In C++, a lambda can capture variables by reference, allowing the lambda to modify the original variables defined outside its scope.
Here’s an example:
#include <iostream>
int main() {
int value = 10;
auto increment = [&value]() { value++; };
increment();
std::cout << value; // Outputs: 11
return 0;
}
Understanding C++ Lambdas
What is a Lambda Expression?
A lambda expression in C++ is an anonymous function that can be defined inline without the need for a named function. It allows you to create small function objects on the fly. The syntax consists of a capture clause, parameter list, and a function body. Understanding this structure is crucial, as it allows for powerful and expressive coding in a concise format.
Why Use Lambda Expressions?
Using lambda expressions streamlines your code by:
- Serving as lightweight function objects that can be defined within a local scope, reducing clutter.
- Seamlessly integrating with the Standard Template Library (STL) algorithms.
- Improving code readability and maintainability, allowing developers to focus on the operation rather than outlining full function definitions.
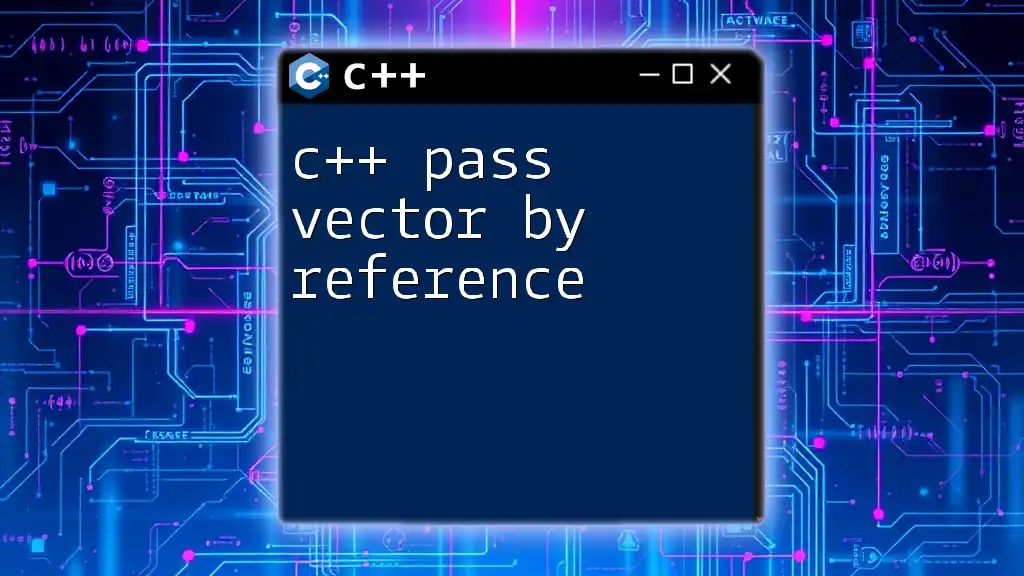
Lambda Capture Basics
Capture Clause Explained
At the heart of the lambda expression is the capture clause. This clause defines which variables from the surrounding scope are accessible within the lambda. The syntax for a lambda captures is as follows:
[capture_list] (parameters) { body }
In this expression, the `capture_list` defines how the outer variables are accessed: either by value or by reference.
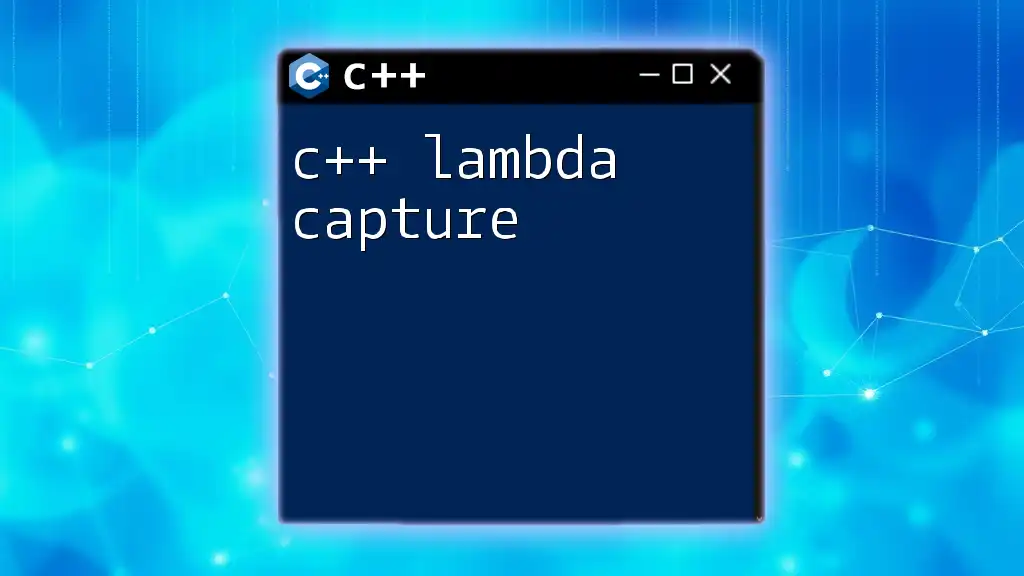
Capturing by Reference
What Does Capturing by Reference Mean?
When you capture by reference, the lambda can access and modify the original variable from the outer scope without creating a copy. This can lead to more efficient code when working with large data structures or when you need to maintain state across multiple invocations of the lambda.
Syntax for Capturing by Reference
To capture a variable by reference, you place an ampersand (`&`) before the variable name in the capture clause:
[&variable_name] (parameters) { body }
Example: Capturing Variables by Reference
Consider the following example where a lambda captures a variable by reference:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
int multiplier = 2;
auto doubleValues = [&multiplier](int& num) {
num *= multiplier;
};
for (auto& num : numbers) {
doubleValues(num);
}
// Output the modified numbers
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this code snippet, the lambda function `doubleValues` captures `multiplier` by reference. Thus, when you modify `num` inside the lambda, you indirectly multiply the numbers in the vector by `multiplier`, resulting in modified values of `2, 4, 6, 8, 10`. The effect is direct, as `num` is modified in the context of its original storage.
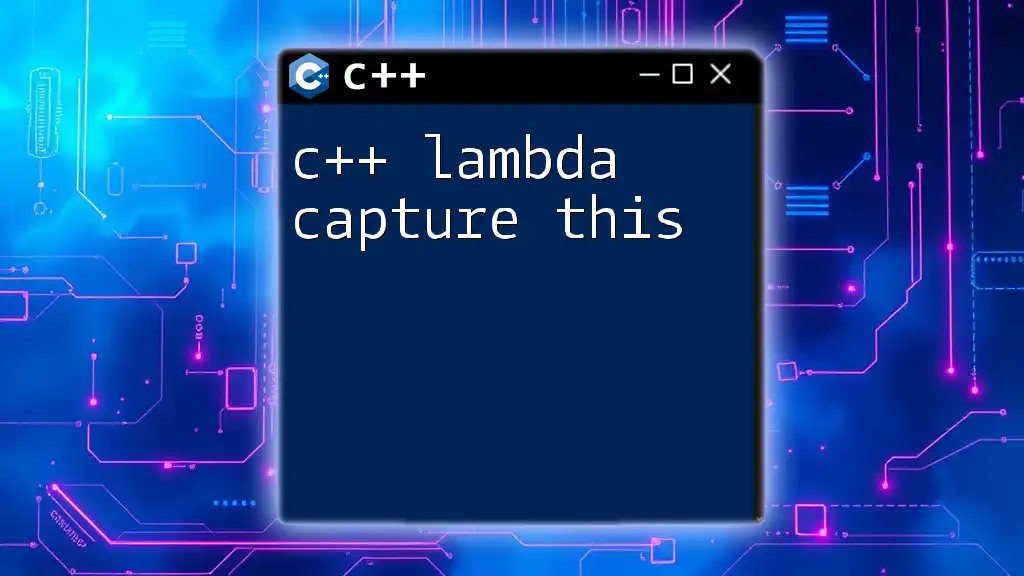
Use Cases for Reference Capture
When to Use Capture by Reference
Capturing by reference is particularly beneficial in scenarios where:
- Modification of Variables: The lambda needs to modify the captured variable's value.
- Memory Efficiency: There is a need to avoid unnecessary copies of large data structures, which could lead to performance bottlenecks.
Practical Applications
Capturing by reference shines in multiple practical applications:
-
Updating Variables in Loops: When you need to use state that evolves across iterations, capturing by reference allows you to maintain access to these variables efficiently.
-
Callbacks with Mutable State: When working with asynchronous operations, using lambdas with reference captures can keep the state consistent across callbacks.
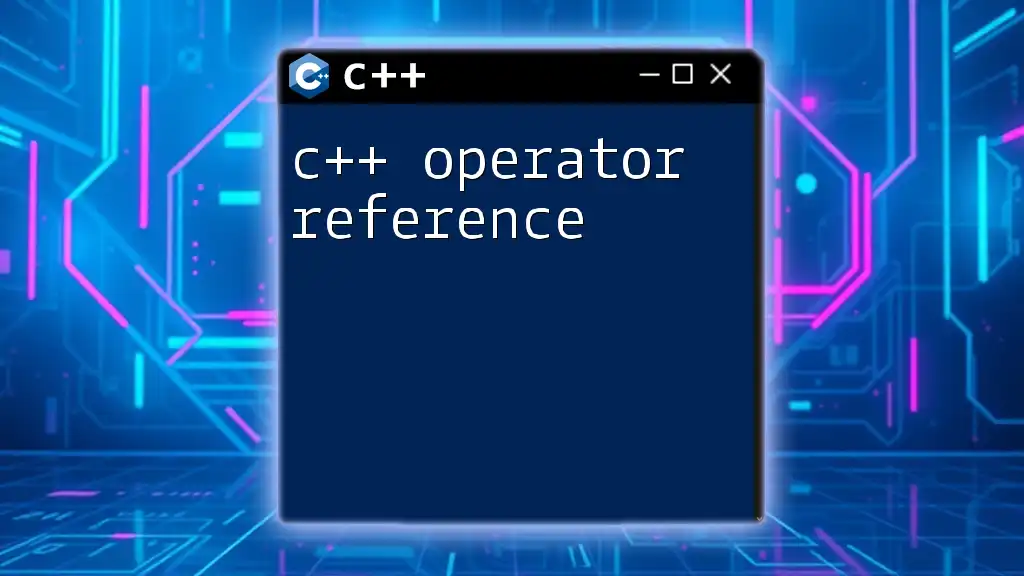
Potential Pitfalls and Best Practices
Dangers of Capturing by Reference
While capturing by reference can enhance performance, it carries risks:
- Dangling References: If the lambda outlives the scope of the referenced variable, the reference becomes invalid, leading to undefined behavior.
Best Practices for Safe Reference Capture
To mitigate risks while capturing by reference:
- Always ensure the captured variables are guaranteed to remain in scope for the lifetime of their references.
- Consider the use of `std::shared_ptr` or other smart pointers if the lambda needs to maintain ownership of the captured state dynamically.
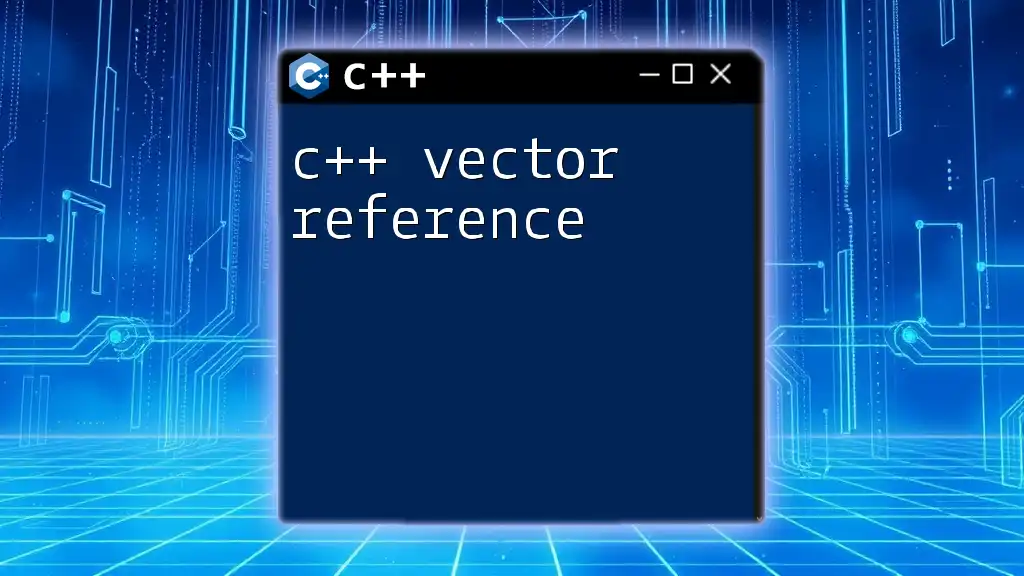
Working with Mutable Lambdas
Understanding the `mutable` Keyword
The `mutable` keyword allows you to modify captured variables even when the lambda is marked as `const`. By default, a lambda that captures variables by reference cannot modify those variables, unless explicitly marked with `mutable`.
Example of Using `mutable` with Reference Capture
Here is how you can utilize the `mutable` keyword in a lambda function:
#include <iostream>
int main() {
int counter = 0;
auto incrementCounter = [&counter]() mutable {
counter++;
return counter;
};
std::cout << incrementCounter() << std::endl; // Output: 1
std::cout << incrementCounter() << std::endl; // Output: 2
return 0;
}
In this example, the lambda `incrementCounter` captures `counter` by reference but is also marked with `mutable`. This enables modifications to the `counter` inside the lambda, resulting in a predictable incremental output.
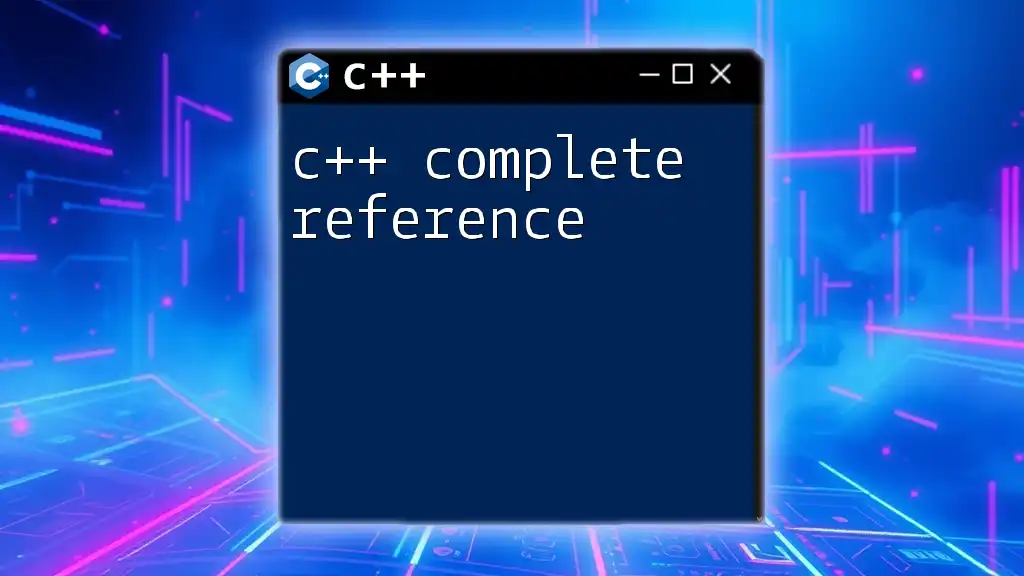
Summary
In summary, understanding C++ lambda capture by reference not only empowers you to write more efficient and expressive code but also highlights the key trade-offs involved. By mastering this concept, you can harness the full potential of lambdas in C++. Practice capturing variables by reference in your projects to build a stronger foundation in C++.
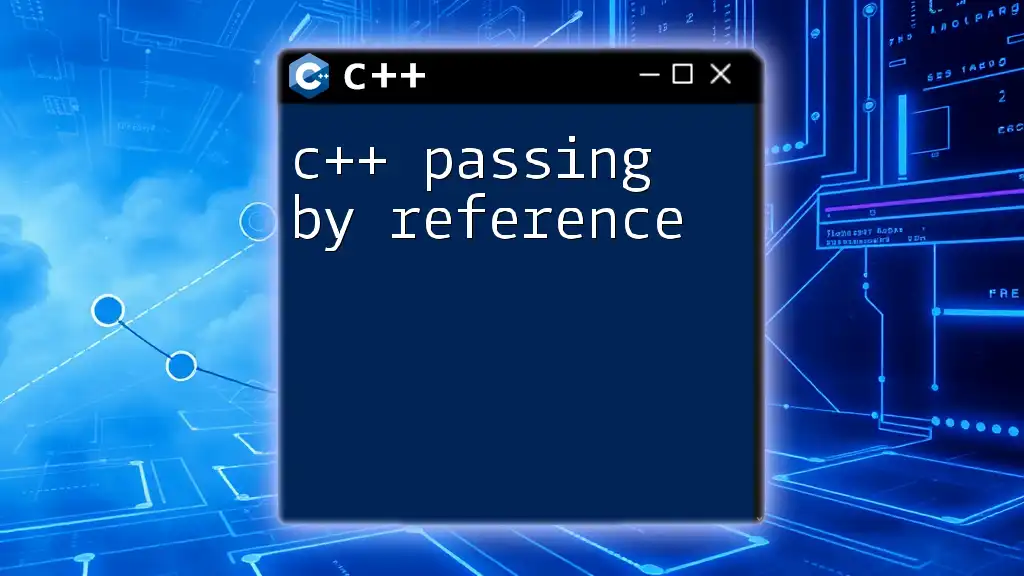
Further Reading
For those eager to improve their grasp of C++, consider exploring the official documentation and recommended literature. Resources on advanced C++ programming concepts will solidify your knowledge and allow you to tackle complex problems with confidence.