In C++, the return type of a lambda expression can be explicitly specified using the `->` syntax, allowing for greater control over the function's output type.
Here's a code snippet demonstrating this:
#include <iostream>
#include <functional>
int main() {
auto add = [](int a, int b) -> int {
return a + b;
};
std::cout << "Result: " << add(5, 3) << std::endl; // Output: Result: 8
return 0;
}
What is a Lambda Expression?
A lambda expression in C++ is a compact and powerful way to define anonymous functions directly in the place where they are needed. Its syntax generally adheres to the structure:
[captures](parameters) -> return_type { body }
This expression consists of three main components:
- Captures: Variables from the surrounding context that the lambda can use.
- Parameters: Inputs the lambda accepts, just like regular functions.
- Return Type: Defines the data type of the value returned by the lambda. This leads us directly to the focus of this article.
Lambdas offer clean and concise syntax that enhances code readability and maintainability. They serve various purposes, such as callbacks for standard algorithms, handling asynchronous events, and encapsulating functionality.
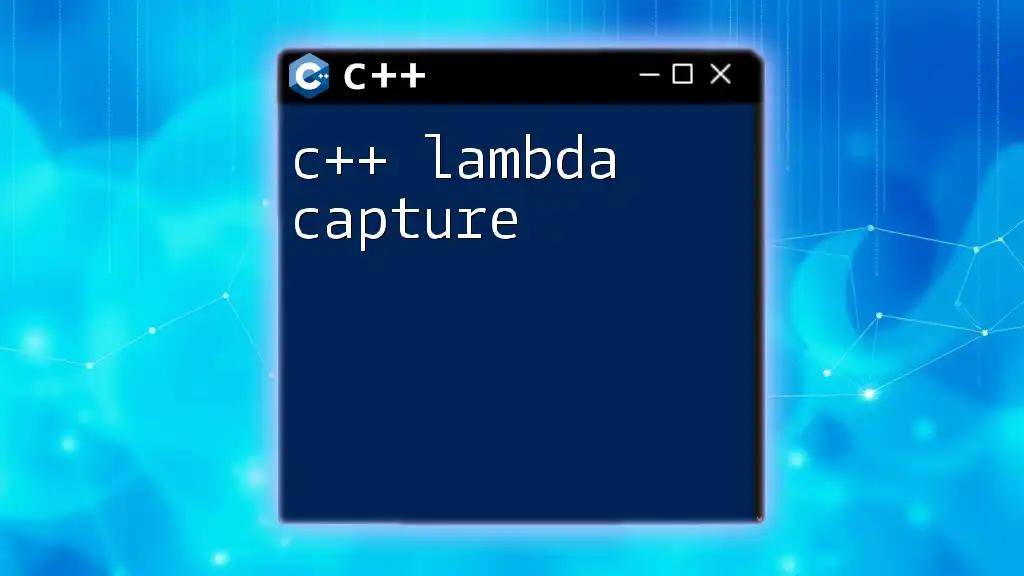
The Concept of Return Type in Lambda Expressions
The return type of a lambda expression indicates what kind of value the lambda will output. It is essential for ensuring that the consuming code understands what to expect when the lambda gets called.
Why Specify Return Types?
Specifying return types in lambdas has several key benefits:
- Clarity: A well-defined return type enhances code readability, making it easier for other programmers to understand what the lambda is designed to do.
- Type Safety: Clearly indicating return types helps to avoid implicit type conversions, providing an added layer of protection against unexpected behaviors.
- Performance: In certain situations, defining return types can provide performance enhancements by reducing the need for type inference overhead.

Using the `->` Syntax for Return Types
The `->` syntax is utilized to explicitly specify a return type in a lambda expression. Understanding its application can clarify how different types interact within the lambda.
How to Specify Return Types
Defining a return type can be quite straightforward. Here’s an example of a lambda that takes an integer parameter and returns an integer:
auto lambda = [](int x) -> int { return x * 2; };
In this example, the lambda is clearly defined to return an int, reinforcing its purpose. Each component works together to define behavior explicitly.
Different Return Types
Lambda expressions can return various types, including built-in types, user-defined types, and even more complex structures.
Returning Built-In Types: When returning simple data types like `int`, `float`, or `double`, the syntax remains similar. It’s crucial to match the expected outputs correctly.
Returning Objects: Lambdas can also return instances of classes or structs. For example, consider defining a struct for 2D points:
struct Point {
int x, y;
};
auto pointLambda = []() -> Point { return {1, 2}; };
In this example, the lambda returns a `Point` object, illustrating how lambdas can safely encapsulate complex return types.
Auto Return Type
The `auto` keyword allows you to delegate type inference to the compiler, simplifying the creation of lambdas. Here's an example:
auto lambdaAuto = [](double x, double y) { return x + y; }; // Returns double
In this case, the lambda's return type will be inferred as `double`, making it more flexible and reducing verbosity.

The Importance of the Return Type with Types Involving References and Pointers
In some situations, it's important to consider how return types interact with references and pointers.
Returning References
When a lambda returns a reference, it's crucial to ensure that the referenced variable remains valid for as long as the reference is in use. Here’s an example:
int a = 5;
auto referenceLambda = [&a]() -> int& { return a; };
Using a reference can enhance performance since no copying occurs. However, developers must be cautious about potential dangling references, which can arise if the referred variable goes out of scope.
Returning Pointers
Lambdas can also return pointers, which can be useful, but with added responsibility. Here’s an example of a lambda that returns a dynamically allocated integer:
int* returnPointerLambda() {
int* p = new int(10);
return p; // Remember to deallocate!
}
It's essential to manage memory properly when dealing with pointers to avoid memory leaks or undefined behavior.

Practical Applications of Lambda Return Types
Understanding lambda return types is vital for leveraging them effectively in real-world applications, especially when working with the Standard Template Library (STL).
Using with STL Algorithms
Lambda expressions frequently serve as custom functions for STL algorithms. When utilizing functions like `std::sort()` or `std::transform()`, defining return types helps ensure correctness.
Here's a classic example involving `std::sort()`:
std::vector<int> vec = {4, 2, 3, 1};
std::sort(vec.begin(), vec.end(), [](int a, int b) -> bool { return a < b; });
In this case, the lambda sorts the vector in ascending order, with an explicit return type of `bool` for clarity.
Callbacks and Asynchronous Operations
In modern C++, lambdas often play a crucial role in asynchronous programming. They can be passed as callbacks that are executed on certain events, such as when a task completes. Specifying a return type here helps prevent mistakes and guarantees that the callbacks yield the expected result.
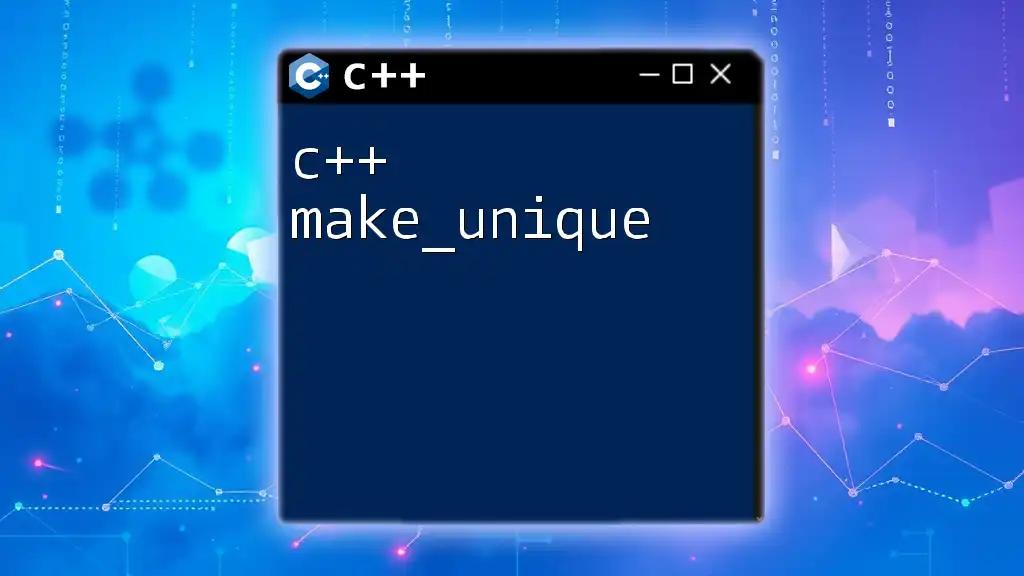
Conclusion
Understanding the C++ lambda return type is fundamental to mastery in modern C++ programming. This knowledge enhances both the clarity and functionality of your code. Remember to practice creating lambdas with different return types to build your understanding.

Additional Resources
For deeper insights, consider exploring the official C++ documentation on lambda expressions and recommended resources, including books and online courses dedicated to advanced C++ concepts.
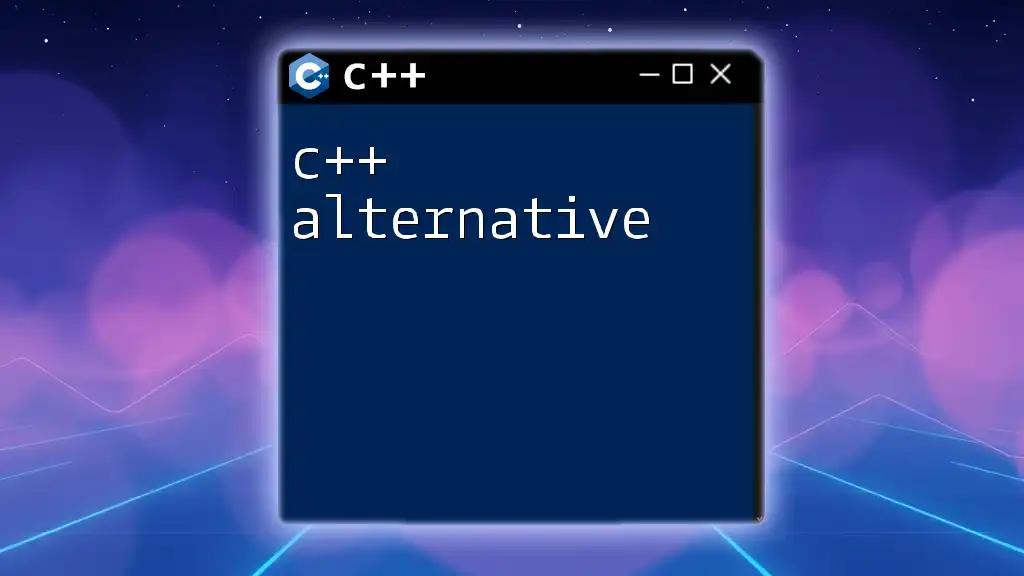
FAQs
Can I omit the return type in lambdas?
Yes, you can often omit the return type because C++ can infer it from the return statements in the lambda body. However, in more complex scenarios, providing an explicit return type can prevent confusion.
What happens if the return type is not specified?
If the return type is not specified and the return statements are ambiguous, the compiler will attempt to infer it. This may lead to errors or unexpected behaviors if the inferred type does not match the intended use of the lambda.