In C++, you can measure the elapsed time of code execution using the `<chrono>` library, which provides high-resolution clock functionality. Here's an example code snippet demonstrating how to measure the time taken by a code block:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Code block to measure
for (int i = 0; i < 1000000; ++i); // Example loop
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> elapsed = end - start;
std::cout << "Time elapsed: " << elapsed.count() << " seconds\n";
return 0;
}
Understanding Time in C++
The Importance of Time Measurement in Programming
In programming, tracking time can be essential for a variety of reasons. Developers often need to assess how long functions take to execute or how quickly an application can respond to user inputs. This is particularly important when aiming to optimize performance, ensuring that applications run efficiently.
Common use cases for measuring time in programming include:
- Performance optimization: Identifying bottlenecks in code and understanding where improvements can be made.
- Timing processes: Establishing how long certain operations take, which can be crucial for tasks like data processing or network requests.
- Measuring intervals: Timing events continuously, for example, in real-time applications, gaming, or user interactions.
C++ Time Utilities
When it comes to handling time in C++, various libraries can assist. The most notable are `<chrono>` for high-resolution timing, `<ctime>` for traditional time functions, and others. However, `<chrono>` is widely regarded as the most efficient and precise for modern C++ applications.
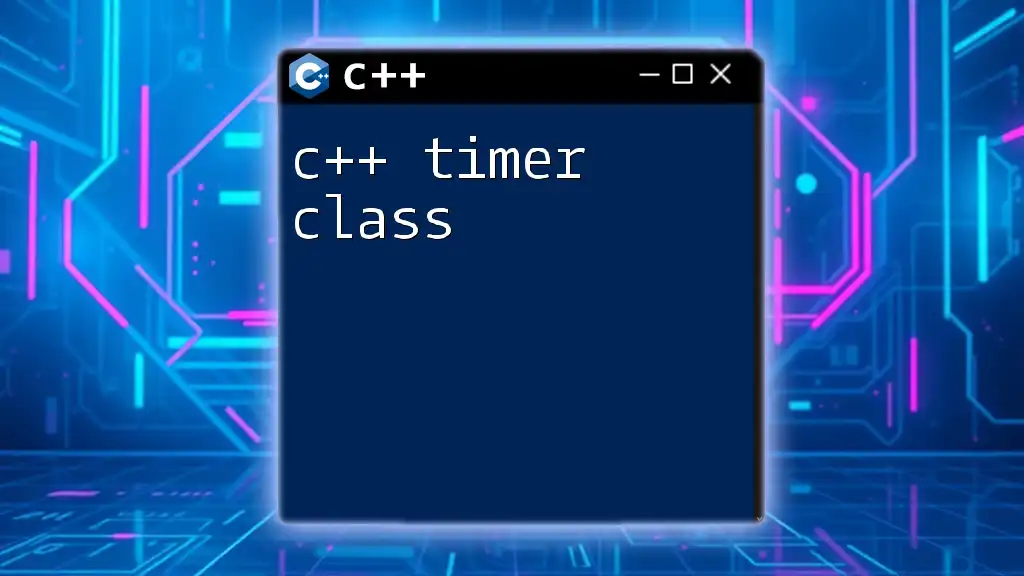
Getting Started with `<chrono>`
Introduction to `<chrono>`
The `<chrono>` library was introduced in C++11 and is designed to simplify time-related tasks. This library provides high-resolution clocks, durations, and time points that allow developers to measure time intervals with ease.
Using `<chrono>` shows advantages compared to traditional methods like `clock()` from `<ctime>`, particularly in terms of flexibility and accuracy.
Components of `<chrono>`
Duration
At the heart of `<chrono>` are the durations, represented by `std::chrono::duration`. This class template allows developers to represent a time span in various units, such as:
- Seconds
- Milliseconds
- Microseconds
- Nanoseconds
For example, you can create a duration representing 1 second like so:
std::chrono::duration<int> one_second(1);
Time Point
Another critical component is the time point, represented by `std::chrono::time_point`. A time point represents a specific moment in time, relative to a clock's epoch. By understanding time points, we can establish when particular events occur.
Clocks
`<chrono>` offers several clocks crucial for time measurement:
- System Clock: Represents the current calendar time.
- Steady Clock: Provides a monotonic clock that cannot go backward; ideal for measuring elapsed time.
- High-Resolution Clock: Offers the smallest tick period but may not be steady.
Each clock serves particular use cases depending on the context in which you need to measure time.
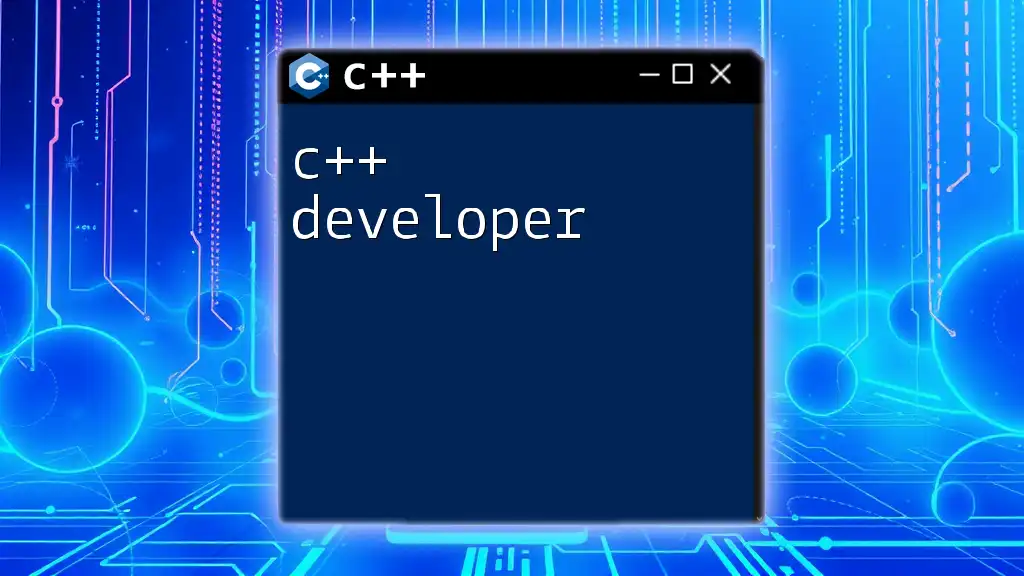
Measuring Time Elapsed
Capturing Start and End Time
To measure time elapsed during execution, one effective approach is to create a simple Timer class. This class can capture the start and end time for any process.
Example: A Basic Timer Class
#include <chrono>
class Timer {
public:
void start() {
begin = std::chrono::high_resolution_clock::now();
}
void stop() {
end = std::chrono::high_resolution_clock::now();
}
double elapsed() {
return std::chrono::duration<double, std::milli>(end - begin).count();
}
private:
std::chrono::high_resolution_clock::time_point begin, end;
};
In this class:
- `start()` captures the current time when the timer starts.
- `stop()` captures the time when the timer stops.
- `elapsed()` calculates the difference in milliseconds between the start and stop times, providing the total elapsed time in a human-readable format.
Example Usage of Timer Class
To see the effectiveness of the Timer class, let's explore how to time a function using this class.
#include <iostream>
#include <thread>
void some_function() {
std::this_thread::sleep_for(std::chrono::seconds(2));
}
int main() {
Timer timer;
timer.start();
some_function();
timer.stop();
std::cout << "Time elapsed: " << timer.elapsed() << " milliseconds." << std::endl;
return 0;
}
In this example, `some_function()` simulates a time-consuming task by sleeping for 2 seconds. When executed, this program will output the time elapsed during the function call, demonstrating how the Timer class provides a straightforward way to measure execution time.
Practical Applications of Timing Functions
Understanding how to capture elapsed time has real-world applications:
- Performance Testing: By timing functions, developers can identify slow processes and optimize where necessary.
- Game Loop Timing: In game development, loops need exact timing to maintain a smooth gameplay experience.
- Event Timing in User Interfaces: Ensuring animations or transitions occur fluidly requires accurate timing.
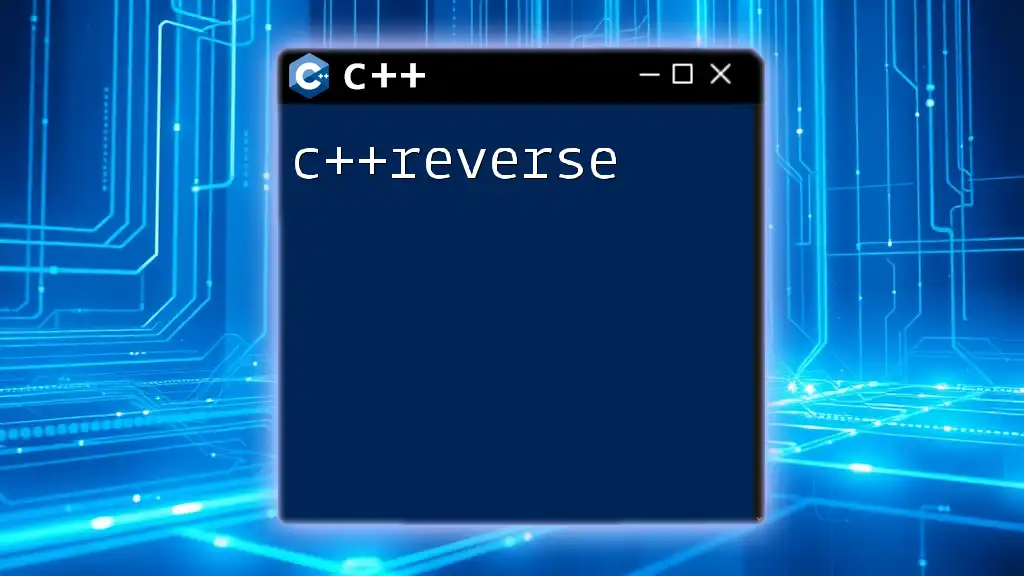
Advanced Time Elapsed Techniques
Measuring Computational Time vs. Real Time
When assessing time in C++, it's crucial to differentiate between computational time and real time. Computational time tracks how long the CPU spends executing a piece of code. In contrast, real time includes all time passages (including waiting on input/output).
For precision in timing, especially for short-lived functions, it is usually better to measure computational time.
Using `std::chrono::steady_clock`
For elapsed time measurement, `std::chrono::steady_clock` is a robust choice. It offers a monotonic time point that guarantees the time does not regress, making it ideal for measuring durations.
Here’s how to use `steady_clock` effectively:
auto start = std::chrono::steady_clock::now();
// Perform some computations
auto end = std::chrono::steady_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count();
std::cout << "Elapsed time: " << elapsed << " milliseconds." << std::endl;
This code captures the start and end time, then calculates the duration in milliseconds. Using `steady_clock` enhances reliability across varied environments, ensuring the elapsed time measured is accurate.
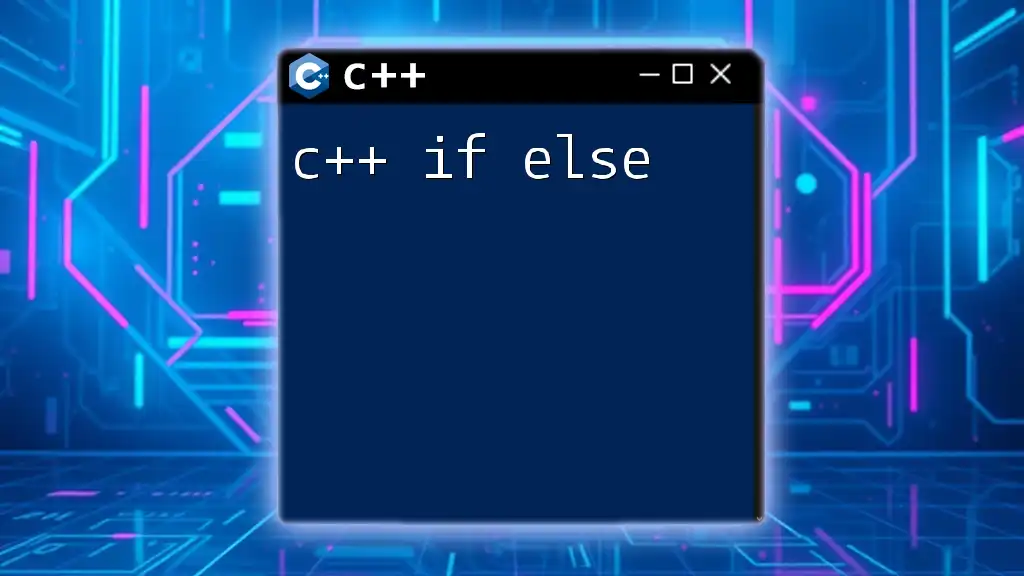
Best Practices for Time Measurement
Avoiding Common Pitfalls
When timing code execution, developers might fall into traps such as measuring too short durations, which can produce misleading results due to the resolution limits of the clock. Always consider the duration you need to measure; longer intervals often yield more accurate results.
Precision and Accuracy
Factors that can significantly impact the precision and accuracy of timing measurements must be understood:
- The choice of clock can influence performance.
- System load and resource availability may cause inconsistencies in measured time.
Choosing the right time unit based on the context — milliseconds for user interface timing, microseconds for computational tasks — is vital for effective timing strategies.
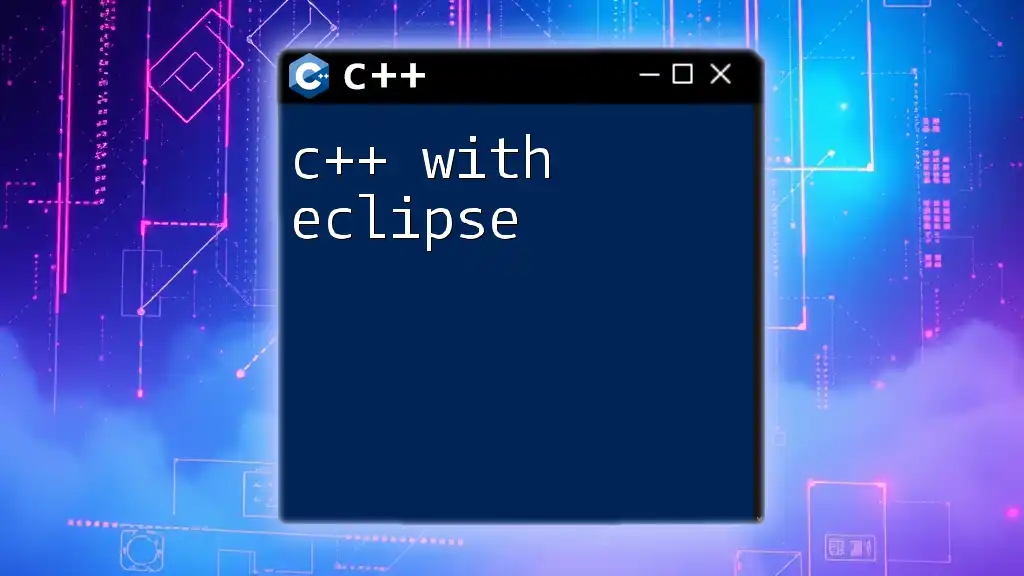
Conclusion: Mastering Time Management in C++
Understanding how to measure time elapsed in C++ provides vast opportunities for optimizing code and enhancing overall application performance. By effectively utilizing the `<chrono>` library, developers can track function execution, assess performance, and make informed decisions to improve their applications.
Incorporating these timing practices into your programming projects will ensure that your applications run smoothly and efficiently. As you explore and implement different timing techniques, you'll uncover new insights into code performance and reliability.
Further Resources
For more detailed guidance, documentation, and resources on timing in C++, consider exploring:
- The official C++ documentation for `<chrono>`.
- Programming books that focus on performance optimization.
Call to Action
Start implementing a timer in your projects today! Whether you're looking to optimize performance or deepen your understanding of time measurement in C++, taking the first step will set you on the path to mastery. Subscribe to learn more quick and concise C++ tips and tricks!