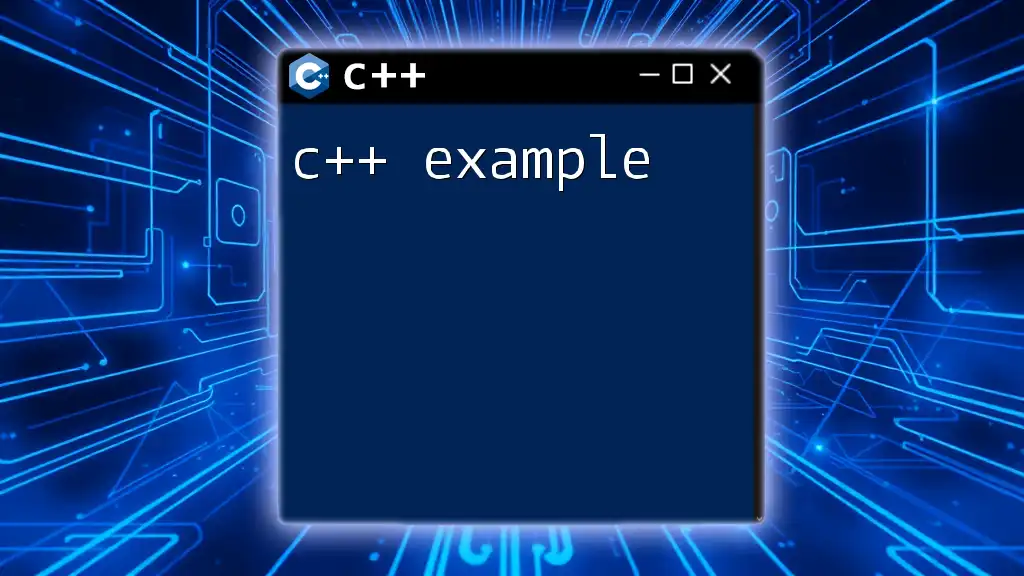
Key Concepts for C++ Exams
Control Structures
Control structures dictate the flow of execution in C++.
Conditional Statements
Conditional statements like `if`, `else`, and `switch` determine the path of execution based on differing conditions. For example:
if (age < 18) {
std::cout << "Minor" << std::endl;
} else {
std::cout << "Adult" << std::endl;
}
In this example, the output changes based on the condition evaluated.
Loops
Loops allow repeated execution of code. There are several types, including `for`, `while`, and `do-while`.
Here’s a `for` loop that prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This constructs a simple iteration where `i` increases with each loop until the condition is false.
Functions in C++
Functions simplify programming by enabling code reuse and readability.
Defining and Calling Functions
Functions can take inputs and return outputs. Here’s a basic example:
int add(int a, int b) {
return a + b;
}
To call this function, you would write:
std::cout << "The sum is: " << add(5, 3) << std::endl;
Function Overloading
C++ supports defining multiple functions with the same name, differing in parameters. For instance:
int add(int a, int b) { return a + b; }
double add(double a, double b) { return a + b; }
This feature allows you to provide more flexible function definitions.
Object-Oriented Programming (OOP) Concepts
OOP principles are fundamental in C++. They facilitate better organization and modularity of code.
Classes and Objects
A class is a blueprint for creating objects. Here’s a simple example of a class and object usage:
class Car {
public:
void start() { std::cout << "Car started" << std::endl; }
};
Car myCar;
myCar.start();
In this snippet, we have defined a class `Car` with a method `start`. An object `myCar` of the class is then created to utilize the method.
Inheritance and Polymorphism
Inheritance allows one class to inherit properties from another class, promoting code reuse. For instance:
class Vehicle { /* Base class */ };
class Bike : public Vehicle { /* Derived class */ };
This demonstrates how `Bike` is derived from `Vehicle`, inheriting its properties.
Polymorphism enables functions to act differently based on the object that calls them, which is commonly achieved through method overriding.
Exception Handling
C++ provides mechanisms to handle potential errors during execution, making programs resilient. This is known as exception handling. Here’s how you can throw and catch exceptions:
try {
throw 20; // Throwing an exception
} catch (int e) {
std::cout << "Caught an exception: " << e << std::endl;
}
Using `try` and `catch`, you can manage errors gracefully, preventing crashes and finding effective solutions.
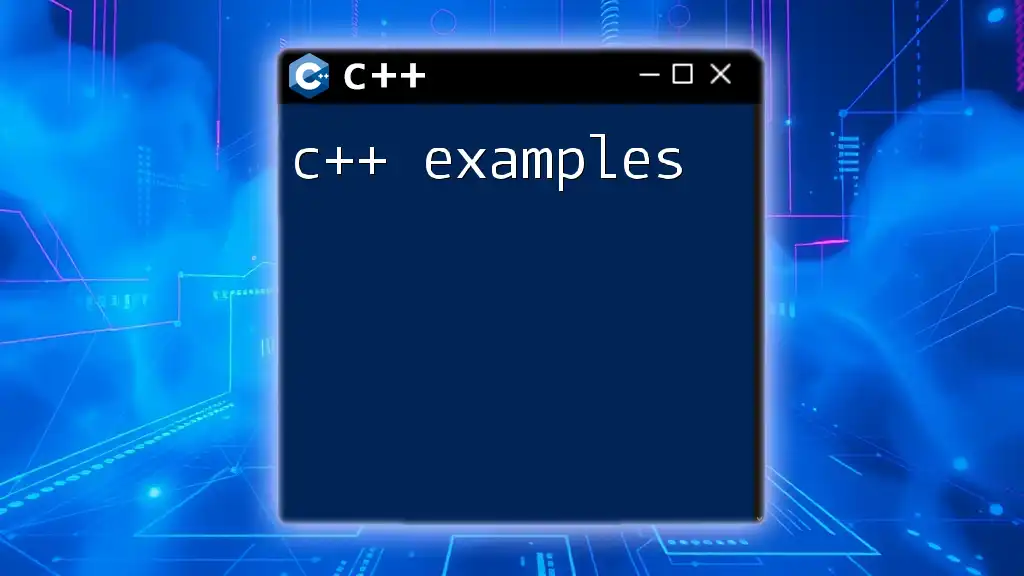
Preparing for Your C++ Exam
Study Strategies
When preparing for the C++ exam, it’s essential to utilize various study materials. Recommended resources include:
- Books: Titles such as "C++ Primer" and "Effective C++" are excellent for in-depth learning.
- Online Courses: Platforms like Coursera and Udemy provide structured learning paths.
- Interactive Websites: Websites like LeetCode and HackerRank offer practical problems to sharpen your skills.
Practice Questions and Exercises
To excel in your exam, solve sample questions that mirror exam patterns. Practice code snippets to reinforce your understanding of concepts. A few common problems you might encounter include:
- Implementing data structures like arrays and linked lists.
- Writing algorithms for sorting or searching.
Mock Tests and Resources
Taking mock tests is instrumental in familiarizing yourself with the exam format. Resources like CodeSignal and ExamPro provide comprehensive practice tests that can boost your confidence.
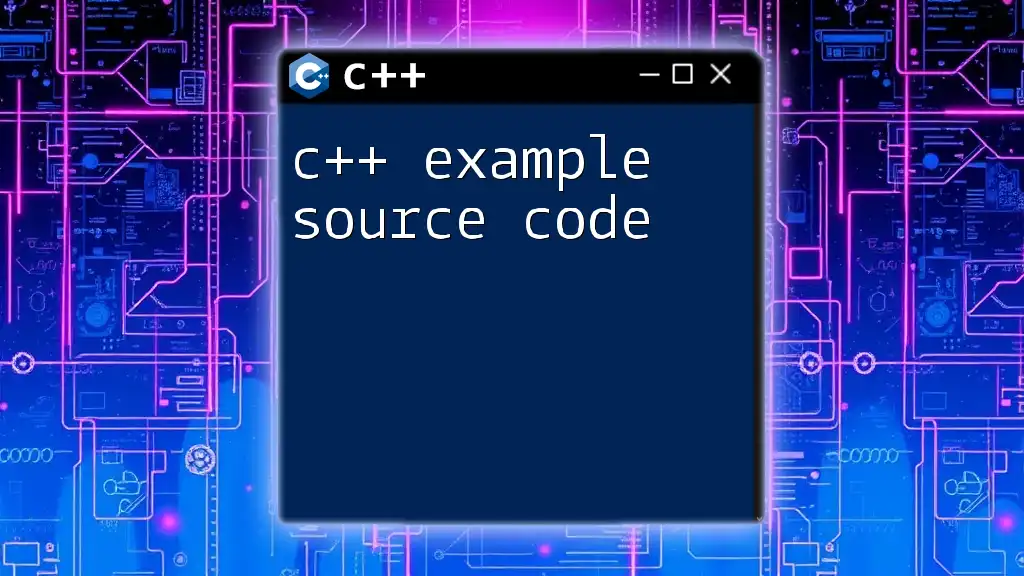
Exam Day Tips
What to Bring
Preparation on exam day can significantly influence performance. Ensure you have:
- Calculator: To assist with complex calculations.
- Notes: A cheat sheet of key concepts.
- Pens/Pencils: For writing answers clearly.
Time Management Strategies
During the exam, managing your time effectively helps ensure you can answer all questions. Allocate time for reviewing your answers and prioritize easier questions before tackling more challenging ones.
Handling Exam Anxiety
It’s common to feel anxious before exams. Techniques such as deep breathing exercises, visualization of success, and positive affirmations can help ease anxiety and boost focus.
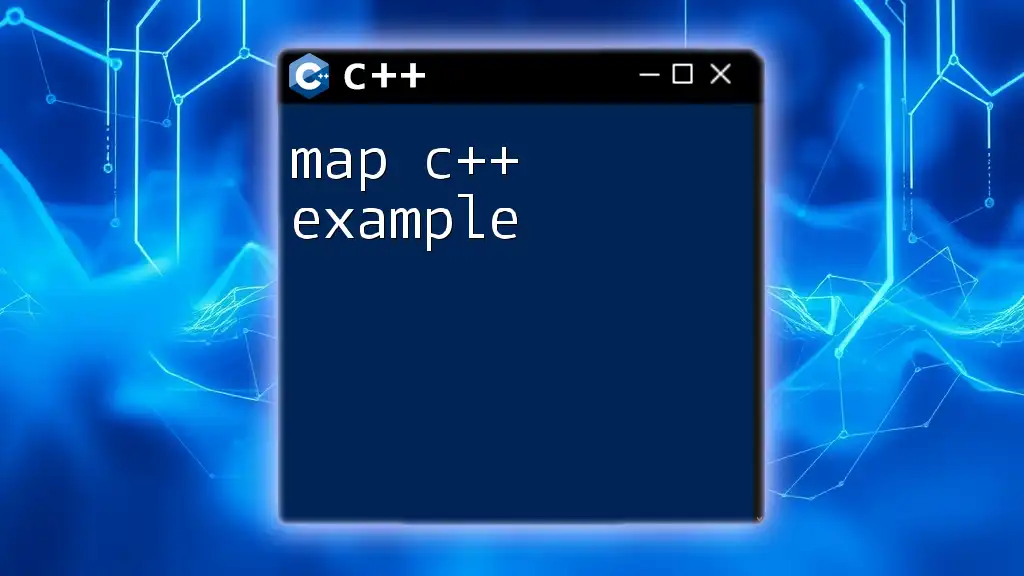
Conclusion
C++ is a versatile programming language that requires understanding of both fundamental and complex concepts. Covering the basics to advanced topics prepares you not just for exams, but also for real-world applications. Continuous practice, utilization of resources, and effective strategies will help you excel in your C++ exam.
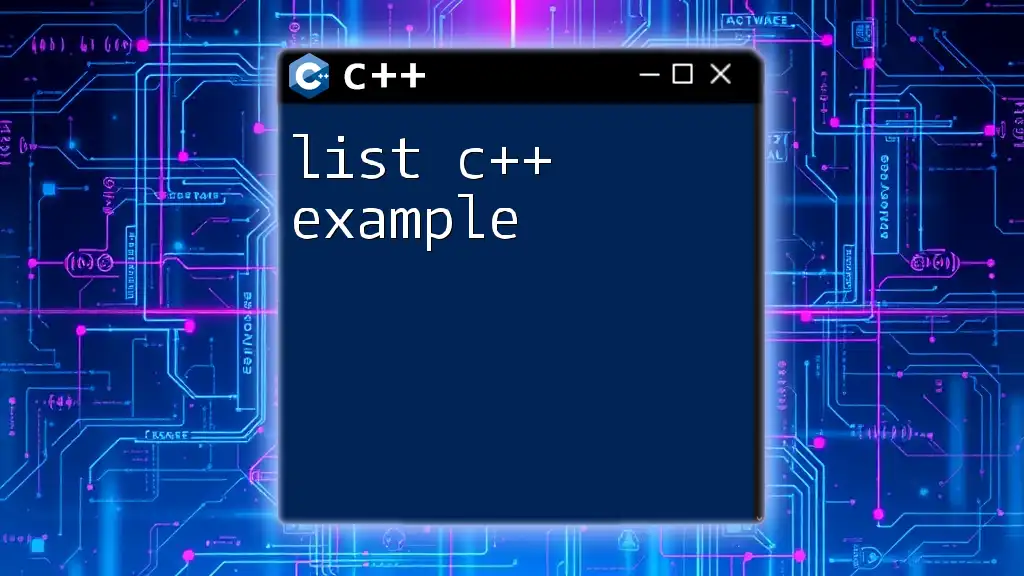
Additional Resources
To continue expanding your C++ knowledge, consider visiting platforms like Codecademy or GitHub repositories that focus on C++ projects. Books like "The C++ Programming Language" will further cement your understanding, providing real-world contexts for the concepts learned.