C++ pointers are variables that store memory addresses, enabling direct memory access and manipulation in your programs.
Here's a simple example demonstrating how to declare a pointer, assign it, and access its value:
#include <iostream>
int main() {
int number = 42; // An integer variable
int* pointer = &number; // A pointer that holds the address of 'number'
std::cout << "Value of number: " << number << std::endl; // Outputs: 42
std::cout << "Address of number: " << pointer << std::endl; // Outputs the memory address
std::cout << "Value via pointer: " << *pointer << std::endl; // Outputs: 42
return 0;
}
Understanding Pointers
What are Pointers?
Pointers are a fundamental concept in C++ that provide significant control over memory management. A pointer is a variable that holds the memory address of another variable. This feature enables developers to manipulate memory directly, facilitating efficient data handling and complex data structures, such as linked lists and trees.
Why Use Pointers?
Using pointers in C++ presents several advantages:
- Memory Efficiency: Pointers allow dynamic memory allocation, which optimizes the use of memory by enabling the allocation of memory blocks only when needed.
- Fast Data Access: Since pointers hold memory addresses, accessing data through pointers can be quicker than by using standard variable references.
- Enhanced Data Structures: Pointers are essential for creating advanced data structures that can grow or shrink in size, such as linked lists and trees.

Understanding Memory in C++
The Role of Memory Management
Memory in C++ is generally divided into two main areas: the stack and heap. The stack is used for static memory allocation, which involves variables whose size is determined at compile-time. Conversely, the heap is utilized for dynamic memory allocation, allowing for data structures that can change in size during runtime.
How C++ Handles Memory
C++ utilizes both static and dynamic memory allocation techniques. In static allocation, the lifetime of a variable is defined by its scope, while in dynamic allocation, memory is manually allocated and deallocated by the programmer, offering greater flexibility but also requiring careful management to avoid memory leaks.

Basics of Pointers
Pointer Declaration and Initialization
Declaring and initializing a pointer is straightforward. The syntax is as follows:
data_type* pointer_name;
To initialize a pointer, you provide the address of a variable using the address-of operator (&):
int a = 5;
int* ptr = &a; // Pointer ptr holds the address of variable a
Accessing Pointer Values
To access the value stored at the address a pointer points to, you use the dereference operator (*):
int value = *ptr; // value will be 5
This ability to reference and dereference values makes pointers powerful in manipulating data.

Types of Pointers
Null Pointers
A null pointer is a pointer that does not point to any valid memory location. It indicates that the pointer is not currently assigned to point to an object or variable.
int* nullPtr = nullptr; // Null pointer using C++11
Using a null pointer helps prevent accidental dereferencing that can lead to undefined behavior.
Void Pointers
Void pointers are pointers that can point to any data type without the need for explicit type conversion. They are particularly useful in generic programming.
void* voidPtr = &a; // Stores address of an int variable
To dereference a void pointer, you must first cast it to the appropriate type.
Pointers to Pointers
A pointer to a pointer is simply a pointer that holds the address of another pointer. This allows for situations where you may need to manipulate pointers themselves.
int** doublePtr = &ptr; // Pointer to pointer to an int
Function Pointers
Function pointers enable the referencing of functions, allowing you to call functions dynamically and pass them as parameters.
void (*funcPtr)(int) = &someFunction; // Pointer to a function taking an int
This feature can be particularly useful in callback mechanisms and implementing event-driven programming.

Common Pointer Operations
Pointer Arithmetic
Pointer arithmetic allows for mathematical operations to be performed on pointers. Given a pointer pointing to a base address, you can add or subtract integer values to navigate through an array or a memory space.
int arr[] = {10, 20, 30};
int* p = arr;
int secondElement = *(p + 1); // Will be 20
Pointers and Arrays
Pointers are inherently tied to arrays. When you declare an array name, it serves as a pointer to the first element of the array. This close relationship allows you to iterate through arrays and manipulate them using pointers.
for (int i = 0; i < 3; i++) {
std::cout << *(arr + i) << " "; // Accessing array elements using pointers
}

Memory Management with Pointers
Dynamic Memory Allocation
Dynamic memory allocation in C++ is performed using the `new` operator, which allocates memory on the heap. Remember to use `delete` after you are finished with the dynamically allocated memory to prevent memory leaks.
int* dynamicArray = new int[5]; // Allocate memory for an array of 5 integers
delete[] dynamicArray; // Deallocate the memory
Common Pitfalls in Pointer Management
When working with pointers, some common pitfalls include memory leaks and dangling pointers. A memory leak occurs when dynamically allocated memory is not released, causing the program to consume excess memory over time. On the other hand, a dangling pointer points to a memory location that has already been freed, leading to undefined behavior if dereferenced.
To prevent these issues, always ensure that dynamic memory is paired with corresponding deallocation, and set pointers to `nullptr` after deletion.
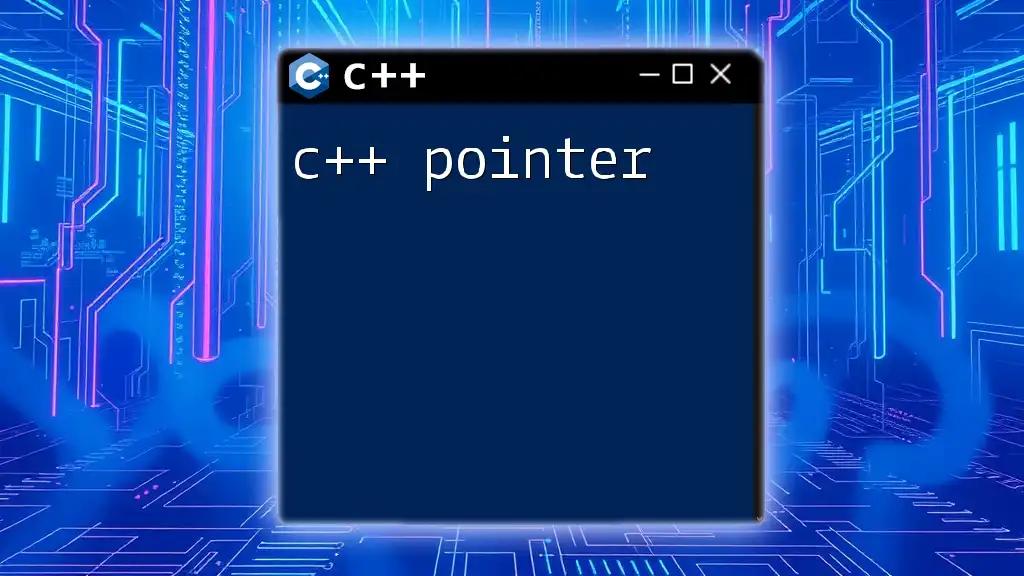
Best Practices for Using Pointers
When to Use Pointers
Avoid using pointers unless necessary. They should primarily be used for performance-critical applications, instances where dynamic memory allocation is essential, or when dealing with low-level system programming.
Safety and Pointers
To enhance the safety of pointer usage, consider these tips:
- Initialize pointers upon declaration.
- Use smart pointers (like `std::unique_ptr` or `std::shared_ptr`) wherever applicable to handle memory automatically.
- Perform checks for null before dereferencing pointers.
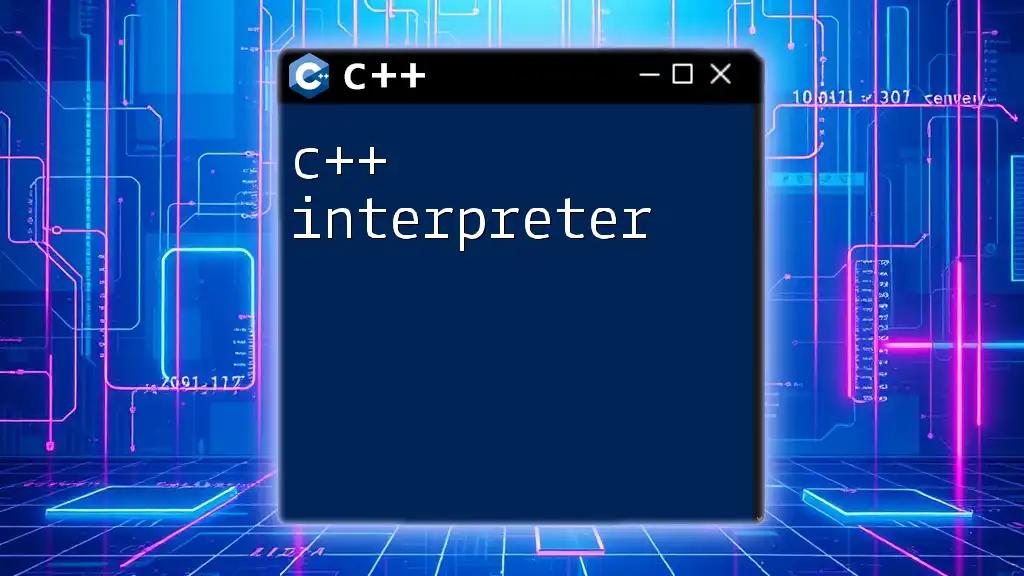
Conclusion
In this exploration of C++ pointers explained, we've covered the fundamental concepts, types, and best practices associated with pointers. Mastery of pointers is essential for any programmer looking to utilize C++ effectively, particularly in contexts requiring intricate memory management. Practice manipulating pointers to strengthen your understanding and proficiency in C++.

Additional Resources
Further Reading and References
For those eager to dive deeper into C++ pointers, consider exploring these resources:
- Classic C++ programming textbooks
- Online courses focused on C++ fundamentals
- Official C++ documentation and tutorials
FAQs about Pointers in C++
Questions surrounding pointers are common. Be sure to check forums and communities for clarifications, as discussing with peers can greatly enhance your understanding and practical application of pointers in C++.