A C++ universal reference is a template type that can bind to both rvalue and lvalue references, typically used in template programming to enable perfect forwarding.
template <typename T>
void process(T&& arg) {
// Process the argument, which could be an lvalue or rvalue
}
Understanding References in C++
What are References?
In C++, a reference is an alias for another variable. This means that a reference does not hold its own value but directly refers to the value of another variable. The main difference between references and pointers is that references must be initialized when declared and cannot be reassigned to refer to a different variable later on. The syntax for declaring a reference is straightforward:
int a = 5;
int& ref = a; // ref is now an alias for a
Types of References
References in C++ can be classified into two main categories: lvalue references and rvalue references.
-
Lvalue References: An lvalue reference, declared using `&`, can only bind to lvalues (variables that occupy identifiable locations in memory). They play a crucial role in modifying variables without using pointers.
void modify(int& value) { value += 10; // modifies the original variable } int main() { int num = 5; modify(num); // num becomes 15 }
-
Rvalue References: Introduced in C++11, rvalue references, declared using `&&`, can bind to rvalues (temporary objects that do not persist in memory). This feature is essential for implementing move semantics, allowing resources to be moved rather than copied, which enhances performance.
void takeOwnership(std::string&& str) { // Ownership of str can be transferred }
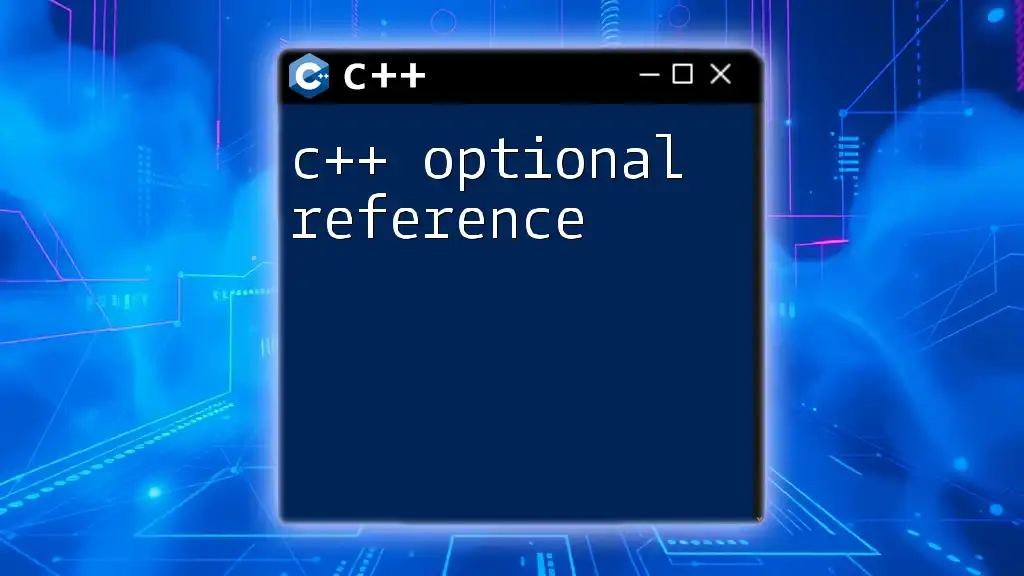
The Concept of Universal References
What is a Universal Reference?
A universal reference is a special type of reference that can bind to both lvalues and rvalues. This concept is crucial for template programming because it allows developers to write generic and highly efficient code, accommodating various value categories without sacrificing performance.
Eric Lippert, a renowned C++ expert, popularized the term "forwarding reference" for universal references, emphasizing their ability to perfectly forward arguments.
Syntax of Universal References
The syntax for declaring a universal reference is using `T&&` in a template function, where `T` is a template type parameter. The context of the type deduced determines if it's treated as an lvalue or rvalue reference.
Example:
template<typename T>
void func(T&& arg) {
// arg can be either an lvalue or an rvalue
}
In this function, when passed an lvalue, `T` is deduced as an lvalue reference and, thus, `arg` behaves as an lvalue reference. Conversely, if an rvalue is passed, `T` deduces as an rvalue reference.
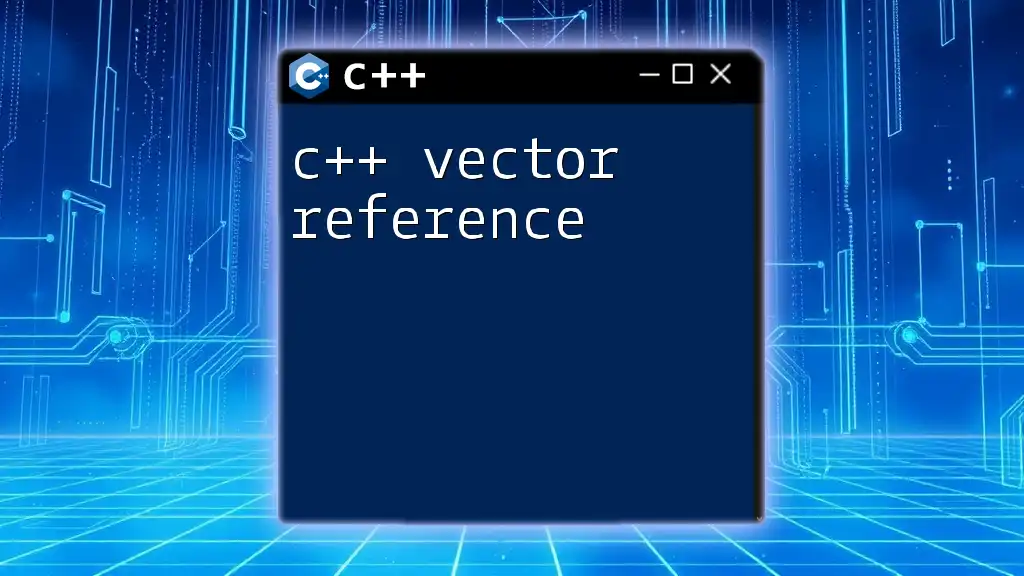
Differences Between Lvalue and Rvalue References
Characteristics of Lvalue and Rvalue
Lvalue references can refer to variables that persist beyond a single expression (such as `int x = 10;`). Rvalue references refer to temporary values that will be immediately destroyed after their use.
- Lvalues: Named variables (e.g., `x`, `myArray`)
- Rvalues: Temporary objects or literals (e.g., `5`, `std::string("temporary")`)
Use Cases in Code
Here's an illustration of how these references work:
void takeLvalue(int& x) { /*...*/ }
void takeRvalue(int&& x) { /*...*/ }
int main() {
int a = 10;
takeLvalue(a); // Valid: lvalue reference
takeRvalue(20); // Valid: rvalue reference
}
In the above code:
- `takeLvalue(a);` accepts `a`, an lvalue.
- `takeRvalue(20);` accepts `20`, an rvalue literal.
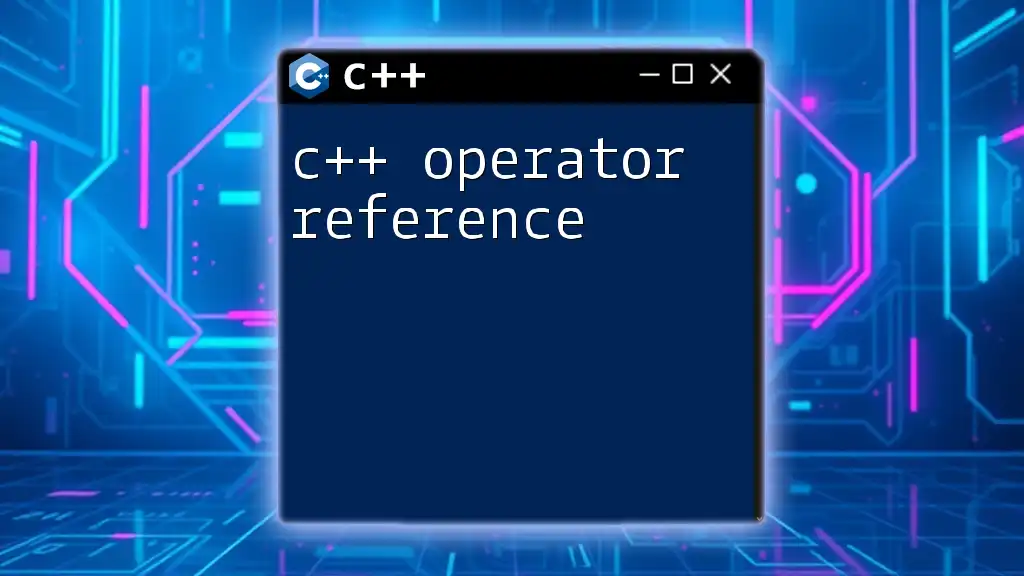
How Universal References Improve Code
Perfect Forwarding
Perfect forwarding allows functions to forward their arguments while preserving their value category (lvalue or rvalue), ensuring optimal function performance.
Using `std::forward` is essential in achieving perfect forwarding. Here’s an example:
template<typename T>
void wrapper(T&& arg) {
func(std::forward<T>(arg)); // Calls func, preserving the original value category
}
In this code, `arg` is forwarded to `func`, taking advantage of whether it's an lvalue or an rvalue, improving efficiency without unnecessary copies.
Performance Benefits
The use of universal references can significantly enhance the performance of applications. By enabling move semantics, developers can transfer ownership of resources without the overhead of deep copying, thereby conserving memory and processing time. This is particularly advantageous in scenarios involving large structures like vectors or custom data types.
Here's an illustration of utilizing `std::move`:
class MyClass {
public:
MyClass(MyClass&& other) : data(std::move(other.data)) {}
template<typename T>
void setData(T&& arg) {
data = std::forward<T>(arg);
}
private:
std::string data;
};
In this class:
- Move Constructor: Incurs no copying cost.
- SetData Method: Accepts both lvalues and rvalues while maintaining efficiency through perfect forwarding.
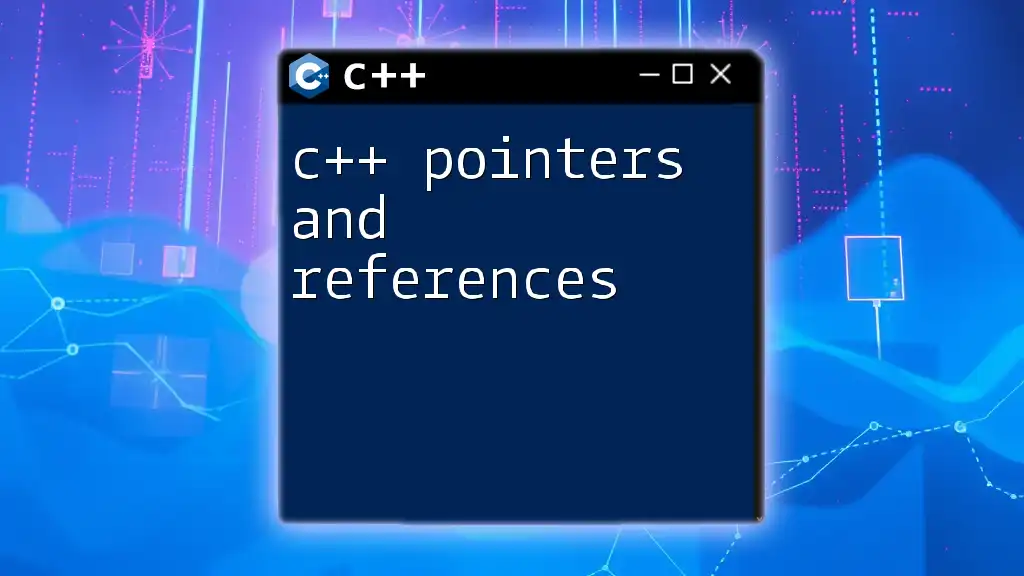
Common Pitfalls
Misunderstanding Universal References
One common mistake developers make is confusing universal references with rvalue references, often leading to unintentional copying in templates. A typical error occurs when a template parameter is not declared correctly:
template<typename T>
void badFunc(T arg) { // This is not a universal reference
// implementations
}
int main() {
int x = 5;
badFunc(x); // Creates a copy instead of forwarding
badFunc(10); // Creates another copy
}
In this example, the failure to use `T&&` means that regardless of whether the argument is an lvalue or an rvalue, `arg` will always result in a copy.
Overuse of Universal References
It’s essential to recognize situations where universal references may not be optimal. There are scenarios where traditional lvalue or rvalue references might be preferred—such as in non-templated functions where performance overhead is not a concern.
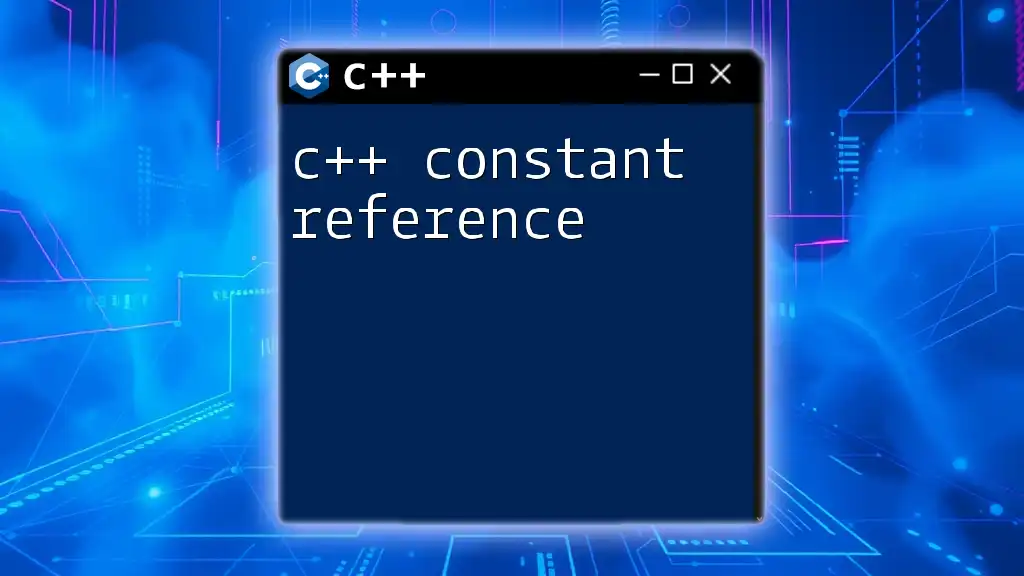
Best Practices for Using Universal References
When to Use Universal References
Universal references should be used when writing generic code, particularly in template functions and when interfacing with perfect forwarding. They shine in libraries and APIs where flexibility in input types is desirable.
Combining Universal References with Other C++ Concepts
To maximize efficiency and maintain compatibility with modern C++, universal references can be effectively integrated with various concepts such as smart pointers, constructors, and destructors. Here’s an example of integrating universal references in a class:
class Example {
public:
Example(Example&& other) : resource(std::move(other.resource)) {} // Move constructor
template<typename T>
void allocate(T&& res) {
resource = std::forward<T>(res); // Forwarding Resource
}
private:
std::string resource;
};
In this class, the constructor efficiently transfers ownership without unnecessary copies, and the `allocate()` method can take different types (lvalues and rvalues) thanks to universal references.
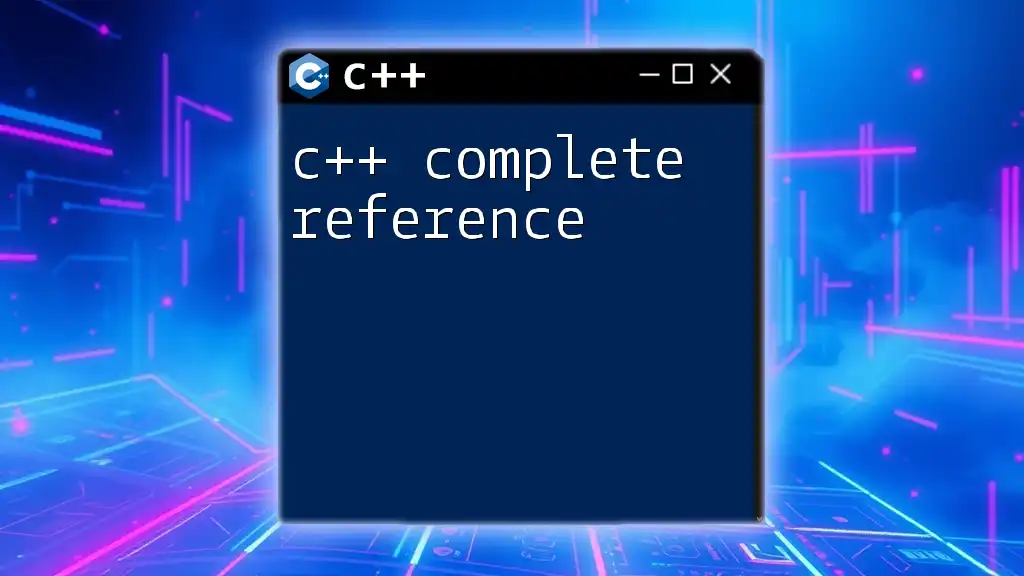
Conclusion
In conclusion, understanding and utilizing C++ universal references can significantly enhance the performance and flexibility of your code. By mastering their application in template programming, you open the door to writing more efficient, elegant C++ programs. Embracing these concepts empowers developers to better manage resources and streamline their coding practices. Further exploration of this feature will undoubtedly yield fruitful results in your C++ journey.
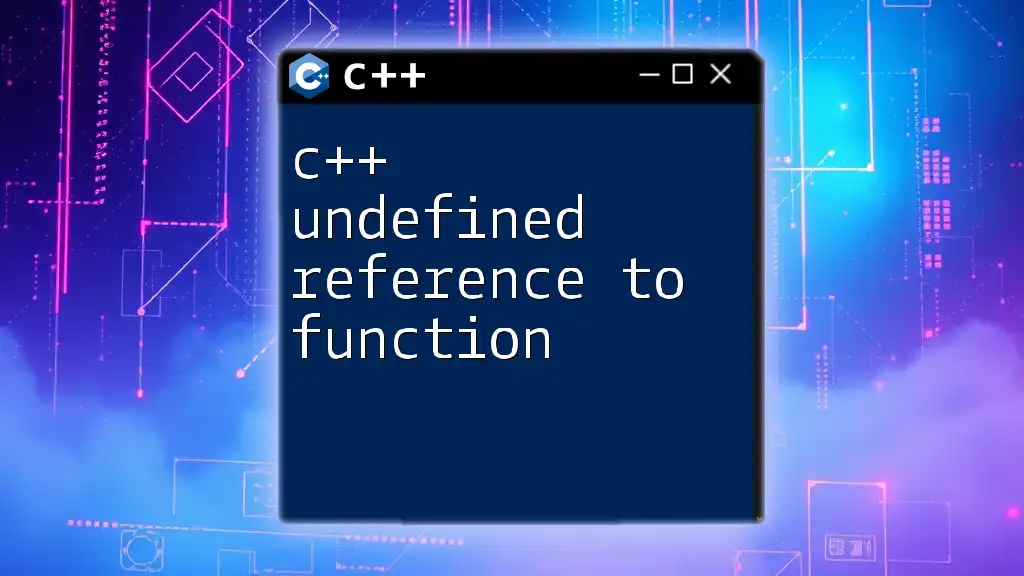
References
Relevant literature and online resources will significantly enhance understanding and provide continued learning opportunities.
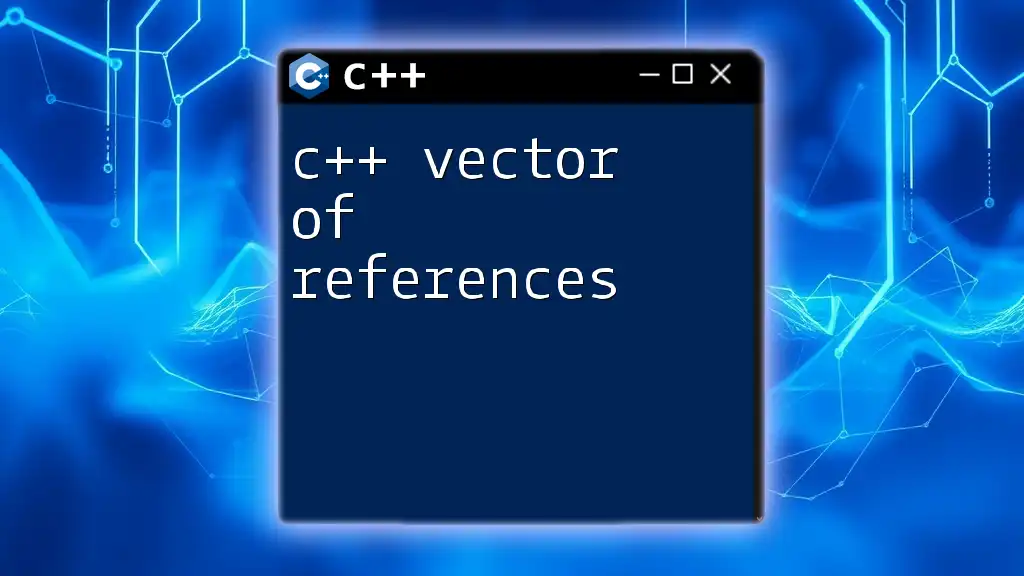
Additional Resources
To deepen your knowledge, consider exploring online C++ courses, forums, and coding challenges that focus specifically on advanced C++ features and best practices.