In C++, lambda capture allows you to access variables from the surrounding scope within a lambda function by specifying them in the capture clause.
Here's a simple code snippet demonstrating lambda capture:
#include <iostream>
int main() {
int x = 10;
auto lambda = [x]() { std::cout << "Captured x: " << x << std::endl; };
lambda();
return 0;
}
What is a Lambda Expression?
Lambda expressions are a powerful feature introduced in C++11 that allow you to create anonymous function objects. They provide a concise way to define functions at the point of invocation without adding the overhead of a separate function declaration. The general syntax for a lambda expression looks like this:
[capture_clause](parameters) -> return_type {
// function body
}
A capture clause defines how variables from the surrounding scope should be used inside the lambda. Understanding how to effectively capture variables is key to using lambdas proficiently in C++.
Example of a Simple Lambda Expression
Here’s a basic example of a lambda expression that prints a message:
auto hello = []() {
std::cout << "Hello, Lambda!" << std::endl;
};
hello();
This lambda function does not take any parameters and captures no external variables, simply printing a message when invoked.

The Concept of Capture in Lambda Functions
Capturing refers to the process of accessing variables from the surrounding scope inside a lambda expression. When you define a lambda, you have the option to specify which variables it can use. This is crucial for closing over the variables, especially within nested scopes.

Types of Capture
Value Capture
Value capture allows you to take a copy of the variables from the enclosing scope. This means that any changes made to the variables inside the lambda will not affect the original variables from the outer scope.
The syntax for capturing variables by value looks like this:
[capture_list]
Example of Value Capture
Here’s how value capturing works:
int x = 10;
auto captureValue = [x]() {
std::cout << "Captured by value: " << x << std::endl;
};
captureValue();
In this snippet, `x` is captured by value. Even if you change `x` after the lambda is defined, the output will still be `10`, because the lambda holds a copy of `x`.
Reference Capture
In contrast, reference capture allows the lambda to access the original variables. Any modifications made to these variables inside the lambda will reflect in the original variables outside.
The syntax for capturing variables by reference is as follows:
[&capture_list]
Example of Reference Capture
Here’s an example demonstrating reference capture:
int y = 20;
auto captureReference = [&y]() {
y += 10;
std::cout << "Captured by reference: " << y << std::endl;
};
captureReference(); // Outputs "Captured by reference: 30"
In the snippet above, `y` is captured by reference. The change to `y` inside the lambda updates the original variable.
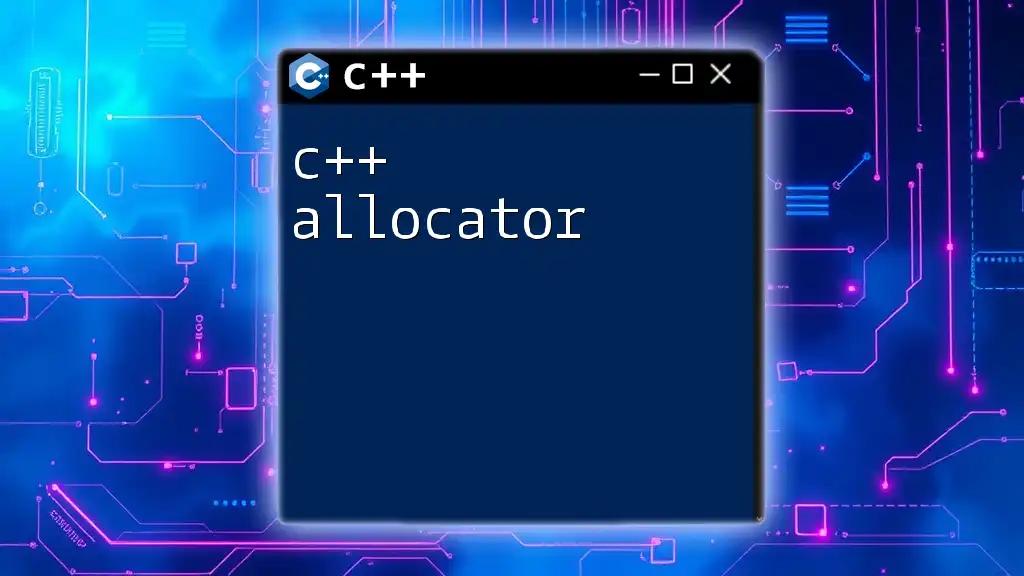
Capturing Everything
Capture Everything by Value
You can capture all variables in the enclosing scope by value using the `=` symbol in the capture clause. This can lead to more predictable behavior because it maintains the original values regardless of changes in the outer scope after the lambda is created.
Here's how to capture all variables by value:
int a = 5, b = 15;
auto captureAllByValue = [=]() {
std::cout << "Sum: " << a + b << std::endl;
};
captureAllByValue();
The output will always reflect the values of `a` and `b` when the lambda was created.
Capture Everything by Reference
You can also capture all local variables by reference using the `&` symbol. This results in the lambda having access to mutable references of the captured variables.
Example:
int c = 1, d = 2;
auto captureAllByReference = [&]() {
c *= 2;
d *= 3;
std::cout << "Updated values: " << c << ", " << d << std::endl;
};
captureAllByReference(); // Outputs updated values
Here, changes made to `c` and `d` reflect outside the lambda because they are captured by reference.

Combined Capture
You can also combine value and reference capture in a single lambda to handle specific requirements. This gives you the flexibility to choose which variables should be captured by value and which by reference.
Syntax and Importance
The syntax for combined capture looks like this:
[=, &var]
This captures all variables by value while allowing a specific variable to be captured by reference.
Example of Combined Capture
int e = 5;
auto combinedCapture = [=, &e]() {
std::cout << "Value: " << e << ", Constant: " << 10 << std::endl;
e += 5; // e captured by reference
};
combinedCapture(); // Outputs original values
In this case, `e` is captured by reference, allowing it to be modified directly within the lambda, while other variables are captured by value.
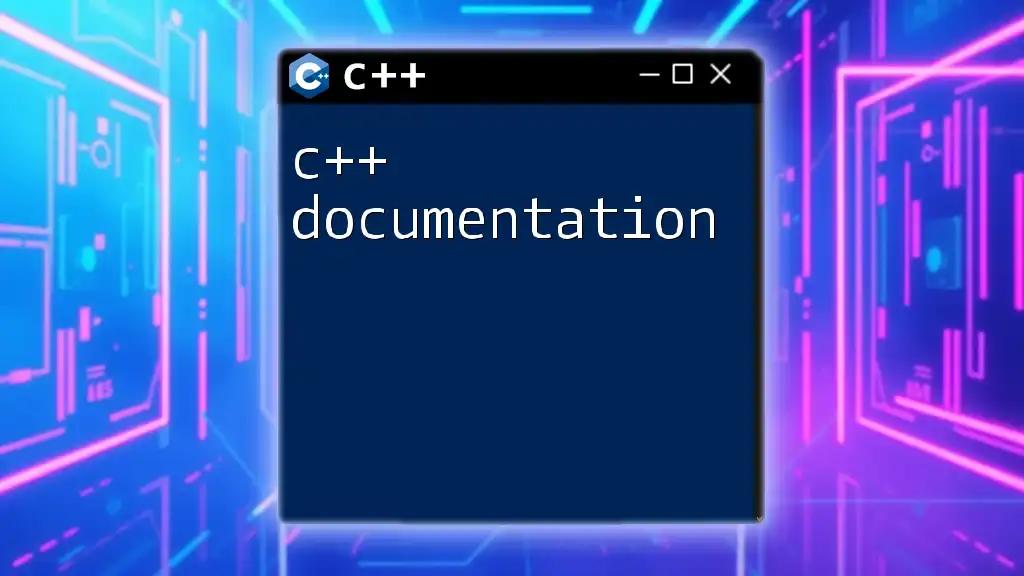
The `mutable` Keyword in Lambda Expressions
By default, lambdas that capture variables by value are not allowed to modify the captured variables. To change a value captured by value, you need to use the `mutable` keyword.
Explanation of `mutable`
The `mutable` keyword allows the lambda to modify its captured values while still keeping the original variables intact.
Example
int f = 1;
auto mutableLambda = [f]() mutable {
f *= 2;
std::cout << "Inside mutable lambda: " << f << std::endl; // Outputs 2
};
mutableLambda();
std::cout << "Outside: " << f << std::endl; // Outputs 1
In this example, `f` inside the lambda is a separate copy, and changing it does not affect the original `f`.
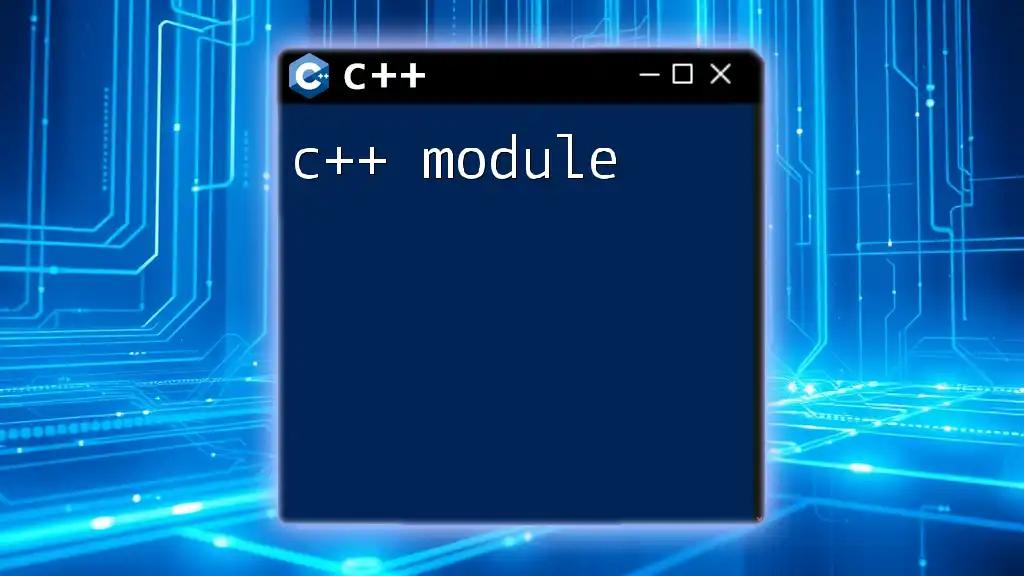
Real-world Use Cases of Lambda Capture
C++ lambda captures find significant real-world applications, especially in algorithms and event handling. They provide a cleaner and more expressive way to write code compared to traditional function pointers or functors.
Use Case in Algorithms
For instance, when working with algorithms such as `std::sort` or `std::for_each`, lambda captures can simplify code significantly:
std::vector<int> vec = {1, 2, 3, 4, 5};
int multiplier = 2;
std::for_each(vec.begin(), vec.end(), [multiplier](int &n) {
n *= multiplier; // capturing multiplier by value
});
In this code, `std::for_each` applies a modification to each element of the vector, using `multiplier` from the outer scope without requiring a separate function object.

Best Practices for Using Lambda Capture
When using lambda captures, adhere to these best practices to enhance both performance and readability:
- Use value capture for variables that do not need to be modified and where copying is cheap.
- Use reference capture for large objects or when you need to modify the original variable.
- Combine captures judiciously to ensure clarity in your code, making it clear which variables are intended to be mutable.
- Avoid capturing unnecessary variables to prevent unexpected behavior or increased complexity.
- Maintain readability by keeping lambdas concise—if they become overly complex, consider refactoring into a named function instead.

Conclusion
C++ lambda capture is a powerful mechanism that allows for concise and effective manipulation of variables from the surrounding context. By understanding different types of capture—value, reference, combined, and using tools like `mutable`—developers can write more responsive, clear, and maintainable code.
Becoming proficient with lambda captures will undoubtedly enhance your coding experience and expand your capability to utilize C++'s expressive power.
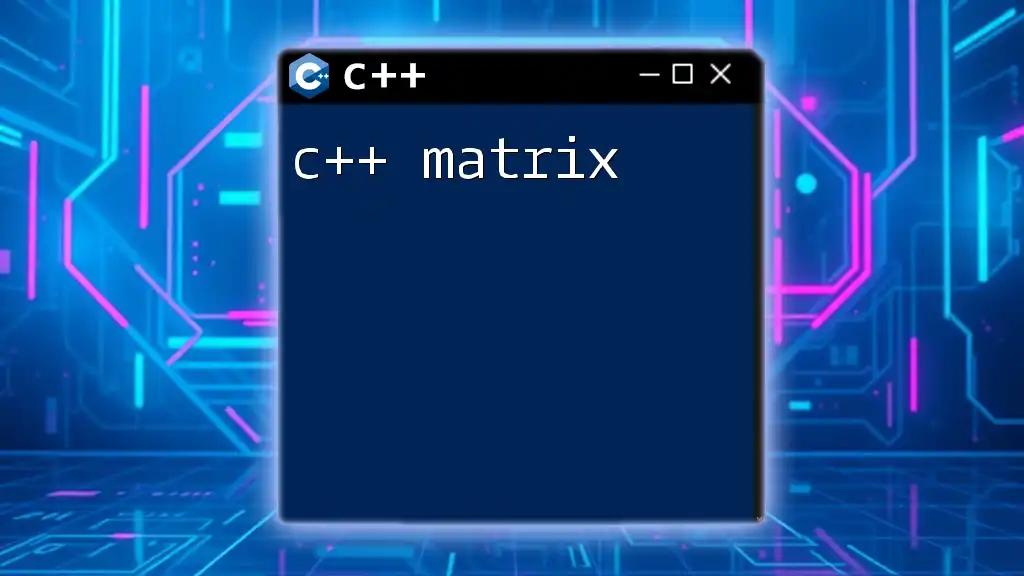
Further Reading and Resources
For those looking to deepen their understanding of C++ lambda capture, consider exploring online resources, official documentation, and community forums. Recommended books include "C++ Primer" and "Effective Modern C++". Engaging with these materials will provide further insight and examples that can supplement your learning journey.