In C++, you can measure and display the elapsed time in milliseconds using the `chrono` library, which allows for precise timing of code execution. Here's a simple code snippet to demonstrate how to do this:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Code to be measured goes here
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double, std::milli> duration = end - start;
std::cout << "Elapsed time: " << duration.count() << " ms" << std::endl;
return 0;
}
Understanding Time in C++
What is Time in C++?
In C++, time is measured using different units depending on the precision required for an application. Generally, time is tracked in seconds, milliseconds, microseconds, and nanoseconds. Each unit serves a purpose, particularly in performance-sensitive applications where timing accuracy can significantly impact the outcome.
Why Use Milliseconds?
Milliseconds provide a fine granularity for timing, making them especially useful in scenarios like performance benchmarking, real-time applications, and tasks that require quick response times. For example, in game development, updating the frame rate, or measuring the response time of certain functionalities, milliseconds offer a practical precision that ensures smoother and faster user experiences.
Using milliseconds can help prevent issues that may arise from less detailed time measurements, such as measuring processes that execute very quickly.

Getting Started with Time in C++
Including Necessary Headers
To begin measuring time in C++, you need to include the appropriate library. The `chrono` library is a part of the C++11 standard and provides various clock types to facilitate time measurement:
#include <iostream>
#include <chrono>
The `chrono` Library
The `chrono` library is designed to provide a rich set of time measurement utilities. It introduces several clock types, namely `steady_clock`, `high_resolution_clock`, and `system_clock`. Understanding these types is crucial to effectively using time measurements in your applications.

C++ Get Time in Milliseconds
Using `std::chrono::steady_clock`
`steady_clock` is ideal for measuring intervals, as it is guaranteed to be steady and won't be affected by changes in the system clock (like time zone changes or daylight saving time adjustments).
Example: Measuring Time with `std::chrono::steady_clock`
Here’s how you can measure time using `steady_clock`:
auto start = std::chrono::steady_clock::now();
// Code block to measure
auto end = std::chrono::steady_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count();
std::cout << "Time taken: " << duration << " ms" << std::endl;
In this example, the code records the start and end times around a block of code you wish to measure, calculates the duration, and expresses that duration in milliseconds using `duration_cast`.
Using `std::chrono::high_resolution_clock`
`high_resolution_clock` offers the highest possible resolution available, making it suitable for applications needing extremely accurate timing measurements. Keep in mind that its precision can vary per system.
Example: Measuring Time with `std::chrono::high_resolution_clock`
To measure execution time, you would use:
auto start = std::chrono::high_resolution_clock::now();
// Code block to measure
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count();
std::cout << "Time taken: " << duration << " ms" << std::endl;
As with `steady_clock`, this code captures the start and end times around a particular code segment, providing the duration in milliseconds afterwards.
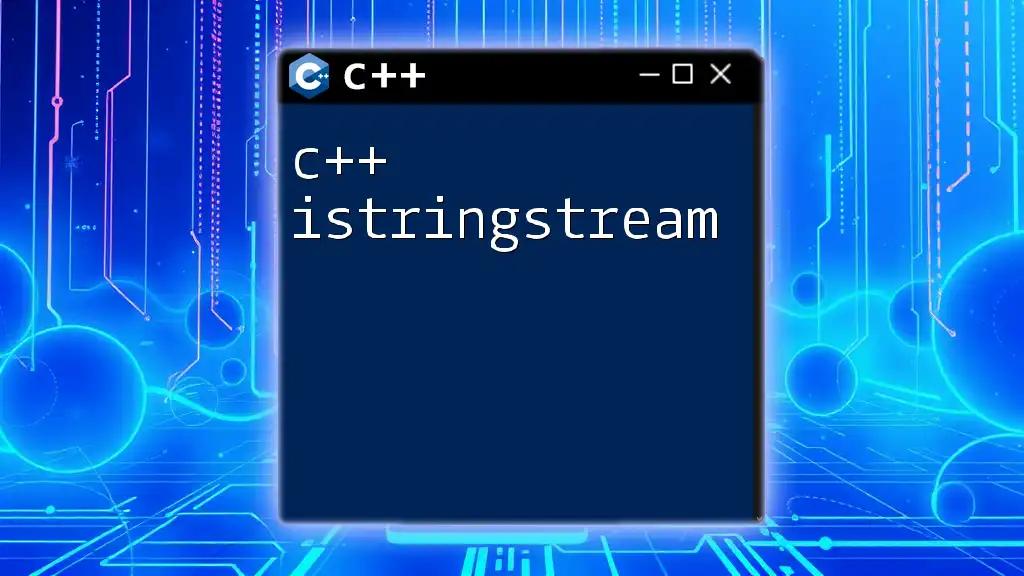
Practical Applications of Time Measurement
Performance Testing
Timing is an essential tool in performance testing and benchmarking. It enables developers to identify bottlenecks in their code and optimize execution paths to enhance performance. For instance, if an algorithm runs slower than expected, measuring its execution time offers direct insights into areas for improvement.
Example: Benchmarking Algorithms
Consider you have a function you want to benchmark:
void exampleFunction() {
// some logic that takes time
}
auto start = std::chrono::high_resolution_clock::now();
exampleFunction();
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start).count();
std::cout << "Function execution time: " << duration << " ms" << std::endl;
This allows you to easily see how long the function takes to complete, which can be particularly informative during the optimization process.
Real-time Applications
In game development and animations, measuring execution time is vital for ensuring that frames update correctly without lag. For instance, if a game’s graphics are running too slowly, developers can analyze timing to adjust assets or improve code efficiency, ensuring players experience smooth gameplay.
Similarly, in data processing tasks, measuring the time taken to process large amounts of data can help in assessing whether your approach needs to be optimized, especially when working on data-heavy applications.

Common Pitfalls and Tips
Misunderstanding Clock Types
One common pitfall is misunderstanding the different types of clocks. The `steady_clock` is perfect for measuring time intervals, while `high_resolution_clock` is best for high-accuracy timing. Choosing the wrong clock type can lead to inaccurate measurements (e.g., if you need a steady interval, using high-resolution clock may not be appropriate).
Accuracy and Resolution
Timing accuracy can be affected by numerous factors including system load, CPU scheduling, and the inherent precision of the clock being used. To improve accuracy:
- Isolate the code block you are measuring to prevent background processes interfere.
- Use the `std::chrono` clocks appropriately based on the requirement of precision for your specific application.
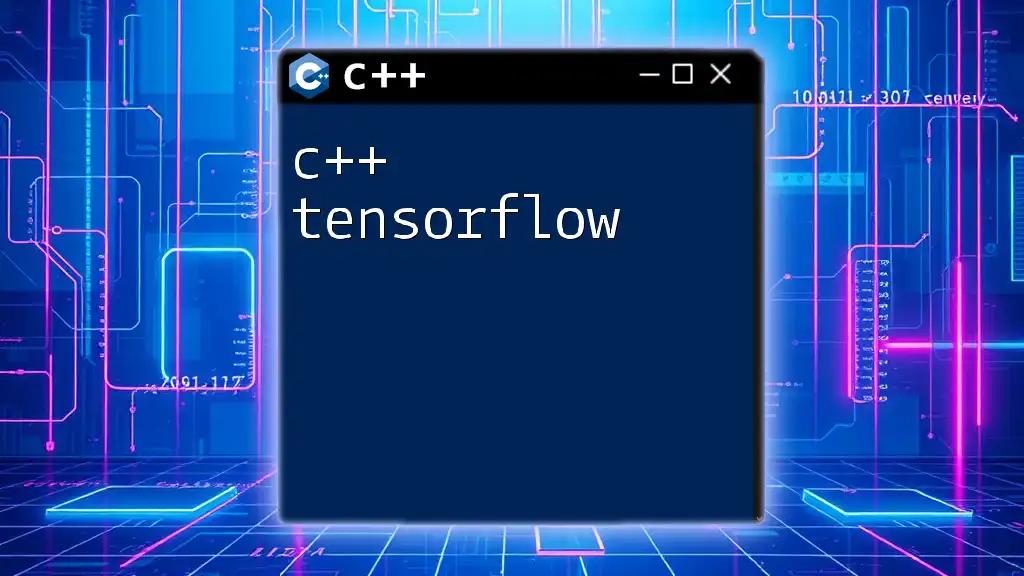
Conclusion
Understanding C++ time in ms is fundamental for effective programming in contexts where performance and timing are critical. By utilizing the `chrono` library, you’re equipped to measure and manage execution time with accuracy and ease. As you continue to develop your skills, applying these techniques will not only enhance your code quality but also improve overall application performance.
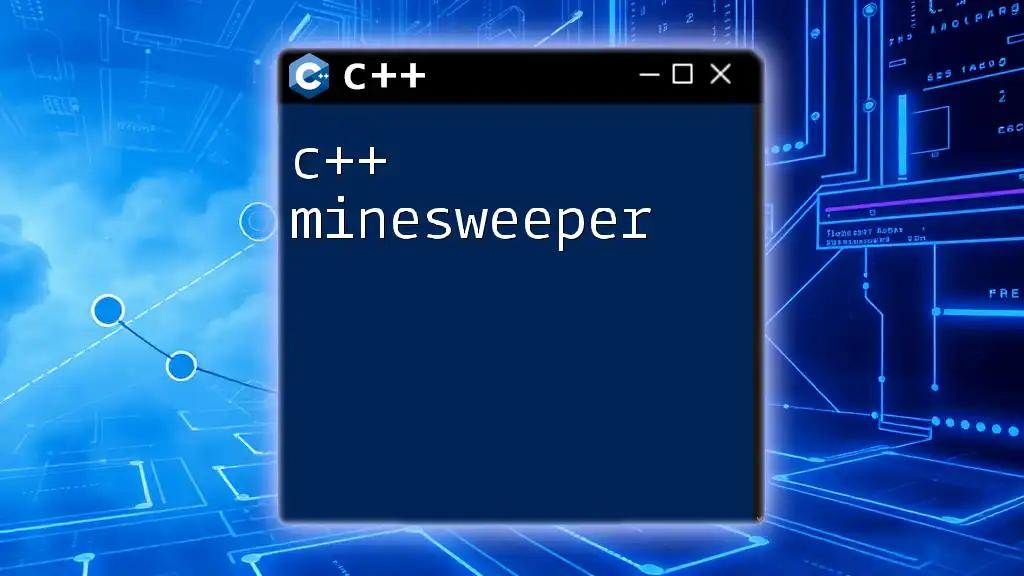
Additional Resources
To dive deeper, I recommend exploring additional resources like books on advanced C++ programming, online courses, and the official documentation for the `std::chrono` library. These will provide you with comprehensive knowledge and practices to optimize your use of time measurements in your programming endeavors.