The `<ctime>` header in C++ provides functionalities for manipulating date and time, allowing you to retrieve the current time, format it, and perform time-based calculations. Here's a simple example of how to use it to get the current time:
#include <iostream>
#include <ctime>
int main() {
std::time_t currentTime = std::time(nullptr);
std::cout << "Current time: " << std::ctime(¤tTime);
return 0;
}
What is time.h?
The `time.h` header file in C++ provides a set of library functions for manipulating and formatting dates and times. This library is crucial for any C++ program that needs to keep track of time, schedule tasks, or handle timestamps. The functionality offered by `time.h` can accommodate a wide array of applications, from simple console applications to complex time-dependent systems.
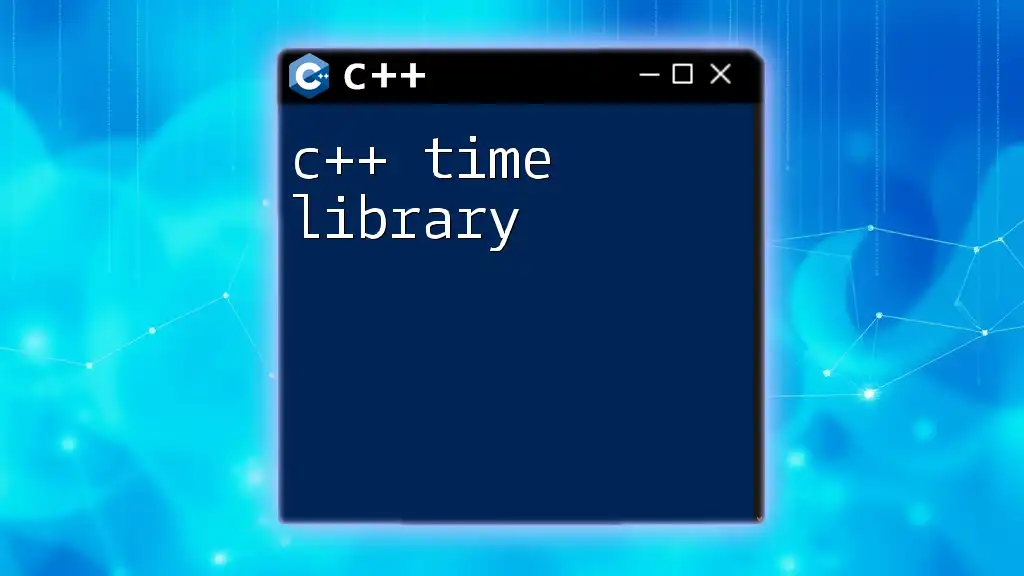
Importance of Time Management in C++
Efficient time management is crucial in programming, especially for applications that require precise timing or time tracking. Whether you’re developing games, scheduling algorithms, or logging systems, how you handle time can greatly influence the performance and user experience of your application. Real-world applications that benefit from time management include:
- Task schedulers that must accurately manage multiple operations.
- Gaming engines that require precise timing to handle processes like animation and refreshing frames.
- Data logging systems that need timestamps for record-keeping.
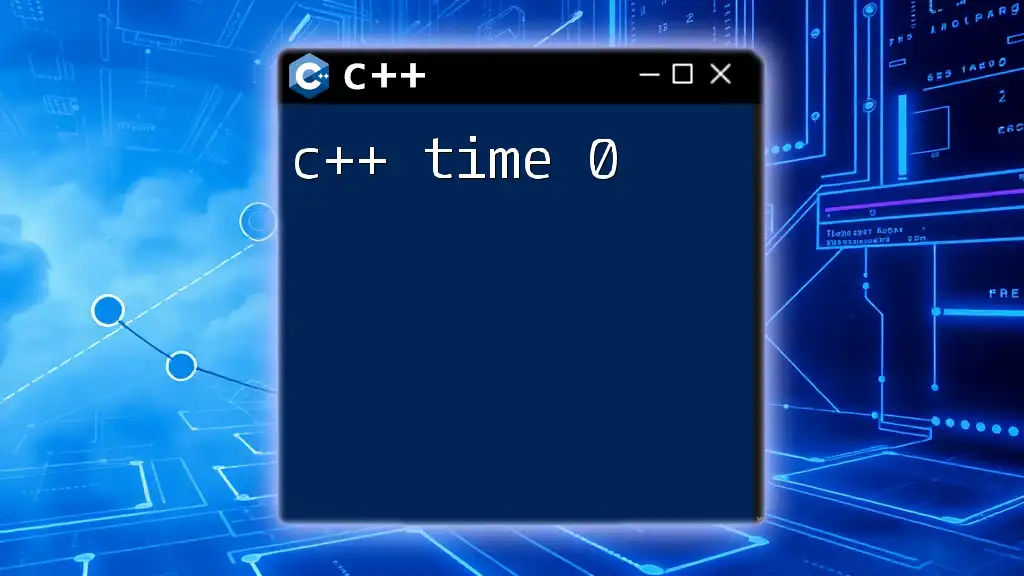
Understanding the time.h Header File
What Does time.h Provide?
`time.h` includes a variety of essential functions for working with system time and dates, such as getting the current time, measuring elapsed time, and converting time formats. Understanding these functions is key for effectively implementing time management in your C++ applications.
Including time.h in Your C++ Program
To utilize the functions provided by `time.h`, you must include it in your program. This is accomplished with the following code:
#include <ctime>
By adding this line at the top of your source file, you gain access to all the time functionalities available in C++.
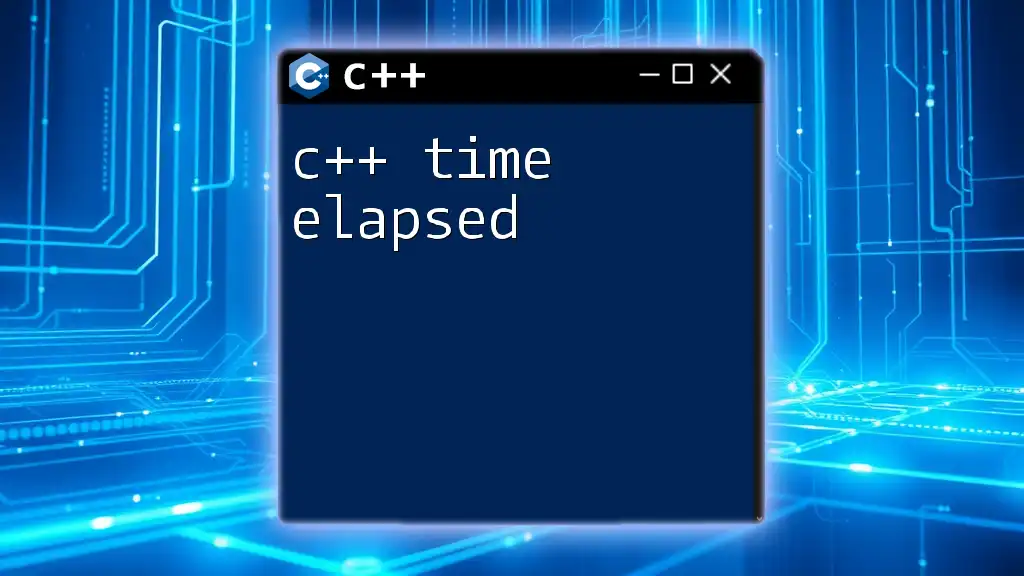
Key Functions in time.h
Getting the Current Time
The `time()` function is one of the simplest and most widely used functions within `time.h`. It retrieves the current calendar time as a `time_t` object. To obtain the current time, you can use the following code snippet:
time_t currentTime = time(0);
Here, `currentTime` holds the time since the Epoch (00:00:00 UTC on 1 January 1970).
Converting Time Formats
When you retrieve the current time, it’s typically in a format that's not easily readable. Functions like `ctime()` and `asctime()` convert `time_t` objects into string representations. Use them as follows:
char* dt = ctime(¤tTime);
This will convert the `time_t` object into a more human-readable string format, making it easy to understand the current date and time.
Working with Time Structures
Handling `time_t` directly can be limiting, so `time.h` provides the `struct tm`, which contains more detailed breakdowns of calendar time such as year, month, day, hour, and so on. You can populate and utilize this structure in the following way:
struct tm *localTime = localtime(¤tTime);
Here, `localTime` now contains a detailed representation of the current time based on your local timezone.
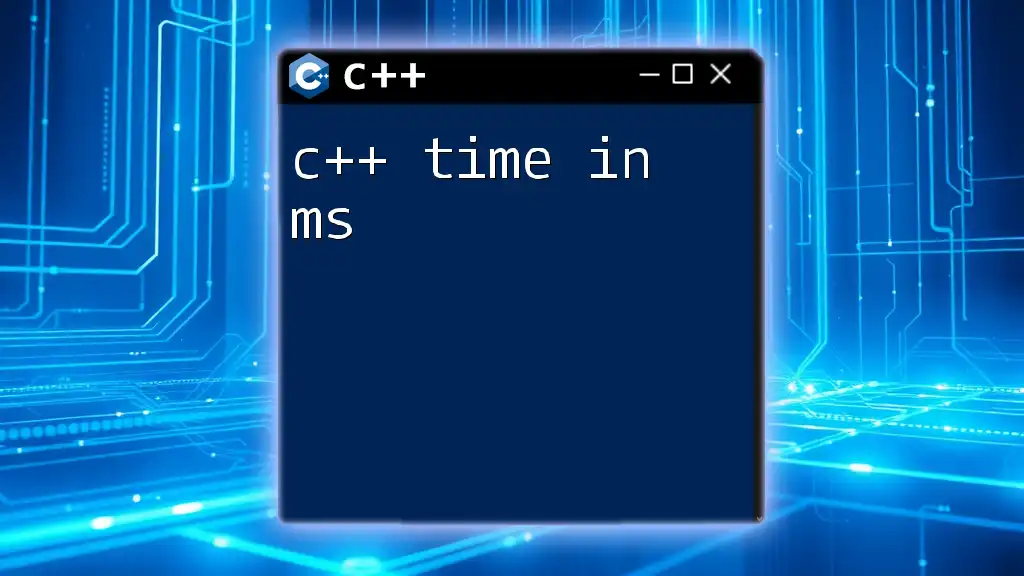
Time Manipulation Functions
Calculating the Difference Between Two Times
Another powerful feature of `time.h` is the ability to calculate the difference between two times using the `difftime()` function. This can be particularly useful for performance monitoring or measuring operation durations. Here's an example:
time_t startTime = time(0);
// ... some operations ...
time_t endTime = time(0);
double seconds = difftime(endTime, startTime);
In this example, `seconds` will hold the elapsed time in seconds between the start and end times.
Working with Time Zones
Understanding time zones is another critical aspect of time management. The `tzset()` function allows you to set or read time zone settings and will be helpful in applications that operate across multiple geographical areas. Sample code looks like this:
#include <ctime>
#include <cstdlib>
#include <iostream>
int main() {
setenv("TZ", "America/New_York", 1);
tzset();
time_t currentTime = time(0);
// Print out the local time for the set time zone
std::cout << ctime(¤tTime);
return 0;
}
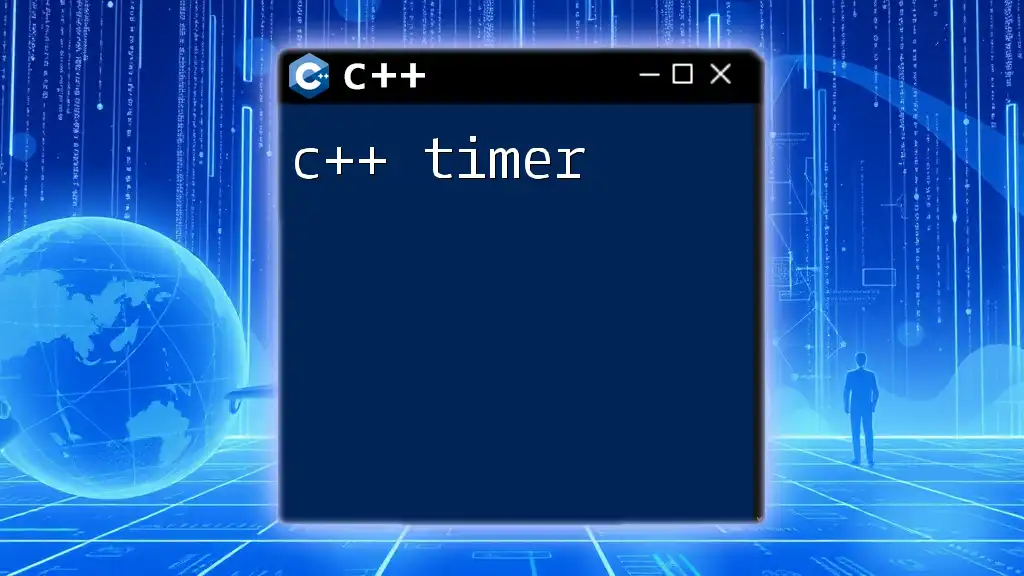
Advanced Features of time.h
Using High-Resolution Clocks
For tasks requiring low-latency operations or execution time measurements, you can employ the `clock()` function. This will return the processor time used by the program, which can be particularly useful. Here’s a sample usage:
clock_t start = clock();
// Code to be measured
clock_t end = clock();
double timeTaken = double(end - start) / CLOCKS_PER_SEC;
In this snippet, `timeTaken` will provide the duration of the operation in seconds, allowing you to optimize your algorithms effectively.
Generating Random Durations
When simulating tasks or creating timed events, you may want to implement a delay mechanism. Implementing `sleep()` can be a very effective way to generate delays:
#include <unistd.h> // For POSIX sleep function
sleep(5); // Pauses the program for 5 seconds
This coding snippet will put your program to sleep for the specified duration, allowing you to manage time-dependent tasks simply.
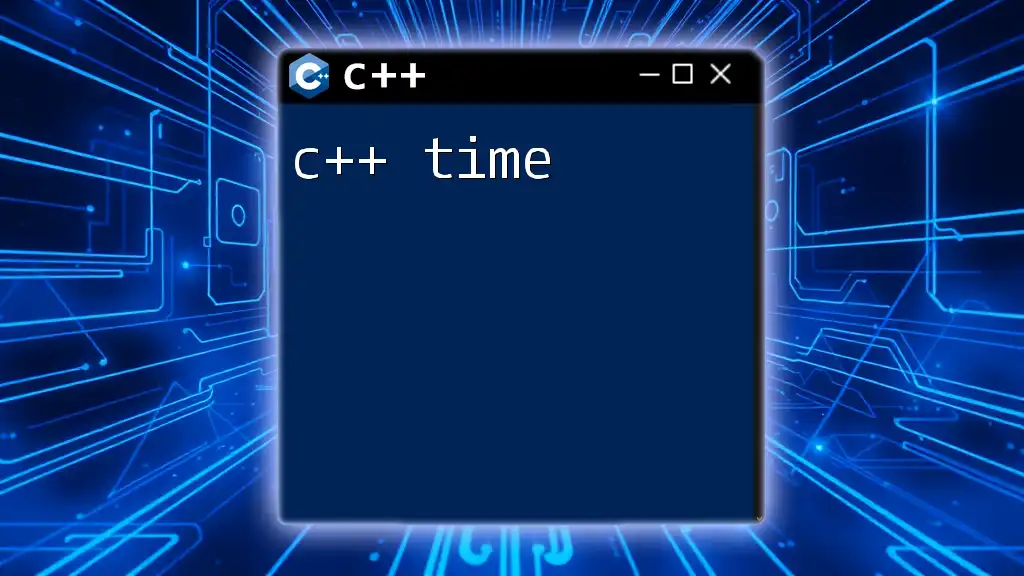
Common Issues and Troubleshooting
Common Errors with time.h
While working with `time.h`, developers may encounter errors related to time calculations, such as discrepancies between local and UTC time. Ensuring you are using the correct functions to convert between them is vital.
Debugging Time-Related Issues
When debugging your time calculations, consider logging the variables at different points in your code to display how they evolve with each function call. This approach can help identify and resolve any anomalies.
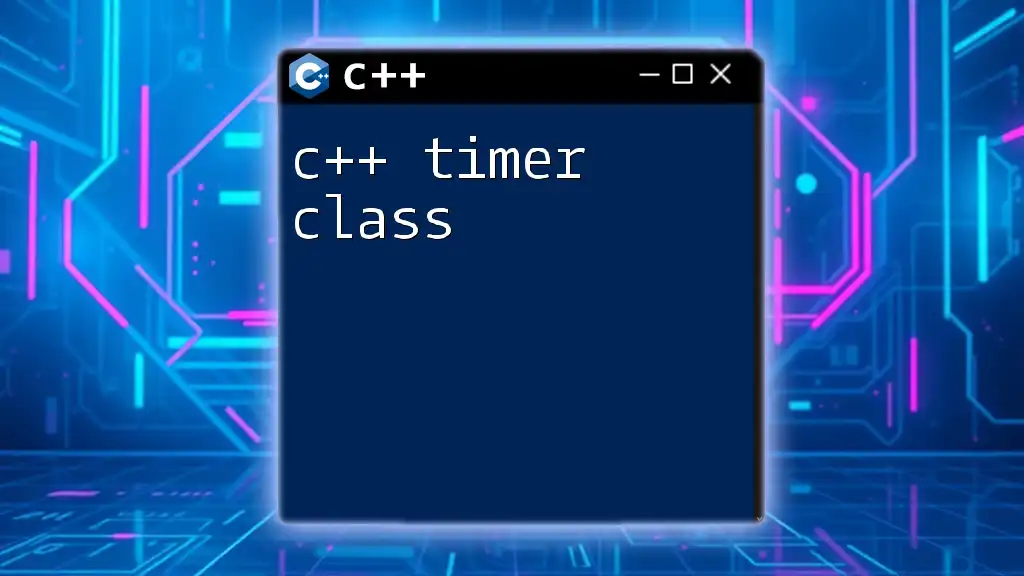
Practical Applications of time.h in Projects
Time Tracking Applications
You might build an application to log users' actions or events over time. Using `time.h`, you can accurately capture timestamps for every key event. For example:
time_t eventTime = time(0);
std::cout << "User logged in at: " << ctime(&eventTime);
Game Development and Time Management
When developing games, particularly those involving animations, precise time measurement is crucial. Implementing frame rates, timed events, and animations can greatly enhance user experience. The `time()` function can be key to optimizing frame rates and ensuring smooth gameplay.
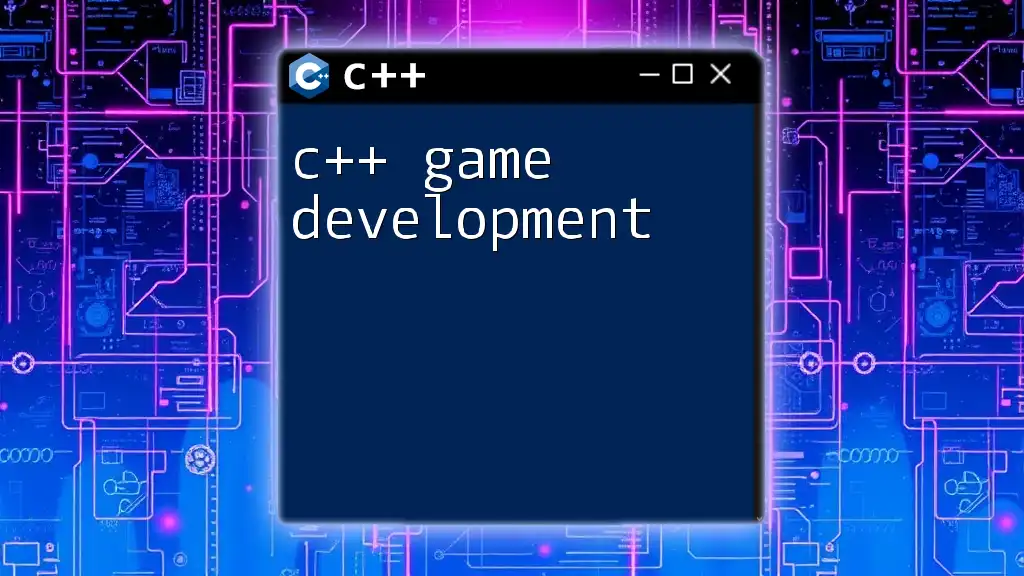
Conclusion
Incorporating `time.h` in your C++ projects helps you manage time effectively, whether for tracking performance, scheduling tasks, or developing engaging applications. Understanding how to utilize the various functions and structures provided by this header can significantly enhance the robustness and functionality of your code.
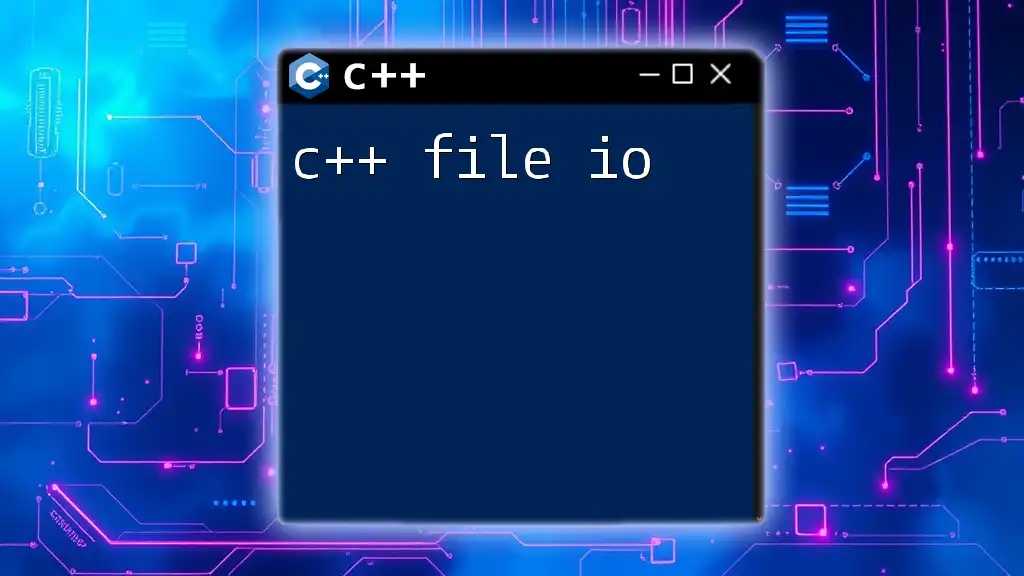
Additional Resources
For more in-depth exploration of `time.h` and time management in C++, consider visiting official documentation and exploring related tutorials that delve further into the subject. Keeping up-to-date with the latest developments in C++ will empower you to implement the most current practices in your programming solutions.