C++ pointers are variables that store the memory address of another variable, while references provide an alias for another variable, allowing direct access without the need for dereferencing.
#include <iostream>
int main() {
int var = 42; // Normal variable
int* ptr = &var; // Pointer to var
int& ref = var; // Reference to var
std::cout << "Pointer: " << *ptr << ", Reference: " << ref << std::endl; // Outputs: Pointer: 42, Reference: 42
return 0;
}
Understanding Pointers
What are Pointers?
Pointers are a fundamental feature in C++ that allow us to store the memory address of a variable instead of the variable's actual value. This concept is crucial for dynamic memory management, as it enables more flexible and efficient usage of memory. Understanding pointers opens up possibilities for complex data structures and efficient resource management.
Declaring Pointers
Declaring a pointer in C++ involves specifying the type of data it points to, followed by an asterisk (*) to indicate that it's a pointer. This tells the compiler what kind of variable the pointer will reference.
int* ptr; // Declaration of a pointer to an integer
In this example, `ptr` is a pointer that can hold the address of an integer variable.
Initializing Pointers
Pointers can be initialized to point to a variable by using the address-of operator (`&`). This operator retrieves the address of a variable.
int var = 42;
int* ptr = &var; // Initialize pointer to the address of var
Here, `ptr` now points to the memory location where `var` is stored.
Dereferencing Pointers
Dereferencing a pointer means accessing the value at the memory address stored in the pointer. This is achieved using the asterisk (*) operator again, but this time in front of the pointer variable.
cout << *ptr; // Outputs: 42
In this case, using `*ptr` retrieves the value stored at the address pointed to by `ptr`, which is `42`.
Pointer Arithmetic
Pointer arithmetic allows you to perform arithmetic operations on pointers. This is useful when dealing with arrays or data structures where you need to navigate between elements.
int arr[] = {10, 20, 30};
int* p = arr; // p points to the first element
cout << *(p + 1); // Outputs: 20
By adding `1` to `p`, we move the pointer to the next integer in the array (`arr[1]`), which contains the value `20`.
Pointers to Pointers
In some scenarios, you might need a pointer that points to another pointer. This is known as a pointer to a pointer. It allows for more complex data structures, such as dynamic arrays of pointers.
int** ptr2Ptr = &ptr;
Here, `ptr2Ptr` is a pointer that holds the address of another pointer (`ptr`). This concept is particularly useful in functions dealing with multi-dimensional arrays or complex data structures.
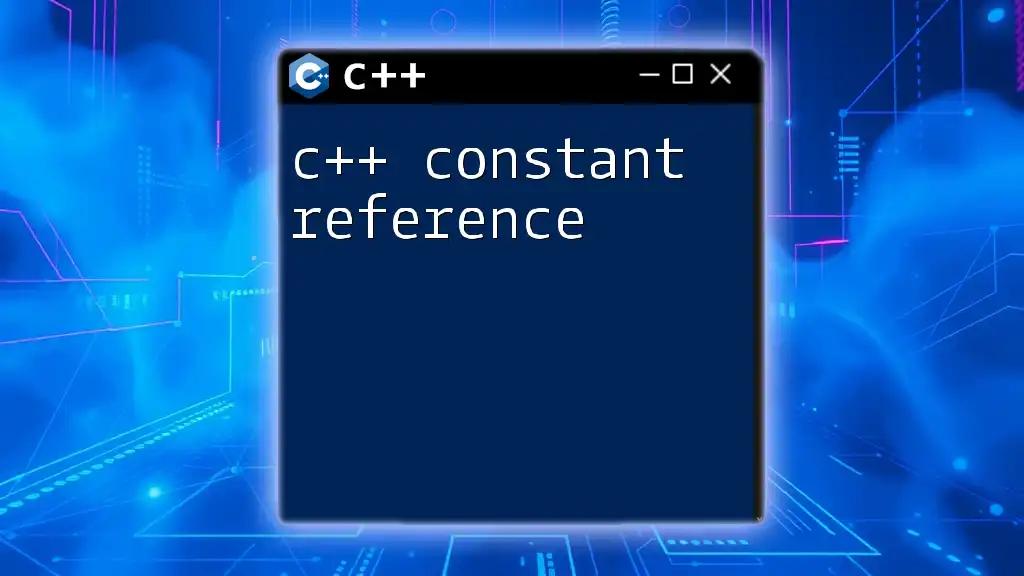
Understanding References
What are References?
A reference is an alias for another variable, meaning it allows you to refer to a variable using a different name. References can simplify the syntax and help avoid common pitfalls associated with pointers, such as dereferencing null values.
Declaring References
To declare a reference, you use the `&` operator. It’s essential to understand that references must be initialized when they are declared; they cannot be null or reassigned.
int var = 5;
int& ref = var; // ref is a reference to var
In this example, `ref` serves as an alternative name for `var`.
Modifying Values through References
Any changes made through a reference affect the original variable it refers to. This can be particularly useful for functions that need to modify their input arguments directly.
ref = 10; // Changes var to 10
cout << var; // Outputs: 10
Here, assigning `10` to `ref` modifies `var` to hold the value `10`.
References and Function Arguments
Using references for function parameters can improve performance and prevent unnecessary copying of data. When a function takes a reference, it can modify the argument directly.
void increment(int& value) {
value++;
}
This function increments its argument directly, allowing for efficient data manipulation without copying the entire value.
Constant References
Constant references are used when you want to ensure that a function does not modify the argument passed to it, while still allowing the function to work with large data structures efficiently.
void display(const int& num) {
cout << num;
}
In this case, the `const` qualifier guarantees that `num` will not be altered within the `display` function, providing safety while allowing optimal performance.
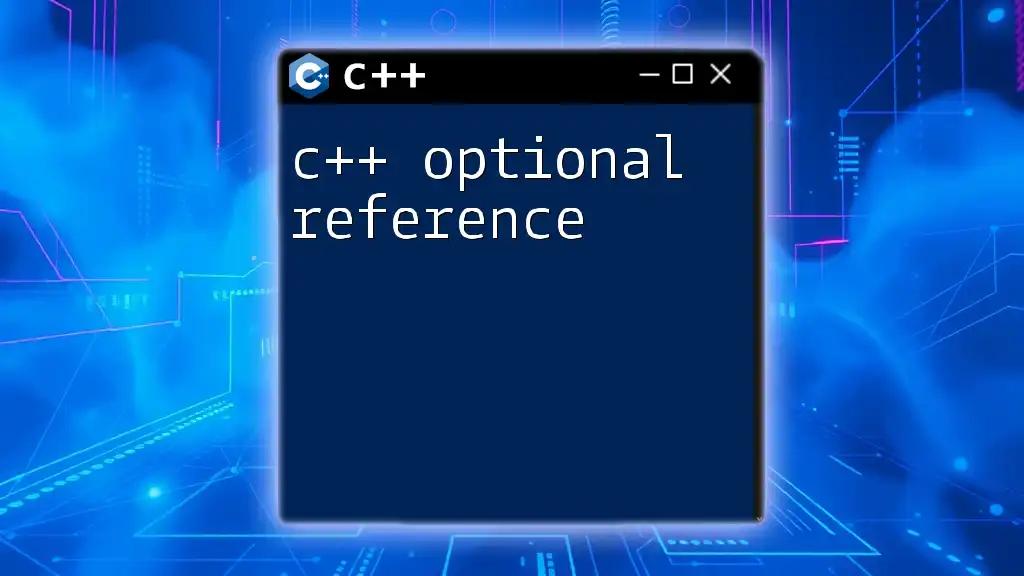
Comparing Pointers and References
Similarities and Differences
Both pointers and references serve the purpose of referring to other variables, but they exhibit significant differences. For instance, pointers can be reassigned and can hold a null value, while references must be initialized at the time of declaration and cannot be made to refer to another variable after their initialization.
When to Use Pointers vs. References
In general, use references when you want to provide a clear and simple interface without the complexities of pointer arithmetic or nullability. Use pointers when you need the ability to have no valid reference (i.e., null pointers), or when dealing with dynamic memory management, complex data structures, or low-level memory manipulation.

Best Practices for Using Pointers and References
Avoiding Common Pitfalls
Pointers can lead to various issues, such as dereferencing null pointers, memory leaks, and dangling pointers. To protect against these mistakes:
- Always initialize pointers before use.
- Avoid memory leaks by ensuring that dynamically allocated memory is freed.
- Use smart pointers (like `std::unique_ptr` and `std::shared_ptr`) where possible to manage memory automatically.
Performance Considerations
When considering performance, both pointers and references can be efficient, but references often yield better clarity and maintainability in code. However, be aware of the overhead of copying large objects, which can be avoided by using references as function parameters.

Conclusion
Understanding C++ pointers and references is crucial for effective programming. Pointers enable flexible memory management and data structure manipulation, while references provide a safer, simpler interface for modifying variables. By mastering these concepts, you can write more efficient and robust C++ code.
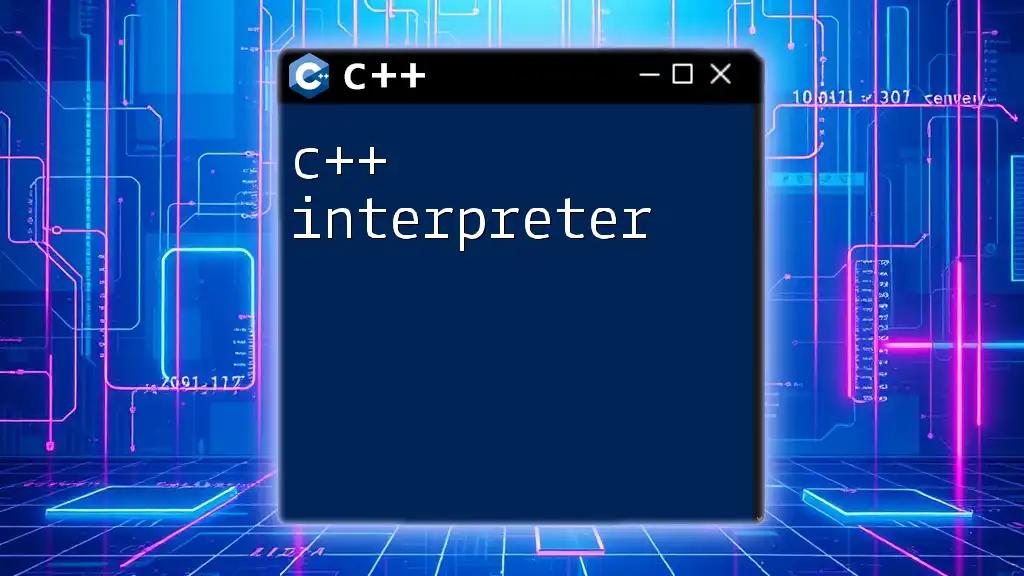
Additional Resources
For further exploration of C++ pointers and references, consider consulting resources such as:
- Books: "The C++ Programming Language" by Bjarne Stroustrup
- Online Courses: Websites such as Coursera or Udacity
- Documentation: C++ Standard Library documentation or GCC documentation for compiler-specific features.