C++ type traits are a set of template classes that provide compile-time information about types, allowing for more flexible and optimized code through type introspection.
#include <iostream>
#include <type_traits>
template<typename T>
void checkTypeTraits() {
std::cout << "Is T an integral type? " << std::boolalpha << std::is_integral<T>::value << '\n';
}
int main() {
checkTypeTraits<int>(); // Output: Is T an integral type? true
checkTypeTraits<float>(); // Output: Is T an integral type? false
return 0;
}
Understanding Type Traits in C++
C++ type traits are a set of compile-time templates designed to provide information about types in a way that is accessible to C++ developers. They play a crucial role in generic programming, enabling developers to create flexible and reusable code by making decisions based on types. This capability makes the code not only safer but also more efficient.
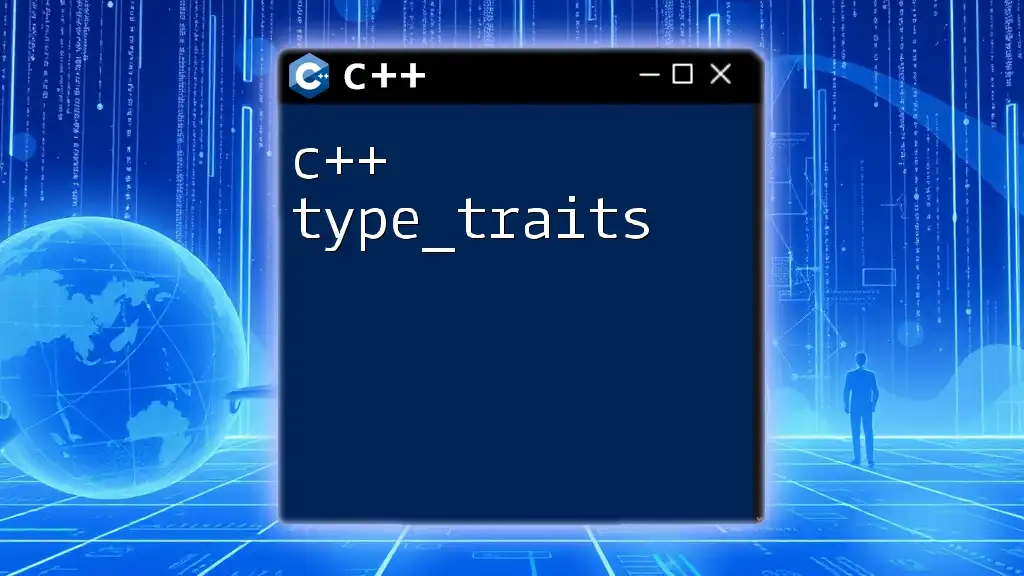
Why Use Type Traits?
Type traits enhance code flexibility and reusability, allowing developers to write template functions that behave differently based on the properties of types. For example, in a templated function, you could enable or disable specific behavior depending on whether the provided type is integral or floating-point.
Another significant application of type traits is in static type checking. By utilizing type traits, developers can catch type-related errors at compile time rather than at runtime, minimizing the risk of running into unexpected behavior in production code.
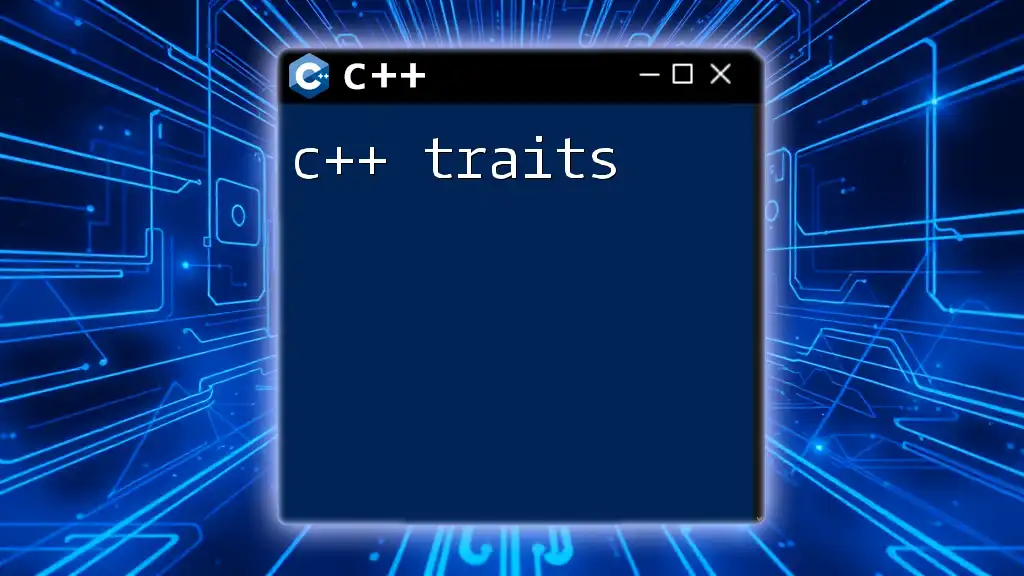
Use Cases for Type Traits
Type traits commonly find use in:
- Template specialization, where templates can be tailored for specific types, improving functionality and performance.
- SFINAE (Substitution Failure Is Not An Error), a feature that prevents compilation errors when a substitution fails, allowing the compiler to pick alternative template overloads.
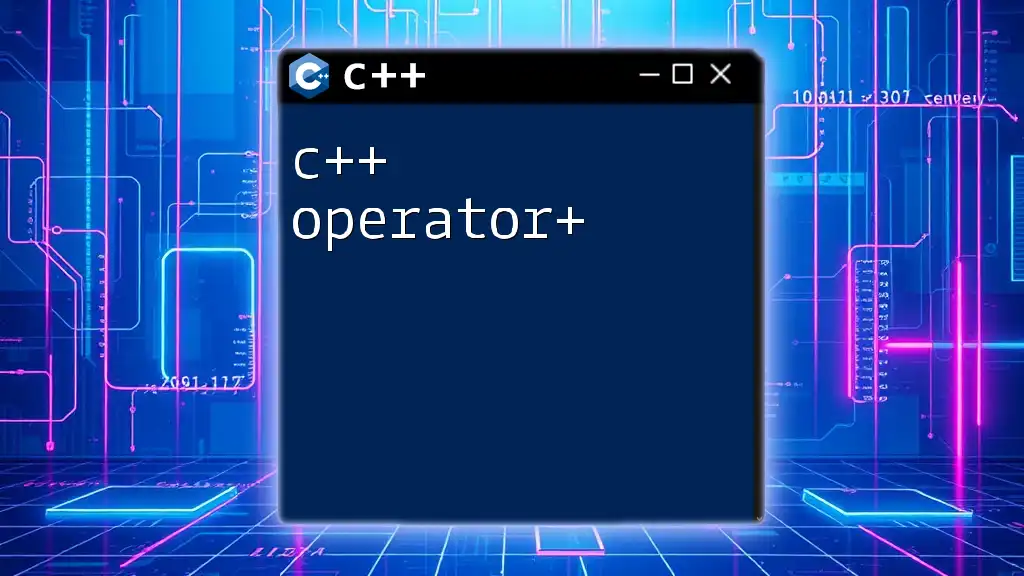
Overview of Common Type Traits
Fundamental Type Traits
-
is_null_pointer The `is_null_pointer` trait checks if a type is a null pointer type. This can guide decisions in template functions that require specific handling for null pointers.
#include <type_traits> static_assert(is_null_pointer<decltype(nullptr)>::value, "Should be a null pointer type");
-
is_integral The `is_integral` trait determines whether a type is an integral type (like int, char, etc.). This trait is often used in mathematical functions to enforce type limitations.
#include <type_traits> static_assert(is_integral<int>::value, "int should be an integral type"); static_assert(!is_integral<float>::value, "float should not be an integral type");
-
is_floating_point This trait checks if a type is a floating-point type (like float, double). This is valuable when defining functions that should only accept floating-point values.
#include <type_traits> static_assert(is_floating_point<double>::value, "double should be a floating-point type");
Type Modifiers
-
remove_const `remove_const` is useful when you want to create a version of a type without the `const` qualifier. This can help in scenarios where you need to modify const objects indirectly.
#include <type_traits> static_assert(std::is_same<remove_const<const int>::type, int>::value, "Should remove const");
-
remove_reference The `remove_reference` trait strips away the reference from a type, making it useful in template programming to work with value types.
#include <type_traits> static_assert(std::is_same<remove_reference<int&>::type, int>::value, "Should remove reference");
-
add_pointer This trait adds a pointer to a type, which is handy for creating pointer types from non-pointer types.
#include <type_traits> static_assert(std::is_same<add_pointer<int>::type, int*>::value, "Should add pointer");
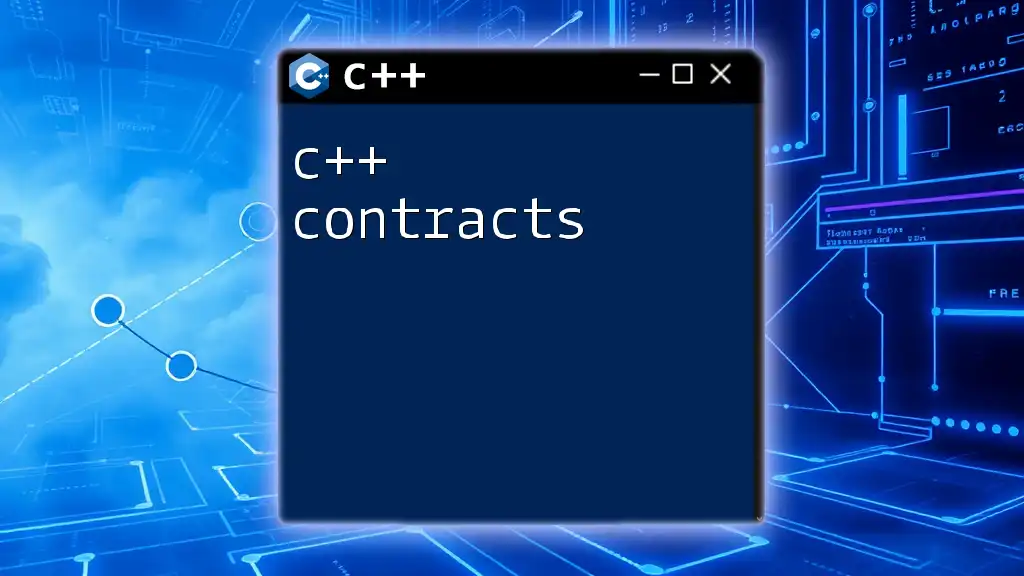
Advanced Type Traits
Conditional Type Traits
-
std::conditional `std::conditional` allows for type selection based on a boolean condition, enabling developers to define types inline based on compile-time conditions.
#include <type_traits> using MyType = std::conditional<is_integral<int>::value, int, float>::type; // MyType is int
Type Property Traits
-
is_same This trait checks if two types are identical. This is particularly useful in template specialization scenarios when you need to confirm that types match.
#include <type_traits> static_assert(is_same<int, int>::value, "Types should be the same"); static_assert(!is_same<int, float>::value, "Types should not be the same");
-
decay `decay` transforms a type into a form suitable for passing as a function argument by removing references and cv-qualifiers, yielding the underlying type.
#include <type_traits> static_assert(std::is_same<decay<int&>::type, int>::value, "Should decay the type");
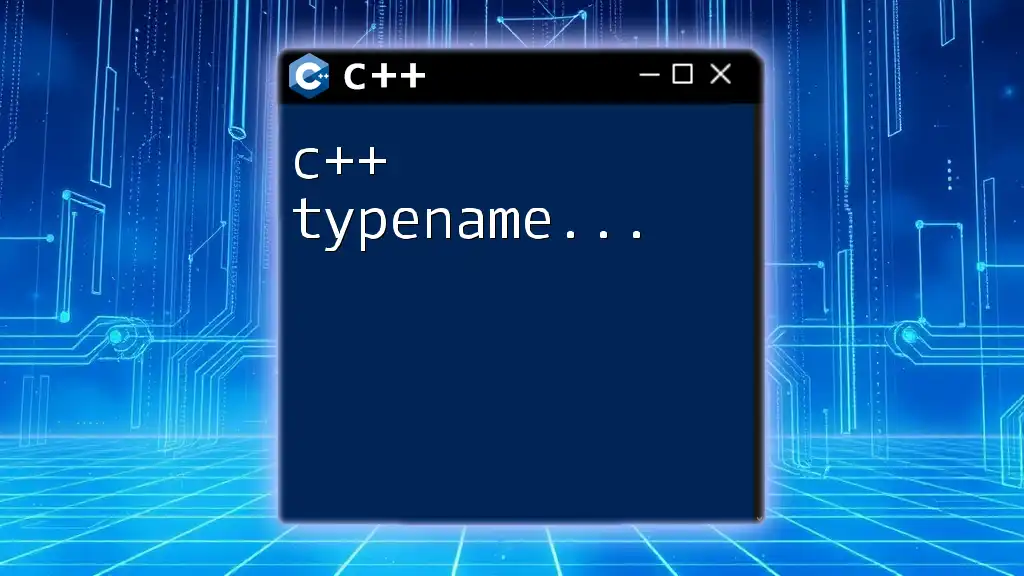
Practical Examples Using Type Traits
Creating a Type Inspector Class
You can create a simple type inspector class using type traits to dispatch behavior based on type:
#include <iostream>
#include <type_traits>
template<typename T>
class TypeInspector {
public:
void inspect() {
if (std::is_integral<T>::value) {
std::cout << "T is an integral type." << std::endl;
} else if (std::is_floating_point<T>::value) {
std::cout << "T is a floating-point type." << std::endl;
} else {
std::cout << "T is some other type." << std::endl;
}
}
};
// Usage
TypeInspector<int> inspector;
inspector.inspect(); // Output: T is an integral type.
Leveraging Type Traits in Template Metaprogramming
Type traits can also help in template specialization. For instance, you can specialize a function template for integral types:
#include <iostream>
#include <type_traits>
template<typename T>
void printType(T value) {
std::cout << "Generic type." << std::endl;
}
template<typename T>
typename std::enable_if<std::is_integral<T>::value>::type printType(T value) {
std::cout << "Integral type: " << value << std::endl;
}
template<typename T>
typename std::enable_if<std::is_floating_point<T>::value>::type printType(T value) {
std::cout << "Floating type: " << value << std::endl;
}
// Usage
printType(42); // Output: Integral type: 42
printType(3.14); // Output: Floating type: 3.14
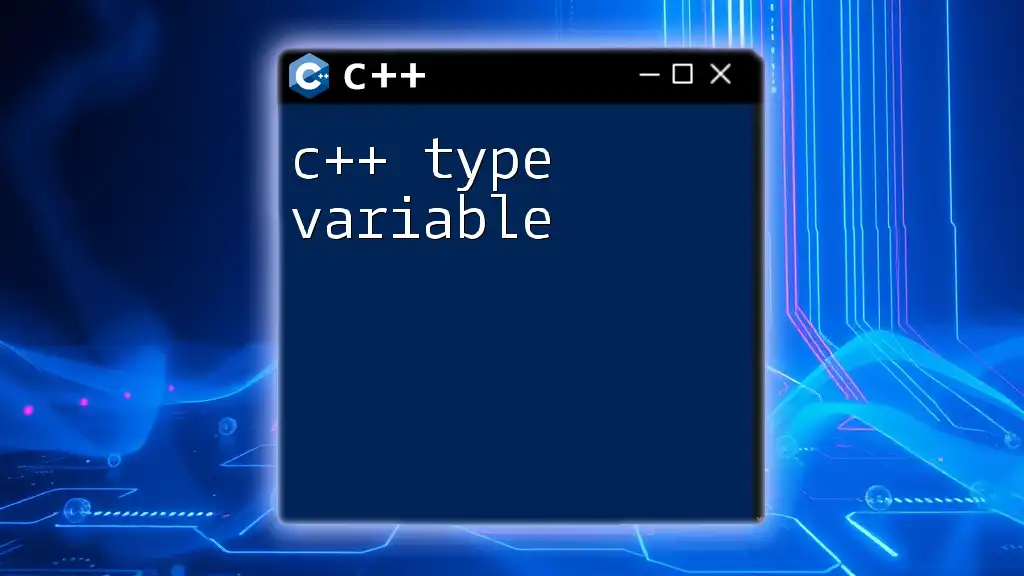
Real-world Applications of Type Traits
Utilizing Type Traits in Standard Library Components
C++ Standard Library (STL) components often utilize type traits to optimize algorithms. For example, operations in the STL might behave differently based on whether the types involved are integral, floating-point, or user-defined types.
Type Traits in Boost Libraries
Boost libraries extensively employ type traits. They provide enhanced utilities and functionalities for developers looking to handle types more effectively and safely, expanding upon the foundation laid by the standard library.
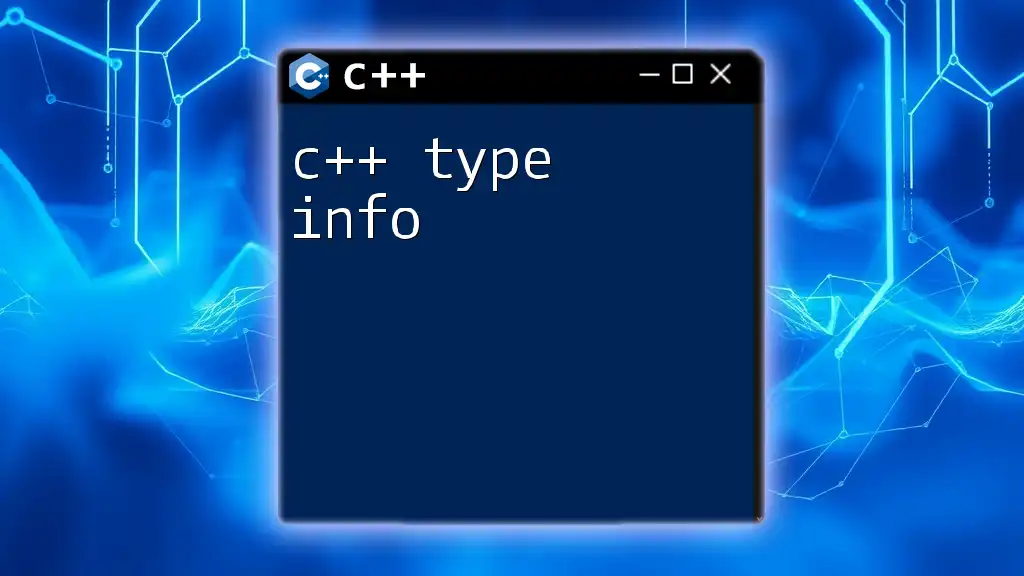
Best Practices for Using C++ Type Traits
Choosing the Right Type Trait
When selecting type traits, consider the intended use case. Avoid overcomplicating templates with unnecessary traits, opting for clarity wherever possible. Familiarize yourself with common type traits to understand their implications on your design.
Performance Considerations
The use of C++ type traits can impact compilation time. While they can offer performance benefits at runtime, be cautious not to introduce excessive complexity into your code. Strive for a balance between compile-time checks and runtime performance.
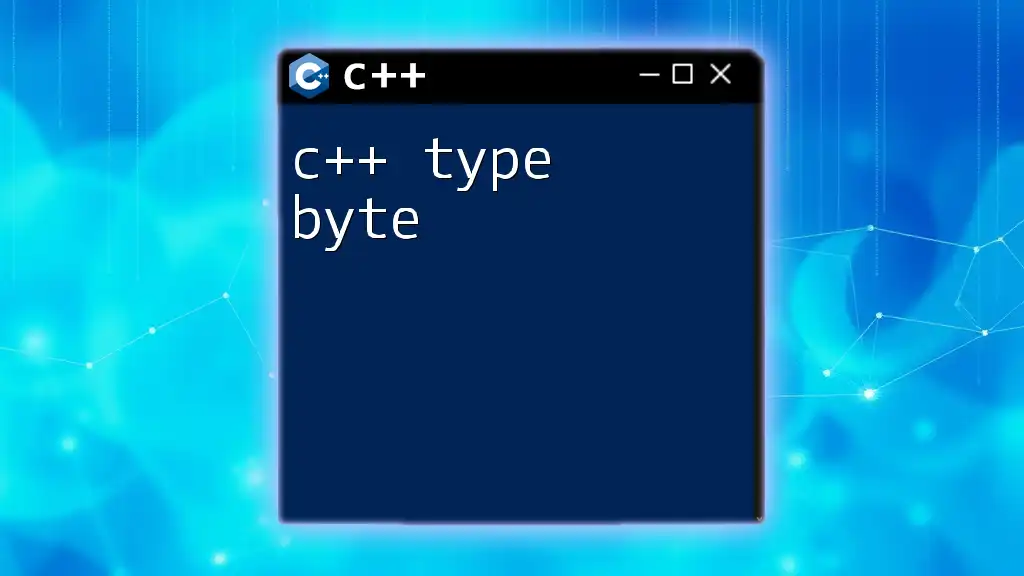
Conclusion
The importance of C++ type traits in programming cannot be overstated. They enhance the safety, flexibility, and readability of code, allowing for robust generic programming. By leveraging these tools, developers can master complex type interactions, leading to cleaner and more efficient software solutions. Experimenting with type traits in your projects will deepen your understanding and enable you to harness their full power.
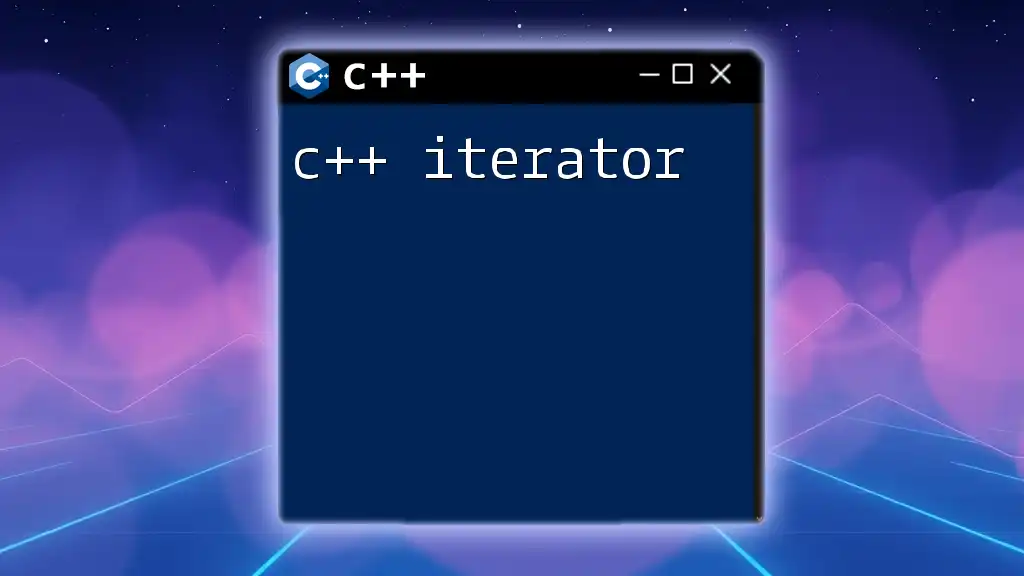
Additional Resources
Recommended Reading
For a deeper understanding of type traits in C++, consider exploring foundational texts on C++ programming and advanced template metaprogramming techniques.
Online Communities and Forums
Engaging with communities and forums dedicated to C++ development can provide insights and alternative perspectives on using type traits effectively.