In C++, a type variable is a declaration that specifies the data type of a variable, thereby defining the size and type of values it can hold.
Here’s a simple example:
int age = 25; // 'age' is a variable of type int.
Understanding Variables in C++
What is a Variable?
A variable in programming is a symbolic name associated with a value and whose associated value can change. In C++, variables are fundamental as they allow programmers to store data in memory, manipulate it, and retrieve it when needed. They serve as placeholders for data throughout a program, helping to easily manage and interact with user inputs, calculations, and other data-driven aspects.
Anatomy of a C++ Variable
A variable in C++ consists of three essential components: declaration, initialization, and assignment.
-
Declaration is where you specify the variable's type and its name. For example:
int age; // Declaration of variable 'age' of type 'int'
-
Initialization is assigning the first value to the variable during its declaration:
int age = 30; // Initialization of variable 'age' with value 30
-
Assignment is modifying the existing value of the variable later in the program:
age = 31; // Assignment, changing the value of 'age' to 31
Understanding these components is crucial, as they dictate how data is managed within a C++ program.
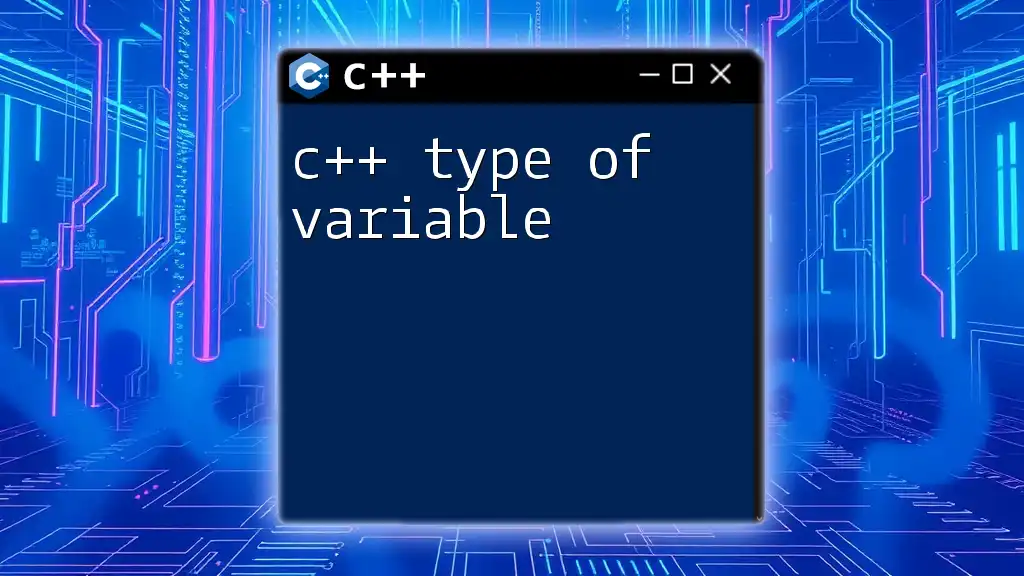
C++ Variable Types
Fundamental Data Types
Integral Types
Integral types are used for representing whole numbers. C++ provides several variants:
- `int`: A standard integer type.
- `short`: A smaller integer type, typically 16 bits.
- `long`: A larger integer type, often 32 bits or more.
Examples of integral type usage are as follows:
int count = 10; // 'count' is an integer variable with value 10
short age = 25; // 'age' is a short integer variable with value 25
long distance = 100000L; // 'distance' is a long integer variable set to 100,000
By understanding integral types, you can choose the right type based on memory and size requirements.
Floating-point Types
Floating-point types are used to represent numbers with fractional parts. The most commonly used types are:
- `float`: Typically a single-precision 32-bit floating-point.
- `double`: A double-precision 64-bit floating-point for greater precision.
These types are essential for computations that require decimals. Example usage:
float temperature = 36.6f; // 'temperature' as a float
double pi = 3.14159; // 'pi' as a double
Selecting the correct floating-point type ensures precision in calculations, especially with scientific data.
Character Types
Character types are used to store single characters. C++ provides the `char` type, which can be declared as follows:
char grade = 'A'; // 'grade' contains a character value
Character types are vital for string manipulations and representing textual data within programming.
Derived Data Types
Arrays
Arrays allow the storage of multiple variables of the same type. They are useful for handling groups of data efficiently. Here’s how you can declare and initialize an integer array:
int scores[5] = {90, 85, 80, 95, 88}; // 'scores' is an array of integers
Using arrays enables concise management of datasets and simplifies iteration.
Pointers
A pointer is a variable that stores the address of another variable. Pointers are powerful in C++, allowing for dynamic memory management and direct variable manipulation. Here’s an example:
int age = 30;
int* p = &age; // 'p' stores the memory address of 'age'
Understanding pointers is crucial for advanced C++ programming, as they provide powerful tools for memory management and manipulation.
Functions
In C++, functions can also be treated as user-defined data types. They perform actions and can return a value, such as integers, floats, or higher data types. Example:
int add(int a, int b) {
return a + b; // Function returning the sum of two integers
}
This abstraction allows for modular coding, enabling better organization and code reuse in programming.
User-defined Data Types
Structures
Structures are user-defined types that allow grouping of different data types. They are useful for creating complex data structures. Here’s an example of a structure representing a person:
struct Person {
string name;
int age;
};
Structures facilitate better data organization, making them relevant in various applications.
Unions
Unions allow storing different data types in the same memory space, optimizing memory usage. Only one of the members can hold a value at a time. Here’s how it looks:
union Data {
int intValue;
float floatValue;
};
Unions are especially useful in situations where memory conservation is paramount, such as in embedded systems.
Enumerations
Enumerations define a variable that can hold a set of predefined constants, making your code clearer and less error-prone. For example:
enum Color { Red, Green, Blue }; // Enum type for colors
Using enums enhances code readability and maintainability by providing context to variable values.
Type Modifiers
Sign Modifiers
C++ includes sign modifiers like `signed` and `unsigned`, which specify whether a type can hold negative values. For example:
unsigned int positive_number = 42; // 'positive_number' can only hold non-negative values
Size Modifiers
Size modifiers, such as `long` and `short`, alter the size of the base data types, allowing for flexibility in variable size. Example:
long long bigNumber = 9223372036854775807LL; // 'bigNumber' as a long long integer
Selecting the correct modifiers helps optimize both performance and memory usage.
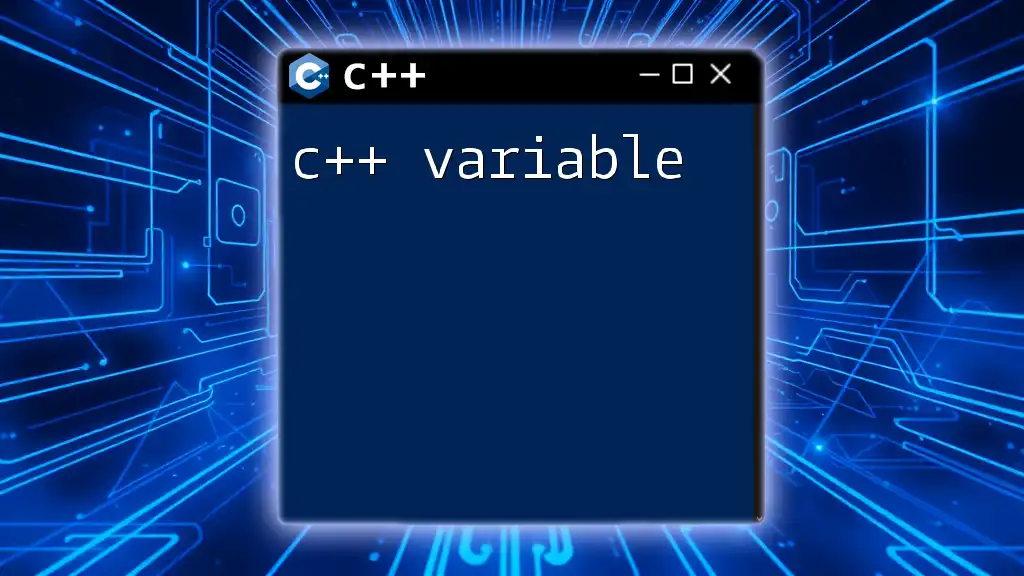
Best Practices for Choosing Variable Types
Choosing the Right Variable Type
When declaring a variable, always choose the most appropriate type based on the nature of the data it will hold. Consider factors such as the expected range of values, memory consumption, and performance. For example, if you need a whole number that won’t exceed 100, a `short` type might be more efficient than an `int`.
Naming Conventions
Clear and descriptive variable names aid in understanding your code at first glance. Avoid ambiguous names. Instead of using names like `x` or `temp`, opt for names like `userAge` or `totalDistance`. This practice enhances code maintainability and collaboration.
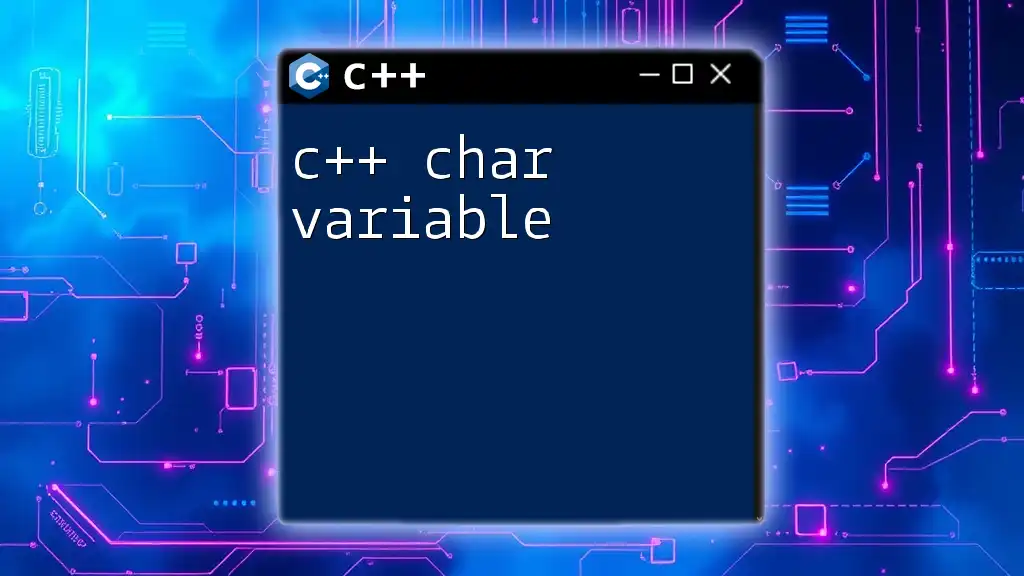
Practical Examples
Example 1: Simple C++ Program Demonstrating Variable Types
Here’s a basic C++ program that showcases different variable types:
#include <iostream>
using namespace std;
int main() {
int myAge = 25;
float myHeight = 5.9;
char initial = 'A';
cout << "Age: " << myAge << ", Height: " << myHeight << ", Initial: " << initial << endl;
return 0;
}
This snippet illustrates how to declare and output values of different variable types within a program.
Example 2: Using Structures and Enums
Here’s a more complex program that employs structures and enumerations:
#include <iostream>
using namespace std;
enum ClassType { Math, Science, History };
struct Student {
string name;
ClassType favoriteClass;
};
int main() {
Student student1 = {"Alice", Math};
cout << student1.name << "'s favorite class is " << student1.favoriteClass << endl;
return 0;
}
In this example, the structure organizes related data, and the enumeration enhances clarity regarding the student's preferred class.
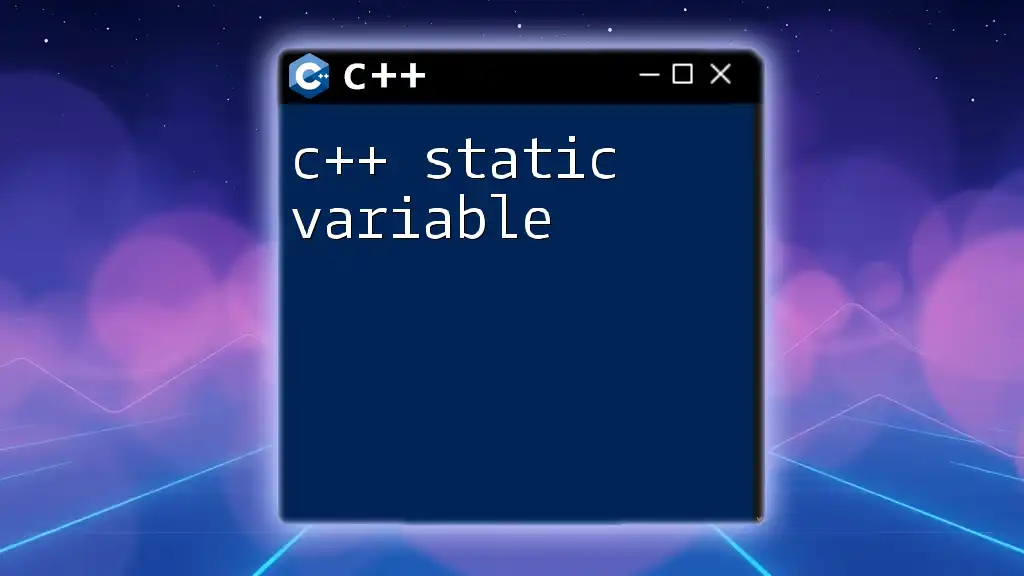
Conclusion
Understanding C++ type variables is foundational for effective programming in C++. By mastering the various types of variables, including fundamental and derived types, as well as user-defined types, programmers can write efficient, clear, and maintainable code. With practice and attention to best practices, you will harness the full power of C++ variable types in your applications.
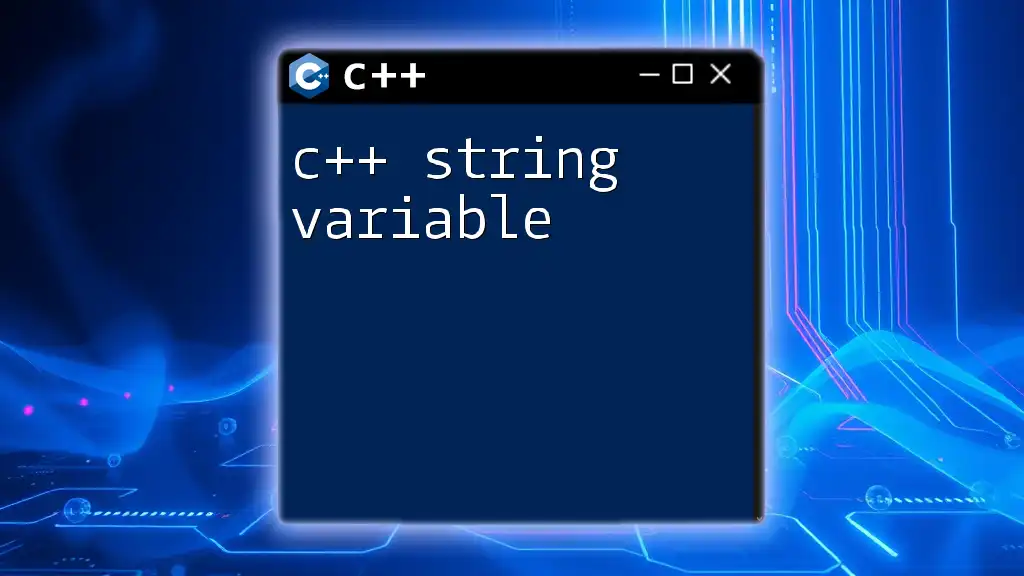
Additional Resources
For further exploration, consider books such as C++ Primer or The C++ Programming Language. Online resources include forums like Stack Overflow or programming-specific communities which foster learning and collaboration in C++.
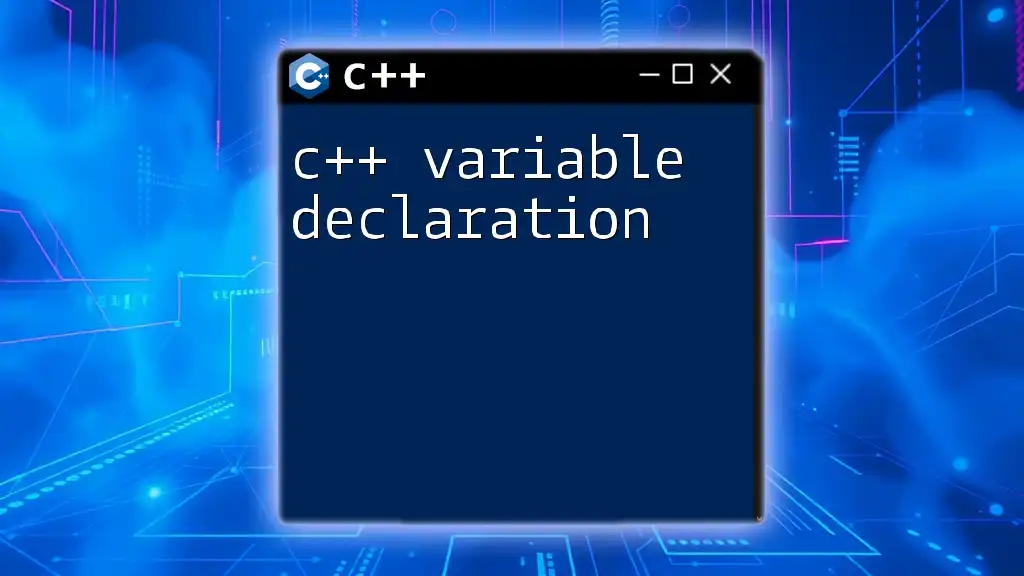
Call to Action
We invite you to share your experiences with C++ variable types. Feel free to practice coding and experimenting with different variable types. Engage in challenges on our platform to solidify your understanding and skills!